Python Case Matching: Your Ultimate Guide
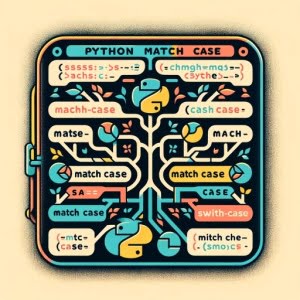
Are you finding it challenging to match cases in Python? You’re not alone. Many developers find themselves puzzled when it comes to handling case matching in Python, but we’re here to help.
Think of Python’s case matching as a detective’s tool – allowing us to find exact matches or ignore case differences, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of mastering case matching in Python, from their basic string methods to advanced regular expressions. We’ll cover everything from the basics of case matching to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Match Case in Python?
In Python, you can use the
str.lower()
orstr.upper()
methods for case-insensitive matching, or the==
operator for case-sensitive matching.
Here’s a simple example:
word = 'Python'
print(word.lower() == 'python') # True
print(word == 'python') # False
# Output:
# True
# False
In this example, we have a string ‘Python’. We use the str.lower()
method to convert it to lowercase and then compare it with the lowercase string ‘python’. The ==
operator checks for case-sensitive matching and returns True
. However, when we directly compare ‘Python’ with ‘python’ using the ==
operator, it returns False
because it’s case-sensitive.
This is a basic way to match case in Python, but there’s much more to learn about case matching in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basics of Case Matching in Python
In Python, we have some straightforward methods to perform case matching. Let’s dive into the str.lower()
, str.upper()
, and ==
methods, and see how they are used for case matching in Python.
The str.lower()
and str.upper()
Methods
The str.lower()
method converts all uppercase characters in a string to lowercase, and the str.upper()
method does the opposite – it converts all lowercase characters in a string to uppercase. These methods are very useful when we want to perform case-insensitive matching.
Here’s a simple example:
word = 'Python'
print(word.lower() == 'python') # True
print(word.upper() == 'PYTHON') # True
# Output:
# True
# True
In this example, we first convert the ‘Python’ string to lowercase using the str.lower()
method and then compare it with ‘python’. The result is True
because both strings are now in the same case. We do the same with the str.upper()
method and also get True
.
The ==
Operator
The ==
operator checks for exact, case-sensitive matching. If the cases of the characters do not match, it will return False
.
print('Python' == 'python') # False
# Output:
# False
In this example, ‘Python’ and ‘python’ are not considered equal by the ==
operator because the cases of the characters do not match.
While these methods are simple and effective, they do have their limitations. For example, they may not handle special characters or non-English letters correctly. In the following sections, we’ll explore more advanced techniques for case matching in Python.
Using Regex for Case Matching
As we dive deeper into case matching in Python, we encounter an extremely powerful tool: regular expressions. Regular expressions, often abbreviated as regex, provide a way to match complex patterns within strings. In the context of case matching, regex can be used for both case-sensitive and case-insensitive matching.
Case-Sensitive Matching with Regex
For case-sensitive matching, we can use the re.match()
function. This function attempts to match a pattern at the start of a string.
Here’s an example:
import re
pattern = 'Python'
text = 'Python is fun'
match = re.match(pattern, text)
print(bool(match)) # True
# Output:
# True
In this example, we’re using the re.match()
function to match the pattern ‘Python’ at the start of the string ‘Python is fun’. The function returns a match object if the pattern is found, or None
if it’s not. We’re converting the match object to a boolean using the bool()
function, so it prints True
when the pattern is found.
Case-Insensitive Matching with Regex
For case-insensitive matching, we can use the re.IGNORECASE
or re.I
flag with the re.match()
function.
Here’s an example:
import re
pattern = 'python'
text = 'Python is fun'
match = re.match(pattern, text, re.I)
print(bool(match)) # True
# Output:
# True
In this example, we’re using the re.I
flag to ignore case differences. So, even though the pattern ‘python’ is in lowercase and the text ‘Python is fun’ starts with an uppercase ‘P’, the function still finds a match.
Regular expressions are a powerful tool for case matching in Python, but they can be complex and may be overkill for simple matching tasks. However, they are essential when you need to match complex patterns or perform case-insensitive matching at a level beyond what the str.lower()
or str.upper()
methods can provide.
Alternative Tools for Case Matching
While the basic and advanced methods we’ve discussed are commonly used for case matching in Python, there are other alternatives that offer unique advantages. In this section, we’ll explore the str.casefold()
method and third-party libraries for case matching.
The str.casefold()
Method
The str.casefold()
method is similar to str.lower()
, but it’s more aggressive. It’s designed to remove all case distinctions in a string, even in cases that str.lower()
can’t handle correctly.
Here’s an example:
word = 'Python'
print(word.casefold() == 'python') # True
# Output:
# True
In this example, str.casefold()
converts ‘Python’ to lowercase and then compares it with ‘python’. The result is True
.
The str.casefold()
method can be especially useful when dealing with non-English characters that have case differences not handled by str.lower()
or str.upper()
.
Third-Party Libraries
There are also third-party libraries available for case matching in Python. These libraries can provide additional functionality beyond what’s available in the standard library.
For instance, the fuzzywuzzy
library can be used for fuzzy string matching, which allows for matches that are approximately equal. This can be useful when you need to match strings that may have minor differences.
Here’s an example:
from fuzzywuzzy import fuzz
ratio = fuzz.ratio('Python', 'python')
print(ratio) # 83
# Output:
# 83
In this example, we’re using the fuzz.ratio()
function from the fuzzywuzzy
library to compare ‘Python’ and ‘python’. The function returns a ratio that represents the similarity between the two strings, with 100 being a perfect match. Despite the case difference, the function returns a high ratio of 83, indicating a strong match.
While third-party libraries can provide powerful tools for case matching, they also introduce additional dependencies to your project. Therefore, they should be used judiciously, especially in larger projects where managing dependencies can become complex.
Solving Errors in Case Matching
While Python provides powerful tools for case matching, there can be some bumps along the way. In this section, we’ll discuss some common issues you might encounter, including unexpected results due to locale settings or Unicode characters, and provide solutions and workarounds.
Dealing with Locale Settings
One common issue in Python case matching involves locale settings. The behavior of the str.lower()
and str.upper()
methods can be affected by the locale settings of your environment. This can lead to unexpected results when dealing with certain characters.
Here’s an example:
import locale
locale.setlocale(locale.LC_ALL, 'tr_TR.utf8')
print('I'.lower()) # 'ı'
# Output:
# 'ı'
In this example, we’re setting the locale to Turkish (‘tr_TR.utf8’). In Turkish, the lowercase of ‘I’ is ‘ı’, not ‘i’. So, the str.lower()
method returns ‘ı’, not ‘i’. This can cause issues in case matching if not handled correctly.
One way to avoid this issue is to use the str.casefold()
method, which is not affected by locale settings.
Handling Unicode Characters
Another common issue involves Unicode characters. Some Unicode characters have unique case folding behavior that can lead to unexpected results in case matching.
Here’s an example:
print('ß'.upper().lower()) # 'ss'
# Output:
# 'ss'
In this example, the uppercase of ‘ß’ is ‘SS’, and the lowercase of ‘SS’ is ‘ss’. So, the sequence str.upper().str.lower()
changes ‘ß’ to ‘ss’, which can cause issues in case matching.
One way to handle this issue is to use the str.casefold()
method, which handles such Unicode characters correctly.
Keep in mind that while these methods can help you navigate the complexities of case matching in Python, understanding the nuances of your data and the specific requirements of your project is crucial for choosing the best approach.
Overview of Strings and Cases
Before we delve deeper into case matching, it’s crucial to understand Python’s string data type and how case matching works in Python. This understanding will provide a solid foundation for mastering case matching.
Python Strings: A Brief Overview
In Python, strings are sequences of characters. They can be created by enclosing characters in quotes. Python treats single quotes the same as double quotes.
Here’s an example:
string1 = 'Hello, World!'
string2 = "Hello, World!"
print(string1 == string2) # True
# Output:
# True
In this example, string1
and string2
are the same string, even though one is enclosed in single quotes and the other in double quotes.
Case Matching in Python: The Basics
Case matching in Python involves comparing strings while considering (case-sensitive matching) or ignoring (case-insensitive matching) the case of the characters. Python provides several methods for case matching, including str.lower()
, str.upper()
, str.casefold()
, and the ==
operator.
Here’s an example of case-sensitive matching:
print('Python' == 'python') # False
# Output:
# False
And here’s an example of case-insensitive matching:
print('Python'.lower() == 'python'.lower()) # True
# Output:
# True
In these examples, the ==
operator performs case-sensitive matching, while the str.lower()
method is used for case-insensitive matching.
The Importance of Case Matching
Case matching plays a crucial role in many areas of programming and data analysis. It’s used in tasks like data cleaning, text processing, and user input validation. A solid understanding of case matching in Python will serve you well in a wide range of scenarios, from simple scripting to complex data analysis.
The Relevance of Case Matching
Case matching in Python extends beyond just syntax and commands. It plays a vital role in various real-world applications, including text processing and data cleaning. Understanding and mastering case matching can significantly enhance your Python programming skills and open up new possibilities.
Case Matching in Text Processing
In text processing, case matching is often used to standardize or normalize text data. For instance, when analyzing text data, it’s common to convert all text to lowercase (case-insensitive matching) to ensure that the analysis is not affected by case differences.
Data Cleaning with Case Matching
In data cleaning, case matching helps in identifying and handling duplicates. For instance, ‘Python’ and ‘python’ might be considered as two different values due to case differences. By converting all values to the same case, we can easily identify and handle such duplicates.
Exploring Related Concepts
Once you’ve mastered case matching in Python, you might want to explore related concepts like string manipulation and regular expressions. String manipulation involves various techniques to modify or analyze strings, while regular expressions provide a powerful tool for matching complex patterns within strings.
Further Resources for Python Strings
For a deeper understanding of case matching and string management in Python, you might find the following resources helpful:
- Python String Mastery: From Novice to Pro: Journey from novice to pro in Python string mastery with this comprehensive guide, covering all you need to know about Python text manipulation.
Python Substring Quick Reference: This quick reference guide provides an overview of slicing and substring techniques in Python, along with examples and explanations for extracting substrings from strings using different methods.
New Line in Python: Guide, Uses, and Examples: Learn about different ways to add new line characters in Python strings, along with examples and use cases for formatting output and creating multiline strings.
Python Documentation on String Methods: This official Python documentation provides a detailed overview of string methods, including those used for case matching.
Python Regular Expressions Tutorial: This tutorial from W3Schools provides a comprehensive guide to regular expressions in Python.
Python String Manipulation Tutorial: Real Python offers an in-depth tutorial on string manipulation in Python, covering a wide range of topics including case matching.
Recap: Case Matching in Python
In this comprehensive guide, we’ve delved into the world of case matching in Python. We’ve explored the basics to the advanced techniques, helping you to understand and master the process of case matching in Python.
We began with the basics, learning how to use the str.lower()
, str.upper()
, and ==
methods for case matching. We then ventured into the world of regular expressions, harnessing their power for both case-sensitive and case-insensitive matching.
Along the way, we tackled common issues you might encounter during case matching, such as unexpected results due to locale settings or Unicode characters, and provided solutions and workarounds for each issue.
We also explored alternative approaches to case matching, including the str.casefold()
method and third-party libraries like fuzzywuzzy
.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
str.lower() , str.upper() , == | Simple and easy to use | May not handle special characters or non-English letters correctly |
Regular expressions | Powerful, can match complex patterns | Can be complex, may be overkill for simple tasks |
str.casefold() | Handles case differences not handled by str.lower() or str.upper() | More aggressive than str.lower() or str.upper() |
Third-party libraries | Can provide additional functionality | Introduce additional dependencies |
Whether you’re just starting out with case matching in Python or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of case matching and its capabilities.
With the knowledge and skills you’ve gained from this guide, you’re well equipped to handle case matching in Python. Happy coding!