Using Pandas to Drop Duplicates: A Detailed Walkthrough
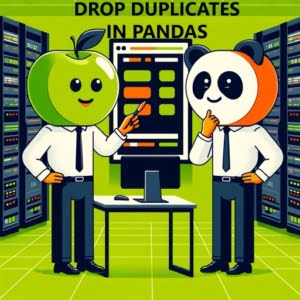
Data cleansing is a critical step in data preprocessing tasks on our servers at IOFLOOD. The pandas drop duplicates function simplifies this process by enabling users to remove duplicate rows from a DataFrame. Join us as we explore how to drop duplicates in Pandas, providing practical examples and strategies for customers ensuring data integrity on our dedicated cloud services.
In this guide, we’ll walk you through the use of drop_duplicates() in Python’s pandas library. Whether you’re a beginner just starting out or an experienced data scientist looking for a refresher, we’ve got you covered.
Ready to master drop_duplicates()? Let’s dive in and start eliminating those pesky duplicates.
TL;DR: How to Drop Duplicates in Pandas?
The
drop_duplicates()
function in pandas is your go-to tool for DataFrame manipulation. It is used with the syntax,df = df.drop_duplicates()
. Here’s a simple example:
import pandas as pd
# Creating a pandas DataFrame
df = pd.DataFrame({'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'], 'B': ['one', 'one', 'two', 'three', 'two', 'two', 'one', 'three']})
# Using drop_duplicates() function
df = df.drop_duplicates()
print(df)
# Output:
# A B
# 0 foo one
# 1 bar one
# 2 foo two
# 3 bar three
In this example, we created a DataFrame with some duplicate rows. By calling df.drop_duplicates(), we were able to remove these duplicates, ensuring each row in our DataFrame is unique. The result is a cleaner, more reliable dataset.
This is just the beginning. Continue reading for a deeper dive into the drop_duplicates() function, including more detailed examples and advanced usage scenarios.
Table of Contents
Basic Uses: Pandas drop_duplicates()
The drop_duplicates() function is a simple yet powerful tool in the pandas library. It’s designed to remove duplicate rows from your DataFrame, which can be essential for accurate data analysis. Here’s how it works:
import pandas as pd
# Creating a DataFrame with duplicate rows
df = pd.DataFrame({'A': ['foo', 'foo', 'bar', 'bar'], 'B': ['one', 'one', 'two', 'two']})
print('Before dropping duplicates:')
print(df)
# Using drop_duplicates()
df = df.drop_duplicates()
print('
After dropping duplicates:')
print(df)
# Output:
# Before dropping duplicates:
# A B
# 0 foo one
# 1 foo one
# 2 bar two
# 3 bar two
#
# After dropping duplicates:
# A B
# 0 foo one
# 2 bar two
In this example, we created a DataFrame with duplicate rows. By calling df.drop_duplicates(), we were able to remove these duplicates, ensuring each row in our DataFrame is unique.
Advantages of drop_duplicates()
The drop_duplicates() function is quick, efficient, and easy to use. It can handle large datasets and is flexible enough to be tailored to your specific needs. This makes it an essential tool for any data scientist working with pandas.
Potential Pitfalls
While drop_duplicates() is a powerful tool, it’s also important to understand its limitations. By default, it considers all columns of the DataFrame when identifying duplicates. This means that if two rows are identical across all columns, one will be dropped. However, if you only want to consider certain columns, you’ll need to specify this (we’ll cover this in the ‘Advanced Use’ section).
Another potential pitfall is that drop_duplicates() does not modify the original DataFrame. Instead, it returns a new DataFrame where the duplicates have been dropped. If you want to modify the original DataFrame, you’ll need to use the ‘inplace’ parameter (also covered in the ‘Advanced Use’ section).
Advanced Use: Function Parameters
The drop_duplicates() function is customizable and allows you to specify certain parameters to better suit your needs. Let’s delve deeper into three key parameters: subset
, keep
, and inplace
.
Subset Selection with drop_duplicates()
The subset
parameter allows you to specify the columns you want to consider when identifying duplicates. This is useful when you only want to consider certain columns for duplication. Let’s see it in action:
import pandas as pd
# Creating a DataFrame with duplicate rows in 'A'
df = pd.DataFrame({'A': ['foo', 'foo', 'bar', 'bar'], 'B': ['one', 'two', 'three', 'four']})
print('Before dropping duplicates:')
print(df)
# Using drop_duplicates() with subset
df = df.drop_duplicates(subset=['A'])
print('
After dropping duplicates:')
print(df)
# Output:
# Before dropping duplicates:
# A B
# 0 foo one
# 1 foo two
# 2 bar three
# 3 bar four
#
# After dropping duplicates:
# A B
# 0 foo one
# 2 bar three
In this example, we specified the subset as [‘A’], so the function only considered column ‘A’ when identifying duplicates. As a result, the second ‘foo’ row was dropped, even though its ‘B’ value was unique.
The Keep Parameter
The keep
parameter lets you decide which duplicate to keep. By default, it’s set to ‘first’, meaning the first occurrence is kept and the rest are dropped. If set to ‘last’, the last occurrence is kept. If set to False, all duplicates are dropped. Let’s see how this works:
import pandas as pd
# Creating a DataFrame with duplicate rows
df = pd.DataFrame({'A': ['foo', 'foo', 'bar', 'bar'], 'B': ['one', 'two', 'three', 'four']})
print('Before dropping duplicates:')
print(df)
# Using drop_duplicates() with keep
df = df.drop_duplicates(keep='last')
print('
After dropping duplicates:')
print(df)
# Output:
# Before dropping duplicates:
# A B
# 0 foo one
# 1 foo two
# 2 bar three
# 3 bar four
#
# After dropping duplicates:
# A B
# 1 foo two
# 3 bar four
In this example, by setting keep=’last’, the function kept the last occurrence of each duplicate and dropped the rest.
Modifying the Original DataFrame with Inplace
By default, drop_duplicates() returns a new DataFrame and leaves the original one unchanged. If you want to modify the original DataFrame, you need to set the inplace
parameter to True. Here’s an example:
import pandas as pd
# Creating a DataFrame with duplicate rows
df = pd.DataFrame({'A': ['foo', 'foo', 'bar', 'bar'], 'B': ['one', 'two', 'three', 'four']})
print('Before dropping duplicates:')
print(df)
# Using drop_duplicates() with inplace
df.drop_duplicates(inplace=True)
print('
After dropping duplicates:')
print(df)
# Output:
# Before dropping duplicates:
# A B
# 0 foo one
# 1 foo two
# 2 bar three
# 3 bar four
#
# After dropping duplicates:
# A B
# 0 foo one
# 2 bar three
In this example, by setting inplace=True, the function modified the original DataFrame and did not return a new one.
By understanding and effectively using these parameters, you can make the drop_duplicates() function work more efficiently for your specific needs.
Alternate Duplicate Removal Methods
While drop_duplicates() is a powerful tool, it’s not the only method available for removing duplicates in pandas. Let’s explore some alternative approaches, such as using the duplicated() function along with boolean indexing.
The duplicated() Function and Boolean Indexing
The duplicated() function returns a Boolean Series indicating whether each row is a duplicate or not. You can pair this with boolean indexing to filter out the duplicates. Here’s an example:
import pandas as pd
# Creating a DataFrame with duplicate rows
df = pd.DataFrame({'A': ['foo', 'foo', 'bar', 'bar'], 'B': ['one', 'two', 'three', 'four']})
print('Before dropping duplicates:')
print(df)
# Using duplicated() with boolean indexing
df = df[~df.duplicated()]
print('
After dropping duplicates:')
print(df)
# Output:
# Before dropping duplicates:
# A B
# 0 foo one
# 1 foo two
# 2 bar three
# 3 bar four
#
# After dropping duplicates:
# A B
# 0 foo one
# 2 bar three
In this example, df.duplicated() returned a Boolean Series where True indicates a duplicate row. We then used the ~ operator to flip these True/False values, and used this to index our DataFrame. The result is a DataFrame without duplicates.
Comparison: drop_duplicates() vs. duplicated()
Both methods can effectively remove duplicates, but there are some differences:
Method | Advantages | Disadvantages |
---|---|---|
drop_duplicates() | Easy to use, customizable with parameters | Creates a new DataFrame by default |
duplicated() with boolean indexing | Allows more control, modifies the original DataFrame | More complex, requires understanding of boolean indexing |
While drop_duplicates() is simpler and more straightforward, using duplicated() with boolean indexing can give you more control over the process. Which one to use depends on your specific needs and level of comfort with pandas.
Troubleshooting drop_duplicates()
While pandas’ drop_duplicates() function is an incredibly useful tool, you might encounter some issues along the way. Let’s discuss some common problems and their solutions.
Memory Errors with Large Datasets
When working with large datasets, you might encounter memory errors. This is because drop_duplicates() needs to create a new DataFrame, which can be memory-intensive. One way to mitigate this is by processing your DataFrame in chunks. Here’s an example:
import pandas as pd
# Assuming df is your large DataFrame
# Split df into chunks
chunks = [df[i:i + 10000] for i in range(0, df.shape[0], 10000)]
# Process each chunk
for chunk in chunks:
chunk.drop_duplicates(inplace=True)
# Concatenate the chunks back into a single DataFrame
df = pd.concat(chunks)
In this example, we first split the DataFrame into chunks of 10,000 rows. We then processed each chunk separately, and finally concatenated them back into a single DataFrame. This can significantly reduce the memory usage of drop_duplicates().
Handling Large Datasets: Efficiency Considerations
Another issue with large datasets is efficiency. drop_duplicates() can be slow with large datasets. One way to improve efficiency is by using the subset parameter to only consider certain columns. This can significantly reduce the computation time.
import pandas as pd
# Assuming df is your large DataFrame
# Use subset to only consider certain columns
df.drop_duplicates(subset=['A', 'B'], inplace=True)
In this example, we used the subset parameter to only consider columns ‘A’ and ‘B’ when identifying duplicates. This can make drop_duplicates() much faster with large datasets.
By understanding these potential issues and their solutions, you can use drop_duplicates() more effectively and efficiently.
Fundamentals of Pandas DataFrame
At the heart of data analysis with pandas is the DataFrame – a two-dimensional, size-mutable, potentially heterogeneous tabular data structure. Think of it as a spreadsheet with rows and columns, where each column can be of a different datatype (numeric, string, boolean, etc.).
import pandas as pd
# Creating a DataFrame
df = pd.DataFrame({'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'three', 'two', 'two', 'one', 'three']})
print(df)
# Output:
# A B
# 0 foo one
# 1 bar one
# 2 foo two
# 3 bar three
# 4 foo two
# 5 bar two
# 6 foo one
# 7 foo three
In this example, we created a DataFrame from a dictionary. Each key-value pair in the dictionary corresponds to a column in the DataFrame. The DataFrame’s tabular structure makes it easy to manipulate and analyze data.
DataFrame Management with drop_duplicates()
Data cleaning is a crucial step in data analysis. Dirty or messy data can lead to inaccurate results and conclusions. One common issue is duplicate data – rows that are repeated in your DataFrame. These duplicates can skew your analysis, leading to misleading results.
That’s where the drop_duplicates() function comes in. By removing duplicates, it ensures your analysis is based on unique, reliable data. Whether you’re calculating the mean of a column or plotting a graph, using drop_duplicates() can help you ensure the accuracy of your results.
Use Cases of drop_duplicates()
The drop_duplicates() function is more than just a tool for cleaning data – it’s a crucial part of data preprocessing, especially in fields like machine learning and data visualization.
drop_duplicates() in Machine Learning
In machine learning, preprocessing is a critical step. It involves cleaning and transforming raw data into a format that can be fed into a machine learning model. drop_duplicates() plays a vital role in this process, ensuring that the training data fed into the model is unique and reliable.
Data Visualization and drop_duplicates()
In data visualization, duplicate data can lead to skewed or misleading visualizations. By using drop_duplicates(), you can ensure that your visualizations accurately represent the unique data points in your DataFrame.
import pandas as pd
import matplotlib.pyplot as plt
# Creating a DataFrame with duplicate rows
df = pd.DataFrame({'A': ['foo', 'foo', 'bar', 'bar'], 'B': [1, 1, 2, 2]})
# Dropping duplicates
df = df.drop_duplicates()
# Plotting the data
plt.bar(df['A'], df['B'])
plt.show()
# Output: A bar plot with 'foo' and 'bar' on the x-axis and their corresponding 'B' values on the y-axis
In this example, we first created a DataFrame with duplicate rows. After dropping duplicates, we plotted the data, resulting in an accurate visualization of the unique data points.
Going Further: Data Preprocessing
While drop_duplicates() is an essential tool, data preprocessing involves more than just removing duplicates. Other important concepts include handling missing values, data transformation, and more. Exploring these topics can further enhance your data analysis skills.
Further Resources for Pandas Library
For a deeper dive into pandas and data preprocessing, consider checking out the pandas documentation, online courses like those on Coursera or edX, or books like ‘Python for Data Analysis’ by Wes McKinney.
Here are a few more articles on our blog that you might find helpful:
- Exploring Advanced Features of Pandas for Data Science: Dive deeper into the advanced aspects of Pandas, enhancing your data science skills with this insightful resource.
A Comprehensive Guide to Using the iloc() Function in Pandas: This comprehensive guide explains the usage of the iloc() function in Pandas to perform indexing and slicing operations on a DataFrame in Python.
How to Fill NaN Values in a Pandas DataFrame using the fillna() Function: This tutorial walks you through the process of using the fillna() function in Pandas to replace missing or NaN values in a DataFrame with specified values or methods.
Pandas drop_duplicates() Method: Remove Duplicate Rows: This tutorial on w3schools.com explains how to use the drop_duplicates() method in Pandas to remove duplicate rows from a DataFrame.
Pandas drop_duplicates(): Remove Duplicate Rows: This DigitalOcean tutorial provides examples and explanations on using the drop_duplicates() function in Pandas to eliminate duplicate rows from a DataFrame.
Python Pandas DataFrame drop_duplicates(): An article on GeeksforGeeks that demonstrates how to utilize the drop_duplicates() method in Pandas to drop duplicate rows from a DataFrame.
These resources can provide a more comprehensive understanding of data analysis with pandas, including the use of drop_duplicates() and beyond.
Wrap Up: Pandas drop_duplicates()
Throughout this guide, we’ve explored the usage of the drop_duplicates() function in pandas. This function is a powerful tool for data cleaning, allowing you to remove duplicate rows from your DataFrame quickly and efficiently.
The drop_duplicates() function is flexible and customizable. You can specify parameters like subset
, keep
, and inplace
to tailor it to your specific needs. Here’s a quick recap of how to use it:
We also discussed some common issues you might encounter with drop_duplicates(), such as memory errors with large datasets and efficiency concerns. By understanding these issues and their solutions, you can use drop_duplicates() more effectively.
Finally, we looked at some alternative approaches to handle duplicates, such as the duplicated() function and boolean indexing. These methods offer more control and can be more efficient with large datasets.
Method | Advantages | Disadvantages |
---|---|---|
drop_duplicates() | Easy to use, customizable with parameters | Creates a new DataFrame by default |
duplicated() with boolean indexing | Allows more control, modifies the original DataFrame | More complex, requires understanding of boolean indexing |
By mastering drop_duplicates() and understanding its alternatives, you can ensure your pandas DataFrame is clean and reliable, leading to more accurate and effective data analysis. Remember, data cleaning is a crucial step in data preprocessing, and drop_duplicates() is an essential tool in your data analysis toolkit.