Bubble Sort Java: How to Implement and Optimize
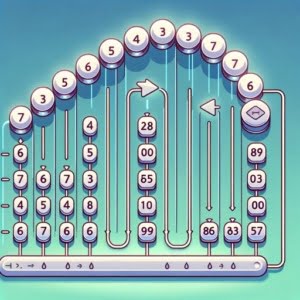
Are you finding it challenging to implement bubble sort in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling sorting algorithms in Java, but we’re here to help.
Think of bubble sort as a meticulous librarian – it helps to bring order to your data, making it easier for you to find what you’re looking for. Bubble sort is a simple and intuitive sorting algorithm, but it’s essential to understand its ins and outs for efficient usage.
This guide will walk you through the process of implementing bubble sort in Java, from the basics to more advanced techniques. We’ll cover everything from understanding the algorithm, writing the code, to optimizing it for better performance.
So, let’s dive in and start mastering bubble sort in Java!
TL;DR: How Do I Implement Bubble Sort in Java?
Implementing bubble sort in Java involves creating a function that uses nested loops to compare and swap adjacent elements in an array. Here’s a simple example:
public void bubbleSortString(String arr[]) {
int n = arr.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
// using compareTo for string comparison
if (arr[j].compareTo(arr[j+1]) > 0) {
// swap arr[j+1] and arr[j]
String temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
In this function, compareTo
is used to compare two Strings. If the current string comes after the next string lexicographically, they are swapped. This process continues until the array is sorted in lexicographic order.
This is a basic implementation of bubble sort in Java, but there’s much more to learn about optimizing this algorithm and understanding its time complexity. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding and Implementing Bubble Sort in Java
- Optimizing Bubble Sort in Java
- Exploring Other Sorting Algorithms in Java
- Troubleshooting Bubble Sort Implementation in Java
- Unraveling the Concept of Sorting Algorithms
- The Relevance of Sorting Algorithms: Beyond Code
- Wrapping Up: Mastering Bubble Sort in Java
Understanding and Implementing Bubble Sort in Java
Bubble sort is a simple sorting algorithm that works by repeatedly stepping through the list, comparing each pair of adjacent items, and swapping them if they are in the wrong order. The pass through the list is repeated until the list is sorted.
Let’s break down how bubble sort works with a simple Java implementation.
public void bubbleSort(int arr[]) {
int n = arr.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1]) {
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
In this code, we start with a function called bubbleSort
that takes an array of integers as an argument. The outer loop runs from the first element to the last, and the inner loop compares each pair of elements. If the current element (arr[j]
) is greater than the next element (arr[j+1]
), they are swapped. This process continues until the array is sorted.
The time complexity of Bubble Sort is O(n^2) in both average and worst-case scenarios, where n is the number of items being sorted. This makes it inefficient on large lists, and generally it is used only for educational purposes or small lists where its inefficiency is not a high penalty.
In the next section, we’ll look at how to optimize this algorithm to improve its efficiency.
Optimizing Bubble Sort in Java
While bubble sort is simple to understand and implement, it’s not the most efficient sorting algorithm. However, with a few tweaks, we can optimize it to improve its performance.
One common optimization is to use a boolean variable to check if any swapping occurred in a pass. If no swapping occurred, it means the array is already sorted, and we can skip the remaining passes.
Here’s an example of an optimized bubble sort in Java:
public void optimizedBubbleSort(int arr[]) {
int n = arr.length;
boolean swapped;
for (int i = 0; i < n-1; i++) {
swapped = false;
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
swapped = true;
}
}
if (!swapped)
break;
}
}
In this code, we’ve added a swapped
variable that is set to true
whenever we make a swap. If we finish a pass without making any swaps (swapped
is still false
), we break out of the loop early, saving unnecessary iterations.
While this optimization doesn’t change the worst-case time complexity (still O(n^2)), it can significantly improve the best-case time complexity to O(n) when the input array is already sorted or nearly sorted. This makes bubble sort more efficient in certain scenarios, but there are still more efficient sorting algorithms for general use, which we’ll discuss in the next section.
Exploring Other Sorting Algorithms in Java
While bubble sort is a great starting point for understanding sorting algorithms, there are several other algorithms that offer better performance in many scenarios. Two such algorithms are Quick Sort and Merge Sort.
Quick Sort in Java
Quick sort is a divide-and-conquer algorithm that works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. Here’s a simple implementation of quick sort in Java:
public void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
private int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
// swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// swap arr[i+1] and arr[high] (or pivot)
int temp = arr[i+1];
arr[i+1] = arr[high];
arr[high] = temp;
return i+1;
}
Merge Sort in Java
Merge sort is another divide-and-conquer algorithm that works by dividing the unsorted list into n sublists, each containing one element (a list of one element is considered sorted), and then repeatedly merges sublists to produce new sorted sublists until there is only one sublist remaining. Here’s a simple implementation of merge sort in Java:
public void mergeSort(int arr[], int l, int r) {
if (l < r) {
int m = (l+r)/2;
mergeSort(arr, l, m);
mergeSort(arr , m+1, r);
merge(arr, l, m, r);
}
}
private void merge(int arr[], int l, int m, int r) {
// merge logic here
}
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(n^2) |
Quick Sort | O(n log(n)) | O(n log(n)) | O(n^2) |
Merge Sort | O(n log(n)) | O(n log(n)) | O(n log(n)) |
As you can see, both quick sort and merge sort offer better average and worst-case performance than bubble sort. However, they are also more complex and can be more difficult to understand and implement. Therefore, while bubble sort may not be the most efficient sorting algorithm, it’s a valuable tool for learning the basics of how sorting algorithms work.
Troubleshooting Bubble Sort Implementation in Java
Even with a straightforward algorithm like bubble sort, it’s common to encounter issues during implementation. One of the most common problems is the off-by-one error. This error typically occurs when you access an array out of its bounds, leading to unexpected results or a runtime error.
Off-By-One Errors in Bubble Sort
Consider this incorrect implementation of bubble sort:
public void faultyBubbleSort(int arr[]) {
int n = arr.length;
for (int i = 0; i < n; i++)
for (int j = 0; j < n; j++)
if (arr[j] > arr[j+1]) {
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
In this code, the inner loop attempts to access arr[j+1]
, which will be out of bounds on the last iteration. This will throw an ArrayIndexOutOfBoundsException
.
The solution is to ensure the inner loop only runs up to n-i-1
, as in the correct implementation of bubble sort. This prevents the loop from going beyond the bounds of the array.
public void bubbleSort(int arr[]) {
int n = arr.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1]) {
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
By understanding the common issues and their solutions, you can avoid these pitfalls and implement bubble sort correctly in your Java programs.
Unraveling the Concept of Sorting Algorithms
Sorting algorithms are fundamental to the field of computer science and have a wide range of applications in the real world. From organizing files on your computer to ranking search engine results, sorting algorithms are the invisible force that brings order to our digital world.
What are Sorting Algorithms?
A sorting algorithm is a method that takes a list or array of items and arranges them in a specific order. The order could be numerical (ascending or descending) or lexicographical (A-Z or Z-A).
// Unsorted array
int[] arr = {4, 2, 9, 7, 5, 1};
// Sorted array (ascending)
int[] sortedArr = {1, 2, 4, 5, 7, 9};
In the code block above, we start with an unsorted array of integers. After applying a sorting algorithm, we get a new array where the integers are in ascending order.
Why are Sorting Algorithms Important?
Sorting algorithms play a crucial role in optimizing the efficiency of other algorithms. They make it easier to search for items, check for duplicates, and find the minimum or maximum values. Without efficient sorting, these tasks could take significantly longer, especially with large datasets.
In addition to their practical applications, sorting algorithms are also an excellent way to understand fundamental concepts in computer science, like algorithmic complexity, divide-and-conquer strategies, and data structures.
In the next section, we’ll explore how these concepts apply to bubble sort, a simple yet powerful sorting algorithm.
The Relevance of Sorting Algorithms: Beyond Code
Sorting algorithms, including bubble sort, hold a significant place in the realm of computer science education and coding interviews. They form the backbone of data structures and algorithm courses, and are often used as a yardstick to gauge a candidate’s problem-solving and coding skills.
Sorting Algorithms in Computer Science Education
Understanding sorting algorithms is crucial for mastering data structures and algorithms – a core area of computer science. The study of sorting algorithms provides insight into algorithm design, complexity analysis, and problem-solving strategies. Implementing bubble sort in Java, for instance, allows you to understand the concept of nested loops and how to manipulate array indices.
Sorting Algorithms in Coding Interviews
In coding interviews, sorting algorithms are a common topic. They are used not only to test your coding skills but also to see how you approach problem-solving, how you handle edge cases, and how you optimize the solution. Having a firm grasp of sorting algorithms, including bubble sort, can give you an edge in these interviews.
Exploring Related Concepts
Once you’re comfortable with sorting algorithms, you can explore related concepts like search algorithms and data structures. Search algorithms, such as binary search and depth-first search, are used to find specific items in data structures. Understanding these algorithms can help you write more efficient code.
Data structures, such as arrays, linked lists, and binary trees, are used to store and organize data in a way that makes it efficient to perform operations like sorting and searching. Learning about these data structures and how they interact with sorting algorithms can deepen your understanding of computer science.
Further Resources for Mastering Sorting Algorithms
To further your understanding of sorting algorithms, here are some resources that provide in-depth explanations and examples:
- Beginner’s Guide to Java Stream – Master the use of Java streams for filtering, mapping, and reducing data elements.
Selection Sort in Java: Basics – Discover how to implement the selection sort algorithm in Java for sorting arrays or lists
Exploring Merge Sort in Java – Master Java merge sort implementation for improved performance in data processing.
GeeksforGeeks’ Sorting Algorithms resource provides detailed tutorials on various sorting algorithms.
Algorithms in Computer Science – A course on algorithms, including videos, practice exercises, and quizzes.
Data Structures and Algorithms Specialization from Coursera covers data structures and algorithms.
Wrapping Up: Mastering Bubble Sort in Java
In this comprehensive guide, we’ve delved into the process of implementing bubble sort in Java, starting from the basics and moving towards more advanced techniques. We’ve explored how this simple yet powerful sorting algorithm can be a great tool for understanding the workings of sorting algorithms.
We started with the basics, learning how to implement bubble sort in Java. We then delved into more advanced usage, such as optimizing the algorithm to improve its efficiency. We also discussed common issues that you might encounter when implementing bubble sort, such as off-by-one errors, and provided solutions to these problems.
Additionally, we explored other sorting algorithms that can be used in Java, such as quick sort and merge sort. We discussed the advantages and disadvantages of these algorithms compared to bubble sort, giving you a broader perspective of sorting algorithms in Java.
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(n^2) |
Quick Sort | O(n log(n)) | O(n log(n)) | O(n^2) |
Merge Sort | O(n log(n)) | O(n log(n)) | O(n log(n)) |
Whether you’re just starting out with bubble sort or you’re looking to deepen your understanding of sorting algorithms, we hope this guide has been helpful. Bubble sort, with its simplicity, serves as a great starting point in the world of sorting algorithms. With the knowledge you’ve gained, you’re now better equipped to tackle more complex sorting algorithms. Happy coding!