Convert String to Date in Python: Object Handling Tutorial
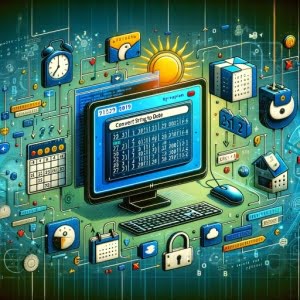
Ever found yourself wrestling with converting strings into dates in Python? You’re not alone. Many developers find this task a bit challenging, but there are various Python tools capable of transforming your strings into dates with ease.
This guide will walk you through the process of converting strings to dates in Python, from the basics to more advanced techniques. We’ll cover everything from using Python’s built-in datetime
module, dealing with different date formats, to troubleshooting common issues and even exploring alternative approaches.
So, let’s dive in and start mastering string to date conversion in Python!
TL;DR: How Do I Convert a String to a Date in Python?
To convert a string to a date in Python, you can use Python’s
datetime
module. Specifically, thestrptime()
function is used to parse a string representing a time according to a format with the syntax,datetime.strptime(date_string, 'format')
.
Here’s a simple example:
from datetime import datetime
date_string = '2022-01-01'
date = datetime.strptime(date_string, '%Y-%m-%d')
print(date)
# Output:
# 2022-01-01 00:00:00
In this example, we import the datetime
module and use the strptime()
function to convert a string to a date. The string ‘2022-01-01’ is parsed according to the format ‘%Y-%m-%d’, resulting in the output ‘2022-01-01 00:00:00’.
But Python’s string to date conversion capabilities go far beyond this. Continue reading for more detailed examples and advanced techniques.
Table of Contents
- The Basics: Using datetime.strptime() for String to Date Conversion
- Handling Various Date Formats
- Exploring Alternative Methods for String to Date Conversion
- Troubleshooting Common Issues in String to Date Conversion
- Understanding Python’s String and Date Data Types
- The Impact of String to Date Conversion in Real-World Applications
- Wrapping Up: Mastering String to Date Conversion in Python
The Basics: Using datetime.strptime()
for String to Date Conversion
Python’s built-in datetime
module provides a function called strptime()
for converting strings to date. This function is very powerful and flexible, allowing you to specify the exact format of your date string.
Here’s how it works:
from datetime import datetime
date_string = '2022-01-01'
date = datetime.strptime(date_string, '%Y-%m-%d')
print(date)
# Output:
# 2022-01-01 00:00:00
In this example, the strptime()
function is used to parse a string representing a time according to a format. The string ‘2022-01-01’ is parsed according to the format ‘%Y-%m-%d’, which means a four-digit year, followed by a two-digit month and a two-digit day.
This method is great for its simplicity and precision. However, it does require you to know the exact format of the date string beforehand, which might not always be the case.
Pitfalls of datetime.strptime()
While datetime.strptime()
is powerful, it’s not without its pitfalls. One common issue is the ValueError
that occurs when the date string does not match the provided format. For example:
from datetime import datetime
date_string = '01-01-2022'
date = datetime.strptime(date_string, '%Y-%m-%d')
# Output:
# ValueError: time data '01-01-2022' does not match format '%Y-%m-%d'
In this example, the date string ’01-01-2022′ does not match the format ‘%Y-%m-%d’, resulting in a ValueError
. This is something to be aware of when using datetime.strptime()
.
Handling Various Date Formats
In the real world, date strings can come in various formats, not just ‘YYYY-MM-DD’. You might encounter date strings like ‘DD/MM/YYYY’, ‘MM-DD-YYYY’, or even ‘Month DD, YYYY’. The good news is, Python’s datetime.strptime()
function is versatile enough to handle these different formats.
Converting ‘DD/MM/YYYY’ Format
Here’s an example of how you can convert a date string in the ‘DD/MM/YYYY’ format:
from datetime import datetime
date_string = '01/01/2022'
date = datetime.strptime(date_string, '%d/%m/%Y')
print(date)
# Output:
# 2022-01-01 00:00:00
In this example, we use the format ‘%d/%m/%Y’ to match the ‘DD/MM/YYYY’ format of our date string. The output is the same as before, but this time we’ve successfully parsed a date string in a different format.
Converting ‘MM-DD-YYYY’ Format
Let’s try another format – ‘MM-DD-YYYY’:
from datetime import datetime
date_string = '01-01-2022'
date = datetime.strptime(date_string, '%m-%d-%Y')
print(date)
# Output:
# 2022-01-01 00:00:00
This time, we use the format ‘%m-%d-%Y’ to match our date string. Again, the output is the same, showing the versatility of the datetime.strptime()
function.
Best Practices
When dealing with different date formats, it’s important to know the format of your date string beforehand. This way, you can provide the correct format string to the strptime()
function. If the format is unknown or can vary, you might need to use a more flexible approach, such as using a date parsing library that can handle a wide range of date formats automatically. We’ll cover this in the next section.
Exploring Alternative Methods for String to Date Conversion
While the datetime.strptime()
function is a powerful tool for converting strings to dates, Python provides other methods that can offer more flexibility and convenience, especially when dealing with various date formats.
The Power of dateutil.parser.parse()
One such method is the dateutil.parser.parse()
function, which is part of the dateutil
library. This function can parse most known formats of dates into datetime objects.
Here’s an example:
from dateutil.parser import parse
date_string = '01/01/2022'
date = parse(date_string)
print(date)
# Output:
# 2022-01-01 00:00:00
In this example, the parse()
function automatically detects the format of the date string and converts it to a datetime object. This can be extremely useful when you’re dealing with date strings of unknown or varying formats.
Advantages and Disadvantages
The main advantage of dateutil.parser.parse()
is its flexibility. It can handle a wide range of date formats without requiring you to specify the format. This makes it a great choice for tasks where the date format may vary or is unknown.
However, this flexibility can also be a disadvantage. If the date string format is not recognized by parse()
, it can lead to incorrect results or errors. Therefore, it’s best to use this function when you’re sure that the date format is supported, or when you’re dealing with multiple date formats and it’s not practical to specify the format for each one.
Comparison between datetime.strptime()
and dateutil.parser.parse()
Method | Flexibility | Precision | Error Handling |
---|---|---|---|
datetime.strptime() | Low (requires exact format) | High (converts based on provided format) | Raises ValueError if format does not match |
dateutil.parser.parse() | High (can handle most known date formats) | Varies (depends on the ability to recognize the date format) | May produce incorrect results if format is not recognized |
Both methods have their own strengths and weaknesses, and the best one to use depends on your specific needs. If you know the exact format of your date strings, datetime.strptime()
is the more precise tool. If you’re dealing with various formats, dateutil.parser.parse()
offers more flexibility.
Troubleshooting Common Issues in String to Date Conversion
Converting strings to dates in Python can sometimes throw up challenges. Let’s discuss some common issues you may encounter during the conversion process, and how to handle them.
Handling ValueError
One of the most common issues you might encounter is the ValueError
. This error typically occurs when the date string does not match the provided format in datetime.strptime()
. Here’s an example:
from datetime import datetime
date_string = '01-01-2022'
date = datetime.strptime(date_string, '%Y-%m-%d')
# Output:
# ValueError: time data '01-01-2022' does not match format '%Y-%m-%d'
In this example, the date string ’01-01-2022′ does not match the format ‘%Y-%m-%d’, resulting in a ValueError
. To avoid this error, ensure that the date string matches the format string you provided.
Dealing with Different Date Formats
Another common challenge is dealing with different date formats. As we’ve discussed earlier, date strings can come in various formats. If you’re unsure of the format, or if it can vary, consider using a more flexible approach like dateutil.parser.parse()
.
Tips for Successful String to Date Conversion
Here are some tips to help you avoid issues when converting strings to dates in Python:
- Always validate your date strings before trying to convert them.
- If you know the exact format of your date strings, use
datetime.strptime()
for a more precise conversion. - If you’re dealing with various date formats, consider using
dateutil.parser.parse()
for its flexibility. - Always handle exceptions to avoid unexpected crashes in your program.
Understanding Python’s String and Date Data Types
Before diving deeper into the process of converting strings to dates in Python, it’s important to understand the fundamental concepts behind Python’s string and date data types.
Python Strings
In Python, strings are sequences of characters enclosed in either single quotes (‘ ‘), double quotes (” “), or triple quotes (”’ ”’ or “”” “””). They are immutable, meaning that once they are created, they cannot be changed. Here’s an example of a Python string:
my_string = 'Hello, World!'
print(my_string)
# Output:
# 'Hello, World!'
Python Dates
Dates in Python are not a separate data type, but they can be represented with a combination of integers and strings using the datetime
module. The datetime
module supplies classes for manipulating dates and times. Here’s an example of a Python date:
from datetime import date
today = date.today()
print(today)
# Output:
# 2022-01-01 (This will output the current date)
In this example, the date.today()
function returns the current local date.
Date Formats in Python
When working with dates in Python, it’s essential to understand date formats. The format of a date is a string representation of how the date is displayed. It is defined by a sequence of character strings, where each character string represents a part of the date, like the day, month, or year.
For example, the format ‘%Y-%m-%d’ represents a four-digit year, followed by a two-digit month and a two-digit day, separated by dashes. So ‘2022-01-01’ is January 1, 2022 in this format.
Understanding these fundamental concepts is key to mastering the conversion of strings to dates in Python.
The Impact of String to Date Conversion in Real-World Applications
The ability to convert strings to dates in Python is not just a theoretical exercise. It has practical applications in various fields, especially in data analysis and web scraping.
String to Date Conversion in Data Analysis
In data analysis, datasets often contain dates in string format. Converting these strings to dates is crucial for time series analysis, where the sequence of data points is indexed in time order. This allows for more meaningful analysis and visualization of the data.
String to Date Conversion in Web Scraping
In web scraping, the data extracted from websites is often in string format. If the data includes dates, these will need to be converted into a date format that Python can understand and manipulate. This is where the skills of converting strings to dates come in handy.
Exploring Related Concepts
Once you’ve mastered the basics of string to date conversion, there are many related concepts to explore. For example, you might want to learn how to handle timezones in Python, or how to parse dates in different formats. These skills will further enhance your ability to manipulate and analyze date data in Python.
Further Resources for Mastering String to Date Conversion
For those who want to delve deeper into the topic, here are some further resources that provide more in-depth information:
- Official Documentation on the datetime module: Python’s official documentation provides an in-depth reference guide to the datetime module.
Python Date and Time Guide: Real Python offers a comprehensive guide on handling date and time in Python.
Python datetime Module: This article on GeeksforGeeks provides a detailed explanation of the datetime module in Python.
Wrapping Up: Mastering String to Date Conversion in Python
In this comprehensive guide, we’ve delved into the process of converting strings to dates in Python, a crucial skill in data manipulation and analysis.
We began with the basics, using Python’s built-in datetime.strptime()
function to convert strings into date objects. We then tackled more complex scenarios, dealing with different date formats and the common issues that arise. We also explored alternative methods, such as the dateutil.parser.parse()
function, showing the versatility of Python in handling various date formats.
Along the way, we faced common challenges, such as ValueError
and dealing with unknown date formats. We provided solutions and workarounds for these issues, equipping you with the knowledge to handle these challenges in your projects.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Precision | Error Handling |
---|---|---|---|
datetime.strptime() | Low (requires exact format) | High (converts based on provided format) | Raises ValueError if format does not match |
dateutil.parser.parse() | High (can handle most known date formats) | Varies (depends on the ability to recognize the date format) | May produce incorrect results if format is not recognized |
Whether you’re a beginner just starting out with Python or an experienced developer looking to enhance your data manipulation skills, we hope this guide has deepened your understanding of string to date conversion in Python.
The ability to convert strings to dates is a powerful tool in data analysis and web scraping, enabling you to manipulate and analyze your data more effectively. Now, you’re well equipped to master this skill. Happy coding!