Merge Sort Algorithm in Java: A Detailed Tutorial
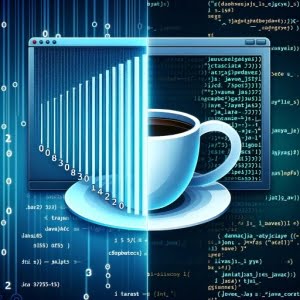
Are you finding it challenging to sort data in your Java programs? You’re not alone. Many developers grapple with this task, but there’s an algorithm that can make this process a breeze.
Like a skilled organizer, Merge Sort is a handy tool that can efficiently sort a list of data in ascending or descending order. This algorithm, when implemented in Java, can streamline your data sorting tasks and make your code more efficient.
This guide will walk you through the process of implementing Merge Sort in Java. We’ll explore Merge Sort’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Merge Sort in Java!
TL;DR: How Do I Implement Merge Sort in Java?
To implement Merge Sort in Java, you’ll create a recursive function with a call such as:
mergeSort(array, 0, array.length - 1);
. This will need to be further defined to split the array, sort the halves, and merge them. Here’s a simple example of Merge Sort implementation in Java:
public class Main {
public static void main(String[] args) {
int[] array = {90, -4, 8, 2, 6, -30};
mergeSort(array, 0, array.length - 1);
for (int i : array) {
System.out.print(i + " ");
}
}
static void mergeSort(int[] array, int start, int end) {
if (start < end) {
int mid = (start + end) / 2;
mergeSort(array, start, mid);
mergeSort(array, mid + 1, end);
merge(array, start, mid, end);
}
}
// Merge function here
}
# Output:
# -30 -4 2 6 8 90
In this example, we’ve created a mergeSort
function that recursively splits and sorts the array. The sorted halves are then merged back together. The result is a sorted array in ascending order.
This is just a basic way to implement Merge Sort in Java, but there’s much more to learn about this powerful sorting algorithm. Continue reading for a more detailed guide on how to implement and understand Merge Sort in Java.
Table of Contents
Implementing Merge Sort in Java: The Basics
Before we dive into the advanced aspects of Merge Sort, let’s first understand how to implement it in Java at a basic level. Implementing Merge Sort involves creating a recursive function that splits the input array, sorts the halves, and merges them.
Here’s a step-by-step guide to implementing Merge Sort in Java:
- Declare Your Array:
First, you need to declare an array that you want to sort. Let’s say we have an array of integers that looks like this:
int[] array = {90, -4, 8, 2, 6, -30};
- Call the Merge Sort Function:
Next, you need to call the mergeSort
function on your array. We’ll define this function in the next step.
mergeSort(array, 0, array.length - 1);
In this command, we’re passing three arguments to the mergeSort
function: the array we want to sort, the starting index of the array (0 in this case), and the ending index of the array (which is array.length - 1
).
- Define the Merge Sort Function:
The mergeSort
function is a recursive function that splits the array into two halves, sorts them, and then merges them. Here’s how you can define this function:
static void mergeSort(int[] array, int start, int end) {
if (start < end) {
int mid = (start + end) / 2;
mergeSort(array, start, mid);
mergeSort(array, mid + 1, end);
merge(array, start, mid, end);
}
}
In this function, we’re first checking if the start
index is less than the end
index. If it is, we calculate the middle index of the array (mid
) and then recursively call the mergeSort
function on the two halves of the array. Finally, we call the merge
function to merge the two sorted halves. We’ll define the merge
function in the next step.
- Define the Merge Function:
The merge
function is used to merge the two sorted halves of the array. We’ll define this function in the Advanced Use section.
After you’ve defined your mergeSort
function and called it on your array, you should have a sorted array. Here’s what your sorted array should look like:
# Output:
# -30 -4 2 6 8 90
And that’s it! That’s how you can implement Merge Sort in Java at a basic level. Remember, this is just the beginning. There’s a lot more to learn about Merge Sort, including its time complexity, space complexity, and how it compares to other sorting algorithms. So, let’s keep going!
Understanding Merge Sort’s Complexities
Now that we’ve covered the basics of implementing Merge Sort in Java, let’s delve a bit deeper. In this section, we’ll discuss the time complexity and space complexity of Merge Sort and provide a more complex example.
Time Complexity of Merge Sort
The time complexity of an algorithm is a measure of the amount of time it takes to run. In the case of Merge Sort, the time complexity is O(n log n), both for the best case and the worst case scenarios. This is because the algorithm consistently divides the array in half during the sorting process.
Space Complexity of Merge Sort
The space complexity of an algorithm is a measure of the amount of memory it needs to run. The space complexity of Merge Sort is O(n), because it requires additional space to hold the helper array, which is used for merging.
A More Complex Example
Let’s now look at a more complex example of Merge Sort in Java, where we define the merge
function that we mentioned in the basic example:
static void merge(int[] array, int start, int mid, int end) {
int[] temp = new int[end - start + 1];
int i = start, j = mid + 1, k = 0;
while (i <= mid && j <= end) {
if (array[i] <= array[j]) {
temp[k] = array[i];
k += 1; i += 1;
} else {
temp[k] = array[j];
k += 1; j += 1;
}
}
while (i <= mid) {
temp[k] = array[i];
k += 1; i += 1;
}
while (j <= end) {
temp[k] = array[j];
k += 1; j += 1;
}
for (i = start; i <= end; i += 1) {
array[i] = temp[i - start];
}
}
In this function, we’re first creating a temporary array to hold the sorted elements. We then use two pointers (i
and j
) to traverse the two halves of the array, and a third pointer (k
) to track the current index of the temporary array. We compare the elements at the i
and j
indices of the array, and place the smaller one in the temporary array. We then increment the pointers accordingly. Finally, we copy the sorted elements from the temporary array back into the original array.
This function, when used in conjunction with the mergeSort
function we defined earlier, forms a complete implementation of Merge Sort in Java.
Exploring Alternatives: Quick Sort and Bubble Sort
While Merge Sort is a powerful and efficient algorithm for sorting data in Java, it’s not the only one. Other popular sorting algorithms include Quick Sort and Bubble Sort. Let’s take a closer look at these alternatives and compare their efficiencies.
Quick Sort
Quick Sort is a divide-and-conquer algorithm, much like Merge Sort. However, it works a bit differently. It selects a ‘pivot’ element from the array and partitions the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The process is then recursively applied to the sub-arrays.
Here’s a basic implementation of Quick Sort in Java:
static void quickSort(int[] array, int low, int high) {
if (low < high) {
int pi = partition(array, low, high);
quickSort(array, low, pi - 1);
quickSort(array, pi + 1, high);
}
}
// Partition function here
# Output:
# -30 -4 2 6 8 90
In this example, we first partition the array around a pivot, then recursively apply the quickSort
function to the partitions. The partition
function is not defined in this snippet, but it is crucial for the Quick Sort algorithm.
Quick Sort’s average and best case time complexity is O(n log n), but its worst-case time complexity is O(n^2), which occurs when the array is already sorted, and the chosen pivot is the smallest or largest element.
Bubble Sort
Bubble Sort is a simpler algorithm that repeatedly steps through the array, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the array is repeated until the array is sorted.
Here’s a basic implementation of Bubble Sort in Java:
static void bubbleSort(int[] array) {
int n = array.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (array[j] > array[j+1]) {
// swap array[j+1] and array[j]
int temp = array[j];
array[j] = array[j+1];
array[j+1] = temp;
}
}
# Output:
# -30 -4 2 6 8 90
In this example, we repeatedly traverse the array and swap adjacent elements if they are in the wrong order. This process continues until the array is sorted.
Bubble Sort’s time complexity is O(n^2) for all cases, making it less efficient than both Merge Sort and Quick Sort, especially for large data sets.
In conclusion, while Merge Sort, Quick Sort, and Bubble Sort can all be used to sort data in Java, Merge Sort is generally the most efficient. However, the best algorithm to use depends on the specific requirements of your project.
Troubleshooting Merge Sort in Java
While implementing Merge Sort in Java, you might encounter some common issues. Let’s discuss them and provide solutions to resolve these problems.
Out of Memory Error
Merge Sort requires additional space proportional to the input size. If you’re working with a large dataset, you might encounter an OutOfMemoryError
. To avoid this, ensure your environment has enough memory to handle the data. If not, consider using an in-place sorting algorithm like Quick Sort.
Stack Overflow Error
Since Merge Sort is a recursive algorithm, a StackOverflowError
might occur with a very large dataset. This is because there’s a limit to the depth of the stack trace, and if you exceed that limit, a StackOverflowError
will be thrown. To resolve this, you can increase the stack size, but this is generally not recommended. Instead, consider using an iterative version of Merge Sort or a different sorting algorithm that uses less stack space.
Incorrect Sorting
If your array isn’t sorted correctly, double-check your merge
function. A common mistake is incorrectly merging the two halves of the array. Make sure you’re comparing the correct elements and placing them in the right order in your temporary array.
Here’s the correct implementation of the merge
function:
static void merge(int[] array, int start, int mid, int end) {
int[] temp = new int[end - start + 1];
int i = start, j = mid + 1, k = 0;
while (i <= mid && j <= end) {
if (array[i] <= array[j]) {
temp[k] = array[i];
k += 1; i += 1;
} else {
temp[k] = array[j];
k += 1; j += 1;
}
}
// Copy the remaining elements
while (i <= mid) {
temp[k] = array[i];
k += 1; i += 1;
}
while (j <= end) {
temp[k] = array[j];
k += 1; j += 1;
}
// Copy the sorted elements back into the original array
for (i = start; i <= end; i += 1) {
array[i] = temp[i - start];
}
}
In this function, we’re correctly comparing the elements at the i
and j
indices and placing the smaller one in the temporary array. We then increment the pointers accordingly. Finally, we copy the sorted elements from the temporary array back into the original array. This function should correctly sort your array in ascending order.
By understanding these common issues and their solutions, you can implement Merge Sort in Java more effectively and troubleshoot any problems that arise.
Understanding Sorting Algorithms and Their Importance
Sorting algorithms are fundamental to programming and computer science. They arrange data in a particular order, which can be numerical (ascending or descending) or lexicographical (A-Z or Z-A). Sorting makes data more understandable and easier to analyze. It also optimizes data searching, as sorted data allows algorithms like binary search to operate significantly faster.
Java provides several sorting algorithms, and one of the most efficient is Merge Sort. Understanding the fundamentals of sorting algorithms, specifically time complexity and space complexity, is crucial to select the right sorting algorithm for your specific needs.
Time Complexity: Measuring Algorithm Speed
Time complexity refers to the computational complexity that describes the amount of computer time taken by an algorithm to run. It’s a measure of the amount of time an algorithm needs to complete its run relative to the input size.
Time complexity is usually expressed using Big O notation, which describes the upper bound of the time complexity in the worst-case scenario. For example, Merge Sort has a time complexity of O(n log n), meaning it can sort n items in the time it takes to sort log n items once.
Space Complexity: Evaluating Memory Usage
Space complexity is a measure of the amount of memory an algorithm needs to run to completion. It’s essential to consider, especially in systems with limited memory. Merge Sort has a space complexity of O(n), which means it requires additional space equal to the original input to operate.
Understanding time complexity and space complexity helps you choose the most efficient algorithm for your specific use case. While Merge Sort is efficient for large datasets due to its O(n log n) time complexity, it might not be the best choice for memory-constrained systems due to its O(n) space complexity. Therefore, it’s crucial to understand these fundamentals and consider them when choosing a sorting algorithm.
Merge Sort in Real-World Applications
While understanding and implementing Merge Sort in Java is a valuable skill, it’s equally important to understand how this algorithm can be applied in larger projects or real-world applications. Merge Sort’s efficiency makes it a popular choice in various fields and use-cases.
Data Processing Applications
Merge Sort is often used in data processing applications where large volumes of data need to be sorted quickly. For instance, databases and information retrieval systems often use Merge Sort due to its efficient handling of large datasets.
External Sorting
Merge Sort is particularly useful in external sorting, where the data being sorted do not fit into the main memory of a computing device and reside in slower external memory, typically a hard drive. Merge Sort is efficient in this scenario because it minimizes the costly reads and writes to external memory.
Multi-threaded Systems
Merge Sort can be easily adapted for multi-threaded systems, allowing for parallel processing of the data. This makes Merge Sort an excellent choice for systems with multiple processors or cores, where dividing the workload can significantly speed up the sorting process.
Further Resources for Mastering Merge Sort in Java
To dive deeper into Merge Sort and its applications in Java, consider exploring the following resources:
- IOFlood’s Java Stream Article – Explore intermediate and terminal operations in Java streams and their applications.
Exploring Binary Search in Java – Master Java binary search implementation for improved performance in data retrieval.
Java Bubble Sort Algorithm – Learn Java bubble sort techniques for simple and intuitive sorting of small datasets.
GeeksforGeeks’ Merge Sort Guide covers its implementation in various programming languages, including Java.
Algorithms, Part I by Coursera covers fundamentals of algorithms, including sorting algorithms like Merge Sort.
Baeldung’s Guide to the MergeSort Algorithm in Java provides a detailed explanation of Merge Sort and its implementation in Java.
Remember, mastering a concept like Merge Sort takes practice. Don’t be afraid to experiment, make mistakes, and learn from them. Happy coding!
Wrapping Up: Mastering Merge Sort in Java
In this comprehensive guide, we’ve explored the process of implementing the Merge Sort algorithm in Java, an efficient method for sorting data in ascending or descending order.
We began with the basics, learning how to implement Merge Sort at a beginner level, before delving into the complexities of the algorithm. We learned about the time complexity and space complexity of Merge Sort and provided a more complex example for intermediate users.
We then discussed alternative sorting algorithms, such as Quick Sort and Bubble Sort, comparing their efficiencies with Merge Sort. We also tackled common issues you might encounter when implementing Merge Sort and provided solutions to these problems.
Here’s a quick comparison of the sorting algorithms we’ve discussed:
Sorting Algorithm | Time Complexity | Space Complexity |
---|---|---|
Merge Sort | O(n log n) | O(n) |
Quick Sort | O(n log n) to O(n^2) | O(log n) |
Bubble Sort | O(n^2) | O(1) |
Whether you’re just starting out with Merge Sort or you’re looking to deepen your understanding of sorting algorithms in Java, we hope this guide has been a valuable resource.
The ability to sort data efficiently is a critical skill in programming, and Merge Sort is a powerful tool in your toolkit. Now, you’re well-equipped to implement Merge Sort in Java and understand its complexities and alternatives. Happy coding!