Python 2D Array with Lists | Guide (With Examples)
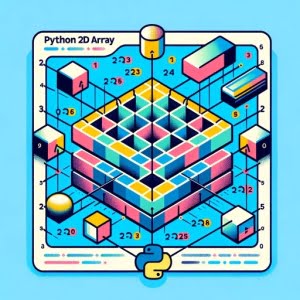
Are you needing to use 2D arrays in Python, but don’t know where to start? Many developers find themselves puzzled when it comes to handling 2D arrays in Python, but we’re here to help.
Think of Python’s 2D arrays as a multi-storey building – allowing us to store data in multiple dimensions, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with 2D arrays in Python, from their creation, manipulation, and usage. We’ll cover everything from the basics of multi-dimensional arrays to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Create a 2D Array in Python?
To create a 2D array in Python, you can use nested lists. EX:
array = [[1, 2], [3, 4], [5, 6]]
. This involves creating a list within a list, where each inner list represents a row in the 2D array.
Here’s a simple example:
array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(array)
# Output:
# [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
In this example, we’ve created a 2D array (or a matrix) with three rows and three columns. Each inner list [1, 2, 3]
, [4, 5, 6]
, and [7, 8, 9]
represents a row in the 2D array. When we print the array, we get the output as a nested list, which is the Pythonic way of representing a 2D array.
This is a basic way to create a 2D array in Python, but there’s much more to learn about manipulating and using 2D arrays in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Python 2D Arrays: Basic Use
In Python, a 2D array is essentially a list of lists. The outer list represents the rows, and each inner list represents a row of elements, similar to how a row works in a matrix. Let’s dive into the basics of creating, accessing, modifying, and iterating over a 2D array in Python.
Creating a 2D Array
First, let’s create a 2D array. As mentioned in the TL;DR section, we can create a 2D array by nesting lists within a list. Here’s an example:
array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(array)
# Output:
# [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
In this example, we’ve created a 2D array with three rows and three columns. Each inner list represents a row in the 2D array.
Accessing Elements
To access elements in a 2D array, you need two indices – one for the row and one for the column. The first index refers to the row number and the second index refers to the column number.
Let’s access the element in the second row, third column (remember, Python indexing starts at 0):
print(array[1][2])
# Output:
# 6
Modifying Elements
Modifying elements in a 2D array is similar to accessing elements. You specify the row and column indices of the element you want to modify. Let’s change the value in the first row, first column to 10:
array[0][0] = 10
print(array)
# Output:
# [[10, 2, 3], [4, 5, 6], [7, 8, 9]]
Iterating Over a 2D Array
To iterate over a 2D array, you can use a nested for loop. The outer loop iterates over the rows, and the inner loop iterates over each row’s elements. Here’s an example:
for row in array:
for elem in row:
print(elem, end=' ')
print()
# Output:
# 10 2 3
# 4 5 6
# 7 8 9
In this example, we printed each element in the 2D array, with each row’s elements separated by a space and each row separated by a new line.
Advanced 2D Array Techniques
Once you’ve grasped the basics of 2D arrays in Python, you can start exploring more complex uses. This section dives into multi-dimensional arrays and nested list comprehensions.
Multi-Dimensional Arrays
Python allows you to create arrays with more than two dimensions. These are called multi-dimensional arrays. They are created and used similarly to 2D arrays but with an extra level of nesting for each additional dimension. Let’s create a 3D array:
array3D = [[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]]
print(array3D)
# Output:
# [[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]]
In this example, we have a 3D array with two 2D arrays, each containing two rows of three elements. To access an element, we need three indices – one for the 2D array, one for the row, and one for the column.
print(array3D[1][0][2])
# Output:
# 9
Nested List Comprehensions
Python’s list comprehensions provide a concise way to create lists. You can also use them to create 2D arrays. Here’s an example of creating a 2D array using a nested list comprehension:
array = [[i*j for j in range(1, 4)] for i in range(1, 4)]
print(array)
# Output:
# [[1, 2, 3], [2, 4, 6], [3, 6, 9]]
In this example, we’ve created a 2D array where each element is the product of its row and column indices (starting from 1). The outer list comprehension (for i in range(1, 4)
) creates the rows, and the inner list comprehension (for j in range(1, 4)
) creates the elements within each row.
Exploring Alternative Approaches with Numpy
Python’s built-in list structure is versatile and powerful, but when it comes to numerical computations and handling large 2D arrays, there’s a more efficient alternative – the numpy library.
Numpy 2D Arrays
Numpy is a Python library used for numerical computations and has a built-in 2D array object that offers more functionalities than the native Python 2D array. Let’s create a 2D array using numpy:
import numpy as np
numpy_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(numpy_array)
# Output:
# [[1 2 3]
# [4 5 6]
# [7 8 9]]
Accessing and modifying elements in a numpy 2D array is similar to Python’s native 2D array. However, numpy provides more advanced features like element-wise operations, mathematical functions, and more.
Advantages and Disadvantages
While numpy 2D arrays offer more functionalities and are more efficient for large-scale computations, they come with their own limitations. Numpy arrays are homogeneous, which means all elements must be of the same type. If you need to store different types of data, Python’s native 2D array (list of lists) would be more suitable.
Recommendations
If you’re working with numerical data, especially large data sets, numpy is a great choice due to its efficiency and additional functionalities. However, for general use and when working with mixed data types, Python’s native 2D arrays would be more suitable.
Troubleshooting Python 2D Arrays
While working with 2D arrays in Python, you might encounter a few common issues. This section will discuss these problems and how to overcome them.
Out of Range Errors
One common issue is the ‘IndexError: list index out of range’ error. This occurs when you try to access an element at an index that doesn’t exist in the array. For example:
array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(array[3][0])
# Output:
# IndexError: list index out of range
In this example, we tried to access the element in the fourth row, first column. However, our array only has three rows, so we get an ‘IndexError’.
To avoid this error, always ensure that the indices you’re using to access elements are within the range of the array’s dimensions.
Modifying Immutable Elements
Another common issue is trying to modify an element in an array of immutable elements, like strings. Python’s strings are immutable, so if you try to change a character in a string within a 2D array, you’ll get a ‘TypeError’.
To overcome this issue, you can create a new string with the desired changes and replace the old string in the array.
Memory Considerations
When working with large 2D arrays, memory can become a concern. Python’s native 2D arrays (list of lists) can consume a lot of memory due to their flexible nature. If you’re working with large 2D arrays, you might want to consider using numpy 2D arrays, which are more memory-efficient.
Understanding Arrays in Python
Before we delve deeper into the intricacies of 2D arrays in Python, it’s essential to understand the fundamental concept of arrays and their implementation in Python.
The Concept of Arrays
An array is a data structure that stores a fixed-size sequence of elements of the same type. It’s like a container that holds a specific number of items, and all items are of the same type. This structure is beneficial when you want to store multiple items of the same type, like a list of integers or a list of strings.
In Python, arrays can be created using the ‘array’ module, but more often than not, lists are used instead due to their flexibility and functionality. In fact, Python’s ‘array’ module arrays are quite limited compared to lists, and are only used when you need to perform some operation that requires a specific functionality provided by these arrays.
Python’s Implementation of Arrays
In Python, arrays are implemented as lists. A list in Python is an ordered collection of items, and these items can be of any type. This is different from the traditional concept of arrays, where all elements must be of the same type.
Here’s an example of a Python list:
list_example = [1, 'two', 3.0, 'four']
print(list_example)
# Output:
# [1, 'two', 3.0, 'four']
As you can see, Python lists (or arrays) are very flexible. They can store items of different types, and they can be resized dynamically (items can be added or removed).
Arrays as Part of Python’s Data Structures
In the broader context of Python’s data structures, arrays (or lists) are just one of many. Python also provides other data structures like tuples, sets, and dictionaries, each with its own advantages and use cases.
However, when it comes to storing and manipulating a collection of related items, arrays are one of the most commonly used data structures due to their flexibility and the wide range of operations they support.
Expanding Your Knowledge: 2D Arrays and Beyond
Understanding 2D arrays is just the beginning. They are the stepping stones to more complex data structures and techniques. The concept of 2D arrays is not limited to small scripts but is widely used in larger projects, data analysis, machine learning, and more. Let’s explore some of these related concepts.
Exploring 3D Arrays and Matrices
Once you’re comfortable with 2D arrays, you might want to explore 3D arrays. These are arrays of 2D arrays, adding an extra level of complexity and usefulness. They are particularly useful in fields like physics and computer graphics.
Matrices, which are a mathematical concept, can be represented as 2D arrays in Python. You can perform various matrix operations using Python’s numpy library.
Data Manipulation with Pandas
Pandas is a Python library used for data manipulation and analysis. It provides data structures and functions needed to manipulate structured data.
It includes two primary data structures, Series (1-dimensional) and DataFrame (2-dimensional), to handle a vast majority of typical use cases in finance, statistics, social science, and engineering.
Further Resources for Mastering Python Arrays
To deepen your understanding of Python arrays and related concepts, here are some resources you might find useful:
- Python Data Types Tutorial: Getting Started – Learn how Python handles integers, floats, and complex numbers for numerical processing.
Simplifying Variable Usage in Python – Learn how to declare and manipulate variables in Python for effective programming.
Set Modification in Python Explained – Master the art of modifying sets in Python to perform set operations effectively.
Python’s official documentation provides a comprehensive overview of data structures, including lists (or arrays).
Numpy’s official documentation is a great resource for understanding numpy arrays and the operations you can perform on them.
Pandas’ official documentation is a must-read if you’re interested in data analysis or data manipulation in Python.
Wrapping Up: Mastering 2D Arrays in Python
In this comprehensive guide, we’ve delved into the world of Python 2D arrays, exploring their creation, manipulation, and usage. From basic operations to advanced techniques, we’ve covered a wide range of topics to give you a solid understanding of 2D arrays in Python.
We began with the basics, learning how to create a 2D array, access and modify its elements, and iterate over it. We then ventured into more advanced territory, exploring multi-dimensional arrays and nested list comprehensions.
Along the way, we tackled common issues you might encounter when working with 2D arrays in Python, such as out of range errors and memory considerations, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handling 2D arrays, introducing the numpy library and its advantages and disadvantages. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Python’s native 2D arrays | Flexible, can hold different types of data | Can consume a lot of memory for large arrays |
Numpy 2D arrays | Efficient, provides more functionalities | All elements must be of the same type |
Whether you’re a beginner just starting out with Python 2D arrays or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of 2D arrays in Python and their capabilities. Happy coding!