Python Bisect Module | Usage Guide (With Examples)
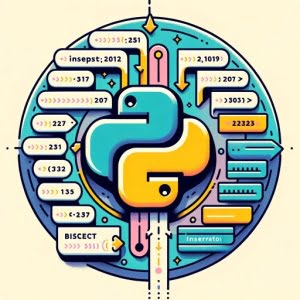
Are you finding it challenging to maintain sorted lists in Python? You’re not alone. Many developers find this task daunting, but there’s a tool that can make this process a breeze.
Like a skilled librarian, the bisect module in Python can help you keep your lists in perfect order. This module, built upon the binary search algorithm, provides support for maintaining a list in sorted order without having to sort the list after each insertion.
In this guide, we’ll walk you through the bisect module in Python, explaining how to use it effectively to manage your sorted lists. We’ll explore the bisect module’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the bisect module in Python!
TL;DR: How Do I Use the Bisect Module in Python?
The bisect module in Python provides support for maintaining a list in sorted order without having to sort the list after each insertion. To insert the number “5” into a list named
numbers
you could usebisect.insort(numbers, 5)
. Here’s a simple example:
import bisect
numbers = [1, 3, 4, 4, 6, 8]
bisect.insort(numbers, 5)
print(numbers)
# Output:
# [1, 3, 4, 4, 5, 6, 8]
In this example, we import the bisect module and use the insort
function to insert the number 5 into the sorted list numbers
. The insort
function finds the correct position for the new element and inserts it there, maintaining the sorted order of the list.
This is just a basic way to use the bisect module in Python, but there’s much more to learn about managing sorted lists efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Python Bisect Basics: bisect and insort
- Dealing with Duplicate Entries and Advanced Bisect Functions
- Exploring Alternative Approaches to Sorted Lists
- Troubleshooting Common Issues with Python Bisect
- Understanding Binary Search and the Bisect Module
- Beyond Python Bisect: Data Structures and Algorithms
- Wrapping Up: Mastering Python’s Bisect Module
Python Bisect Basics: bisect
and insort
The bisect module in Python offers two primary functions that you’ll use most often: bisect
and insort
. Let’s delve into how these functions work with sorted lists.
The bisect
Function
The bisect
function finds the insertion point for a specified element in a sorted list to maintain the sorted order. Here’s a simple example:
import bisect
numbers = [1, 3, 4, 4, 6, 8]
position = bisect.bisect(numbers, 5)
print(position)
# Output:
# 4
In this example, the bisect
function returns the position in the list where the number 5 should be inserted to maintain the sorted order. Note that the list remains unchanged. The bisect
function only provides the position and does not perform the insertion.
The insort
Function
On the other hand, the insort
function not only finds the insertion point but also inserts the element into the sorted list. Let’s see it in action:
import bisect
numbers = [1, 3, 4, 4, 6, 8]
bisect.insort(numbers, 5)
print(numbers)
# Output:
# [1, 3, 4, 4, 5, 6, 8]
In this example, the insort
function inserts the number 5 into the correct position in the list to maintain the sorted order.
The bisect
and insort
functions are powerful tools for managing sorted lists in Python. However, it’s important to note that they assume the list is already sorted. If the list is not sorted, the results may not be what you expect.
Dealing with Duplicate Entries and Advanced Bisect Functions
In Python, the bisect module provides two additional functions for more precise control over your sorted lists: bisect_left
and bisect_right
. These functions become particularly useful when dealing with duplicate entries in your list.
The bisect_left
Function
The bisect_left
function works similarly to the bisect
function, but when duplicates are present, it returns the position of the first occurrence. Let’s illustrate this with an example:
import bisect
numbers = [1, 3, 4, 4, 6, 8]
position = bisect.bisect_left(numbers, 4)
print(position)
# Output:
# 2
Here, the bisect_left
function returns the position of the first occurrence of the number 4 in the list.
The bisect_right
Function
On the other hand, the bisect_right
function (which is identical to the bisect
function) returns the position where the element should be inserted to maintain the sorted order. This position is after any existing entries of the element in the list. Let’s see this in action:
import bisect
numbers = [1, 3, 4, 4, 6, 8]
position = bisect.bisect_right(numbers, 4)
print(position)
# Output:
# 4
In this case, the bisect_right
function returns the position after the last occurrence of the number 4 in the list.
Best Practices
When dealing with sorted lists in Python, especially those with duplicate entries, it’s best to use the bisect_left
function when you need to find the position of a specific element, and the bisect_right
function when you want to maintain the sorted order of the list after insertion. Remember, the bisect module assumes your list is already sorted, so always sort your list before using these functions for the best results.
Exploring Alternative Approaches to Sorted Lists
While the bisect module is a powerful tool for maintaining sorted lists in Python, there are other methods you can use, including sorted containers and third-party libraries. Let’s explore these alternatives and their effectiveness.
Sorted Containers
Sorted containers in Python provide a way of maintaining sorted lists with a variety of different data structures. Here’s an example using a SortedList from the sortedcontainers module:
from sortedcontainers import SortedList
numbers = SortedList([1, 3, 4, 4, 6, 8])
numbers.add(5)
print(numbers)
# Output:
# SortedList([1, 3, 4, 4, 5, 6, 8])
In this example, we create a SortedList and use the add
method to insert an element while maintaining the sorted order. Sorted containers can be more flexible than the bisect module, but they may also be slower for large lists due to the overhead of maintaining the sorted data structure.
Third-Party Libraries
There are also several third-party libraries that can help with maintaining sorted lists in Python. For example, the blist module provides a sorted list type with better performance for large lists. Here’s an example:
from blist import sortedlist
numbers = sortedlist([1, 3, 4, 4, 6, 8])
numbers.add(5)
print(numbers)
# Output:
# sortedlist([1, 3, 4, 4, 5, 6, 8])
This code is similar to the sortedcontainers example, but it uses the blist module instead. The blist module can be faster for large lists, but it’s not included with Python and must be installed separately.
Making the Right Choice
When choosing a method for maintaining sorted lists in Python, consider the size of your list and the operations you need to perform. The bisect module is a great choice for small to medium lists, especially if you’re only inserting elements. For large lists or more complex operations, consider using a sorted container or a third-party library.
Troubleshooting Common Issues with Python Bisect
As with any tool, you might encounter some issues when using the bisect module in Python. Let’s discuss some common problems and how to resolve them.
Handling Non-Unique Elements
One common issue arises when handling non-unique elements in a list. The bisect module’s functions, by default, consider the rightmost position to insert an element. However, if you want to insert an element at the leftmost possible position, you can use the bisect_left
function. Here’s an example:
import bisect
numbers = [1, 3, 4, 4, 6, 8]
bisect.insort_left(numbers, 4)
print(numbers)
# Output:
# [1, 3, 4, 4, 4, 6, 8]
In this example, the insort_left
function inserts the number 4 at the leftmost possible position, resulting in the output shown.
Incorrect Ordering
Another issue might occur if your list is not sorted before using the bisect module. The bisect functions assume that the list is already sorted, so if it’s not, you might get unexpected results. Always make sure to sort your list before using these functions.
import bisect
numbers = [4, 2, 1, 6, 3]
bisect.insort(numbers, 5)
print(numbers)
# Output:
# [4, 2, 1, 5, 6, 3]
In this example, the list was not sorted before using the insort
function, so the number 5 was inserted at the wrong position. To avoid this issue, always sort your list first.
Best Practices and Tips
When using the bisect module in Python, always remember to sort your list first and consider whether you want to insert at the leftmost or rightmost possible position for non-unique elements. By understanding these considerations, you can use the bisect module more effectively to manage your sorted lists.
Understanding Binary Search and the Bisect Module
The efficiency of the bisect module in Python comes from its underlying concept: the binary search algorithm. Let’s delve into this fundamental concept and understand why the bisect module is so efficient.
The Binary Search Algorithm
Binary search is a classic algorithm in computer science. It works by repeatedly dividing in half the portion of the list that could contain the item, until you’ve narrowed down the possible locations to just one.
Here’s a simple example of how binary search works:
def binary_search(item_list, item):
low = 0
high = len(item_list) - 1
while low <= high:
mid = (low + high) // 2
guess = item_list[mid]
if guess == item:
return mid
if guess > item:
high = mid - 1
else:
low = mid + 1
return None
numbers = [1, 3, 4, 4, 6, 8]
print(binary_search(numbers, 4))
# Output:
# 2
In this example, the binary_search
function finds the position of the number 4 in the sorted list numbers
using the binary search algorithm.
Time Complexity and Efficiency
One of the main advantages of the binary search algorithm, and consequently the bisect module, is its time complexity. Binary search has a time complexity of O(log n), making it incredibly efficient for large lists. This is because with each step, it halves the part of the list that needs to be searched.
In contrast, a simple linear search, which checks each element in the list sequentially, has a time complexity of O(n). For large lists, binary search can be significantly faster. That’s why the bisect module, which is built on binary search, is such a powerful tool for handling sorted lists in Python.
Beyond Python Bisect: Data Structures and Algorithms
The bisect module in Python is not just a tool for maintaining sorted lists. It’s a practical implementation of a fundamental computer science concept: the binary search algorithm. Understanding this can open the door to a deeper understanding of data structures and algorithms.
Bisect and Data Structures
Data structures like heaps and priority queues are often used in conjunction with the bisect module. For example, a priority queue can be implemented as a sorted list, with the bisect module used to insert items at the correct position to maintain the sorted order.
Exploring Related Concepts
After mastering the bisect module, you might want to explore related concepts like heaps and priority queues. These data structures also deal with ordered collections and can be more efficient for certain operations. For example, a heap can insert and remove items in O(log n) time, making it more efficient than a sorted list for certain tasks.
Further Resources for Python Bisect Mastery
If you’re interested in learning more about the bisect module and related concepts, here are some resources you might find helpful:
- IOFlood’s Python Data Types Guide – Master Python dictionaries for efficient data organization and retrieval.
Index Retrieval in Python – Learn how to use Python indexing to retrieve specific elements and slices.
Understanding Tuples in Python – Learn how to create, access, and manipulate tuples in Python for structured data representation.
Official Documentation on Python’s Bisect Module – Python’s built-in support for bisect algorithm.
Guide on Binary Search and Bisect Module – Binary search in Python, detailed by Real Python.
Python Data Structures Guide – Comprehensive tutorial on Python data structures.
These resources offer a wealth of information on the bisect module and related topics. By exploring these resources, you can deepen your understanding of Python and computer science fundamentals.
Wrapping Up: Mastering Python’s Bisect Module
In this comprehensive guide, we’ve explored the bisect module in Python, a powerful tool for maintaining sorted lists in an efficient manner.
We began with the basics, learning how to use the bisect
and insort
functions to manage sorted lists. We then delved into more advanced usage, exploring the bisect_left
and bisect_right
functions and their applications in handling duplicate entries.
Along the way, we tackled common issues you might encounter when using the bisect module, such as handling non-unique elements and incorrect ordering, providing you with solutions and best practices for each issue.
We also looked at alternative approaches to maintaining sorted lists, comparing the bisect module with sorted containers and third-party libraries. Here’s a quick comparison of these methods:
Method | Flexibility | Speed | Ease of Use |
---|---|---|---|
Bisect Module | Moderate | High | High |
Sorted Containers | High | Moderate | Moderate |
Third-Party Libraries | High | Varies | Varies |
Whether you’re just starting out with the bisect module or you’re looking to level up your skills in managing sorted lists in Python, we hope this guide has given you a deeper understanding of the bisect module and its capabilities.
With the bisect module in your toolkit, you’re well-equipped to handle sorted lists efficiently in Python. Happy coding!