Java LinkedList: A Complete Usage Guide
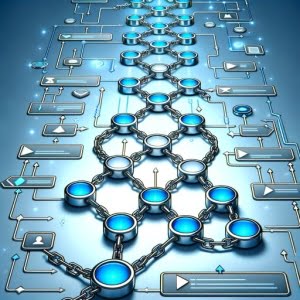
Are you finding it challenging to manage and manipulate data structures in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling LinkedList in Java, but we’re here to help.
Think of Java’s LinkedList as a chain of connected elements – a versatile tool that can be used to store and manage data efficiently. LinkedLists can be a powerful tool in your Java toolkit, once you understand how to use them.
This guide will walk you through the process of using LinkedList in Java, from the basics to more advanced techniques. We’ll cover everything from creating a LinkedList, adding elements to it, retrieving elements from it, to more complex operations like inserting elements at a specific position, removing elements, and iterating over a LinkedList. We’ll also introduce you to other List implementations in Java, such as ArrayList and Vector, and discuss when to use LinkedList over these alternatives.
So, let’s dive in and start mastering LinkedList in Java!
TL;DR: How Do I Use LinkedList in Java?
LinkedList in Java is a class that implements the List and Deque interfaces, created with the syntax
LinkedList<String> list = new LinkedList<String>();
. It allows you to store and manipulate a collection of elements.
Here’s a simple example:
LinkedList<String> list = new LinkedList<String>();
list.add("Hello");
System.out.println(list);
// Output:
// [Hello]
In this example, we create a LinkedList of Strings and add the element ‘Hello’ to it. We then print the list, which outputs ‘[Hello]’.
This is a basic way to use LinkedList in Java, but there’s much more to learn about LinkedList and its capabilities. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
Using LinkedList in Java: The Basics
LinkedList in Java is a powerful tool for storing and managing data. Let’s start with the basics – creating a LinkedList, adding elements to it, and retrieving those elements.
Creating a LinkedList
To create a LinkedList, you need to instantiate it. Here’s how you can do it:
LinkedList<String> list = new LinkedList<String>();
In this code block, we’re creating a LinkedList of Strings named ‘list’.
Adding Elements to LinkedList
Once you have a LinkedList, you can add elements to it using the ‘add’ method. Let’s add some elements to our list:
list.add("Hello");
list.add("World");
System.out.println(list);
// Output:
// [Hello, World]
In this example, we’re adding two elements (‘Hello’ and ‘World’) to our list and then printing the list.
Retrieving Elements from LinkedList
You can retrieve elements from your LinkedList using the ‘get’ method. Here’s how to retrieve the first element:
String firstElement = list.get(0);
System.out.println(firstElement);
// Output:
// Hello
In this code block, we’re retrieving the first element (index 0) from our list and printing it.
LinkedLists in Java offer several advantages, such as dynamic sizing and easy insertion or deletion of elements. However, they can be slower than other data structures like arrays or ArrayLists when it comes to accessing elements, as they need to traverse the list from the start to get to a specific element.
Advanced LinkedList Operations in Java
After mastering the basics of LinkedList in Java, it’s time to explore some more complex operations. This section will cover inserting elements at a specific position, removing elements, and iterating over a LinkedList.
Inserting Elements at a Specific Position
LinkedList allows you to insert an element at a specific position using the ‘add’ method with two parameters: the index at which you want to insert the element, and the element itself. Here’s an example:
list.add(1, "Java");
System.out.println(list);
// Output:
// [Hello, Java, World]
In this code block, we’re inserting the element ‘Java’ at the second position (index 1) of our list. The existing elements shift to accommodate the new one.
Removing Elements from LinkedList
You can remove an element from a LinkedList either by specifying the element itself or its position. Here’s how to do it:
list.remove(1);
System.out.println(list);
// Output:
// [Hello, World]
In this example, we’re removing the element at the second position (index 1) from our list.
Iterating Over a LinkedList
There are several ways to iterate over a LinkedList in Java. One of the most common ways is using a for-each loop. Here’s an example:
for (String element : list) {
System.out.println(element);
}
// Output:
// Hello
// World
In this code block, we’re iterating over our list and printing each element.
While LinkedLists provide flexibility with these operations, keep in mind that inserting and removing elements in the middle of the list require shifting of elements, which can be slower than in an ArrayList. Therefore, LinkedLists are best used when you frequently add or remove elements from the ends of the list, and less so when you need to access elements in the middle.
Exploring Alternative List Implementations in Java
While LinkedLists are a powerful tool in Java, they’re not the only way to manage collections of elements. Other List implementations, such as ArrayList and Vector, offer alternative approaches to handling data.
Understanding ArrayLists
ArrayLists are a popular choice for handling collections in Java. They are dynamic arrays and provide fast access to elements because they are index-based.
ArrayList<String> arrayList = new ArrayList<String>();
arrayList.add("Hello");
arrayList.add("World");
System.out.println(arrayList);
// Output:
// [Hello, World]
In this example, we create an ArrayList, add elements to it, and print the list. The usage is very similar to LinkedList, but the underlying data structure is different.
Discovering Vectors
Vectors in Java are very similar to ArrayLists, but they are synchronized, making them thread-safe.
Vector<String> vector = new Vector<String>();
vector.add("Hello");
vector.add("World");
System.out.println(vector);
// Output:
// [Hello, World]
Here, we create a Vector, add elements, and print the list. Again, the usage is similar to LinkedList and ArrayList, but the Vector is thread-safe.
Choosing Between LinkedList, ArrayList, and Vector
When should you use LinkedList over ArrayList or Vector? It depends on your needs. LinkedLists are ideal when you frequently add or remove elements from the ends of the list, while ArrayLists are better for situations where you need fast access to elements. Vectors are best used in multi-threaded environments where you need to ensure thread safety.
LinkedList | ArrayList | Vector | |
---|---|---|---|
Fast Access | No | Yes | Yes |
Fast Insertion/Deletion at Ends | Yes | No | No |
Thread-Safe | No | No | Yes |
Remember, choosing the right data structure can greatly affect the performance of your Java program.
Troubleshooting Common LinkedList Issues in Java
While LinkedLists in Java are a powerful tool, they can sometimes cause issues that can be difficult to troubleshoot. This section will discuss some common issues you may encounter when using LinkedList, such as the ConcurrentModificationException, and provide solutions and workarounds for each issue.
Understanding ConcurrentModificationException
One of the most common issues you might encounter when using LinkedList in Java is the ConcurrentModificationException. This exception is thrown when a thread is modifying a collection while another thread is traversing through it using an iterator.
Here’s an example of what this might look like:
LinkedList<String> list = new LinkedList<String>();
list.add("Hello");
list.add("World");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String element = iterator.next();
if (element.equals("World")) {
list.remove(element);
}
}
// Output:
// Exception in thread "main" java.util.ConcurrentModificationException
In this example, we’re trying to remove an element from the list while we’re iterating over it, which causes a ConcurrentModificationException.
Handling ConcurrentModificationException
So how can you avoid this exception? One way is to use the ‘remove’ method of the Iterator itself, instead of the LinkedList’s ‘remove’ method. Here’s how you can do it:
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String element = iterator.next();
if (element.equals("World")) {
iterator.remove();
}
}
System.out.println(list);
// Output:
// [Hello]
In this code block, we’re using the ‘remove’ method of the Iterator to remove the current element. This way, we avoid the ConcurrentModificationException and successfully remove the element ‘World’ from our list.
Remember, when using LinkedList in Java, it’s important to understand the potential issues and how to troubleshoot them. This will help you write more robust and efficient code.
Understanding LinkedList in Java
To effectively use LinkedLists in Java, it’s crucial to understand the underlying concepts and the LinkedList class itself. Let’s dive deeper into the LinkedList class, its methods, and how it implements the List and Deque interfaces.
LinkedList Class and Its Methods
In Java, LinkedList is a class that belongs to the Java Collections Framework. It implements the List and Deque interfaces, meaning it can be used as a list or a double-ended queue.
LinkedList<String> list = new LinkedList<String>();
In this code block, we’re creating an instance of the LinkedList class. This LinkedList can store String objects, but it can store any type of objects.
The LinkedList class provides a wide range of methods to manipulate data. Some of the most commonly used methods are ‘add’, ‘get’, ‘remove’, ‘size’, and ‘clear’.
LinkedList and List and Deque Interfaces
As LinkedList implements the List and Deque interfaces, it inherits methods from these interfaces. The List interface provides methods for manipulating elements based on their position in the list, while the Deque interface provides methods for adding, removing, and examining elements from both ends of the deque.
The Concept of Linked Lists in Computer Science
In computer science, a linked list is a linear data structure where each element points to the next one. It consists of nodes, where each node contains a data field and a reference to the next node in the list.
In a LinkedList in Java, each node is an object that contains a reference to the data and the next node. This allows for efficient insertions and deletions, as you only need to update the references of the neighboring nodes.
Understanding these fundamental concepts is key to mastering the use of LinkedList in Java.
LinkedList in Real-World Applications
LinkedLists in Java are not just a theoretical concept – they have a wide range of real-world applications. Let’s explore a couple of examples to illustrate their practical use.
LinkedList as a Music Playlist
Consider a music playlist. You can think of it as a LinkedList, where each song is a node. The ‘next’ reference points to the next song in the playlist, allowing you to easily skip to the next song or go back to the previous one.
LinkedList<String> playlist = new LinkedList<String>();
playlist.add("Song 1");
playlist.add("Song 2");
playlist.add("Song 3");
System.out.println(playlist);
// Output:
// [Song 1, Song 2, Song 3]
In this example, we’re creating a playlist as a LinkedList and adding songs to it.
LinkedList for Browser History
Another common use of LinkedLists is in web browsers to manage browsing history. Each website you visit is a node in the LinkedList. The ‘back’ and ‘forward’ buttons in your browser navigate through this LinkedList.
LinkedList<String> browsingHistory = new LinkedList<String>();
browsingHistory.add("Website 1");
browsingHistory.add("Website 2");
browsingHistory.add("Website 3");
System.out.println(browsingHistory);
// Output:
// [Website 1, Website 2, Website 3]
In this code block, we’re simulating a browsing history as a LinkedList.
Exploring Related Concepts
Once you’re comfortable with LinkedLists in Java, there are other Java collections and data structures worth exploring, such as ArrayLists, Vectors, Stacks, Queues, and Trees. Each of these data structures has its own set of advantages, disadvantages, and use cases.
Further Resources for Mastering LinkedList in Java
- Understanding Java’s Data Structures – Discover how Java data structures facilitate storage, retrieval, and manipulation of data.
Exploring Java Tuple – Learn about Tuple implementations and their usage in various programming scenarios.
Java Stack Explained – Dive into the Stack data structure in Java, following the Last-In-First-Out (LIFO) principle.
Oracle’s official Java Documentation provides an in-depth look at the LinkedList class and its methods.
Geeks for Geeks offers a comprehensive guide on linked lists and their types, operations, and applications.
JavaTpoint covers Java’s Collections Framework, including LinkedList and other data structures.
Wrapping Up
In this comprehensive guide, we’ve explored the ins and outs of LinkedList in Java, from basic usage to advanced techniques. We’ve also discussed alternative approaches to handling collections in Java, providing you with a broader understanding of data structures and their applications.
We began with the basics of LinkedList, learning how to create a LinkedList, add elements to it, and retrieve those elements. We then delved into more advanced operations, such as inserting elements at a specific position, removing elements, and iterating over a LinkedList. We also tackled common issues you might encounter when using LinkedList, such as the ConcurrentModificationException, and provided solutions to these challenges.
In addition to LinkedList, we explored other List implementations in Java, such as ArrayList and Vector, comparing their use cases, pros, and cons. Here’s a quick comparison of the methods we’ve discussed:
LinkedList | ArrayList | Vector | |
---|---|---|---|
Fast Access | No | Yes | Yes |
Fast Insertion/Deletion at Ends | Yes | No | No |
Thread-Safe | No | No | Yes |
Whether you’re just starting out with LinkedList in Java or looking to level up your skills, we hope this guide has given you a deeper understanding of LinkedList and its capabilities.
With its flexibility and power, LinkedList is a valuable tool in your Java toolkit. Now, you’re well equipped to tackle any data structure challenges that come your way. Happy coding!