Joining Lists in Python: A Step-by-Step Tutorial
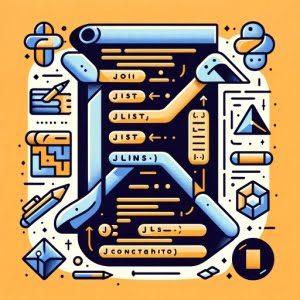
Joining lists in Python is an essential task for consolidating data in our scripts at IOFLOOD. In our experience, this operation ensures consistent merging of multiple lists into one cohesive data set. Today’s article aims to provide valuable insights and examples to assist our dedicated bare metal servers customers.
In this guide, we’ll walk you through the process of joining lists in Python, from the basics to more advanced techniques. We’ll cover everything from the basic +
operator and extend()
function, to more advanced methods like list comprehension and the itertools.chain()
function.
Let’s get started!
TL;DR: How Do I Join Lists in Python?
To join lists in Python, you can use the
+
operator or theextend()
function with the syntax,list1.extend(list2)
. These methods allow you to combine two or more lists into a single list.
Here’s a simple example using the +
operator:
list1 = ['Hello', 'world']
list2 = ['Python', 'is', 'awesome']
joined_list = list1 + list2
print(joined_list)
# Output:
# ['Hello', 'world', 'Python', 'is', 'awesome']
In this example, we have two lists: list1
and list2
. We join them using the +
operator, resulting in a new list joined_list
that contains all elements from both list1
and list2
. The print()
function is then used to display the joined list.
This is a basic way to join lists in Python, but there’s much more to learn about this topic. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Techniques: Python List Joining
Python offers several ways to join lists. For beginners, the simplest methods involve using the +
operator and the extend()
function. Let’s explore these two methods in more detail.
Using the +
Operator to Join Lists
The +
operator in Python can be used to concatenate or join two or more lists. It’s straightforward to use and works similarly to how you would use the +
operator to add numbers.
Here’s an example:
list1 = ['apple', 'banana', 'cherry']
list2 = ['date', 'elderberry', 'fig']
joined_list = list1 + list2
print(joined_list)
# Output:
# ['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig']
In this example, list1
and list2
are joined together using the +
operator. The result is a new list joined_list
that contains all the elements from both list1
and list2
.
One thing to note is that the +
operator does not modify the original lists. Instead, it creates a new list that contains the elements of the joined lists. This can be advantageous if you need to keep the original lists unchanged.
Using the extend()
Function to Join Lists
The extend()
function is another way to join lists in Python. Unlike the +
operator, the extend()
function modifies the original list.
Here’s how you can use the extend()
function:
list1 = ['grape', 'honeydew', 'ice cream']
list2 = ['jelly', 'kiwi', 'lemon']
list1.extend(list2)
print(list1)
# Output:
# ['grape', 'honeydew', 'ice cream', 'jelly', 'kiwi', 'lemon']
In this example, list2
is added to the end of list1
using the extend()
function. Notice that list1
is modified directly, and we don’t need to create a new list.
The extend()
function can be useful when you need to add elements to an existing list without creating a new one. However, be aware that the original list will be changed.
Different Data Types and Joining Lists
Python is a dynamically typed language, meaning a list can contain elements of different types. You might wonder what happens when you join such lists. Let’s explore this scenario.
list1 = ['apple', 100, 15.2]
list2 = ['banana', 200, 30.4]
joined_list = list1 + list2
print(joined_list)
# Output:
# ['apple', 100, 15.2, 'banana', 200, 30.4]
In this example, list1
and list2
contain strings, integers, and floats. When we join these lists using the +
operator, Python doesn’t raise any errors. The elements in the joined list retain their original data types.
This flexibility allows Python to handle lists of mixed types. However, be aware that certain operations might not work as expected with mixed types. For example, trying to sort a list containing both strings and numbers will result in a TypeError.
Advanced Use: Joining Nested Lists
Nested lists, or lists containing other lists, can also be joined using the +
operator or the extend()
function. However, the results might not be what you expect.
list1 = ['apple', ['banana', 'cherry']]
list2 = ['date', ['elderberry', 'fig']]
joined_list = list1 + list2
print(joined_list)
# Output:
# ['apple', ['banana', 'cherry'], 'date', ['elderberry', 'fig']]
In this example, list1
and list2
each contain a string and a nested list. When we join these lists, the nested lists remain as individual elements in the joined list.
If you want to flatten the nested lists into the parent list, you’ll need to use a different approach, such as list comprehension or the itertools.chain()
function, which we’ll cover in the next section.
Other Methods for Joining Python Lists
While the +
operator and extend()
function are straightforward and easy to use, Python offers other, more complex methods to join lists. These methods provide more flexibility and can be more efficient in certain scenarios. Let’s delve into two of these methods: list comprehension and the itertools.chain()
function.
Joining Lists Using List Comprehension
List comprehension is a powerful feature in Python that allows you to create new lists based on existing ones. It can also be used to flatten and join nested lists.
Here’s an example of how you can use list comprehension to join lists:
nested_list = [['apple', 'banana'], ['cherry', 'date'], ['elderberry', 'fig']]
flattened_list = [item for sublist in nested_list for item in sublist]
print(flattened_list)
# Output:
# ['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig']
In this example, nested_list
is a list of lists. We use list comprehension to iterate over each sublist in nested_list
, and then over each item in the sublist. The result is a new list flattened_list
that contains all the elements from the sublists.
List comprehension provides a concise and readable way to join and flatten lists. However, it can be less intuitive than the +
operator or extend()
function, especially for beginners.
Joining Lists Using the itertools.chain()
Function
The itertools.chain()
function is part of Python’s itertools
module, which provides a set of tools for handling iterators. This function can be used to join two or more lists.
Here’s how you can use itertools.chain()
to join lists:
import itertools
list1 = ['grape', 'honeydew', 'ice cream']
list2 = ['jelly', 'kiwi', 'lemon']
joined_list = list(itertools.chain(list1, list2))
print(joined_list)
# Output:
# ['grape', 'honeydew', 'ice cream', 'jelly', 'kiwi', 'lemon']
In this example, list1
and list2
are joined together using itertools.chain()
. The result is an iterator that can be converted into a list using the list()
function.
The itertools.chain()
function can be more efficient than the +
operator or extend()
function, especially for large lists. However, it requires importing the itertools
module and is less straightforward to use.
Troubleshooting Errors With Lists
While Python makes it easy to join lists, you might encounter some common issues. Let’s discuss these potential pitfalls and how to handle them.
Dealing with Type Errors
One common issue when joining lists in Python is a TypeError. This usually occurs when you try to join a list with a non-list item. For example:
list1 = ['apple', 'banana', 'cherry']
non_list_item = 'date'
# This will raise a TypeError
joined_list = list1 + non_list_item
In this example, trying to join list1
and non_list_item
will raise a TypeError because non_list_item
is not a list. To resolve this issue, you can convert non_list_item
into a list before joining:
list1 = ['apple', 'banana', 'cherry']
non_list_item = 'date'
# Convert non_list_item into a list
non_list_item = [non_list_item]
# Now this will work
joined_list = list1 + non_list_item
print(joined_list)
# Output:
# ['apple', 'banana', 'cherry', 'date']
In the corrected code, non_list_item
is wrapped in square brackets []
to make it a list. Now, list1
and non_list_item
can be joined without raising a TypeError.
Handling Large Lists
When dealing with large lists, you might notice that your code runs slower. This is because the +
operator and extend()
function can be inefficient for large lists. In such cases, consider using the itertools.chain()
function, which can handle large lists more efficiently.
Remember, Python is a versatile language with multiple ways to accomplish the same task. If one method isn’t working for you, there’s likely another that will. Happy coding!
Understanding Python List Mutability
Before we delve deeper into joining lists in Python, it’s crucial to understand what a list is and how it works in Python. In addition, the concept of mutability plays a significant role in how lists are joined. Let’s break down these fundamental concepts.
Python Lists Explained
In Python, a list is a collection of items that are ordered and changeable. It allows duplicate members and is one of the most versatile data types in Python. A list can contain any type of items, including numbers, strings, and other lists. Here’s a simple example of a Python list:
fruits = ['apple', 'banana', 'cherry']
print(fruits)
# Output:
# ['apple', 'banana', 'cherry']
In this example, fruits
is a list that contains three strings. The print()
function is used to display the contents of the list.
Mutability in Python
Mutability is a fundamental concept in Python. In Python, an object is considered mutable if its state or contents can be changed after it’s created. Lists in Python are mutable, meaning you can change their contents, add new items, or remove existing items. For example:
fruits = ['apple', 'banana', 'cherry']
fruits[1] = 'blueberry'
print(fruits)
# Output:
# ['apple', 'blueberry', 'cherry']
In this example, the second item in the fruits
list is changed from ‘banana’ to ‘blueberry’. This is possible because lists are mutable in Python.
Mutability plays a significant role when joining lists. As we saw earlier, the extend()
function modifies the original list, while the +
operator creates a new list. This is because Python lists are mutable, and their contents can be changed.
If you are wanting more information, check out this helpful article on the differences between mutable and immutable objects.
Practical Usage of Joining Lists
Joining lists in Python is not just a fundamental operation; it’s a stepping stone to more advanced programming concepts and applications. Understanding how to efficiently join lists can significantly impact your efficiency in data manipulation, machine learning, and more.
The Role of List Joining in Data Manipulation
In data manipulation, we often need to combine or merge different data sets. Python lists, being dynamic and versatile, are commonly used for this purpose. Being able to join lists effectively means you can merge data sets, manipulate them, and extract useful insights.
Python List Joining in Machine Learning
In machine learning, data is everything. Often, this data comes in the form of arrays or lists. Joining lists allows for the combination of these data sets, and can be a crucial step in data preprocessing for machine learning algorithms.
Exploring Related Concepts
Joining lists is just one of many operations you can perform on lists in Python. Other related concepts worth exploring include list slicing, which allows you to access specific portions of a list, and list comprehension, a powerful feature that lets you create new lists based on existing ones.
# List slicing example
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
print(fruits[1:3])
# Output:
# ['banana', 'cherry']
In this example, list slicing is used to print the second and third items from the fruits
list. The :
operator is used to specify a range of indices.
Further Resources for Python List Mastery
To deepen your understanding of Python lists and related concepts, here are a few resources:
- The Art of Python List Manipulation: Delve deep into the art of list manipulation in Python, mastering advanced methods and shortcuts to enhance your coding skills.
Tutorial on Python List Comprehension: This tutorial by IOFlood covers the syntax and usage of list comprehension, including examples of filtering and transforming elements, and creating new lists.
Guide on Appending Elements to a List in Python: This IOFlood guide demonstrates different approaches to appending elements to a list in Python.
Python Lists – W3Schools: A comprehensive guide on Python lists, including how to create, access, change, and manipulate lists.
Python Data Structures: Lists – TutorialsPoint: This tutorial covers the basics of lists in Python and includes examples and explanations of different list operations.
Python List Comprehension – Real Python: A deep dive into Python list comprehension, a powerful feature that can make your code more efficient and readable.
Wrap Up: Python List Joining Methods
In this comprehensive guide, we’ve journeyed through the process of joining lists in Python. We’ve explored different methods, from the basic +
operator and extend()
function to more advanced techniques like list comprehension and the itertools.chain()
function.
We began with the basics, explaining how to use the +
operator and extend()
function to join lists. We then delved into more complex scenarios, such as joining lists of different data types and handling nested lists. We also introduced alternative methods for joining lists, including list comprehension and the itertools.chain()
function.
Along the way, we tackled common issues you might encounter when joining lists, such as TypeErrors and performance considerations with large lists, providing you with solutions and tips to handle these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
+ Operator | Simple, intuitive, creates a new list | Can be inefficient for large lists |
extend() Function | Modifies existing list, no new list created | Changes original list |
List Comprehension | Powerful, flexible, can flatten nested lists | Less intuitive for beginners |
itertools.chain() Function | Efficient for large lists | Requires importing itertools module |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of how to join lists in Python and the power of this operation. Happy coding!