Initialize an ArrayList in Java: Easy and Advanced Methods
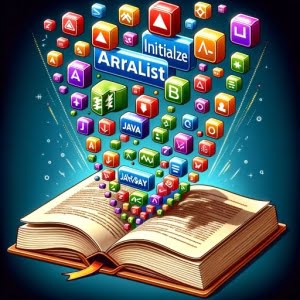
Are you finding it difficult to initialize ArrayLists in Java? You’re not alone. Many developers face this hurdle, especially when they’re new to the language. Think of an ArrayList like a dynamic array, which can grow and shrink in size as needed, providing a flexible and powerful tool for handling data.
This guide will walk you through the process of initializing an ArrayList in Java, from the basic to more advanced methods. We’ll cover everything from the simplest ways to create an ArrayList, to more complex techniques, and even discuss common issues and their solutions.
So, let’s dive in and start mastering ArrayList initialization in Java!
TL;DR: How Do I Initialize an ArrayList in Java?
The simplest way to initialize an ArrayList is with the syntax:
ArrayList<String> list = new ArrayList<String>();
which creates an empty ArrayList named ‘list’ that can hold String objects. It’s the most straightforward way to initialize an ArrayList in Java.
Here’s a simple example:
ArrayList<String> names = new ArrayList<String>();
names.add("John");
names.add("Alice");
System.out.println(names);
# Output:
# [John, Alice]
In this example, we first create an empty ArrayList named ‘names’. Then, we add two names, ‘John’ and ‘Alice’, to the list using the add
method. Finally, we print out the list, which shows the names we added.
But Java’s ArrayList initialization capabilities go far beyond this. Continue reading for more detailed examples and advanced initialization techniques.
Table of Contents
- Initializing an ArrayList: The Basics
- Initializing ArrayList with Predefined Elements
- Exploring Alternative ArrayList Initialization Techniques
- Troubleshooting ArrayList Initialization
- Understanding ArrayList in Java
- Comparing ArrayList with LinkedList and Array
- The Importance of ArrayList in Java Programming
- Wrapping Up: ArrayList Initialization in Java
Initializing an ArrayList: The Basics
In Java, the simplest way to initialize an ArrayList involves using the ‘new’ keyword and the ‘ArrayList’ constructor. This method is perfect for beginners and is often used in a wide range of Java programs.
Here’s how you can initialize an ArrayList:
ArrayList<String> names = new ArrayList<String>();
In this line of code, ArrayList
declares a new ArrayList that can hold objects of type String. names
is the name of the ArrayList, and new ArrayList()
creates a new, empty ArrayList.
You can add elements to an ArrayList using the add
method. For example:
names.add("Felix");
names.add("Josie");
System.out.println(names);
// Output:
// [Felix, Josie]
In this example, we added ‘Felix’ and ‘Josie’ to the ‘names’ ArrayList, then printed the contents of the ArrayList.
Advantages and Potential Pitfalls
This method of initializing an ArrayList is straightforward and easy to understand, making it great for beginners. However, it does have a potential pitfall: it creates an empty ArrayList. If you need an ArrayList with predefined elements, you’ll need to add them manually using the add
method or use a different method of initialization, which we’ll cover in the next section.
Initializing ArrayList with Predefined Elements
Beyond the basics, Java offers methods to initialize an ArrayList with predefined elements. This can be particularly useful when you know the elements at the time of ArrayList creation.
Using Arrays.asList()
One such method is Arrays.asList()
. This method allows you to create an ArrayList and fill it with elements in a single line of code.
Here’s an example:
ArrayList<String> names = new ArrayList<String>(Arrays.asList("John", "Alice"));
System.out.println(names);
# Output:
# [John, Alice]
In this example, Arrays.asList("John", "Alice")
creates a list with two elements, ‘John’ and ‘Alice’. This list is then passed to the ArrayList constructor to create a new ArrayList filled with these elements.
Using Collections.addAll()
Another method to initialize an ArrayList with predefined elements is Collections.addAll()
. This method adds all the specified elements to the specified collection.
Here’s how you can use it:
ArrayList<String> names = new ArrayList<String>();
Collections.addAll(names, "John", "Alice");
System.out.println(names);
# Output:
# [John, Alice]
In this example, we first create an empty ArrayList named ‘names’. We then use Collections.addAll()
to add ‘John’ and ‘Alice’ to the ‘names’ ArrayList.
Advantages and Disadvantages
Both Arrays.asList()
and Collections.addAll()
provide a quick and convenient way to initialize an ArrayList with predefined elements. However, they have one major drawback: the resulting ArrayList is fixed-size. This means you cannot add or remove elements from it. If you need a dynamic ArrayList, you’ll need to use a different method of initialization, which we’ll cover in the next section.
Exploring Alternative ArrayList Initialization Techniques
Java offers several other advanced techniques for initializing ArrayLists. Two such methods are the ‘Stream’ API and the ‘Double Brace Initialization’. These methods can offer more flexibility or readability in certain situations.
Stream API
The Stream API in Java can be used to initialize an ArrayList. This is particularly useful when you’re dealing with a sequence of elements and want to perform computations on them.
Here’s an example of initializing an ArrayList using the Stream API:
ArrayList<String> names = Stream.of("John", "Alice")
.collect(Collectors.toCollection(ArrayList::new));
System.out.println(names);
# Output:
# [John, Alice]
In this example, Stream.of("John", "Alice")
creates a new stream containing ‘John’ and ‘Alice’. The collect
method is then used to accumulate the elements into a new ArrayList.
Double Brace Initialization
Double Brace Initialization is a less common, but still useful, method of initializing an ArrayList. It involves creating an anonymous inner class with an instance initializer (also known as a ‘double brace’).
Here’s how you can use it:
ArrayList<String> names = new ArrayList<String>() {{
add("John");
add("Alice");
}};
System.out.println(names);
# Output:
# [John, Alice]
In this example, we create a new anonymous inner class that extends ArrayList. The instance initializer (the block of code inside the double braces) adds ‘John’ and ‘Alice’ to the ArrayList.
Advantages and Disadvantages
The Stream API and Double Brace Initialization offer more advanced ways to initialize an ArrayList. They can make your code more concise and readable, especially in complex scenarios.
However, these methods also have their drawbacks. The Stream API can be overkill for simple tasks, and Double Brace Initialization can lead to memory leaks because it creates anonymous inner classes. Therefore, they should be used judiciously and in appropriate situations.
Troubleshooting ArrayList Initialization
While initializing an ArrayList in Java is generally straightforward, you might encounter some issues. Two of the most common are ‘NullPointerException’ and ‘UnsupportedOperationException’. Let’s discuss these problems and provide some solutions.
Dealing with NullPointerException
A ‘NullPointerException’ can occur when you try to use an ArrayList that hasn’t been initialized. Here’s an example:
ArrayList<String> names;
names.add("John");
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we declared an ArrayList but didn’t initialize it. When we tried to add an element to it, Java threw a ‘NullPointerException’.
The solution is to ensure your ArrayList is properly initialized before you try to use it:
ArrayList<String> names = new ArrayList<String>();
names.add("John");
System.out.println(names);
# Output:
# [John]
Handling UnsupportedOperationException
An ‘UnsupportedOperationException’ can occur when you try to modify an ArrayList that doesn’t support modification. This often happens when you use methods like Arrays.asList()
to initialize your ArrayList, as the resulting list is fixed-size.
Here’s an example:
ArrayList<String> names = new ArrayList<String>(Arrays.asList("John", "Alice"));
names.add("Bob");
# Output:
# Exception in thread "main" java.lang.UnsupportedOperationException
In this example, we initialized an ArrayList with Arrays.asList()
, which returns a fixed-size list. When we tried to add another element to the list, Java threw an ‘UnsupportedOperationException’.
The solution is to create a modifiable ArrayList. You can do this by passing the fixed-size list to the ArrayList constructor:
ArrayList<String> names = new ArrayList<String>(new ArrayList<String>(Arrays.asList("John", "Alice")));
names.add("Bob");
System.out.println(names);
# Output:
# [John, Alice, Bob]
In this example, we first created a new ArrayList from the fixed-size list returned by Arrays.asList()
. This new ArrayList is modifiable, so we could add ‘Bob’ without any issues.
Remember, understanding these common issues and their solutions can help you avoid pitfalls when initializing ArrayLists in Java.
Understanding ArrayList in Java
The ArrayList class in Java is a part of the Java Collections Framework. It is a resizable array, which means it can grow or shrink dynamically. This feature makes ArrayList a popular choice for handling data in Java.
An ArrayList has several key characteristics and methods that make it unique:
- Dynamic Size: Unlike arrays, an ArrayList can automatically adjust its capacity to accommodate additional elements. This makes ArrayList more flexible than a traditional array.
Element Manipulation: ArrayList provides methods to add, remove, and modify elements. For example, you can use the
add
method to add elements, theremove
method to remove elements, and theset
method to modify an existing element.Order Preservation: ArrayList maintains the insertion order of elements. When you iterate through an ArrayList, the elements are returned in the order they were added.
Here’s an example of using some of these methods:
ArrayList<String> names = new ArrayList<String>();
names.add("John");
names.add("Alice");
names.set(0, "Bob");
names.remove(1);
System.out.println(names);
# Output:
# [Bob]
In this example, we first created an ArrayList named ‘names’. We then added ‘John’ and ‘Alice’, replaced ‘John’ with ‘Bob’, and removed ‘Alice’. The final output is an ArrayList containing just ‘Bob’.
Comparing ArrayList with LinkedList and Array
While ArrayList is a powerful tool, it’s not always the best choice. Other data structures, such as LinkedList and Array, might be more suitable depending on the situation.
- ArrayList vs LinkedList: Both are part of the Java Collections Framework and can store elements dynamically. However, ArrayList provides faster access to elements because it uses an array under the hood. LinkedList, on the other hand, provides faster insertion and deletion of elements at the beginning and end.
ArrayList vs Array: While both can store elements, ArrayList is resizable and provides built-in methods for element manipulation. Arrays are fixed-size and don’t have these built-in methods. However, arrays can be more efficient in terms of memory and access speed, especially for a large number of elements.
Understanding these fundamentals can help you choose the right data structure for your specific needs in Java.
The Importance of ArrayList in Java Programming
ArrayList plays a crucial role in Java programming. It’s a versatile tool that can handle a wide range of tasks, making it a go-to data structure for many developers.
Data Manipulation
ArrayList is often used in data manipulation. It provides methods to add, remove, and modify elements, making it easy to manipulate data. For example, you might use an ArrayList to store and manipulate a list of user names in a social networking application.
File Handling
ArrayList can also be useful in file handling. For instance, you could read lines from a file into an ArrayList, manipulate the data as needed, and then write the data back to a file.
Java Collections Framework and Generics
ArrayList is a part of the Java Collections Framework, which provides a unified architecture for manipulating and controlling groups of objects. Learning about this framework can give you a deeper understanding of ArrayList and other similar data structures.
Generics in Java is another related topic. Generics allow you to define a common method or class that can be used with different types, providing stronger type checks, eliminating the need for typecasting, and enabling programmers to implement generic algorithms. By understanding generics, you can use ArrayList and other data structures more effectively.
Further Resources for Mastering ArrayList
If you’re interested in learning more about ArrayList and related topics, here are some resources that you might find useful:
- IOFlood’s Java ArrayList Guide – Learn about ArrayList’s implementation of the List interface in Java Collections Framework.
Array vs ArrayList Comparison – Understand the differences between arrays and ArrayLists in Java.
Java ArrayList Sorting – Learn about sorting techniques like Collections.sort() and using custom comparators.
Oracle Java Documentation provides information about ArrayList and other Java Collections Framework classes.
Baeldung’s Guide to Java ArrayList covers everything you need to know about ArrayList.
GeeksforGeeks Java ArrayList Framework provides an in-depth look at the ArrayList and other data structures.
Wrapping Up: ArrayList Initialization in Java
In this comprehensive guide, we’ve delved into the process of initializing an ArrayList in Java, exploring everything from basic to advanced methods.
We began with the basics, learning how to initialize an ArrayList using the ‘new’ keyword and the ‘ArrayList’ constructor. We then advanced to intermediate techniques, discussing how to initialize an ArrayList with predefined elements using methods like ‘Arrays.asList()’ and ‘Collections.addAll()’. Finally, we explored expert-level techniques like using the ‘Stream’ API and ‘Double Brace Initialization’.
Along the way, we tackled common issues you might encounter when initializing ArrayLists, such as ‘NullPointerException’ and ‘UnsupportedOperationException’, and provided solutions for each issue. We also compared ArrayList with other data structures in Java, such as LinkedList and Array, giving you a well-rounded understanding of when to use each structure.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Basic Initialization | Simple, easy to understand | Creates an empty ArrayList |
Arrays.asList() | Quick, convenient | Results in fixed-size ArrayList |
Collections.addAll() | Quick, convenient | Results in fixed-size ArrayList |
Stream API | Useful for computations | Can be overkill for simple tasks |
Double Brace Initialization | Concise, readable | Can lead to memory leaks |
Whether you’re new to Java or an experienced developer looking to deepen your understanding of ArrayList, we hope this guide has been a valuable resource. The ability to initialize an ArrayList effectively is a fundamental skill in Java programming, and now you’re well-equipped to do just that. Happy coding!