Python Append Function | List Manipulation Guide
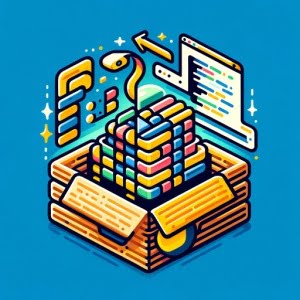
The append function in Python is a fundamental tool for adding elements to lists. Here at IOFLOOD, we often utilize this technique in our scripts when we require dynamic data manipulation. This article shares our techniques and best practices for using the append function in Python, helping our dedicated cloud server hosting customers manage their data more effectively.
This guide is designed to walk you through the ins and outs of the append function in Python. We’ll start with the basics, gradually moving to more advanced uses, and even explore some alternative approaches. Whether you’re a beginner just starting out or an intermediate coder looking to brush up your skills, this guide has got you covered.
So, let’s dive in and master the Python append function together.
TL;DR: How Do I Use the Append Function in Python?
To add an item to a list in Python, you use the
append()
function and the syntax,my_list.append(value)
. Here’s a simple example:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list)
# Output:
# [1, 2, 3, 4]
In this example, we created a list named my_list
with three elements. We then used the append()
function to add the number 4 to the end of the list. When we print the list, we can see that it now includes the number 4. This is the basic usage of the append()
function in Python.
If you’re interested in more detailed usage and advanced scenarios, keep reading. We’ll dive deeper into the Python append function, exploring its potential and how you can leverage it to manipulate lists effectively.
Table of Contents
The Basics: Python Append
The append()
function in Python is a built-in method for the list data type. It allows you to add an item to the end of a list. Here’s how it works:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Use append to add an item
fruits.append('dragonfruit')
print(fruits)
# Output:
# ['apple', 'banana', 'cherry', 'dragonfruit']
In this example, we started with a list of fruits. We then used the append()
function to add ‘dragonfruit’ to the end of the list. When we print the list, we see that ‘dragonfruit’ has been added to the end.
One of the main advantages of the append()
function is its simplicity. With just one line of code, you can add an item to a list. However, the append()
function only adds one item at a time. If you try to append multiple items at once, it won’t work as you might expect. Additionally, the append()
function modifies the original list. It doesn’t create a new list. This is because lists are ‘mutable’ in Python, meaning they can be changed after they are created.
Advanced Usage with Python Append
Let’s now explore some more complex uses of the Python append function. A common scenario is when you want to append multiple items or another list to your existing list. Let’s see how the append()
function behaves in these cases.
Appending Multiple Items
If you try to append multiple items at once using append()
, you might not get the result you expect. Here’s an example:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Try to append multiple items
fruits.append('dragonfruit', 'elderberry')
# Output:
# TypeError: append() takes exactly one argument (2 given)
As you can see, trying to append multiple items at once throws a TypeError. This is because the append()
function only takes a single argument.
Appending Another List
What happens if you try to append another list? Let’s find out:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Create another list
more_fruits = ['dragonfruit', 'elderberry']
# Append the second list
fruits.append(more_fruits)
print(fruits)
# Output:
# ['apple', 'banana', 'cherry', ['dragonfruit', 'elderberry']]
In this case, the entire second list is appended as a single item, creating a sublist within the original list.
These examples illustrate that while the append()
function is powerful and useful, it has its limitations. Understanding these will help you use the function more effectively in your Python coding.
When a sublist exists within an original list, this is called a Nested List in Python. To find out to handle these, you can refer to this article.
Alternatives Methods to append()
While the append()
function is a powerful tool for list manipulation in Python, it is not the only one. There are other methods you can use to add items to a list, each with its own advantages and disadvantages. Let’s explore two of these alternatives: the extend()
function and list concatenation.
Using Python’s Extend Function
The extend()
function is similar to append()
, but instead of adding a single item, it adds all elements of an iterable (like a list or a tuple) to the end of a list. Here’s an example:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Create another list
more_fruits = ['dragonfruit', 'elderberry']
# Use extend to add the elements of the second list
fruits.extend(more_fruits)
print(fruits)
# Output:
# ['apple', 'banana', 'cherry', 'dragonfruit', 'elderberry']
As you can see, extend()
adds each element of the second list individually, not as a sublist. This is a key difference from append()
and can be very useful when you want to combine lists.
List Concatenation in Python
Another way to add items to a list is through list concatenation, which involves using the +
operator to join two lists together. Here’s how it works:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Create another list
more_fruits = ['dragonfruit', 'elderberry']
# Concatenate the lists
all_fruits = fruits + more_fruits
print(all_fruits)
# Output:
# ['apple', 'banana', 'cherry', 'dragonfruit', 'elderberry']
List concatenation also adds the elements of the second list individually. However, unlike extend()
, it creates a new list and leaves the original lists unchanged. This can be advantageous if you need to preserve the original lists for later use.
Both extend()
and list concatenation offer more flexibility than append()
in certain scenarios. However, they also have their own limitations and may not be suitable for all use cases. As with any tool, the key is to understand how they work and when to use them. With this knowledge, you can choose the method that best fits your needs in any given situation.
Solving Errors with append() Method
While the append()
function is generally straightforward to use, you might encounter some issues along the way. Let’s discuss a couple of common problems and their solutions.
TypeError: append() takes exactly one argument
As we’ve seen earlier, trying to append multiple items at once results in a TypeError. Here’s a reminder of what that looks like:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Try to append multiple items
fruits.append('dragonfruit', 'elderberry')
# Output:
# TypeError: append() takes exactly one argument (2 given)
The solution here is to either use append()
multiple times to add each item individually or use the extend()
function if you have an iterable of items to add.
Memory Issues with Large Lists
Another potential issue is memory consumption when you’re dealing with large lists. Each call to append()
can potentially resize the list and allocate new memory, which can lead to inefficiency and high memory usage for very large lists.
One workaround for this issue is to use list comprehensions or generators, which can be more memory-efficient for large data sets. We’ll touch on these concepts in the ‘Beyond’ section.
Remember, understanding the potential pitfalls and how to navigate them is just as important as understanding how to use a function. With these troubleshooting tips and considerations in mind, you’ll be well-equipped to use the Python append function effectively and efficiently.
Working with Lists: Python Append
Before delving deeper into the usage of the append()
function, it’s crucial to understand the basics of Python’s list data type and how it interacts with the append()
function.
A list in Python is a collection of items that are ordered and changeable, or in more technical terms, mutable. This means that once a list is created, you can add, remove, or change items in the list.
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Change the second item
fruits[1] = 'blueberry'
print(fruits)
# Output:
# ['apple', 'blueberry', 'cherry']
In this example, we created a list of fruits and then changed the second item (‘banana’) to ‘blueberry’. When we print the list, we can see that the list has been updated with the new item.
Mutable vs Immutable in Python
The ability to change a list after it’s been created is a characteristic of mutable objects in Python. In contrast, some objects in Python are immutable, meaning they cannot be changed after they’re created. Examples of immutable objects include strings and tuples.
Understanding the difference between mutable and immutable objects is important when working with the append()
function because it directly modifies the list. This is in contrast to some other functions or methods that return a new object and leave the original object unchanged.
With this foundational understanding of Python lists and the concept of mutability, you’re now better equipped to understand and use the Python append()
function effectively.
Going Beyond Python Append
The append()
function, while simple, plays a significant role in Python programming. It’s a fundamental tool for list manipulation, which is a common task in many scripts and projects. As you progress in your Python journey and start working on larger and more complex projects, you’ll find append()
invaluable.
Exploring Related Concepts
Once you’ve mastered the append()
function, there are several related concepts that you might find interesting. For instance, list comprehension is a powerful Python feature that allows you to create new lists based on existing ones in a concise and readable way.
Generators, on the other hand, are a type of iterable in Python. They’re a bit more advanced than lists and have some unique characteristics that make them particularly useful in certain scenarios, such as working with large data sets.
Further Resources for Python List Mastery
To deepen your understanding of the append()
function and related concepts, here are some additional resources you might find helpful:
- Enhancing Your Python Skills with List Manipulations: Level up your Python skills by learning various list manipulation techniques, enabling you to write more efficient and effective code.
Python Lists and List Manipulation: A comprehensive guide to lists in Python, including how to manipulate them using functions like
append()
.Python List Comprehension Tutorial: An in-depth tutorial on list comprehension in Python, a powerful tool for creating and manipulating lists.
Python Generators Explained: A detailed explanation of generators in Python, including how they differ from lists and when to use them.
Joining a List into a String in Python: This tutorial explores various methods to join the elements of a list into a string in Python.
Guide on Joining a List in Python: This guide by IOFlood provides an overview of different ways to join elements of a list in Python.
These resources should give you a solid foundation in list manipulation in Python and help you take your skills to the next level.
Wrap Up: Python’s Append Function
In this guide, we’ve taken a deep dive into the append()
function in Python.
We’ve explored its basic usage, learned how to add items to a list, and discovered the nuances of list manipulation in Python. We’ve also tackled some common issues you might encounter when using append()
, such as TypeError
and memory concerns with large lists, and provided strategies to handle them effectively.
We didn’t stop at append()
, though. We ventured into alternative methods like extend()
and list concatenation, each with its own strengths and caveats. Understanding these alternatives equips you with more tools in your Python toolkit and gives you the flexibility to choose the best method for your specific needs.
To summarize, here’s a quick comparison of the methods we discussed:
Method | Use | Pros | Cons |
---|---|---|---|
append() | Add a single item to a list | Simple and easy to use | Can only add one item at a time |
extend() | Add multiple items or another list to a list | Adds items individually, not as a sublist | Can be less intuitive than append() |
List Concatenation | Combine two lists | Creates a new list, leaving the original lists unchanged | Can be less efficient for large lists |
Remember, the append()
function is a fundamental part of Python’s list manipulation capabilities. Whether you’re a beginner just starting out or an intermediate coder looking to brush up your skills, mastering append()
and related functions is a significant step in your Python journey.
Keep practicing, keep exploring, and you’ll be a Python list pro in no time!