Java Try-Catch Blocks: Usage Cases Explained
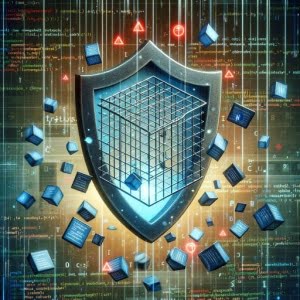
Ever found yourself stuck with handling exceptions in Java? You’re not alone. Many developers find themselves in a maze when it comes to handling exceptions in Java, but we’re here to help.
Think of the try-catch block in Java as a safety net – it’s there to save your program from unexpected crashes, much like a safety net saves a trapeze artist from a fall. It’s an essential part of Java programming that ensures the smooth execution of your code.
This guide will walk you through the basics to advanced usage of the try-catch block in Java for efficient exception handling. We’ll cover everything from the fundamental concept of exception handling, the use of try-catch blocks, to more advanced techniques and alternative approaches.
So, let’s dive in and start mastering Java exception handling with try-catch!
TL;DR: How Do I Use a Try-Catch Block in Java?
You use the try-catch block to handle exceptions in Java. This block allows you to define a region of code to be tested for errors while it’s being executed:
try
, and a region of code to be executed if an error occurs:catch
.
Here’s a simple example:
try {
// code that may throw an exception
} catch (Exception e) {
// handle exception
}
// Output:
// Depends on the exception thrown by the code inside the try block. If an exception is thrown, the code inside the catch block is executed.
In this example, we’ve used a try-catch block to handle potential exceptions. The try
block contains the code that might throw an exception, and the catch
block contains the code to handle the exception if one is thrown.
This is just a basic way to use try-catch in Java, but there’s much more to learn about exception handling. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Basic Use of Try-Catch in Java
- Handling Multiple Exceptions with Try-Catch
- Exploring Alternative Exception Handling Methods
- Troubleshooting Common Issues in Java Exception Handling
- Understanding Java’s Exception Hierarchy
- Exception Handling in Real-World Applications
- Wrapping Up: Mastering Java Exception Handling with Try-Catch
Basic Use of Try-Catch in Java
The try-catch block is the simplest and most common way of handling exceptions in Java. Let’s break down how it works.
The try
block contains the code that may potentially throw an exception. You’re essentially telling Java to ‘try’ running this code, but if an exception occurs, don’t crash the program.
The catch
block, on the other hand, is there to catch exceptions if they are thrown. This block contains the code to handle the exception, allowing the program to continue running despite the error. The type of exception that the catch
block can handle is specified in parentheses next to catch
.
Here’s a simple example of a try-catch block:
try {
int divideByZero = 5 / 0;
} catch (ArithmeticException e) {
System.out.println("An error occurred: " + e.getMessage());
}
// Output:
// An error occurred: / by zero
In this code, we’re trying to divide a number by zero, which is not allowed in mathematics and will throw an ArithmeticException
in Java. The catch
block catches this exception and prints an error message. Instead of crashing, our program handles the error gracefully and continues running.
While the try-catch block is a powerful tool, it’s important to use it wisely. Overusing it can lead to ‘exception swallowing’ where important errors are caught and ignored, making it difficult to debug issues. It’s best to only use try-catch blocks around the specific code that might throw an exception, and to handle each exception in a meaningful way.
Handling Multiple Exceptions with Try-Catch
Java allows you to handle different types of exceptions using multiple catch
blocks. This is particularly useful when your try
block contains code that can throw more than one type of exception. Each catch
block can handle a different type of exception, allowing you to respond to each exception in a specific way.
Let’s explore this with an example:
try {
String str = null;
System.out.println(str.length());
int divideByZero = 5 / 0;
} catch (ArithmeticException e) {
System.out.println("Arithmetic Exception: " + e.getMessage());
} catch (NullPointerException e) {
System.out.println("Null Pointer Exception: " + e.getMessage());
}
// Output:
// Null Pointer Exception: null
In the above code, we have two potential exceptions. The first line within the try
block can throw a NullPointerException
if str
is null
, and the second line can throw an ArithmeticException
if we try to divide by zero.
We have two catch
blocks, each handling a different type of exception. The first catch
block handles ArithmeticException
and the second handles NullPointerException
. When the exception occurs, the corresponding catch
block is executed.
It’s important to note that the order of catch
blocks matters. Java checks the catch
blocks from top to bottom, and the first one that can handle the exception is executed. Once an exception is handled, no further catch
blocks are checked. Therefore, when dealing with multiple catch
blocks, it’s best practice to place more specific exceptions before more general ones.
Handling multiple exceptions effectively can make your Java programs more robust and easier to debug, as you can provide more specific error messages and handle each exception in the most appropriate way.
Exploring Alternative Exception Handling Methods
While the try-catch block is a fundamental tool for handling exceptions in Java, it’s not the only tool available. Let’s explore some alternative methods for dealing with exceptions: the finally
block and the try-with-resources
statement.
The Finally Block
The finally
block is used to execute important code such as cleaning up resources, regardless of whether an exception was thrown or not. It always executes after the try
and catch
blocks. Here’s an example:
try {
int divideByZero = 5 / 0;
} catch (ArithmeticException e) {
System.out.println("Arithmetic Exception: " + e.getMessage());
} finally {
System.out.println("This line always executes.");
}
// Output:
// Arithmetic Exception: / by zero
// This line always executes.
In this code, the finally
block executes after the try
and catch
blocks, regardless of whether an exception was thrown.
The Try-With-Resources Statement
The try-with-resources
statement is a try statement that declares one or more resources. A resource is an object that must be closed after the program is done using it, such as a FileInputStream
or FileOutputStream
. The try-with-resources
statement ensures that each resource is closed at the end of the statement, which can help prevent resource leaks.
Here’s an example:
try (FileInputStream fis = new FileInputStream("file.txt")) {
int i = fis.read();
while (i != -1) {
System.out.print((char) i);
i = fis.read();
}
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage());
}
// Output:
// Depends on the contents of file.txt. If an exception is thrown, the IO Exception message is printed.
In this code, the FileInputStream
is declared within the parenthesis of the try
block. This makes it a resource that will be closed automatically after the try
block is executed, regardless of whether an exception was thrown.
These alternative methods provide more flexibility and can make your code safer and more efficient. However, they should be used judiciously, as they each have their own advantages and disadvantages. For example, the finally
block is great for cleanup, but it can make your code more complex. The try-with-resources
statement can prevent resource leaks, but it’s only available in Java 7 and later. Always consider the specific needs and constraints of your project when choosing how to handle exceptions.
Troubleshooting Common Issues in Java Exception Handling
While the try-catch block and its alternatives are powerful tools for handling exceptions, they are not without their challenges. Let’s discuss some common issues you may encounter during exception handling, such as unchecked exceptions and errors, and provide solutions and workarounds for each issue.
Unchecked Exceptions
Unchecked exceptions are exceptions that are not checked at compile-time but at runtime. They are subclasses of RuntimeException
, and they include NullPointerException
, ArithmeticException
, ArrayIndexOutOfBoundsException
, and others.
Unchecked exceptions can cause your program to crash if they are not handled. However, they usually indicate programming errors, such as invalid method arguments or null objects, which should be fixed in the code rather than caught and handled.
Here’s an example of an unchecked exception:
try {
String str = null;
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("Null Pointer Exception: " + e.getMessage());
}
// Output:
// Null Pointer Exception: null
In this code, we’re trying to call the length()
method on a null string, which throws a NullPointerException
. The catch block catches this exception and prints an error message.
Errors
Errors are serious problems that are not intended to be caught by the application. They are subclasses of Error
, and they include OutOfMemoryError
, StackOverflowError
, AssertionError
, and others.
Errors usually indicate a severe problem that the application should not attempt to handle, such as a OutOfMemoryError
when the JVM runs out of memory. Instead, the application should be terminated and the error should be reported for debugging.
Here’s an example of an error:
try {
int[] arr = new int[Integer.MAX_VALUE];
} catch (OutOfMemoryError e) {
System.out.println("Out of Memory Error: " + e.getMessage());
}
// Output:
// Out of Memory Error: Requested array size exceeds VM limit
In this code, we’re trying to create an array with a size greater than the maximum allowed by the JVM, which throws an OutOfMemoryError
. The catch block catches this error and prints an error message.
While it’s possible to catch and handle errors in Java, it’s generally not recommended, as errors indicate serious problems that should be fixed rather than handled. Instead, you should focus on writing robust and error-free code, and use exception handling to deal with exceptional conditions that are outside of your control.
Understanding Java’s Exception Hierarchy
Before we delve deeper into exception handling, it’s important to understand the hierarchy of exceptions in Java. At the top of the hierarchy is the Throwable
class, which has two subclasses: Exception
and Error
.
Exception Class
The Exception
class represents exceptions that can be caught and handled by the program. It has two main subclasses: IOException
(checked exceptions) and RuntimeException
(unchecked exceptions).
Checked Exceptions
Checked exceptions are exceptions that need to be declared in a method or constructor’s throws
clause if they can be thrown by the execution of the method or constructor and propagate outside the method or constructor boundary.
Here’s an example of a checked exception:
try {
File file = new File("nonexistent.txt");
FileReader fr = new FileReader(file);
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
// Output:
// File not found: nonexistent.txt (No such file or directory)
In this example, the FileReader
constructor can throw a FileNotFoundException
if the file does not exist. This is a checked exception that must be caught and handled.
Unchecked Exceptions
Unchecked exceptions are exceptions that do not need to be declared in a method or constructor’s throws
clause. They can occur anywhere in a program, and they usually indicate programming errors, such as logic errors or improper use of an API.
Error Class
The Error
class represents serious problems that a reasonable application should not try to catch. Most such errors are abnormal conditions.
Understanding the exception hierarchy and the difference between checked and unchecked exceptions can help you write better exception handling code and create more robust and reliable Java programs.
Exception Handling in Real-World Applications
In real-world Java applications, exception handling plays a crucial role. It’s not just about preventing crashes – it’s about creating applications that can handle unexpected events gracefully and continue to function reliably under various circumstances.
Exploring Related Concepts
While we’ve covered the basics of exception handling using try-catch blocks, there are more advanced concepts to explore. For instance, you might want to create custom exceptions that are specific to your application’s needs. This can make your code more readable and easier to debug, as you can throw and catch exceptions that precisely describe the problem that occurred.
Another important concept is exception propagation. In Java, unchecked exceptions are automatically propagated from methods to the calling code, while checked exceptions must be explicitly propagated by declaring them with the throws
keyword. Understanding how exception propagation works can help you design better exception handling strategies.
Further Resources for Mastering Java Exception Handling
To further deepen your understanding of Java exception handling, here are some resources you might find useful:
- IOFlood’s Guide to Java Errors presents information about handling Java runtime errors.
Exploring Try-With-Resources in Java – Learn how to automatically close resources in Java applications.
How to Properly Throw Exceptions in Java – Master custom exception handling in Java applications.
Java: A Beginner’s Guide – A comprehensive book that covers all the basics of Java, including exception handling.
Java Exception Handling Tutorial – A detailed online tutorial that delves into the nuances of exception handling in Java.
Oracle Java Documentation – The official Oracle documentation provides in-depth information about exception handling in Java.
Remember, mastering exception handling is a journey. Keep exploring, keep coding, and keep improving your skills!
Wrapping Up: Mastering Java Exception Handling with Try-Catch
In this comprehensive guide, we’ve navigated through the intricacies of Java exception handling, exploring the use of the try-catch block, its alternatives, and the various issues that may arise.
We began with the basics, understanding how to use the try-catch block in Java, and its significance in preventing unexpected program crashes. We then delved deeper, discussing the handling of multiple exceptions using multiple catch blocks, and how the order of these blocks matters.
We ventured further, exploring alternative methods of exception handling, such as the finally block and the try-with-resources statement. We also tackled common issues like unchecked exceptions and errors, providing solutions and workarounds for each.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Try-Catch Block | Handles specific exceptions, prevents crashes | Overuse can lead to exception swallowing |
Finally Block | Executes important code regardless of exceptions | Can make code more complex |
Try-With-Resources | Prevents resource leaks, automatically closes resources | Only available in Java 7 and later |
Whether you’re a beginner just starting out with Java, or an intermediate user looking to solidify your understanding of exception handling, we hope this guide has been an enlightening journey.
Exception handling is a crucial aspect of Java programming. With the knowledge gained from this guide, you’re now equipped to write more robust and reliable Java programs. Happy coding!