Bash Shell Scripting | Random Number Generation
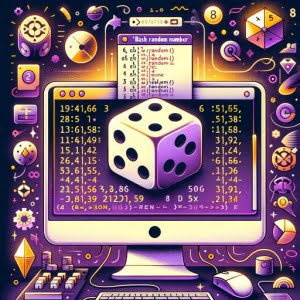
Have you ever found yourself needing to generate random numbers in Bash, but unsure of how to go about it? You’re not alone. Many developers find themselves in a similar situation, but Bash has a built-in solution for this.
Think of Bash’s random number generation as a magician’s trick, pulling numbers out of a hat. It’s a built-in feature that can produce random numbers, serving as a powerful tool for a variety of tasks.
In this guide, we’ll walk you through the process of generating random numbers in Bash, from the basics to more advanced techniques. We’ll cover everything from the simple use of the $RANDOM
variable, to generating random numbers within a specific range, and even alternative approaches.
So, let’s dive in and start mastering random number generation in Bash!
TL;DR: How Do I Generate a Random Number in Bash?
In Bash, you can generate a random number using the built-in variable
$RANDOM
. It will return a random integer between 0 and 32767 which you can attach to a variable or print it to terminal with the syntaxecho $RANDOM
. This variable provides a simple and efficient way to create random numbers in your scripts.
Here’s a quick example:
echo $RANDOM
# Output:
# A random number between 0 and 32767
In this example, we’re using the echo
command to print the value of $RANDOM
. Each time you call $RANDOM
, Bash generates a new random number between 0 and 32767.
But there’s more to generating random numbers in Bash than just
$RANDOM
. Continue reading to learn about generating random numbers within a specific range, alternative methods, and more!
Table of Contents
- Understanding the $RANDOM Variable
- Generating Random Numbers Within a Specific Range
- Exploring Alternative Methods for Random Number Generation
- Troubleshooting Common Issues with Bash Random Numbers
- Understanding Randomness in Bash
- Practical Applications of Bash Random Numbers
- Generating Random Strings in Bash
- Wrapping Up: Mastering Random Number Generation in Bash
Understanding the $RANDOM Variable
The $RANDOM
variable is a built-in Bash variable that generates a random integer each time it’s referenced. It’s the simplest and most straightforward way to create random numbers in Bash.
The $RANDOM Variable in Action
Let’s take a look at an example of how $RANDOM
works:
# Generate a random number
random_number=$RANDOM
# Print the random number
echo "The random number is $random_number"
# Output:
# The random number is [Random number between 0 and 32767]
In this code block, we first assign the value of $RANDOM
to the variable random_number
. Then, we print the value of random_number
using the echo
command. Each time you run this script, a new random number between 0 and 32767 will be printed.
The Range of $RANDOM
The $RANDOM
variable generates an integer between 0 and 32767. This is because $RANDOM
uses a pseudo-random number generator that produces numbers in this range. This range is sufficient for many tasks, but what if you need a random number within a different range? Stay tuned, we’ll cover that in the ‘Advanced Use’ section.
Generating Random Numbers Within a Specific Range
While $RANDOM
provides a random number between 0 and 32767, there might be instances where you need a random number within a specific range. Fortunately, Bash provides a way to achieve this by using the modulo operation (%
) and arithmetic expansion ($(( ))
).
Using Modulo Operation and Arithmetic Expansion
The modulo operation returns the remainder of a division operation. When used with $RANDOM
, it helps limit the range of the generated number. Arithmetic expansion allows the evaluation of an arithmetic expression and its replacement with the result.
Let’s generate a random number between 1 and 100:
# Generate a random number within a specific range
random_number=$((1 + RANDOM % 100))
# Print the random number
echo "The random number is $random_number"
# Output:
# The random number is [Random number between 1 and 100]
In this example, RANDOM % 100
generates a random number between 0 and 99. By adding 1 to the result (1 + RANDOM % 100
), we shift the range to be between 1 and 100. Each time you run this script, a new random number within this range will be printed.
This approach gives you more control over the range of random numbers generated by your Bash scripts, making $RANDOM
a more versatile tool.
Exploring Alternative Methods for Random Number Generation
While $RANDOM
is a handy tool, Bash also supports other methods for generating random numbers, such as utilizing /dev/urandom
or external tools like shuf
. Let’s explore these alternatives and their benefits and drawbacks.
Using /dev/urandom
/dev/urandom
is a special file in Unix-like operating systems that serves as a cryptographically secure random number generator. Here’s how you can use it to generate a random number:
# Generate a random number using /dev/urandom
random_number=$(od -An -N2 -i /dev/urandom)
# Print the random number
echo "The random number is $random_number"
# Output:
# The random number is [Random number]
In this example, od
is a command-line utility for binary file processing. -An
removes the address section, -N2
reads two bytes, and -i
interprets the bytes as a signed decimal.
This method provides a larger range of numbers and a higher level of randomness compared to $RANDOM
. However, it’s more complex and might be overkill for simple tasks.
Using shuf
shuf
is a command-line utility that generates random permutations. You can use it to generate a random number within a specific range:
# Generate a random number using shuf
random_number=$(shuf -i 1-100 -n 1)
# Print the random number
echo "The random number is $random_number"
# Output:
# The random number is [Random number between 1 and 100]
In this example, -i 1-100
specifies the range of numbers, and -n 1
tells shuf
to output one number.
shuf
is easy to use and provides a simple way to generate a random number within a specific range. However, it’s not built into Bash and might not be available on all systems.
Troubleshooting Common Issues with Bash Random Numbers
When working with random numbers in Bash, you might encounter some common issues. Let’s discuss these potential pitfalls and how to navigate them.
Addressing Lack of True Randomness
One common issue with Bash’s $RANDOM
variable is that it doesn’t generate truly random numbers. It uses a pseudo-random number generator, which means the numbers are predictable to some extent. This might not be an issue for simple tasks, but for more complex or security-sensitive applications, you might need a more robust solution.
One potential solution is to use /dev/urandom
or openssl
for generating random numbers, as they provide a higher level of randomness. Here’s an example using openssl
:
# Generate a random number using openssl
random_number=$(openssl rand -base64 6)
# Print the random number
echo "The random number is $random_number"
# Output:
# The random number is [Random number]
In this example, openssl rand -base64 6
generates a random number with a higher degree of randomness than $RANDOM
. However, keep in mind that this method is more complex and might not be necessary for all applications.
Ensuring Availability of External Tools
While tools like shuf
and openssl
can be useful for generating random numbers, they might not be available on all systems. If your script is intended to run on different systems, make sure to check the availability of these tools, or stick with $RANDOM
which is built into Bash.
Handling Large Numbers
If you need to generate a very large random number, $RANDOM
might not suffice as it’s limited to 32767. In such cases, you can use /dev/urandom
or openssl
as shown above, or combine multiple $RANDOM
calls. However, remember that combining $RANDOM
calls doesn’t increase the randomness of the result.
Understanding Randomness in Bash
When we talk about generating random numbers in Bash, it’s important to understand what we mean by ‘random’. In the world of computing, there are two types of randomness: true randomness and pseudo-randomness.
True Randomness vs. Pseudo-randomness
True randomness is unpredictable and lacks any patterns. It’s typically derived from physical phenomena, like atmospheric noise or radioactive decay. However, computers, being deterministic machines, find it challenging to generate truly random numbers.
That’s where pseudo-randomness comes in. Pseudo-random numbers appear random, but they are generated by deterministic processes. They start from a ‘seed’ value, and a mathematical algorithm generates a sequence of numbers that seem random.
# Setting the seed for RANDOM
RANDOM=42
# Generate a random number
random_number=$RANDOM
# Print the random number
echo "The random number is $random_number"
# Output:
# The random number is [A number between 0 and 32767]
In this example, we’re setting the seed for $RANDOM
. If you run this script multiple times, it will always print the same number, demonstrating the deterministic nature of pseudo-random number generators.
How Bash Generates Random Numbers
Bash uses a pseudo-random number generator to generate numbers when you call $RANDOM
. The generator uses a seed value (which changes with each shell session) and a mathematical algorithm to produce a sequence of numbers that appear random.
While these numbers are not truly random and can be predicted if the seed value and the algorithm are known, they are sufficient for many tasks in scripting and programming. However, for tasks that require a high level of unpredictability, like cryptography, other methods may be more suitable.
Practical Applications of Bash Random Numbers
Random numbers in Bash scripts find their use in a variety of practical scenarios. Let’s explore a couple of these applications to give you an idea of their potential.
Random File Naming
Random numbers can be used to generate unique file names in Bash scripts. This can be useful when you need to create temporary files or avoid overwriting existing files.
# Generate a random file name
file_name="file_$RANDOM.txt"
# Create a new file with the random name
touch $file_name
# Output:
# A new file named 'file_[Random number].txt' is created
In this example, we’re appending $RANDOM
to a string to create a unique file name, then using the touch
command to create a new file with this name.
Task Scheduling
Random numbers can also be used to schedule tasks at random intervals. This can be useful for load balancing or avoiding predictable patterns.
# Run a task at a random time
sleep $((RANDOM % 60)) && echo 'Task completed!'
# Output:
# 'Task completed!' is printed after a random number of seconds (up to 60)
In this example, we’re using sleep
to pause the script for a random number of seconds before printing ‘Task completed!’.
Generating Random Strings in Bash
In addition to generating random numbers, Bash can also generate random strings. This can be useful for creating unique identifiers, generating passwords, and more. We won’t dive into it here, but it’s a related topic that’s worth exploring.
Further Resources for Bash Random Number Mastery
- Bash scripting guide by Ryan’s Tutorials
- Advanced Bash-Scripting Guide by The Linux Documentation Project
- Bash Tutorial by Learn Shell
These resources provide a deeper dive into Bash scripting and random number generation. They’ll help you master the art of generating random numbers in Bash and applying them in your scripts.
Wrapping Up: Mastering Random Number Generation in Bash
In this comprehensive guide, we’ve delved into the process of generating random numbers in Bash, exploring from the basics to more advanced techniques.
We started with the simple use of the $RANDOM
variable, demonstrating how it can produce random numbers with ease. We then moved on to more advanced usage, showing how to generate random numbers within a specific range using modulo operation and arithmetic expansion.
We also explored alternative methods for generating random numbers in Bash, such as using /dev/urandom
and external tools like shuf
. Each method has its pros and cons, and the choice depends on your specific needs and the environment in which your script runs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
$RANDOM | Built-in, easy to use | Limited range, not truly random |
/dev/urandom | Larger range, more random | More complex |
shuf | Easy to use, specific range | Not built-in, might not be available on all systems |
We also discussed common issues you might encounter when generating random numbers in Bash, such as the lack of true randomness, and provided potential solutions and considerations.
Whether you’re just starting out with Bash or you’re looking to enhance your scripting skills, we hope this guide has given you a deeper understanding of how to generate random numbers in Bash. With this knowledge, you’re well-equipped to add a touch of randomness to your Bash scripts. Happy scripting!