Converting Strings to Lowercase | Bash String Manipulation
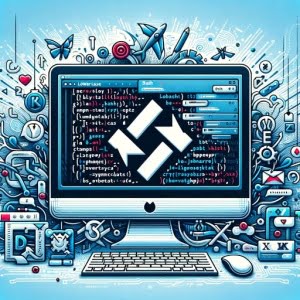
Are you finding it challenging to convert strings to lowercase in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling this task, but there’s a tool that can make this process a breeze.
Think of Bash as a skilled linguist, capable of transforming your text in various ways. These transformations can be crucial in many scripting and automation tasks, making Bash an essential tool for any developer’s toolkit.
This guide will walk you through the process of converting strings to lowercase in Bash, from basic usage to advanced techniques. We’ll explore Bash’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Bash lowercase conversion!
TL;DR: How Do I Convert Strings to Lowercase in Bash?
In Bash, you can convert strings to lowercase using the
tr
command and the syntax,tr '[:upper:]' '[:lower:]'
. This command can be used in a pipeline to transform input from uppercase to lowercase.
Here’s a simple example:
echo 'HELLO' | tr '[:upper:]' '[:lower:]'
# Output:
# 'hello'
In this example, we’re using the echo
command to output the string ‘HELLO’. This output is then piped into the tr
command, which transforms all uppercase characters into lowercase. The result is the output ‘hello’.
This is a basic way to convert strings to lowercase in Bash, but there’s much more to learn about string manipulation in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Bash Lowercase Conversion: The Basics
When it comes to converting strings to lowercase in Bash, the tr
command is your best friend. This command is a part of the standard Unix toolset and is available on virtually all Unix-like operating systems.
Understanding the tr
Command
The tr
command stands for ‘translate’ or ‘transliterate’. It reads from the standard input, translates or deletes characters, and writes the result to the standard output. In our case, we’re using it to translate uppercase characters to lowercase.
Let’s take a look at a basic example of how the tr
command can be used to convert strings to lowercase in Bash:
echo 'BASH LOWERCASE' | tr '[:upper:]' '[:lower:]'
# Output:
# 'bash lowercase'
In this example, we’re echoing the string ‘BASH LOWERCASE’ and piping it to the tr
command. The tr
command then takes this string and converts all the uppercase characters to lowercase, outputting ‘bash lowercase’.
Advantages and Potential Pitfalls
The tr
command is a powerful and flexible tool for string manipulation in Bash. It’s simple to use and can handle large amounts of data efficiently. However, it’s important to note that the tr
command only works with single-byte characters. This means it may not work as expected with multi-byte characters, such as those found in Unicode. For these situations, alternative methods may be more suitable, which we’ll discuss later in this guide.
Advanced Bash Lowercase Conversion
As you become more comfortable with the tr
command, you can start exploring its more advanced applications. One such application is converting strings to lowercase within files or scripts.
Converting Strings in Files
Suppose you have a file with several lines of text, and you want to convert all the uppercase characters to lowercase. You can do this with the tr
command combined with the cat
command in a pipeline.
Here’s an example of how you can do this:
cat myfile.txt | tr '[:upper:]' '[:lower:]' > output.txt
In this example, the cat
command reads the file ‘myfile.txt’ and pipes the content to the tr
command. The tr
command then converts all uppercase characters to lowercase. The result is then redirected to ‘output.txt’.
Converting Strings in Scripts
The tr
command can also be used within scripts to convert strings to lowercase. Here’s an example of a script that reads a string from the user, converts it to lowercase, and prints the result:
#!/bin/bash
echo 'Enter a string:'
read input_string
echo $input_string | tr '[:upper:]' '[:lower:]'
In this script, the read
command is used to get a string from the user. This string is then piped into the tr
command, which converts it to lowercase.
These examples illustrate the flexibility of the tr
command and its power in handling more complex Bash lowercase conversion tasks.
Alternative Methods for Bash Lowercase Conversion
While the tr
command is a straightforward and efficient way to convert strings to lowercase in Bash, it’s not the only tool available. Two other powerful commands that can accomplish this task are awk
and sed
. Both of these commands offer additional flexibility and can handle more complex scenarios.
Lowercase Conversion with awk
awk
is a versatile programming language designed for text processing. It’s powerful and can handle multi-byte characters, making it a suitable alternative to tr
for Unicode strings.
Here’s how you can use awk
to convert strings to lowercase:
echo 'Bash Lowercase with AWK' | awk '{print tolower($0)}'
# Output:
# 'bash lowercase with awk'
In this example, awk
reads the input string and uses the tolower
function to convert it to lowercase. The $0
variable represents the entire line of input.
Lowercase Conversion with sed
sed
, short for ‘stream editor’, is another tool for text processing. It can perform a wide range of text transformations, including converting strings to lowercase.
Here’s an example of how to use sed
for this purpose:
echo 'Bash Lowercase with SED' | sed 's/.*/\L&/g'
# Output:
# 'bash lowercase with sed'
In this command, sed
uses a substitution command (s
) to replace all characters (.*
) with their lowercase equivalents (\L
). The &
symbol represents the entire matched string.
Choosing the Right Tool
Each of these commands has its strengths. tr
is simple and efficient, but it only works with single-byte characters. awk
and sed
are more flexible and can handle multi-byte characters, but they’re also more complex.
The best tool for converting strings to lowercase in Bash depends on your specific needs. If you’re working with simple ASCII strings, tr
is likely the best choice. If you’re dealing with Unicode strings or need more complex text processing, awk
or sed
might be more suitable.
Troubleshooting Bash Lowercase Conversion
While Bash provides powerful tools for string conversion, you might encounter some challenges along the way. Let’s discuss some common issues and their solutions.
Dealing with Multi-byte Characters
As mentioned earlier, the tr
command only works with single-byte characters. This can cause unexpected results when dealing with multi-byte characters, like those in Unicode. Here’s an example:
echo 'HELLO ÆØÅ' | tr '[:upper:]' '[:lower:]'
# Output:
# 'hello æøå'
In this example, the tr
command fails to convert the multi-byte characters ‘Æ’, ‘Ø’, and ‘Å’ to lowercase. To handle these characters, you can use the awk
or sed
commands, which we discussed earlier.
Handling Special Characters
Another common issue is dealing with special characters. For instance, if you have a string with underscores and you want to convert it to lowercase, you might find that the tr
command doesn’t work as expected. Here’s an example:
echo 'HELLO_WORLD' | tr '[:upper:]' '[:lower:]'
# Output:
# 'hello_world'
In this example, the tr
command correctly converts the string ‘HELLO_WORLD’ to ‘hello_world’. However, if your string contains other special characters, such as hyphens or spaces, you might need to handle them separately.
Case Sensitivity in Scripts
When writing scripts, it’s important to remember that Bash is case sensitive. This means that ‘Hello’ and ‘hello’ are considered different strings. If your script relies on string comparisons, this can lead to unexpected results. To avoid this, you can convert all strings to lowercase before comparing them.
These are just a few of the potential issues you might encounter when converting strings to lowercase in Bash. By understanding these challenges and their solutions, you can write more robust and reliable scripts.
Understanding Bash String Manipulation
To fully grasp the process of converting strings to lowercase in Bash, it’s essential to understand the basics of string manipulation in Bash and the commands we’re using: tr
, awk
, and sed
.
String Manipulation in Bash
In Bash, a string is a sequence of characters. Bash provides various ways to manipulate these strings, such as extracting substrings, replacing substrings, and changing the case of characters.
Here’s an example of extracting a substring in Bash:
string='bash lowercase'
echo ${string:5:8}
# Output:
# 'lower'
In this example, we’re extracting a substring from the string ‘bash lowercase’. The numbers 5 and 8 specify the starting position and length of the substring, respectively.
The tr
Command
The tr
command is a standard Unix utility that translates or deletes characters. It reads from the standard input and writes to the standard output. We’ve been using tr
to convert uppercase characters to lowercase.
The awk
Command
awk
is a powerful text processing language. It’s particularly useful for data extraction and reporting. We’ve used awk
‘s tolower
function to convert strings to lowercase.
The sed
Command
sed
stands for ‘stream editor’. It’s used to perform basic text transformations on an input stream. We’ve used sed
to substitute uppercase characters with lowercase ones.
By understanding these fundamental concepts and commands, you’ll have a solid foundation for converting strings to lowercase in Bash.
Exploring Beyond Bash Lowercase Conversion
Bash lowercase conversion is just the tip of the iceberg when it comes to text manipulation in Bash. There’s a vast world of scripting and automation tasks where these skills can be applied. By mastering these, you can automate repetitive tasks, process large amounts of data, and solve complex problems.
Exploring Regular Expressions
Regular expressions are a powerful tool for matching patterns in text. They’re used in many text processing commands, including awk
and sed
. By learning regular expressions, you can perform more complex text manipulations in Bash.
# Example: Use regex to match and replace text
echo 'Hello 123 world 456' | sed 's/[0-9]*//g'
# Output:
# 'Hello world '
In this example, we’re using sed
with a regular expression ([0-9]*
) to match and remove all numbers from the string.
Delving into File Manipulation
Bash provides numerous commands for file manipulation, such as cat
, grep
, find
, and sort
. These commands can be combined with string manipulation techniques to process and analyze files.
# Example: Use grep to find a string in a file
grep 'bash' myfile.txt
# Output:
# 'bash lowercase'
In this case, we’re using grep
to search for the string ‘bash’ in the file ‘myfile.txt’.
Further Resources for Bash Mastery
To continue your journey in mastering Bash and string manipulation, here are some resources that can help:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and covers all features of the language.
Advanced Bash-Scripting Guide: This guide covers advanced topics in Bash scripting. It’s a great resource for learning more complex scripting techniques.
Bash Academy: This is an interactive learning platform for Bash. It provides hands-on exercises and detailed explanations.
By exploring these resources and practicing your skills, you can become proficient in Bash scripting and text manipulation.
Wrapping Up: Mastering Bash Lowercase Conversion
In this comprehensive guide, we’ve delved into the process of converting strings to lowercase in Bash. We’ve explored various commands and techniques, shedding light on their usage, advantages, and potential pitfalls.
We began with the basics, learning how to use the tr
command for simple Bash lowercase conversion. We then ventured into more advanced territory, discussing how to use tr
in more complex scenarios like converting strings within files or scripts.
We didn’t stop there. We explored alternative methods for Bash lowercase conversion, introducing the awk
and sed
commands as powerful tools for this task. We also tackled common issues you might encounter when converting strings to lowercase in Bash, providing solutions and workarounds for each challenge.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
tr command | Simple and efficient | Only works with single-byte characters |
awk command | Can handle multi-byte characters | More complex than tr |
sed command | Can handle multi-byte characters | More complex than tr |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of Bash lowercase conversion.
With its balance of simplicity and power, Bash is a formidable tool for text manipulation. Now, you’re well equipped to handle any string conversion task in Bash. Happy scripting!