Solving java.lang.Nullpointerexception Errors: How-To
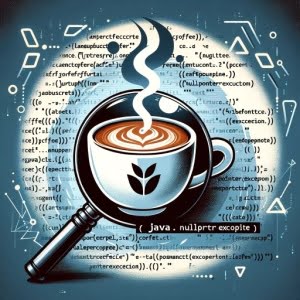
Are you constantly running into the dreaded NullPointerException in your Java code? Like a roadblock in your coding journey, NullPointerExceptions can be frustrating and confusing. They can halt your progress and leave you scratching your head, wondering what went wrong.
But don’t worry, you’re not alone. Many Java developers, especially beginners, often find themselves grappling with these elusive exceptions. Think of NullPointerExceptions as a missing piece in a puzzle – they occur when you’re trying to access something that simply isn’t there.
This guide will help you understand what a NullPointerException is, why it occurs, and how to effectively handle it in your Java programs. We’ll cover everything from the basics to more advanced techniques, providing practical examples along the way. So, let’s dive in and start mastering NullPointerExceptions in Java!
TL;DR: What is a NullPointerException in Java and How Do I Handle It?
A NullPointerException in Java occurs when you try to use a reference that points to no location in memory (null) in a situation where an object is required. For example, the line
System.out.println(str.length());
will result in an error when ran againstString str = null;
The best way to handle it is by using proper null checks and exception handling mechanisms, the simplest being atry{} catch(){}
block.
Here’s a simple example:
String str = null;
try {
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException");
}
# Output:
# 'Caught a NullPointerException'
In this example, we’ve tried to access the length()
method on a null string reference, which throws a NullPointerException. We’ve caught this exception in a try-catch block and printed a custom message.
This is a basic way to handle a NullPointerException in Java, but there’s much more to learn about preventing and handling these exceptions. Continue reading for more detailed information and advanced techniques.
Table of Contents
- Digging into NullPointerExceptions
- Advanced NullPointerException Handling
- Best Practices to Avoid NullPointerExceptions
- Troubleshooting NullPointerExceptions
- Understanding Null in Java
- Exception Handling in Java: A Broader View
- The Bigger Picture: NullPointerExceptions in Larger Applications
- Wrapping Up: NullPointerExceptions in Java
Digging into NullPointerExceptions
What is a NullPointerException?
In Java, a NullPointerException is a RuntimeException that occurs when you try to use a reference that points to no location in memory (null) in a situation where an object is required. In other words, you’re trying to do something with an object reference that currently points to null.
Why Does a NullPointerException Occur?
A NullPointerException can occur for several reasons, but the most common cause is calling an instance method or accessing or modifying an instance variable of a null object. Here’s a simple example:
String str = null;
System.out.println(str.length());
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve declared a String variable str
and assigned it the value null
. Then we’ve tried to call the length()
method on str
. Since str
is null, there’s no object to call length()
on, and we get a NullPointerException.
Handling NullPointerExceptions: Basic Techniques
The most basic way to handle a NullPointerException is to use a try-catch block. Here’s how you can modify the above code to handle the exception:
String str = null;
try {
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException");
}
# Output:
# 'Caught a NullPointerException'
In this code, we’ve wrapped the problematic code in a try block. If a NullPointerException occurs, the catch block catches the exception and executes the code within it, preventing the program from crashing.
Remember, while this method can prevent your program from crashing, it doesn’t solve the root cause of the NullPointerException. In the next sections, we’ll discuss more advanced techniques to prevent NullPointerExceptions from occurring in the first place.
Advanced NullPointerException Handling
Embrace the Power of Optional
Java 8 introduced a new class called Optional
that can help handle situations where you might have a null reference. Optional
is a container object that may or may not contain a non-null value.
Here’s how you can use Optional
to avoid a NullPointerException:
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> str = Optional.ofNullable(null);
System.out.println(str.orElse("Default String"));
}
}
# Output:
# 'Default String'
In this example, we create an Optional
that might hold a String
and assign it a null value. When we try to print the value of str
, instead of throwing a NullPointerException, the Optional
returns the default value that we provided with the orElse
method.
Use Objects.requireNonNull
Another method to prevent NullPointerExceptions is by using the Objects.requireNonNull
method. This method returns the first argument if it’s not null and throws a NullPointerException otherwise. Here’s an example:
import java.util.Objects;
public class Main {
public static void main(String[] args) {
String str = null;
try {
str = Objects.requireNonNull(str, "String is null");
} catch (NullPointerException e) {
System.out.println(e.getMessage());
}
}
}
# Output:
# 'String is null'
In this code, Objects.requireNonNull
checks if str
is null. If it is, it throws a NullPointerException with the custom error message we provided. We catch this exception in a try-catch block and print the custom error message.
These techniques can help you prevent NullPointerExceptions in your code. However, the best way to handle NullPointerExceptions is to avoid them altogether. In the next section, we’ll discuss best practices to prevent NullPointerExceptions.
Best Practices to Avoid NullPointerExceptions
Proper Object Initialization
One of the best ways to avoid NullPointerExceptions is to properly initialize your objects. When declaring an object reference, make sure to initialize it to a valid object instead of leaving it null. If necessary, you can initialize it to a default value.
Here’s an example:
String str = ""; // Instead of String str = null;
System.out.println(str.length());
# Output:
# 0
In this example, instead of initializing str
to null, we initialize it to an empty string. When we call length()
, it returns 0 instead of throwing a NullPointerException.
Using @NotNull Annotation
Another best practice is to use the @NotNull
annotation. This annotation can be used on a method, parameter, or field to indicate that null is not a valid value. Here’s an example:
import org.jetbrains.annotations.NotNull;
public class Main {
public static void main(String[] args) {
Main main = new Main();
main.printLength(null);
}
public void printLength(@NotNull String str) {
System.out.println(str.length());
}
}
# Output:
# Exception in thread "main" java.lang.IllegalArgumentException: Argument for @NotNull parameter 'str' of Main.printLength must not be null
In this example, we’ve annotated the str
parameter of the printLength
method with @NotNull
. When we try to pass a null value to printLength
, it throws an IllegalArgumentException instead of a NullPointerException.
These best practices can help you prevent NullPointerExceptions in your code. However, even with these practices, there might be scenarios where a NullPointerException can occur. In the next section, we’ll discuss common scenarios and their solutions.
Troubleshooting NullPointerExceptions
Scenario 1: Calling Methods on Null
The most common scenario for a NullPointerException is calling a method on a null reference. Here’s an example:
String str = null;
System.out.println(str.length());
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve tried to call the length()
method on a null string reference, which throws a NullPointerException. To avoid this, always check if a reference is null before calling methods on it.
Scenario 2: Accessing or Modifying a Null Object’s Fields
Another common scenario is accessing or modifying the fields of a null object. Here’s an example:
import java.awt.Point;
public class Main {
public static void main(String[] args) {
Point point = null;
System.out.println(point.x);
}
}
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve tried to access the x
field of a null Point object, which throws a NullPointerException. To avoid this, always check if an object is null before accessing or modifying its fields.
Scenario 3: Throwing Null
You can also get a NullPointerException by throwing null. Here’s an example:
public class Main {
public static void main(String[] args) {
throw null;
}
}
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve tried to throw null, which throws a NullPointerException. To avoid this, never throw null in your code.
Remember, the best way to avoid NullPointerExceptions is to follow the best practices we discussed earlier. Always initialize your objects properly, use the Optional
class and the @NotNull
annotation, and always check for null before calling methods or accessing fields.
Understanding Null in Java
The Concept of Null
In Java, null
is a special value that represents the absence of a value or object reference. It’s not the same as zero or false, and it’s not even the same as an empty string. It’s a unique value that indicates that a variable doesn’t point to any object or value.
Here’s a simple example:
String str = null;
System.out.println(str);
# Output:
# null
In this example, we’ve declared a String variable str
and assigned it the value null
. When we print str
, it outputs null
, indicating that str
doesn’t point to any object.
How Java Handles Null References
When you try to call a method or access a field on a null reference, Java throws a NullPointerException
. This is Java’s way of telling you that you’re trying to do something with a reference that points to no location in memory.
Exception Handling in Java: A Broader View
In Java, an exception is an event that disrupts the normal flow of the program. NullPointerException
is a type of unchecked exception, which means it’s a subclass of RuntimeException
and the compiler doesn’t check to see if a method handles or throws it.
There are many ways to handle exceptions in Java, such as using try-catch blocks, throwing exceptions, and using the finally
block. However, the best way to handle exceptions is to prevent them from occurring in the first place. As we’ve discussed, you can prevent NullPointerExceptions
by using proper null checks, using the Optional
class, and following other best practices.
The Bigger Picture: NullPointerExceptions in Larger Applications
Understanding and handling NullPointerExceptions is not just crucial for small programs, but it’s also vital in larger applications. In complex software, NullPointerExceptions can be harder to trace and can cause significant issues if not handled properly.
Memory Management in Java
One of the key areas where NullPointerExceptions play a role is memory management. When an object is null, it means that it’s not pointing to any location in memory. Understanding how Java handles memory allocation and deallocation can help you avoid NullPointerExceptions.
Other Common Exceptions in Java
NullPointerExceptions are just one type of exception in Java. There are many other common exceptions like ArrayIndexOutOfBoundsException, ClassNotFoundException, and IOException, each with their own causes and handling techniques. Understanding these exceptions can help you write more robust and error-free code.
Further Resources for Mastering Java Exceptions
If you’re interested in going beyond the basics and mastering exceptions in Java, here are some resources you might find useful:
- Java Error Fundamentals Covered – Explore Java error prevention techniques.
Guide to Throwing Exceptions in Java – Learn syntax and best practices for exception throwing.
Understanding “Could Not Find or Load Main Class” Issue – Learn troubleshooting steps to resolve the issue.
Java: A Beginner’s Guide covers all the basics of Java and includes a comprehensive section on exceptions.
The Java Tutorials: Exceptions provides in-depth information on how to handle exceptions in Java.
Java Exception Handling covers all aspects of Java exception handling, including best practices and common pitfalls.
Remember, understanding NullPointerExceptions and other exceptions is just one part of becoming a proficient Java developer. Keep exploring, keep learning, and you’ll continue to grow your skills.
Wrapping Up: NullPointerExceptions in Java
In this comprehensive guide, we’ve navigated the intricacies of the Java Lang NullPointerException, a common stumbling block for many Java developers. We’ve dissected what a NullPointerException is, why it occurs, and most importantly, how to effectively handle it in your Java programs.
We began with the basics, providing a solid understanding of what a NullPointerException is and why it occurs. We then delved into basic handling techniques, using simple null checks and try-catch blocks. From there, we explored more advanced techniques, such as utilizing the Optional class introduced in Java 8 and the Objects.requireNonNull
method.
We also discussed best practices to avoid NullPointerExceptions, emphasizing the importance of proper object initialization and the use of annotations like @NotNull
. We then highlighted common scenarios where NullPointerExceptions are likely to occur and provided solutions for each.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Basic Handling (try-catch) | Simple, handles exceptions | Doesn’t solve root cause |
Optional Class | Prevents NullPointerExceptions | More complex |
Objects.requireNonNull | Throws NullPointerException early | Requires custom error message |
Proper Initialization and @NotNull | Prevents NullPointerExceptions | Requires careful coding |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up on your skills, we hope this guide has provided you with a deeper understanding of NullPointerExceptions and how to handle them.
Understanding and properly handling NullPointerExceptions is crucial for writing robust and error-free Java code. Now, you’re well equipped to tackle any NullPointerException that comes your way. Happy coding!