NPM Install Command | A Node.js Package Guide
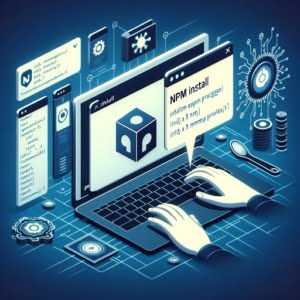
Ever found yourself scratching your head over npm package installation? At IOFLOOD, we’ve tackled this hurdle countless times. That’s why we’ve crafted a simple guide on using npm to install packages. By following our straightforward instructions, you’ll navigate package installation with ease.
This guide will walk you through the basics to advanced usage of this essential command. Whether you’re looking to understand the node_modules
directory, manage project dependencies, or explore advanced package management strategies, we’ve got you covered. With npm install
, you’re not just adding packages; you’re equipping your project with the capabilities it needs to navigate the challenges of modern web development.
Let’s simplify npm package installation and keep your development journey smooth sailing!
TL;DR: How Do I Use npm to Install Packages?
To install a package using npm, run the command
npm install <package-name>
in your project directory.
npm install <package-name>
This command adds the specified package to your project’s dependencies, ensuring that your application has access to the tools it needs. For instance, if you wanted to add the express framework to your Node.js project, you would execute:
npm install express
# Output:
# + [email protected]
# added 50 packages from 37 contributors and audited 50 packages in 2.765s
In this example, we’ve installed the Express framework, which is crucial for building web applications in Node.js. The output shows that Express and its dependencies have been added to your project. This is a fundamental step in setting up your development environment, but there’s much more to npm and package management.
Curious about managing specific versions, or troubleshooting common issues? Keep reading for more detailed instructions and insights.
Table of Contents
Starting with npm Install
Understanding npm Install Basics
When you’re new to Node.js, one of the first commands you’ll likely use is npm install
. This command is your gateway to utilizing various packages that can help enhance your project. But what exactly happens when you run this command? Let’s break it down with a simple, yet popular package: lodash.
npm install lodash
# Output:
# + [email protected]
# added 1 package in 0.524s
In this example, we installed lodash, a utility library offering a wide range of functions for programming tasks. The output indicates that lodash and its version number have been added to your project. This operation also creates or updates a node_modules
directory in your project folder, where lodash and other installed packages reside.
The Role of node_modules
The node_modules
directory is a crucial component of Node.js projects. It’s where all the packages you install via npm are stored. This setup allows Node.js to efficiently locate and load these packages when they’re needed in your code.
Understanding package.json
Another important aspect of using npm install
is the package.json
file. This file acts as a manifest for your project, outlining its dependencies, scripts, and more. When you install a package like lodash, npm adds an entry to the package.json
under dependencies
, like so:
"dependencies": {
"lodash": "^4.17.15"
}
This entry ensures that anyone else working on the project, or any deployment processes, know that lodash is a required dependency. It also locks the version of lodash to ensure consistency across environments.
By mastering these basics of npm install
, you’re laying a solid foundation for your Node.js projects. Understanding how packages are added and managed is key to building robust and maintainable applications.
Advanced npm Install Techniques
Global Package Installation
Sometimes, you might want to install a package globally on your system. This is particularly useful for tools you’ll use across projects, like the Angular CLI or Create React App. To install a package globally, you use the -g
flag with npm install
.
npm install -g create-react-app
# Output:
# + [email protected]
# added 67 packages from 41 contributors in 4.326s
This command installs Create React App globally on your system, allowing you to use it in any project. The output confirms the installation and the version of the package installed.
Development Dependencies
When working on a project, there are packages you may only need during development, like testing frameworks or build tools. These are not required in your production environment. To save a package as a development dependency, use the --save-dev
or -D
flag.
npm install jest --save-dev
# Output:
# + [email protected]
# added 345 packages from 205 contributors in 10.76s
This example shows installing Jest, a popular testing framework, as a development dependency. The output indicates the number of packages added, showcasing how npm manages these dependencies separately from your main project dependencies.
Specifying Package Versions
Control over package versions is crucial for ensuring consistency and reliability in your projects. To install a specific version of a package, you simply include the version number after the package name.
npm install [email protected]
# Output:
# + [email protected]
# added 1 package in 0.524s
This command installs a specific version of lodash, ensuring that you have the exact functionality and compatibility needed for your project. The output confirms the version installed, highlighting the precision available with npm.
By exploring these advanced npm install
options, you’re enhancing your toolkit for managing Node.js projects. Whether it’s installing packages globally, managing development dependencies, or specifying package versions, understanding these techniques allows for more efficient and controlled development workflows.
Alternative Package Managemers
npm ci: A Robust Installer
For those looking for a more stable and predictable way to manage dependencies, npm ci
presents an excellent alternative. This command is especially useful in continuous integration (CI) environments. It installs dependencies directly from package-lock.json
, ensuring that every install results in the exact same file structure in node_modules
across all installations.
npm ci
# Output:
# added 1050 packages in 10.123s
The output shows that npm ci
has installed all the packages as specified in package-lock.json
, offering a more reliable and faster installation process, particularly beneficial in automated environments.
Yarn: A Popular npm Alternative
Yarn emerged as a strong competitor to npm, offering faster installations and improvements in package management. Its caching and parallel downloading features significantly speed up the installation process.
yarn add lodash
# Output:
# success Saved 1 new dependency.
# info Direct dependencies
# └─ [email protected]
# info All dependencies
# └─ [email protected]
This code block demonstrates adding a package using Yarn. The output is more verbose, providing detailed information about the dependency added, including direct and all dependencies. Yarn’s approach to package management emphasizes transparency and speed, making it a favored choice for many developers.
npm vs. Yarn vs. npm ci
When comparing npm install
with yarn add
and npm ci
, it’s important to consider your project’s needs. npm install
is versatile, ideal for both development and production environments. npm ci
offers consistency and speed, perfect for CI pipelines. Yarn excels in performance and user experience, with features like offline package installation and more informative outputs.
Each tool has its strengths and weaknesses, and the choice often comes down to personal preference or specific project requirements. By understanding these alternatives, you can make an informed decision on the best tool for managing your Node.js project dependencies.
Solving Common npm Errors
During your development journey, you might encounter errors like EPERM
or ENOENT
when using npm install
. These errors can be frustrating but are often easily resolved with a bit of knowledge.
One common issue is the EPERM
error, which typically occurs when npm does not have the permission to write to the filesystem. This can often be resolved by adjusting the permissions of the node_modules
folder or by running the command prompt as an administrator.
# Example command to change permissions (Unix-based systems)
chmod -R 777 node_modules
Another frequent hiccup is the ENOENT
error, indicating that a required file or directory could not be found. This often happens when the package-lock.json
file is out of sync with package.json
or after merging branches. A simple solution is to delete the node_modules
folder and the package-lock.json
file, then run npm install
again.
rm -rf node_modules package-lock.json
npm install
# Output:
# added 1050 packages from 528 contributors and audited 1050 packages in 20.123s
This process refreshes your project’s dependencies, resolving discrepancies that caused the ENOENT
error. The output confirms the successful reinstallation of your packages.
Optimizing Package Installation
To optimize your npm installations, consider using the --prefer-offline
flag to speed up the process by reducing the number of requests to the npm registry. This is particularly useful when you’re reinstalling packages or working in environments with slow internet connections.
npm install --prefer-offline
# Output:
# added 1050 packages in 15.123s
This command attempts to use the local cache as much as possible, speeding up the installation process. The output shows a quicker installation time, demonstrating the effectiveness of this optimization.
By understanding and applying these troubleshooting tips and optimizations, you can navigate the common issues with npm install
and improve your package management workflow.
npm: The Heart of Node.js
The Power of Package Management
In the Node.js ecosystem, npm
stands not just as a tool but as the cornerstone for managing packages. It’s a package manager that allows developers to share and consume code, fostering a vibrant ecosystem of reusable software. The command npm install
is at the heart of this system, enabling the addition of packages to your project with ease.
Understanding package.json
At the core of any Node.js project lies the package.json
file. This file serves as the project’s manifest, outlining its dependencies, scripts, and more. When you run npm install
for a specific package, npm automatically updates this file, adding the new dependency.
{
"dependencies": {
"express": "^4.17.1"
}
}
In this code block, we’ve added Express, a fast, unopinionated, minimalist web framework for Node.js. The caret (^) before the version number tells npm to install the latest minor version available, ensuring you have the latest features that are still compatible with your project.
The Role of package-lock.json
While package.json
gives an overview of your project’s dependencies, package-lock.json
plays a critical role in ensuring consistency. This file locks down the exact versions of each package and its dependencies that were installed, making sure that every install results in the same file structure in the node_modules
directory, regardless of when or where you install them.
{
"name": "your-project-name",
"version": "1.0.0",
"lockfileVersion": 1,
"requires": true,
"dependencies": {
"express": {
"version": "4.17.1",
"resolved": "https://registry.npmjs.org/express/-/express-4.17.1.tgz",
"integrity": "sha512-11qMajnAXkb6bUYWCaq5YXnMNlcLA0YoVJjTRUFJYS4mXV6t4jQn1D6C2Nrj/mBN12pTOC4JWnRoJ4KvTrTUMw==",
"requires": {
...
}
}
}
}
This detailed snapshot from package-lock.json
shows the exact version of Express installed, including its dependencies. It ensures that anyone else working on the project or any deployment processes will have the exact same setup, reducing inconsistencies and potential errors.
Understanding the significance of npm
, along with the roles of package.json
and package-lock.json
, is essential for any Node.js developer. These elements work together to manage your project’s dependencies effectively, ensuring a stable and consistent development environment.
npm Install in CI/CD Pipelines
Integrating npm Install into CI/CD
The use of npm install
extends far beyond local development environments. In the realm of Continuous Integration/Continuous Deployment (CI/CD) pipelines, npm install
plays a pivotal role. It ensures that your Node.js applications are always built with the correct and latest versions of dependencies, thus maintaining consistency across development, testing, and production environments.
npm install --production
# Output:
# added 450 packages in 35.123s
In this example, the --production
flag is used to install only the dependencies necessary for production, omitting any development-specific packages. This is crucial for creating lightweight, efficient production builds. The output shows the number of packages added, highlighting the streamlined nature of production builds.
npm Install’s Role in Large Projects
For larger projects, npm install
facilitates the management of complex dependencies and ensures that all team members and deployment processes are working with the same set of packages. This consistency is vital for minimizing “it works on my machine” issues and streamlining the development process.
Semantic Versioning and Security
Understanding semantic versioning and utilizing npm audit
are essential practices for maintaining project security and dependency management. Semantic versioning helps manage package updates more predictably, while npm audit
scans your project for vulnerabilities, making it easier to address potential security issues.
Further Resources for Mastering npm Install
To deepen your understanding of npm and its ecosystem, consider exploring the following resources:
- The official npm Documentation provides guides and references for npm commands, including
npm install
. Node.js Guides: Node.js official guides offer insights into Node.js package management.
The npm Blog is a great resource for staying updated on the latest trends, features, and best practices in npm.
These resources offer valuable information and guidance for developers looking to enhance their skills in package management and Node.js development. Whether you’re implementing npm install
in CI/CD pipelines, managing dependencies in large projects, or ensuring your applications are secure, these resources can provide the knowledge you need to succeed.
Recap: npm Install Command Usage
In this comprehensive guide, we’ve navigated through the essential command npm install
, a cornerstone in managing Node.js project dependencies. From adding packages to your project’s ship to steering through advanced package management techniques, we’ve covered the journey of npm install
from the basics to more complex uses.
We began with the fundamentals, understanding how npm install
adds necessary packages to your project. We explored the creation of the node_modules
directory and the significance of the package.json
file, laying down the foundation for any Node.js project.
Moving forward, we delved into advanced usage scenarios, such as installing packages globally, managing development dependencies, and specifying package versions. These techniques enhance your project management skills, allowing for a more tailored and efficient development environment.
Exploring alternative approaches, we compared npm install
with other package management tools like yarn
and npm ci
, highlighting their pros and cons. This comparison equips you with the knowledge to choose the right tool for your project’s needs.
Approach | Use Case | Pros | Cons |
---|---|---|---|
npm install | General package installation | Flexible, widely used | May face version discrepancies |
npm ci | CI/CD pipelines | Fast, reliable installations | Less flexible than npm install |
Yarn | Alternative package management | Fast, caches packages | Requires separate installation |
In conclusion, whether you’re just starting out or looking to refine your package management skills, npm install
offers a robust solution for managing Node.js project dependencies. We encourage you to further explore npm’s broader ecosystem, including commands like npm audit
and npm update
, to ensure efficient and secure project management. Happy coding!