Python Join List to String: Step-by-Step Guide
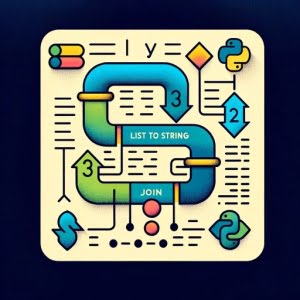
Converting lists to strings in Python has been a common operation for us at IOFLOOD while working to improve our data handling software. This process combines list elements into a single string, enhancing data readability. Today’s article delves into the methods for joining a list to a string in Python, providing practical examples to assist our customers in handling data scripting on their dedicated server configurations.
This guide will walk you through the steps to use Python’s join()
function to achieve this. We’ll explore the join()
function’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Python’s join()
function!
TL;DR: How Do I Join a List to a String in Python?
To join a list to a string in Python, you use the
join()
function likestring = ' '.join(list)
. This function allows you to seamlessly combine elements of a list into a single string.
Here’s a simple example:
list = ['Hello', 'World']
string = ' '.join(list)
print(string)
# Output:
# 'Hello World'
In this example, we’ve used the join()
function to combine the elements of the list into a single string. The space character ' '
is used as the separator between the elements. The result is the string ‘Hello World’.
This is just a basic usage of the
join()
function in Python. There’s much more to learn about this function, including its advanced features and common issues. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Understanding Python’s join()
Function
Python’s join()
function is a string method that concatenates any number of string elements, with a specified string delimiter. The syntax of the join()
function is as follows:
str.join(iterable)
In this syntax, str
is the separator that you want to use for joining the elements of the iterable (like a list). The iterable can be any collection of strings.
Let’s look at a simple example of the join()
function:
list = ['I', 'am', 'learning', 'Python']
string = ' '.join(list)
print(string)
# Output:
# 'I am learning Python'
In this example, we’ve used the join()
function to combine the elements of the list into a single string. The space character ' '
is used as the separator between the elements. The result is the string ‘I am learning Python’.
Advantages and Potential Pitfalls of join()
The join()
function is a powerful tool in Python. It allows you to combine elements of a list into a single string in a fast, efficient way. However, it’s important to note that the join()
function can only join strings. If the list contains non-string elements, the join()
function will raise a TypeError.
Here’s an example:
list = ['Hello', 123]
string = ' '.join(list)
# Output:
# TypeError: sequence item 1: expected str instance, int found
In this example, the list contains an integer, which causes the join()
function to raise a TypeError. To avoid this error, you need to ensure that all elements of the list are strings before using the join()
function.
Handling Different List Types
Python’s join()
function is flexible and can handle different types of lists. However, remember that it can only join strings. If your list contains non-string elements, such as integers or None values, you need to convert them to strings before using the join()
function.
Joining a List of Integers
If you have a list of integers, you can use the map()
function to convert the integers to strings. Here’s an example:
list = [1, 2, 3, 4, 5]
string = ' '.join(map(str, list))
print(string)
# Output:
# '1 2 3 4 5'
In this example, the map()
function applies the str()
function to each element of the list, converting the integers to strings. Then, the join()
function combines these string elements into a single string.
Handling Lists with None Values
If your list contains None values, you can use a list comprehension to remove the None values or convert them to a string. Here’s an example:
list = ['Hello', None, 'World']
string = ' '.join([str(i) for i in list if i is not None])
print(string)
# Output:
# 'Hello World'
In this example, the list comprehension [str(i) for i in list if i is not None]
generates a new list that excludes the None values. The join()
function then combines the elements of this new list into a single string.
Alternate Methods: Join Lists to Strings
As we saw above, there are several alternatives to the join()
function for combining elements of a list into a single string. These methods can be useful in different scenarios, depending on the structure and content of your list.
Comparing the Methods
Method | Advantages | Disadvantages |
---|---|---|
join() | Fast, efficient, easy to use | Can only join strings |
List comprehension | Flexible, can handle complex conditions | Can be slower for large lists |
map() function | Fast, efficient for large lists | Less intuitive for beginners |
In conclusion, the best method to join a list to a string in Python depends on your specific needs. If you’re dealing with a list of strings, the join()
function is the easiest and most efficient method. If you need to convert the elements of the list to strings, you can use list comprehension or the map()
function, depending on the complexity of your conditions and the size of your list.
Debugging: Combining List Elements
While Python’s join()
function is a powerful tool for combining elements of a list into a single string, you may encounter some issues when using it. Let’s discuss some of the common issues and how to solve them.
Handling ‘TypeError’
One common issue is the ‘TypeError’, which occurs when you try to join a list that contains non-string elements. Here’s an example:
list = ['Hello', 123]
string = ' '.join(list)
# Output:
# TypeError: sequence item 1: expected str instance, int found
In this example, the list contains an integer, which causes the join()
function to raise a TypeError. To solve this issue, you need to convert all elements of the list to strings before using the join()
function. You can do this using the map()
function or a list comprehension, as discussed in the previous sections.
Dealing with Different List Types
Another common issue is dealing with different types of lists, such as lists of integers or lists with None values. As we’ve discussed, you need to convert the elements of these lists to strings before using the join()
function.
Remember that the join()
function is a string method, so it expects all elements of the list to be strings. If your list contains non-string elements, you need to convert them to strings before using the join()
function.
Understanding Python Data Types
Before we delve into the join()
function, it’s essential to understand Python’s list and string data types, as they form the foundation of the join()
operation.
Python Lists
In Python, a list is a collection of items that are ordered and changeable. Lists allow duplicate items and can contain different data types. Here’s an example of a Python list:
list = ['apple', 'banana', 'cherry']
print(list)
# Output:
# ['apple', 'banana', 'cherry']
In this example, the list contains three string elements: ‘apple’, ‘banana’, and ‘cherry’.
Python Strings
A string in Python is a sequence of characters. Python treats single quotes the same as double quotes. Here’s an example of a Python string:
string = 'Hello, World!'
print(string)
# Output:
# 'Hello, World!'
In this example, the string is ‘Hello, World!’.
Practical Uses: Joining Lists to Strings
Python’s join()
function is not just a tool for combining strings. It plays a crucial role in various areas of Python programming, from data manipulation to file handling.
Data Manipulation
When working with data in Python, you often need to convert lists of data into strings for processing or output. The join()
function makes this task easy and efficient, allowing you to seamlessly combine elements of a list into a single string.
File Handling
In file handling, the join()
function is often used to combine elements of a list into a single string for writing to a file. This is especially useful when you need to write a list of data to a CSV file or a text file.
For more info on file handling, we have numerous articles contained within our Python OS Module series, start your journey here!
Learn More: Python Lists and Strings
If you’re interested in learning more about Python’s join()
function, you might also want to explore related concepts like list comprehension and string formatting in Python.
List comprehension is a concise way to create lists in Python, while string formatting allows you to insert variables into a string in a convenient and readable way. These concepts, combined with the join()
function, can greatly enhance your Python programming skills.
Further Resources for Mastering Python’s join()
Function
If you want to deepen your understanding of Python’s join()
function and related concepts, here are some resources that you might find helpful:
- Navigating Python Lists: A Comprehensive Tutorial: An in-depth tutorial that explores the intricacies of Python lists, providing a thorough understanding of this data structure.
Creating a Unique List in Python: This tutorial explores methods to create a unique list in Python, removing duplicate elements.
Appending Elements to a List in Python: A guide demonstrating different approaches, including the
append()
method,extend()
method, list concatenation, and list comprehension, to append elements to a list in Python.Python
join()
Function Documentation: The official Python documentation providing a detailed explanation of thejoin()
function and its usage.Python String Formatting: This tutorial from Real Python provides a comprehensive guide to string formatting in Python, a related concept that you might find useful.
Python List Comprehension: This guide from Programiz explains list comprehension in Python, another related concept that can enhance your use of the
join()
function.
Recap: Python Convert List to String
In this comprehensive guide, we’ve delved into the process of joining lists to strings in Python, using the join()
function. We’ve explored the function’s basic usage, advanced features, and common issues, providing you with solutions and workarounds for each challenge.
We began with the basics, showing you how to use the join()
function to combine elements of a list into a single string. We then ventured into more advanced territory, demonstrating how to handle different types of lists and how to avoid common issues like the ‘TypeError’.
Along the way, we also explored alternative methods for joining lists to strings, such as using list comprehension and the map()
function. We compared these methods and discussed their advantages and disadvantages, giving you a sense of the broader landscape of tools for joining lists to strings in Python.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
join() | Fast, efficient, easy to use | Can only join strings |
List comprehension | Flexible, can handle complex conditions | Can be slower for large lists |
map() function | Fast, efficient for large lists | Less intuitive for beginners |
Whether you’re just starting out with Python’s join()
function or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to join lists to strings in Python and the various methods you can use.
With its balance of speed, efficiency, and flexibility, Python’s join()
function is a powerful tool for any Python programmer. Happy coding!