Python Max() Function Guide: Uses and Examples
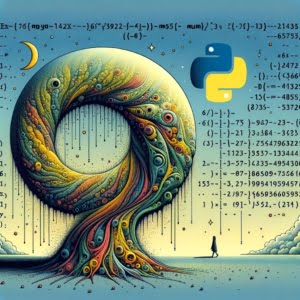
Python, a language known for its simple syntax and rich library of built-in functions, offers a range of powerful tools for both beginner and seasoned developers.
Among these, the max()
function stands out for its robustness and versatility. This built-in function is often used in daily programming tasks due to its ability to return the largest item in an iterable or the largest of two or more arguments.
In this comprehensive guide, we’ll delve into the max()
function, exploring its syntax, functionality, and application in Python coding. You’ll be provided with practical examples to better understand its usage.
TL;DR: What is Python’s max() function?
Python’s
max()
function is a built-in tool that returns the largest item in an iterable or the largest of two or more arguments. It’s versatile and can work with different data types and Python data structures. For a more in-depth understanding and advanced usage, continue reading the article.
print(max([1, 2, 3])) # Output: 3
Table of Contents
- Understanding Python’s max() Function
- Syntax and Usage of Python’s max() Function
- Practical Examples of Python’s max() Function
- max() with Python Data Structures
- max() with Multiple Iterables
- Potential Errors with the max() Function
- Python’s max() and min() Functions Compared
- Further Resources for Python Functions
- Final Thoughts
Understanding Python’s max() Function
Python’s max()
function is a built-in tool that returns the largest item in an iterable, or the largest of two or more arguments. If the iterable is empty, it raises a ValueError exception. The items in the iterable can be numbers (integers, floats), strings, or any other object that can be compared.
Compared to similar functions in other languages like Java or C++, this built-in function offers more flexibility. In Java, for instance, you would need to write a loop to find the maximum value in an array. With Python’s
max()
function, you can accomplish this with a single line of code, making your code cleaner and more efficient.
This versatile tool isn’t just for numbers, it works with any iterable – lists, tuples, dictionaries, sets, and even strings. For example, when used with a string, max()
returns the character that has the maximum ASCII value.
# Using max() with a string
string_example = "python"
print(max(string_example)) # Output: y
One of the standout features of the max()
function is its ability to find the maximum value based on a custom function. This is done using the key
argument. For instance, you could use the max()
function to find the longest word in a list of words, or the employee with the highest salary in a list of employees.
# Using max() to find the longest word
words = ['Python', 'is', 'a', 'popular', 'programming', 'language']
print(max(words, key=len)) # Output: programming
# Using max() to find the employee with the highest salary
employees = [
{'name': 'John', 'salary': 50000},
{'name': 'Jane', 'salary': 60000},
{'name': 'Bob', 'salary': 70000}
]
print(max(employees, key=lambda employee: employee['salary']))
# Output: {'name': 'Bob', 'salary': 70000}
The adaptability of the max()
function makes it an essential tool in Python programming. Whether you’re working with numbers, strings, or custom objects, the max()
function can assist you in finding the maximum value in a simple and efficient way.
Syntax and Usage of Python’s max() Function
To effectively use Python’s max()
function in your programs, it’s crucial to understand its syntax. In this section, we’ll break down the syntax when used with objects and iterables, and discuss the parameters that the function accepts.
Syntax with Objects
When used with two or more arguments (objects), the max()
function syntax is: max(arg1, arg2, *args[, key])
Here, arg1
, arg2
, … are the objects to be compared. The key
is an optional parameter that specifies a function of one argument that is used to extract a comparison key from each input element.
max_val = max(3, 9, 5)
print(max_val) # Output: 9
In the above example, max()
compares the numbers directly and returns the largest number.
Syntax with Iterables
When used with an iterable, the max()
function syntax is: max(iterable, *[, key, default])
Here, iterable
can be a list, tuple, string, etc. The key
and default
are optional parameters. The key
functions as above, and the default
value is returned if the iterable is empty.
max_val = max([1, 2, 3])
print(max_val) # Output: 3
In this example, max()
finds the largest value in the list.
Parameters and Return Values
The max()
function accepts two types of arguments:
- Two or more objects (like integers, floats, strings, etc.)
- An iterable (like list, tuple, string, etc.)
It also accepts two optional keyword-only arguments:
Parameter | Type | Description |
---|---|---|
key | function (optional) | A function of one argument that is used to extract a comparison key from each input element. The default value is None . |
default | varies (optional) | A value that is returned if the provided iterable is empty. The default value is None . If the iterable is empty and default is not provided, a ValueError is raised. |
The max()
function returns the largest item in the iterable or the largest of two or more arguments. If the iterable is empty and default is provided, it returns the default value. If the iterable is empty and default is not provided, it raises a ValueError
.
Using key and default parameters
Here’s a Python code block that shows the use of max()
function with the key
and default
parameters:
# A list of words
words = ['Python', 'is', 'versatile']
# Using max() with `key` parameter
print(max(words, key=len))
# Output: versatile
# An empty list
empty_list = []
# Using max() with `default` parameter on an empty list
print(max(empty_list, default="List is empty"))
# Output: List is empty
# Using max() without `default` parameter on an empty list
print(max(empty_list))
# Raises ValueError: max() arg is an empty sequence
In the first max()
use, the key
parameter is used to determine the word with maximum length. In the second use, max()
is used on an empty list with default
parameter, so it returns the default value. In the final use, max() is used on an empty list without default
parameter, so it raises a ValueError
.
Practical Examples of Python’s max() Function
While understanding the theory behind the max()
function is essential, seeing it in action offers a more tangible grasp of its application. In this section, we’ll explore practical examples of the max()
function with different data types and Python data structures.
max() with Different Data Types
Python’s max()
function can handle different data types such as integers and strings. Let’s examine how it works with these data types.
Integers
print(max(5, 10, 15)) # Output: 15
In this example, max()
compares the integers and returns the largest one.
Strings
print(max('apple', 'banana', 'cherry')) # Output: 'cherry'
Floats
print(max(5.5, 10.3, 15.2)) # Output: 15.2
Here, max()
compares the strings lexicographically based on the ASCII values of the characters and returns the ‘largest’ string.
max() with Python Data Structures
The max()
function is also compatible with Python data structures such as lists and dictionaries. Let’s see how it performs in these scenarios.
Lists
print(max([5, 10, 15])) # Output: 15
In this example, max()
returns the largest value from the list.
Dictionaries
print(max({'apple': 1, 'banana': 2, 'cherry': 3})) # Output: 'cherry'
Sets
print(max({5, 10, 15})) # Output: 15
Here, max()
returns the key with the maximum value when used with a dictionary.
max() with Multiple Iterables
Python’s max()
function can also handle multiple iterables.
print(max([5, 10, 15], [20, 25, 30])) # Output: [20, 25, 30]
In this scenario, max()
compares the first elements of the lists, then the second elements, and so on, until it can determine the maximum list.
Potential Errors with the max() Function
Despite the versatility of the max()
function, it’s crucial to be aware of potential errors. For instance, using max()
with different data types can result in a TypeError
.
print(max(5, '10')) # Raises TypeError: '>' not supported between instances of 'str' and 'int'
Python’s max() and min() Functions Compared
While we’ve been focusing on the max()
function, it’s important to also consider its counterpart: the min()
function. Just as max()
identifies the largest value, min()
finds the smallest. The syntax and usage of min()
are similar to max()
, making it easy to switch between the two when needed.
print(min(5, 10, 15)) # Output: 5
print(min('apple', 'banana', 'cherry')) # Output: 'apple'
Despite their similarities, there are key differences between max()
and min()
.
Function | Returns | Usage |
---|---|---|
max() | The largest item in the iterable or the largest of two or more arguments. | max(arg1, arg2, *args[, key]) or max(iterable, *[, key, default]) |
min() | The smallest item in the iterable or the smallest of two or more arguments. | min(arg1, arg2, *args[, key]) or min(iterable, *[, key, default]) |
In ordinary practice, the only difference between the two functions is that max()
returns the largest value, while min()
returns the smallest. Otherwise they are used the same way and have the same features.
Further Resources for Python Functions
If you’re setting out to expand your knowledge about Python functions, the resources we’ve listed below could be instrumental in your journey:
- Python Built-In Functions: Quick Dive – Dive deep into Python’s functions for working with iterators and generators.
Finding Magnitude in Python with the abs() Function – Explains absolute value computation for integers and floating-point numbers.
Python filter() Function: Filtering Data with Ease – Discover Python’s “filter” function for selective item filtration in iterables.
Python Built-In Functions Guide – A Tutorialspoint walk-through of Python’s built-in functions for efficient programming.
Python’s Official Library Documentation – Explore Python’s extensive library resources in this official documentation.
Python’s Official Documentation on Built-In Functions – Get detailed insights into Python’s built-in functions.
These handpicked materials aim to augment your understanding and efficient use of Python functions, honing your skills as a Python developer.
Final Thoughts
In this comprehensive guide, we’ve delved into the max()
function in Python, a powerful built-in function that returns the largest item in an iterable or the largest of two or more arguments.
We’ve explored its syntax, shedding light on how it can be used with objects and iterables, and how the key
and default
parameters can be used to customize its behavior.
Through practical examples, we’ve seen the max()
function in action, using it with different data types and Python data structures, and demonstrating its use in various practical programming scenarios. We’ve also addressed potential errors and provided tips on how to avoid them.
Brush up on your Python knowledge with this detailed syntax reference.
With the knowledge gained from this guide, you’re now better equipped to use the max()
function effectively in your Python programs. Happy coding!