Lombok Maven Integration | Step-by-Step Tutorial
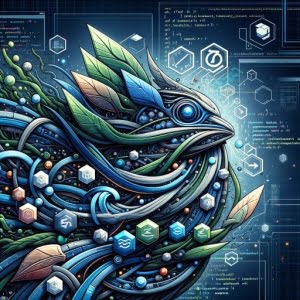
Are you finding it challenging to integrate Lombok with Maven in your Java project? You’re not alone. Many developers find themselves in a similar situation, but there’s a solution that can make this process a lot easier.
Think of Lombok as a handy tool that reduces boilerplate code, and Maven as a powerful project manager. These tools can make your Java development process more efficient and less error-prone.
This guide will walk you through the process of using Lombok and Maven together, from basic setup to advanced configurations. We’ll cover everything from adding the Lombok dependency to your Maven project, using Lombok annotations in your Java code, to more complex configurations and common issues you might encounter.
So, let’s dive in and start mastering Lombok and Maven!
TL;DR: How Do I Integrate Lombok with Maven?
To integrate
Lombok
withMaven
, you need to add the Lombok dependency to your Mavenpom.xml
file with the lines,<groupId>org.projectlombok</groupId>
,<artifactId>lombok</artifactId>
,<version>1.18.20</version>
,<scope>provided</scope>
. Here’s a simple example:
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
</dependencies>
In this example, we’ve added the Lombok dependency to the Maven pom.xml
file. The groupId
is org.projectlombok
, the artifactId
is lombok
, and the version
is 1.18.20
. The scope
is set to provided
, which means that the dependency is not required for normal use of the application, but is only available to the classpath during the compilation and testing phases.
This is a basic way to integrate Lombok with Maven, but there’s much more to learn about using these tools together. Continue reading for a more detailed guide and advanced usage scenarios.
Table of Contents
Integrating Lombok with Maven: The Basics
Now that you’ve learned the basics of adding the Lombok dependency to your Maven pom.xml file, you can start using Lombok annotations in your Java code.
Using Lombok Annotations in Java Code
Here’s a simple example of a Java class using Lombok annotations:
import lombok.Getter;
import lombok.Setter;
public class Employee {
@Getter @Setter
private String name;
@Getter @Setter
private int age;
}
In the above example, we’ve used the @Getter
and @Setter
annotations from Lombok. These annotations automatically generate getter and setter methods for the name
and age
fields of the Employee
class. This saves you from having to write these boilerplate methods yourself.
Advanced Lombok-Maven Configurations
As you become more comfortable with integrating Lombok and Maven, you can start exploring more complex configurations. This can involve using Lombok with other Maven plugins or in multi-module projects.
Using Lombok with Other Maven Plugins
Lombok can work seamlessly with other Maven plugins. For example, let’s consider the Maven Compiler Plugin. To ensure that the Lombok code is correctly compiled, you need to add the maven-compiler-plugin
to your pom.xml
and configure it as follows:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
<annotationProcessorPaths>
<path>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
</plugins>
</build>
In this configuration, the maven-compiler-plugin
is set to use Java 1.8, and Lombok is added to the annotationProcessorPaths
. This ensures that Lombok annotations are processed during the compilation phase.
Lombok in Multi-Module Projects
Lombok can also be used in multi-module Maven projects. The Lombok dependency should be added to the parent pom.xml
file, and it will be inherited by all sub-modules. Here’s an example:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>parent</artifactId>
<version>1.0</version>
<packaging>pom</packaging>
<modules>
<module>module1</module>
<module>module2</module>
</modules>
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
In the above example, the pom.xml
file is for a parent project that has two modules: module1
and module2
. The Lombok dependency is added to the parent project, so it will be available to both sub-modules.
Exploring Alternatives to Lombok
While Lombok is a powerful tool for reducing boilerplate code in Java, it’s not the only option. There are other methods and tools you can use, depending on your specific needs and preferences.
Using Other Libraries
There are several libraries that provide similar functionality to Lombok. One such library is Apache Commons Lang, which provides helper utilities for the Java language.
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
public class Employee {
private String name;
private int age;
// getters and setters
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Employee employee = (Employee) obj;
return new EqualsBuilder().append(name, employee.name).append(age, employee.age).isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37).append(name).append(age).toHashCode();
}
}
In this example, we’re using the EqualsBuilder
and HashCodeBuilder
classes from Apache Commons Lang to implement the equals()
and hashCode()
methods. This approach can reduce boilerplate code, but it’s not as concise as using Lombok annotations.
Leveraging Java’s Built-In Features
Java itself has features that can help reduce boilerplate code. For example, starting from Java 14, you can use records to create classes that are intended to be simple data carriers.
public record Employee(String name, int age) {}
In this example, we’ve defined a record Employee
with fields name
and age
. Java automatically generates constructors, getters, equals()
, hashCode()
, and toString()
methods for records, which can save a significant amount of boilerplate code.
Choosing between Lombok, other libraries, or Java’s built-in features depends on your specific needs and constraints. Consider factors such as the complexity of your project, your team’s familiarity with the tools, and the compatibility with your existing tech stack when making your decision.
Resolving Common Lombok-Maven Issues
When using Lombok with Maven, you may encounter some common issues. These can range from compilation errors to issues with Integrated Development Environment (IDE) integration. Let’s look at some of these problems and their solutions.
Compilation Errors
One of the most common issues when using Lombok with Maven is compilation errors. These can occur if the Lombok annotations are not processed correctly during the compilation phase. To resolve this, ensure that Lombok is correctly configured in your pom.xml
file, as shown in the Advanced Use section.
If you’re still facing compilation errors, try cleaning your project and rebuilding it. In Maven, you can do this using the following commands:
mvn clean
mvn install
These commands clean your project by removing all previously compiled classes and resources, and then rebuild your project.
IDE Integration Issues
Another common issue is problems with IDE integration. If your IDE does not recognize Lombok annotations, it may highlight them as errors, even though they will compile and run correctly.
To resolve this, you need to install the Lombok plugin in your IDE. The process varies depending on the IDE you’re using. For example, in IntelliJ IDEA, you can install the Lombok plugin through the ‘Plugins’ section in the ‘Settings’ menu.
Once the plugin is installed, you should restart your IDE and enable annotation processing. In IntelliJ IDEA, you can do this in the ‘Annotation Processors’ section under ‘Build, Execution, Deployment > Compiler’ in the ‘Settings’ menu.
Remember, troubleshooting is a normal part of the development process. Don’t be discouraged if you encounter issues along the way. With a bit of patience and persistence, you’ll be able to overcome them and successfully integrate Lombok with Maven in your Java projects.
Understanding Lombok and Maven
Before diving into the integration of Lombok and Maven, let’s take a step back and understand what these tools are and why they are used in Java development.
Lombok: Reducing Boilerplate Code
Lombok is a Java library that helps reduce boilerplate code. It provides annotations that can automatically generate methods like getters, setters, equals()
, hashCode()
, and toString()
.
For example, consider a simple Java class with Lombok annotations:
import lombok.Getter;
import lombok.Setter;
public class Employee {
@Getter @Setter
private String name;
@Getter @Setter
private int age;
}
In this class, we’ve used the @Getter
and @Setter
annotations from Lombok. Instead of manually writing getter and setter methods for the name
and age
fields, Lombok generates them for us. This makes our code cleaner and easier to maintain.
Maven: Powerful Project Management
Maven is a project management tool that goes beyond simple build automation. It handles project’s build, reporting, and documentation from a central piece of information: the project object model (POM), defined in a pom.xml
file.
Here’s an example of a basic pom.xml
file in a Maven project:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0</version>
</project>
This pom.xml
file defines a simple project with a groupId
of com.example
, an artifactId
of my-app
, and a version
of 1.0
. Maven uses this information to build the project and manage its dependencies.
The Power of Lombok and Maven Together
When used together, Lombok and Maven can significantly improve your Java development process. Lombok reduces boilerplate code, making your code cleaner and easier to maintain. Maven manages your project’s build process and dependencies, ensuring that your project is correctly built and that it has everything it needs to run. The integration of these tools is what we will be focusing on in this guide.
Expanding Your Lombok-Maven Knowledge
Integrating Lombok and Maven in your Java projects is just the beginning. As you become more comfortable with these tools, you can start to explore their application in larger, more complex projects. You can also delve into related topics like other Maven plugins and Java best practices.
Applying Lombok and Maven in Larger Projects
In larger Java projects, Lombok and Maven can prove to be invaluable tools. Lombok’s ability to reduce boilerplate code can significantly streamline your codebase, making it easier to read and maintain. Maven’s project management capabilities can help you manage complex dependencies and build configurations, ensuring that your project is correctly built and packaged.
Exploring Other Maven Plugins
Maven’s functionality can be extended with plugins, and there are many plugins that can enhance your Java development process. For example, the Maven Surefire Plugin can be used to run unit tests, and the Maven Javadoc Plugin can generate Javadoc documentation for your project. Exploring these plugins can help you get the most out of Maven.
Embracing Java Best Practices
In addition to using tools like Lombok and Maven, it’s also important to follow Java best practices. This includes practices related to coding style, design patterns, testing, and more. Following these practices can help you write high-quality Java code that is easy to read, maintain, and debug.
Further Resources for Lombok-Maven Integration
To deepen your understanding of Lombok and Maven, consider exploring the following resources:
- Code Efficiency with Java Packages – Learn the advantages of using packages for Java project structuring.
Lombok Data Annotation – Learn how @Data auto-generates getters, setters, and other methods for Java classes.
Maven Concepts Explained – Learn how Maven manages project dependencies and compiles source code.
Project Lombok Documentation – Comprehensive documentation covering all features of Lombok.
Apache Maven Project – Official guides and tutorials for Maven.
Baeldung’s Guide on Lombok is an in-depth guide to using Lombok in Java projects.
These resources provide a wealth of information and practical examples that can help you master the use of Lombok and Maven in your Java projects.
Wrapping Up: Mastering Lombok-Maven Integration
In this comprehensive guide, we’ve navigated through the process of integrating Lombok with Maven for efficient Java development.
We began with the basics, explaining how to add the Lombok dependency to your Maven project and use Lombok annotations in your Java code. We then ventured into more complex configurations, such as using Lombok with other Maven plugins and in multi-module projects.
We also addressed common issues that you might encounter when using Lombok with Maven, including compilation errors and IDE integration issues, and provided solutions to help you overcome these challenges.
Furthermore, we explored alternative approaches to reducing boilerplate code in Java, comparing Lombok with other libraries and Java’s built-in features. Here’s a quick comparison of the methods we’ve discussed:
Method | Boilerplate Reduction | Complexity |
---|---|---|
Lombok | High | Low |
Other Libraries (e.g., Apache Commons Lang) | Moderate | Moderate |
Java’s Built-In Features (e.g., Records) | High | Low |
Whether you’re just starting out with Lombok and Maven or you’re looking to level up your Java development skills, we hope this guide has given you a deeper understanding of Lombok-Maven integration and its benefits.
With its ability to reduce boilerplate code and streamline your Maven project, Lombok is a powerful tool for any Java developer. Now, you’re well equipped to enjoy these benefits. Happy coding!