Python Add to List | Methods, Syntax, Examples
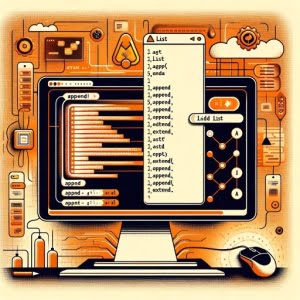
Adding an element to a list in Python is a common technique needed when scripting data processing tasks at IOFLOOD. In our experience, this task allows for dynamic data updates and expansions, all within a simple method. This article shares our techniques and best practices for adding elements to lists in Python, helping our dedicated server hosting customers manage their data more flexibly.
This comprehensive guide is designed to be your ticket conductor, teaching you how to efficiently add passengers to your Python list train. We’ll start from the simplest methods and gradually move to more advanced techniques.
So, fasten your seatbelts, and let’s get this Python list train moving!
TL;DR: How Do I Add an Element to a List in Python?
The simplest way to add an element to a list in Python is by using the
append()
method with the syntax,my_list.append(valueToAppend)
.
Here’s a quick illustration:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list)
# Output:
# [1, 2, 3, 4]
In this example, we started with a list my_list
containing elements 1, 2, and 3. We then added the number 4 to the end of the list using the append()
method. When we print the list, we can see that it now includes 1, 2, 3, and 4.
This method is straightforward and widely used, but Python offers more techniques for adding elements to a list. So, if you’re curious about more advanced methods and scenarios, keep reading!
Table of Contents
Basic Use of the append() Method
The append()
method is the go-to tool for beginners when they need to add an element to a Python list. It’s like a friendly train conductor helping you add passengers (elements) to your train (list) one at a time.
Here’s how it works:
# Let's start with an empty train (list)
my_train = []
# Now, let's add passengers (elements) using the append() method
my_train.append('John')
my_train.append('Mary')
my_train.append('Steve')
print(my_train)
# Output:
# ['John', 'Mary', 'Steve']
In the example above, we started with an empty list called my_train
. We then used the append()
method to add three elements (‘John’, ‘Mary’, and ‘Steve’) to the list. When we print the list, we can see that it now includes ‘John’, ‘Mary’, and ‘Steve’.
The append()
method is straightforward and easy to use, making it perfect for beginners. However, it’s important to note that it only adds one element at a time. If you try to add multiple elements at once, you’ll end up with a nested list, which might not be what you want.
# Let's try to add multiple passengers at once
my_train.append(['Lisa', 'Bob'])
print(my_train)
# Output:
# ['John', 'Mary', 'Steve', ['Lisa', 'Bob']]
In this case, ‘Lisa’ and ‘Bob’ were added as a sublist instead of individual elements. This is a common pitfall for beginners when using the append()
method. So remember, append()
is like a ticket for one. If you have a group, you’ll need to add them one by one.
Alternate Methods for Extending Lists
As you become more comfortable with Python, you’ll discover that append()
isn’t the only way to add elements to a list. Let’s explore two more techniques: the extend()
method and the +
operator.
The Extend Method
Think of extend()
as a method that lets you add multiple passengers to your train all at once, instead of one at a time. It takes an iterable (like a list or a tuple) and adds each of its elements to the list.
Here’s an example:
# Let's add a group of passengers to our train
my_train.extend(['Lisa', 'Bob'])
print(my_train)
# Output:
# ['John', 'Mary', 'Steve', 'Lisa', 'Bob']
In this case, ‘Lisa’ and ‘Bob’ were added as individual elements, not as a sublist. This is because extend()
unpacks the iterable and adds each of its elements to the list.
The + Operator
Another way to add elements to a list is by using the +
operator. This operator can be used to concatenate two lists, effectively adding the elements of the second list to the first.
Here’s how it works:
# Let's create a new group of passengers
new_passengers = ['Emma', 'Oliver']
# Now, let's add them to our train using the + operator
my_train = my_train + new_passengers
print(my_train)
# Output:
# ['John', 'Mary', 'Steve', 'Lisa', 'Bob', 'Emma', 'Oliver']
In this example, we created a new list new_passengers
and then added its elements to my_train
using the +
operator. The result is a longer list that includes all the original elements plus the new ones.
Both extend()
and the +
operator are more powerful than append()
because they allow you to add multiple elements at once. However, they also require a bit more care to use correctly. Always remember to use an iterable with extend()
and to assign the result back to the list when using the +
operator.
Advanced Techniques for Adding Items
For those who have mastered the basics and are ready for more advanced techniques, Python offers list comprehensions and the insert()
method. These techniques provide more control and flexibility when adding elements to a list.
List Comprehensions
List comprehensions are a unique feature of Python that allow you to create a new list based on an existing one. It’s like creating a new train filled with passengers from an old train, but with the freedom to choose who gets on.
Here’s an example:
# Let's create a list of numbers
numbers = [1, 2, 3, 4, 5]
# Now, let's create a new list with these numbers squared
squares = [number**2 for number in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this case, we started with a list of numbers. We then used a list comprehension to create a new list where each number is squared. The result is a list of squares.
The Insert Method
The insert()
method allows you to add an element at a specific position in the list. It’s like having the power to decide where each passenger will sit in your train.
Here’s how it works:
# Let's add a passenger in the middle of the train
my_train.insert(2, 'Laura')
print(my_train)
# Output:
# ['John', 'Mary', 'Laura', 'Steve', 'Lisa', 'Bob', 'Emma', 'Oliver']
In this example, we used the insert()
method to add ‘Laura’ at index 2 (remember, Python lists are zero-indexed). When we print the list, we can see that ‘Laura’ is now the third element.
These advanced techniques offer more control and flexibility when working with Python lists. However, they also require a deeper understanding of Python. So, if you’re just starting out, it’s okay to stick with append()
, extend()
, and the +
operator until you’re ready for more.
For instructions on the ways to get the Index of an item in a list, check out this tutorial!
While Python makes it relatively easy to add elements to a list, you might encounter some common issues along the way. Let’s discuss some of these potential pitfalls and how to navigate around them.
Type Errors
One common issue is the TypeError. This can occur when you try to add incompatible types to a list. For example, trying to add a string to a list of integers using the +
operator will result in a TypeError.
# Let's try to add a string to a list of integers
numbers = [1, 2, 3]
numbers = numbers + '4'
# Output:
# TypeError: can only concatenate list (not "str") to list
In this case, Python raises a TypeError because it doesn’t know how to concatenate a string (‘4’) to a list of integers. The solution is to make sure you’re always adding elements of the correct type to your list.
Index Errors
Another common issue is the IndexError. This can happen when you try to insert an element at an index that doesn’t exist in the list.
# Let's try to insert an element at an index that doesn't exist
my_train.insert(100, 'Alice')
# Output:
# No error, but 'Alice' is added at the end of the list
In this case, Python doesn’t raise an error but it doesn’t behave as you might expect either. It simply adds ‘Alice’ at the end of the list, regardless of the index you specified. This is because Python treats any index that’s greater than the length of the list as the end of the list.
To avoid having this come up accidentally, you can check the length of a list with the len() function prior to using the index value.
Best Practices
Here are some tips to avoid these common issues:
- Always make sure you’re adding elements of the correct type to your list. If you’re not sure, you can use the
type()
function to check the type of an element before adding it. - Be careful when specifying an index with the
insert()
method. Remember that Python lists are zero-indexed and that any index greater than the length of the list will be treated as the end of the list.
By keeping these tips in mind, you can avoid common pitfalls and make your journey with Python lists smoother and more enjoyable.
Key Concepts of Python Lists
Before we dive deeper into adding elements to lists, it’s crucial to understand what lists are in Python and why they are used. A Python list is a built-in data structure that can hold different types of items. In our train analogy, a list is like the train itself, and the items are the passengers. Lists are used because they are mutable, ordered, and can hold a variety of data types.
What Does Mutability Mean?
In Python, mutability refers to the ability to change an object after it has been created. Lists are mutable, which means you can add, remove, or change elements after the list is created. This is why we can add elements to a list using methods like append()
, extend()
, and insert()
.
# Let's create a list and then change it
my_list = [1, 2, 3]
print('Before:', my_list)
# Now, let's add an element
my_list.append(4)
print('After:', my_list)
# Output:
# Before: [1, 2, 3]
# After: [1, 2, 3, 4]
In this example, we started with a list containing 1, 2, and 3. We then added the number 4 using the append()
method. When we print the list again, we can see that it now includes 4. This demonstrates the mutability of Python lists.
Understanding the concept of mutability is crucial when working with Python lists. It allows us to manipulate lists in various ways, including adding elements, which is the focus of this guide.
Use Cases for Python Lists Additions
Understanding how to add elements to a Python list is a fundamental skill, but it’s just the tip of the iceberg. In larger scripts or projects, you’ll often find yourself performing more complex operations on lists.
Lists in Larger Projects
In real-world projects, lists are used to store and manipulate data. You might find yourself adding elements to a list in a loop, or based on a condition. Here’s an example of adding elements to a list inside a loop:
# Let's create an empty list
numbers = []
# Now, let's add numbers to the list inside a loop
for i in range(10):
numbers.append(i)
print(numbers)
# Output:
# [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we used a for loop to add numbers from 0 to 9 to the list. This is a common pattern in larger scripts or projects where you need to generate a list based on some logic.
Beyond Adding Elements
Adding elements to a list is just one aspect of list manipulation in Python. There are other related concepts like removing elements, iterating over lists, and sorting lists. If you’re interested in learning more about these topics, I recommend exploring Python’s official documentation and other online resources.
Remember, the key to mastering Python lists (or any programming concept, for that matter) is practice. So, don’t just read about these techniques, try them out in your own projects. Happy coding!
Further Resources for Python
If you’re interested in learning more about filtering lists and appending elements to a list in Python, here are a few resources that you might find helpful:
- Transforming Data Operations with Python Lists: Learn to transform your data operations by harnessing the power of Python lists with the help of this informative guide.
IOFlood’s Tutorial on Filtering a List in Python: This tutorial explores different methods to filter a list in Python, covering techniques such as list comprehension, the filter() function, lambda functions, and using the itertools module.
IOFlood’s Guide on Appending Elements to a List in Python: This guide demonstrates different approaches to append elements to a list in Python, including using the append() method, the += operator, and list concatenation.
Python: Adding Elements to a List: A tutorial on DigitalOcean that demonstrates different methods to add elements to a list in Python.
Python Lists: Adding Elements: A tutorial on w3schools.com that covers various techniques for adding elements to a list in Python.
Python – Adding Elements to a List using Indexing: An article on GeeksforGeeks that explains how to add elements to a list in Python using indexing techniques.
These resources will help you expand your knowledge of filtering lists and appending elements in Python, providing you with a range of techniques and examples to apply in your coding projects.
Recap: Python List Additions
In this guide, we’ve journeyed through the world of adding elements to Python lists. We’ve covered a range of techniques, from the basic append()
method to more advanced approaches like list comprehensions and the insert()
method.
Here’s a quick comparison of the methods we discussed:
Method | Description | Example |
---|---|---|
append() | Adds a single element to the end of the list | my_list.append('Alice') |
extend() | Adds multiple elements to the end of the list | my_list.extend(['Bob', 'Charlie']) |
+ operator | Concatenates two lists | my_list = my_list + ['Dave', 'Eve'] |
List comprehension | Creates a new list based on an existing one | squares = [number**2 for number in numbers] |
insert() | Adds an element at a specific position | my_list.insert(2, 'Frank') |
We also discussed common pitfalls and best practices when adding elements to a list. Remember, it’s important to ensure you’re adding elements of the correct type to your list and to be careful when specifying an index with the insert()
method.
Adding elements to a list is a fundamental skill in Python, but it’s just the beginning. Lists are a versatile data structure, and there’s much more you can do with them. So, keep exploring, keep practicing, and you’ll be a Python list pro in no time!