Java’s ‘this’ Keyword: A Detailed Exploration
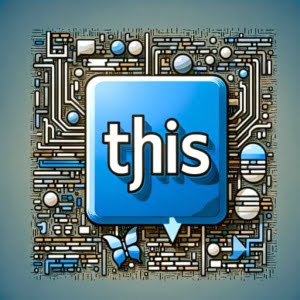
Are you finding it hard to grasp the ‘this’ keyword in Java? You’re not alone. Many developers find themselves puzzled when it comes to using ‘this’ in Java, but we’re here to help.
Think of the ‘this’ keyword in Java as a name tag – it helps identify the current object in your code. It’s a crucial part of Java, and understanding it can significantly improve your programming skills.
In this guide, we’ll walk you through the process of understanding and using ‘this’ in Java, from its basic usage to more advanced applications. We’ll cover everything from how ‘this’ works, its advantages, potential pitfalls, and even alternative approaches.
So, let’s dive in and start mastering ‘this’ in Java!
TL;DR: What is ‘this’ in Java?
In Java, ‘this’ is a keyword that refers to the current object. It’s used to differentiate between class attributes and parameters with the same name:
this.x = x
Here’s a simple example:
class MyClass {
int x;
MyClass(int x) {
this.x = x;
}
}
MyClass obj = new MyClass(10);
System.out.println(obj.x);
// Output:
// 10
In this example, ‘this.x’ refers to the current object’s ‘x’ variable. The ‘this’ keyword is used in the constructor to refer to the current object’s ‘x’ variable, differentiating it from the ‘x’ parameter passed into the constructor. The output of the code is ’10’, which is the value we passed to the ‘x’ variable of the ‘MyClass’ object.
This is a basic usage of ‘this’ in Java, but there’s much more to learn about this powerful keyword. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Unraveling ‘this’: Basic Use in Java
- Delving Deeper: Advanced Use of ‘this’ in Java
- Navigating Java Without ‘this’: Alternative Approaches
- Solving Common Issues with ‘this’ in Java
- Java and ‘this’: A Fundamental Understanding
- The Impact of ‘this’ in Larger Java Projects
- Wrapping Up: Mastering ‘this’ in Java
Unraveling ‘this’: Basic Use in Java
The keyword ‘this’ in Java serves a fundamental purpose: it refers to the current object. In other words, ‘this’ represents the instance of the class where it’s used. It’s commonly used to access or modify the fields of the current object, especially when field names are the same as local variable names.
Let’s consider a simple example:
class Student {
String name;
Student(String name) {
this.name = name;
}
void introduce() {
System.out.println("Hello, my name is " + this.name);
}
}
Student student = new Student("John");
student.introduce();
// Output:
// Hello, my name is John
In this code, the ‘this.name’ in the constructor refers to the current object’s ‘name’ field. It distinguishes it from the ‘name’ parameter passed into the constructor. Moreover, ‘this.name’ in the ‘introduce’ method refers to the ‘name’ field of the current ‘Student’ object.
The usage of ‘this’ is advantageous as it enhances readability and clarity in your code. It allows you to use the same name for instance variables (fields) and parameters, reducing confusion.
However, there are potential pitfalls to be aware of. Misusing ‘this’ can lead to unexpected behavior or bugs in your code. For instance, forgetting to use ‘this’ when the field names and parameter names are the same can lead to the parameter value not being correctly assigned to the field.
Understanding ‘this’ and its basic use is the first step in mastering this powerful keyword in Java.
Delving Deeper: Advanced Use of ‘this’ in Java
As you gain proficiency in Java, you’ll encounter more complex uses of ‘this’. Let’s explore two such scenarios: using ‘this’ to call another constructor in the same class, and using ‘this’ to pass the current object as a parameter to another method.
Using ‘this’ to Call Another Constructor
In Java, ‘this’ can be used to call one constructor from another within the same class. This is known as constructor chaining and helps in reducing duplicated code.
Here’s an example:
class Rectangle {
int width, height;
// Default constructor
Rectangle() {
this(1, 1);
}
// Parameterized constructor
Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
}
Rectangle rect1 = new Rectangle();
Rectangle rect2 = new Rectangle(5, 10);
System.out.println("rect1 dimensions: " + rect1.width + " x " + rect1.height);
System.out.println("rect2 dimensions: " + rect2.width + " x " + rect2.height);
// Output:
// rect1 dimensions: 1 x 1
// rect2 dimensions: 5 x 10
In this code, ‘this’ is used in the default constructor to call the parameterized constructor, setting the width and height to 1. This ensures that a Rectangle object always has a default size, even if no dimensions are specified during creation.
Using ‘this’ to Pass Current Object as a Parameter
Another advanced use of ‘this’ is to pass the current object as a parameter to another method. This can be particularly useful when working with event listeners or when you need to provide other objects with a reference to the current object.
Here’s an example:
class Printer {
void print(Student student) {
System.out.println(student.name + " is studying.");
}
}
class Student {
String name;
Printer printer;
Student(String name, Printer printer) {
this.name = name;
this.printer = printer;
}
void study() {
printer.print(this);
}
}
Printer printer = new Printer();
Student student = new Student("John", printer);
student.study();
// Output:
// John is studying.
In this code, the ‘study’ method in the ‘Student’ class uses ‘this’ to pass the current ‘Student’ object to the ‘print’ method of the ‘Printer’ class. This allows the ‘Printer’ class to access the ‘name’ field of the ‘Student’ object and print a message.
Mastering these advanced uses of ‘this’ can help you write more efficient and cleaner Java code.
While ‘this’ is a powerful and convenient keyword in Java, it’s not always necessary. There are alternative approaches to writing code that could eliminate the need for ‘this’. Let’s explore these alternatives, their benefits, drawbacks, and when it might be appropriate to use them.
Using Different Variable Names
One simple alternative to using ‘this’ is to use different names for your parameters and fields. This can eliminate the ambiguity that ‘this’ is often used to resolve.
class Student {
String name;
Student(String studentName) {
name = studentName;
}
void introduce() {
System.out.println("Hello, my name is " + name);
}
}
Student student = new Student("John");
student.introduce();
// Output:
// Hello, my name is John
In this code, we’ve used ‘studentName’ for the constructor parameter and ‘name’ for the field. This makes ‘this’ unnecessary. However, this approach can lead to longer and potentially more confusing variable names.
Using Setter Methods
Another alternative is to use setter methods to set field values. This can provide more control over how and when fields are set.
class Student {
String name;
void setName(String name) {
this.name = name;
}
void introduce() {
System.out.println("Hello, my name is " + name);
}
}
Student student = new Student();
student.setName("John");
student.introduce();
// Output:
// Hello, my name is John
In this code, we’ve used a ‘setName’ method to set the ‘name’ field. This eliminates the need for ‘this’ in the constructor. However, this approach can make your code longer and more complex.
Choosing the right approach depends on your specific situation and personal coding style. While ‘this’ is a powerful tool, understanding its alternatives can give you more flexibility and control over your Java code.
Solving Common Issues with ‘this’ in Java
While ‘this’ is an incredibly useful keyword in Java, it’s not without its potential pitfalls. Misunderstanding or misusing ‘this’ can lead to confusion and bugs in your code. Let’s explore some common issues and how to avoid them.
Confusion Between Instance Variables and Local Variables
One of the most common issues arises when local variables (variables defined within methods) have the same name as instance variables (variables defined within the class but outside any method). This can lead to confusion and unexpected behavior.
Consider the following code:
class Student {
String name;
void setName(String name) {
name = name;
}
void introduce() {
System.out.println("Hello, my name is " + name);
}
}
Student student = new Student();
student.setName("John");
student.introduce();
// Output:
// Hello, my name is null
In this code, the ‘setName’ method is supposed to set the ‘name’ field to the given value. However, because the local variable ‘name’ and the instance variable ‘name’ have the same name, the instance variable is not being set. Instead, the local variable is being set to its own value, which is not what we intended.
The solution here is to use ‘this’ to clearly distinguish between the instance variable and the local variable:
class Student {
String name;
void setName(String name) {
this.name = name;
}
void introduce() {
System.out.println("Hello, my name is " + name);
}
}
Student student = new Student();
student.setName("John");
student.introduce();
// Output:
// Hello, my name is John
By using ‘this.name’, we are clearly referring to the instance variable ‘name’, not the local variable. This resolves the confusion and ensures that the instance variable is correctly set.
Understanding and properly using ‘this’ in Java can help you avoid these common issues and write cleaner, more readable code.
Java and ‘this’: A Fundamental Understanding
To truly grasp the ‘this’ keyword in Java, we need to delve into the fundamentals of Java and Object-Oriented Programming (OOP).
Java is an OOP language, which means it’s built around the concept of objects. An object is a self-contained entity that bundles together variables (data) and methods (behavior). In Java, objects are instances of classes, which serve as blueprints for creating objects.
Instance Variables, Constructors, and Methods
Let’s break down these key concepts:
- Instance Variables: These are variables that belong to an instance of a class (an object). Each instance has its own copy of these variables. They represent the state of an object.
Constructors: These are special methods that are used to initialize new objects. They’re called when an object is created and can set initial values for instance variables.
Methods: These are behaviors that an object can perform. They can access and modify instance variables.
In the context of these concepts, the ‘this’ keyword in Java has a special meaning. It refers to the current object – the object whose method or constructor is being called.
Here’s a simple example to demonstrate these concepts:
class Student {
String name; // Instance variable
Student(String name) { // Constructor
this.name = name;
}
void introduce() { // Method
System.out.println("Hello, my name is " + this.name);
}
}
Student student = new Student("John");
student.introduce();
// Output:
// Hello, my name is John
In this code, ‘name’ is an instance variable, ‘Student’ is a constructor, and ‘introduce’ is a method. The ‘this’ keyword is used to refer to the current ‘Student’ object.
Understanding these fundamentals is key to mastering the use of ‘this’ in Java.
The Impact of ‘this’ in Larger Java Projects
In larger Java projects, the ‘this’ keyword plays a significant role in maintaining clean, readable code. It helps to clearly distinguish between instance variables and parameters, reducing ambiguity and making your code easier to understand for other developers. Moreover, understanding ‘this’ is crucial for using advanced features of Java, such as inheritance, encapsulation, and polymorphism.
The Role of ‘this’ in Inheritance
Inheritance is a fundamental concept in OOP that allows one class to inherit the fields and methods of another. In the context of inheritance, ‘this’ can be used to refer to the current object within the subclass, even when methods are being overridden.
‘this’ and Encapsulation
Encapsulation is another key OOP concept that involves bundling the data (variables) and the methods that operate on the data into a single unit, i.e., a class. ‘this’ can be used to access encapsulated data from within the class’s methods.
Polymorphism and ‘this’
Polymorphism is a feature of OOP that allows one interface to be used for a general class of actions. The specific action is determined by the exact nature of the situation. In the context of polymorphism, ‘this’ can be used to refer to the current object within the method that is being overridden.
Further Resources for Mastering ‘this’ in Java
To further your understanding of ‘this’ in Java and its role in larger projects, consider the following resources:
- Demystifying Java Keywords – Learn about the role of keywords like “null,” “true,” and “false” in Java literals and values.
Exploring Java Synchronized Blocks – Explore best practices and pitfalls of using synchronized in Java for synchronization.
Understanding ‘super’ in Java – Understand how ‘super’ invokes superclass constructors and methods from a subclass.
Oracle’s Java Tutorials – A comprehensive guide to Java, including an in-depth section on ‘this’.
GeeksforGeeks Java Programming Language – A vast collection of Java-related articles, tutorials, and practice problems.
W3Schools Java Tutorial – A beginner-friendly Java tutorial with interactive coding examples.
Wrapping Up: Mastering ‘this’ in Java
In this comprehensive guide, we’ve delved into the intricacies of ‘this’ in Java, a keyword that plays a pivotal role in object-oriented programming.
We started with the basics, understanding how ‘this’ is used to refer to the current object. We then explored more complex uses, such as using ‘this’ to call another constructor in the same class or to pass the current object as a parameter to another method. Along the way, we tackled common issues that you might encounter when using ‘this’, providing you with solutions and workarounds for each problem.
We also looked at alternative approaches to writing code that could eliminate the need for ‘this’, giving you a broader perspective on coding in Java. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Using ‘this’ | Enhances readability, reduces confusion | Misuse can lead to unexpected behavior |
Using Different Variable Names | Eliminates need for ‘this’ | Can lead to long, confusing variable names |
Using Setter Methods | Provides more control over setting fields | Can make code longer and more complex |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of ‘this’, we hope this guide has been a valuable resource.
Mastering ‘this’ in Java can significantly improve your programming skills, helping you write cleaner, more efficient code. Now, you’re well equipped to navigate the world of Java with confidence. Happy coding!