Java Tuples: Guide to Object Pairing with Various Classes
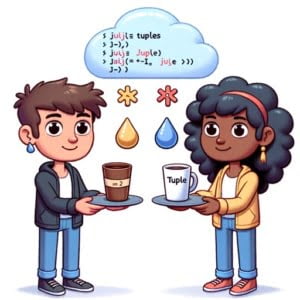
Ever felt puzzled about how to use tuples in Java? You’re not alone. Many developers find themselves in a similar situation, but we’re here to help.
Think of Java tuples as a multi-functional toolbox – they can hold multiple data types, making them a versatile tool for various tasks.
In this guide, we’ll walk you through the concept of tuples in Java, how to use them, and the alternatives available. We’ll cover everything from the basics of tuples to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering tuples in Java!
TL;DR: How Do I Use Tuples in Java?
Java doesn’t have built-in support for tuples. However, you can use classes from libraries like Apache Commons Lang’s
Pair
class, used with the syntaxPair<dataType1, dataType2> pair
. Here’s a simple example using Apache Commons Lang:
Pair<String, Integer> pair = Pair.of('Hello', 5);
# Output:
# Pair: ('Hello', 5)
In this example, we’ve used the Pair
class from Apache Commons Lang to create a tuple. The Pair.of()
method is used to create a new pair of elements, in this case, a string ‘Hello’ and an integer 5. The resulting pair can be used as a tuple.
This is just a basic way to use tuples in Java, but there’s much more to learn about creating and manipulating tuples. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Java Tuples
In Java, tuples aren’t a built-in feature like in some other languages. But don’t worry, we have a handy workaround for you – using the Pair
class from the Apache Commons Lang library. This class allows us to create a simple tuple of two elements.
Let’s see it in action:
import org.apache.commons.lang3.tuple.Pair;
public class Main {
public static void main(String[] args) {
Pair<String, Integer> pair = Pair.of('Hello', 5);
System.out.println(pair);
}
}
# Output:
# (Hello,5)
In this example, we’ve imported the Pair
class from Apache Commons Lang library. We then create a Pair
object, pair
, with a String ‘Hello’ and an Integer 5. The Pair.of()
method is used to instantiate a new pair. When we print the pair
, it shows the pair of elements as output.
Advantages of Using Pair
The Pair
class is simple and easy to use, making it a great choice for beginners. It’s also part of a well-maintained library, which means it’s reliable and efficient.
Potential Pitfalls
However, the Pair
class is limited to only two elements. If you need to store more than two elements in a tuple, you’ll need to use another approach, which we’ll discuss in the Advanced Use section. Also, remember to include the Apache Commons Lang library in your project before using the Pair
class.
Crafting Your Own Java Tuple Class
For more flexibility, you can create your own class to represent a tuple. This approach gives you the freedom to design a tuple that fits your specific needs, such as accommodating more than two elements.
Let’s create a simple Triple
class to represent a tuple of three elements:
public class Triple<A, B, C> {
public final A first;
public final B second;
public final C third;
public Triple(A first, B second, C third) {
this.first = first;
this.second = second;
this.third = third;
}
}
public class Main {
public static void main(String[] args) {
Triple<String, Integer, Boolean> triple = new Triple<>('Hello', 5, true);
System.out.println('(' + triple.first + ',' + triple.second + ',' + triple.third + ')');
}
}
# Output:
# (Hello,5,true)
In this example, we’ve created a Triple
class that can hold three elements of any type. The elements are public and final, meaning they can be accessed directly but cannot be changed after they are initialized. We then create a Triple
object, triple
, with a String ‘Hello’, an Integer 5, and a Boolean true. When we print the triple
, it shows the triple of elements as output.
Advantages of Creating Your Own Tuple Class
Creating your own tuple class offers more flexibility and control. You can define the types and number of elements that your tuple class can hold.
Potential Pitfalls
The downside is that it requires more effort and understanding of Java’s generics. It can also lead to code duplication if you need tuples of different lengths. In such cases, using a library might be a better option.
Exploring Alternative Java Tuple Methods
While we’ve covered using the Apache Commons Lang library and creating your own class to represent tuples in Java, there are other methods that offer more flexibility or simplicity depending on your needs.
Using Other Libraries
There are other libraries, such as Javatuples and Guava, that provide classes to represent tuples. These libraries offer more options in terms of the number of elements a tuple can hold.
Here’s an example using Javatuples:
import org.javatuples.Triplet;
public class Main {
public static void main(String[] args) {
Triplet<String, Integer, Boolean> triplet = Triplet.with('Hello', 5, true);
System.out.println(triplet);
}
}
# Output:
# [Hello, 5, true]
In this example, we’ve used the Triplet
class from Javatuples library to create a tuple of three elements. The Triplet.with()
method is used to create a new triplet.
Using Arrays or Lists
You can also use arrays or lists to represent tuples. However, these data structures don’t enforce a fixed size or type for the elements, which can lead to errors if not handled correctly.
Here’s an example using an array:
public class Main {
public static void main(String[] args) {
Object[] tuple = new Object[]{'Hello', 5, true};
System.out.println(Arrays.toString(tuple));
}
}
# Output:
# [Hello, 5, true]
In this example, we’ve created an array tuple
that holds three elements of different types. The Arrays.toString()
method is used to print the array.
Comparing the Methods
Each method has its own advantages and trade-offs. Using a library like Apache Commons Lang or Javatuples provides predefined classes that are easy to use and can hold multiple elements. Creating your own class gives you more flexibility but requires more effort. Using arrays or lists is simple but doesn’t enforce type or size constraints.
Choosing the right method depends on your specific needs and the complexity of your project.
While tuples can be a powerful tool in your Java arsenal, they come with their own set of challenges. Let’s explore some common issues you might encounter when working with tuples in Java and how to overcome them.
Issue: Type Safety
One of the main challenges when using tuples is ensuring type safety. Since tuples can hold different types of data, it’s easy to run into type mismatch issues.
Consider the following code:
Pair<String, Integer> pair = Pair.of('Hello', 'World');
This will result in a compile-time error because the second element of the pair is supposed to be an Integer, but a String is provided.
Solution: Type Checking
To avoid type mismatch issues, always ensure that the data you’re storing in a tuple matches the type you’ve defined for each element.
Issue: Readability
Tuples can make your code harder to read and understand, especially if you’re using them to store unrelated data.
Solution: Use Descriptive Names
When creating your own tuple class, use descriptive names for the elements. For example, instead of naming your elements ‘first’, ‘second’, and ‘third’, you could use more descriptive names like ‘userName’, ‘userId’, and ‘isVerified’.
Issue: Libraries
If you’re using a library to create tuples, you need to ensure that the library is included in your project. If it’s not, you’ll encounter errors.
Solution: Include the Necessary Libraries
Before using a tuple class from a library, make sure to include the library in your project. Most IDEs provide easy ways to add libraries to your project.
Working with tuples in Java can be a bit tricky, but with these tips and considerations in mind, you’ll be able to navigate any challenges that come your way.
Understanding Tuples and Their Absence in Java
A tuple is a collection of values that are grouped together. It’s a simple concept, but it’s incredibly powerful. Tuples allow you to store multiple values in a single variable, and those values can be of different types. This makes tuples a flexible and versatile tool for handling data.
In some programming languages, like Python and Scala, tuples are a built-in feature. Here’s an example of a tuple in Python:
my_tuple = ('Hello', 5, True)
print(my_tuple)
# Output:
# ('Hello', 5, True)
In this Python code, we’ve created a tuple my_tuple
that holds three elements of different types. This is a common use of tuples in languages that support them natively.
However, Java does not have built-in support for tuples. This absence can be attributed to Java’s strong typing system and its focus on object-oriented programming. In Java, data is typically grouped together in objects, not tuples.
Despite this, there are ways to achieve similar functionality in Java, as we’ve discussed in previous sections. Through the use of external libraries or custom classes, we can create and manipulate tuples in Java.
Understanding the concept of tuples and the reasons for their absence in Java is key to using them effectively. With this knowledge, you can make informed decisions about when and how to use tuples in your Java projects.
Tuples in Large-Scale Java Projects
Tuples can be a valuable asset in larger Java projects. They can simplify your code by allowing you to return multiple values from a method, which can reduce the need for creating additional classes.
Consider a method that needs to return multiple values. Without tuples, you might create a class to hold these values. But with tuples, you can simply return a tuple, making your code cleaner and more concise.
Exploring Related Concepts: Generics and Collections
If you’re interested in further expanding your Java knowledge, exploring related concepts like generics and collections can be a great next step. Generics allow you to write a single method or class that works on different types, making your code more reusable. Collections, on the other hand, are a fundamental part of Java that allows you to group and manipulate objects.
Further Resources for Java Tuple Mastery
To deepen your understanding of tuples in Java and related concepts, here are a few resources that you might find helpful:
- Fundamentals of Data Structures in Java – Understand dynamic programming and its application in Java data structures.
Java Dictionary Explained – Learn about the Dictionary interface in Java for key-value pair storage.
Java LinkedList Overview – Explore LinkedList, a linear data structure in Java offering dynamic memory allocation.
Java Generics Tutorial – A comprehensive guide on Java generics from Oracle.
Java Collections Tutorial – An in-depth tutorial on Java collections, also from Oracle.
Apache Commons Lang – The official documentation for the Apache Commons Lang library, which includes the
Pair
class.
These resources provide a wealth of information that can help you become more proficient in Java and its various features, including tuples.
Wrapping Up: Tuples in Java
In this comprehensive guide, we’ve explored the concept of tuples in Java, how to use them, and the alternatives available.
We began with the basics, learning how to use the Pair
class from the Apache Commons Lang library to represent a tuple. We then ventured into more advanced territory, discussing how to create your own class to represent a tuple. Along the way, we explored alternative methods to represent tuples, such as using classes from other libraries or using arrays or lists.
We also tackled common challenges you might face when working with tuples in Java, such as ensuring type safety and readability, and provided solutions to help you overcome these challenges. Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Ease of Use |
---|---|---|
Using Apache Commons Lang | Medium | High |
Creating Your Own Class | High | Medium |
Using Other Libraries | High | Medium |
Using Arrays or Lists | Low | High |
Whether you’re just starting out with tuples in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of tuples and their capabilities.
With its balance of flexibility and ease of use, tuples can be a powerful tool in your Java programming arsenal. Happy coding!