Java HashSet: Your Key to Unique Collections
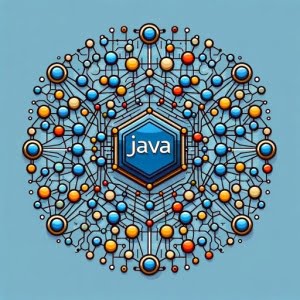
Are you finding it challenging to work with HashSet in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Think of Java’s HashSet as a unique collection of elements – a place where duplicates are not allowed. It’s a powerful tool that can help you manage collections of data in a way that ensures uniqueness.
This guide will walk you through everything you need to know about using HashSet in Java, from basic usage to advanced techniques. We’ll cover everything from creating a HashSet, adding elements to it, iterating over its elements, to more complex operations such as removing elements, checking if an element exists, and clearing the set.
So, let’s dive in and start mastering HashSet in Java!
TL;DR: How Do I Use HashSet in Java?
You can instantiate a new HashSet with:
HashSet<String> set = new HashSet<>()
You can use a Hashset in Java to store unique elements and ensure uniqueness in a collection, making it an essential part of Java’s data structures.
Here’s a simple example:
HashSet<String> set = new HashSet<>();
set.add("Hello");
set.add("World");
System.out.println(set);
# Output:
# [Hello, World]
In this example, we create a HashSet named ‘set’ and add two elements to it: ‘Hello’ and ‘World’. When we print the set, it displays both elements, maintaining their uniqueness. Note that HashSet does not maintain any order of its elements.
This is just a basic way to use HashSet in Java, but there’s much more to learn about managing unique collections efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Java HashSet
HashSet is a part of Java’s collections framework and implements the Set interface. It’s primarily used for storing unique elements, and it doesn’t maintain any order of its elements.
Creating a HashSet
Creating a HashSet in Java is straightforward. Here’s how you do it:
HashSet<String> set = new HashSet<>();
In this line of code, we’ve declared a HashSet named ‘set’ that will store elements of type String.
Adding Elements to HashSet
Once you’ve created a HashSet, you can start adding elements to it. Here’s how:
set.add("Hello");
set.add("World");
In these lines of code, we’ve added two elements: ‘Hello’ and ‘World’ to the HashSet ‘set’.
Iterating Over HashSet
To access all the elements in a HashSet, you can use a for-each loop. Here’s an example:
for (String element : set) {
System.out.println(element);
}
# Output:
# Hello
# World
In this example, we’re using a for-each loop to iterate over the HashSet ‘set’. For each iteration, the loop variable ‘element’ takes on the value of the current HashSet element, and we print it out.
Remember, HashSet does not maintain any order of its elements, so the order in which you added elements may not be the order in which they’re printed.
These are the basics of using HashSet in Java. Understanding these will help you manage unique collections of data effectively.
Advanced Operations with Java HashSet
As you become more comfortable with the basics of HashSet, you may find yourself needing to perform more complex operations. Let’s delve into some of these operations.
Removing Elements from HashSet
Removing an element from a HashSet is as simple as adding one. Here’s how you do it:
set.remove("Hello");
System.out.println(set);
# Output:
# [World]
In this example, we’ve removed the element ‘Hello’ from the HashSet ‘set’. When we print the set, it only displays ‘World’.
Checking if an Element Exists
You can check if a particular element exists in a HashSet using the contains()
method. Here’s an example:
boolean exists = set.contains("World");
System.out.println(exists);
# Output:
# true
In this code, we’re checking if ‘World’ exists in the HashSet ‘set’. The contains()
method returns true if the element exists and false otherwise.
Clearing the HashSet
If you want to remove all elements from a HashSet, you can use the clear()
method. Here’s how:
set.clear();
System.out.println(set);
# Output:
# []
In this example, we’ve cleared all elements from the HashSet ‘set’. When we print the set, it displays an empty set.
These operations allow you to manipulate your HashSet in Java effectively, giving you more control over your data.
Exploring TreeSet and LinkedHashSet in Java
While HashSet is a powerful tool for managing unique collections, Java also offers other types of sets that could be more suitable depending on your needs. Two such alternatives are TreeSet and LinkedHashSet.
Understanding TreeSet
TreeSet is another implementation of the Set interface. Unlike HashSet, TreeSet guarantees that the elements will be sorted in ascending order. Let’s see it in action:
TreeSet<String> treeSet = new TreeSet<>();
treeSet.add("Hello");
treeSet.add("World");
System.out.println(treeSet);
# Output:
# [Hello, World]
In this example, we’ve created a TreeSet and added two elements to it. When we print the TreeSet, it displays the elements in ascending order.
Diving into LinkedHashSet
LinkedHashSet is yet another implementation of the Set interface. It maintains the insertion order of elements, unlike HashSet. Here’s an example:
LinkedHashSet<String> linkedSet = new LinkedHashSet<>();
linkedSet.add("Hello");
linkedSet.add("World");
System.out.println(linkedSet);
# Output:
# [Hello, World]
In this code, we’ve created a LinkedHashSet and added two elements. When we print the LinkedHashSet, it displays the elements in the order they were inserted.
When to Use Each
Choosing between HashSet, TreeSet, and LinkedHashSet depends on your specific needs:
- Use HashSet when you don’t care about the order of elements.
- Use TreeSet when you want elements to be sorted.
- Use LinkedHashSet when you want to maintain the insertion order of elements.
By understanding these alternatives, you can choose the right tool for your specific needs and master the art of managing unique collections in Java.
Common Challenges with Java HashSet
While HashSet is a powerful tool, it’s not without its quirks. Here are some common issues you might encounter and how to resolve them.
Handling Null Values
One common issue with HashSet is handling null values. HashSet allows one null value, but adding more can lead to confusion. Let’s see what happens:
set.add(null);
set.add(null);
System.out.println(set);
# Output:
# [null]
In this example, we’ve added two null values to the HashSet ‘set’. However, when we print the set, it only displays one null value. This is because HashSet treats all null values as the same, so it only stores one.
Dealing with Custom Objects
Another common issue arises when you’re using custom objects in a HashSet. If you don’t override the equals()
and hashCode()
methods in your custom class, HashSet may not behave as expected. Here’s an example:
class CustomObject {
String name;
CustomObject(String name) {
this.name = name;
}
}
HashSet<CustomObject> set = new HashSet<>();
set.add(new CustomObject("Hello"));
set.add(new CustomObject("Hello"));
System.out.println(set.size());
# Output:
# 2
In this code, we’ve created a custom class ‘CustomObject’ with a single field ‘name’. We then create a HashSet of ‘CustomObject’ and add two objects with the same name. When we print the size of the set, it displays ‘2’, even though we added two objects with the same name. This is because we didn’t override the equals()
and hashCode()
methods in ‘CustomObject’.
To resolve this issue, you should override these methods in your custom class to define what makes two objects equal.
By understanding these common issues, you can avoid pitfalls and use HashSet more effectively in your Java applications.
Understanding Set Interface and HashSet in Java
Before we delve into the specifics of HashSet, it’s crucial to understand the fundamentals that underpin its functionality. This includes the Set interface and the concept of hashing.
The Role of Set Interface
In Java, the Set interface is a member of the Java Collections Framework. It is used to store a collection of elements, but unlike the List interface, it does not allow duplicate elements.
HashSet is one of the classes that implements the Set interface. It uses a mechanism called hashing to store elements, which allows it to perform operations like add, remove, and search in constant time, making it highly efficient.
The Power of Hashing
Hashing is a process of converting an object into an integer, which can then be used as an index to store this object in the collection. When an element is inserted into the HashSet, the hashCode()
method of the object is called, and the returned hashcode is used to find a bucket location where this object is stored.
This process is crucial for HashSet’s performance. It allows HashSet to find, add, and remove elements in constant time, making it a powerful tool for managing collections.
HashSet<String> set = new HashSet<>();
set.add("Hello");
System.out.println("Hello".hashCode());
# Output:
# 69609650
In this example, we’ve added an element ‘Hello’ to the HashSet and then printed its hashcode. The hashcode is a unique integer that HashSet uses to store and retrieve the element efficiently.
Understanding these fundamentals is key to leveraging the power of HashSet in Java and creating efficient applications.
Harnessing HashSet in Larger Applications
As you delve deeper into Java, you’ll find that HashSet is not just a tool for managing unique collections—it’s also a powerful tool for larger applications, particularly in data filtering and duplicate removal.
Leveraging HashSet for Data Filtering
HashSet’s ability to store unique elements makes it an excellent tool for data filtering. For instance, if you’re working with a large dataset and you want to filter out duplicate data points, you can add all the data points to a HashSet. The resulting set will only contain unique data points, effectively filtering out duplicates.
Using HashSet for Duplicate Removal
Similarly, HashSet is a powerful tool for removing duplicates from a collection. Suppose you have a list with duplicate elements and you want to remove these duplicates. You can add all the elements to a HashSet, which will only keep the unique elements. Then, you can convert the HashSet back to a list, resulting in a list without duplicates.
List<String> listWithDuplicates = Arrays.asList("Hello", "Hello", "World");
HashSet<String> set = new HashSet<>(listWithDuplicates);
List<String> listWithoutDuplicates = new ArrayList<>(set);
System.out.println(listWithoutDuplicates);
# Output:
# [Hello, World]
In this code, we’ve created a list with duplicate elements, converted it to a HashSet to remove duplicates, and then converted it back to a list. The resulting list only contains unique elements.
Further Resources for Mastering Java Collections
- Understand HashMap resizing and rehashing strategies for optimal performance.
There are plenty of resources available online to continue your Java Hashing research. For example, our complete guide to Java Hashmaps can be found by Clicking Here!
And to deepen your understanding of HashSet and similar Java collections, consider exploring these other resources:
- Understanding Rehashing in Java – Explore how rehashing ensures efficient performance in arraya.
How to Initialize HashMap in Java – Master initializing HashMaps with initial capacity and optimal load factor settings.
Oracle’s Java Collections Tutorial – The official Oracle tutorial on using collections in Java.
Java Collections Framework Overview – Thorough overview of Java collections framework from GeeksforGeeks.
Java HashSet Class Tutorial – Master the Java HashSet class with this clear tutorial from W3Schools.
Remember, mastering any programming concept requires practice. So, keep exploring, keep experimenting, and keep coding!
Wrapping Up: Mastering HashSet in Java
In this comprehensive guide, we’ve delved deep into the world of Java’s HashSet, a powerful tool for managing unique collections of data. We’ve demystified the concepts and operations associated with HashSet, providing you with the knowledge you need to leverage its capabilities effectively.
We started with the basics, learning how to create a HashSet, add elements to it, and iterate over its elements. We then moved on to more advanced operations, such as removing elements, checking if an element exists, and clearing the set. Along the way, we tackled common challenges, such as handling null values and dealing with custom objects, offering solutions to help you navigate these issues.
We also explored alternative approaches to managing unique collections in Java, comparing HashSet with TreeSet and LinkedHashSet. Here’s a quick comparison of these classes:
Class | Ordering | Speed | Use Case |
---|---|---|---|
HashSet | No | Fast | When you don’t care about the order of elements |
TreeSet | Yes (Ascending) | Moderate | When you want elements to be sorted |
LinkedHashSet | Yes (Insertion Order) | Moderate | When you want to maintain the insertion order of elements |
Whether you’re a beginner just starting out with HashSet or a seasoned developer looking to refine your skills, we hope this guide has provided you with valuable insights and practical knowledge.
HashSet is a powerful tool in the Java developer’s toolkit, and mastering it is a significant step towards becoming a more effective and efficient programmer. Happy coding!