Understanding ‘else if’ in Java: A Detailed Tutorial
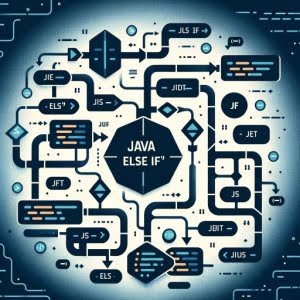
Are you finding it challenging to understand the ‘else if’ statement in Java? You’re not alone. Many developers find themselves puzzled when trying to control the flow of their programs using ‘else if’.
Think of the ‘else if’ statement as a traffic cop directing traffic – it helps control the flow of your program, ensuring that the right code is executed at the right time based on specific conditions.
In this guide, we will walk you through the use of ‘else if’ in Java, from basic use to advanced techniques. We’ll cover everything from the basics of ‘else if’, its advantages, potential pitfalls, to more advanced uses and alternative approaches.
So, let’s dive in and start mastering the ‘else if’ statement in Java!
TL;DR: How Do I Use ‘else if’ in Java?
The ‘else if’ statement in Java is used to test multiple conditions in a specific order, declared with the syntax,
if (firstCondition) {// code to execute} else if (secondCondition) {// code to execute}
If the condition in the ‘if’ statement is false, the program checks the condition in the ‘else if’ statement. If this condition is true, the code block within the ‘else if’ statement is executed.
Here’s a simple example:
int x = 20;
if (x > 30) {
System.out.println('x is greater than 30');
} else if (x > 10) {
System.out.println('x is greater than 10');
}
// Output:
// 'x is greater than 10'
In this example, the program first checks if x
is greater than 30. Since x
is 20, this condition is false. The program then moves to the ‘else if’ statement and checks if x
is greater than 10. Since this condition is true, the program prints ‘x is greater than 10’.
This is just a basic way to use the ‘else if’ statement in Java, but there’s much more to learn about controlling the flow of your programs. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- The ‘else if’ Statement in Java: A Detailed Explanation
- Delving Deeper: Nested ‘else if’ Statements in Java
- Exploring Alternatives: Beyond ‘else if’ in Java
- Troubleshooting ‘else if’ in Java: Common Issues and Solutions
- Understanding Control Flow in Java
- The Relevance of ‘else if’ in Larger Programs
- Exploring Related Concepts in Java
- Wrapping Up: Mastering the ‘else if’ Statement in Java
The ‘else if’ Statement in Java: A Detailed Explanation
The ‘else if’ statement in Java is a powerful tool used to test multiple conditions in a specific order. It is an extension of the ‘if’ statement and is always used in conjunction with it.
Here’s how it works: The program first checks the condition in the ‘if’ statement. If this condition is false, the program moves on to the condition in the ‘else if’ statement. If the ‘else if’ condition is true, the code block within the ‘else if’ statement is executed. If the ‘else if’ condition is also false, the program moves to the next ‘else if’ statement (if there is one), or to the ‘else’ statement (if there is one).
Let’s consider a simple example:
int score = 85;
if (score > 90) {
System.out.println('Excellent');
} else if (score > 80) {
System.out.println('Good');
} else if (score > 70) {
System.out.println('Fair');
} else {
System.out.println('Poor');
}
// Output:
// 'Good'
In this example, the program first checks if the score is greater than 90. Since the score is 85, this condition is false. The program then moves to the ‘else if’ statement and checks if the score is greater than 80. Since this condition is true, the program prints ‘Good’ and ignores the remaining ‘else if’ and ‘else’ statements.
The ‘else if’ statement is advantageous because it allows for multiple conditions to be checked in a specific order. However, a potential pitfall is that the order of the conditions matters. If the conditions are not ordered correctly, the program may not work as expected. For instance, if we had placed the ‘score > 80’ condition before the ‘score > 90’ condition in the above example, the program would always print ‘Good’ for scores greater than 80, even if they are also greater than 90.
Delving Deeper: Nested ‘else if’ Statements in Java
As you gain more experience with Java, you’ll encounter situations that require more complex decision-making processes. This is where nested ‘else if’ statements come into play.
A nested ‘else if’ statement is an ‘else if’ statement within another ‘else if’ statement. This allows for more complex conditions to be checked.
Here’s an example:
int x = 35;
if (x > 30) {
System.out.println('x is greater than 30');
if (x < 40) {
System.out.println('x is less than 40');
} else if (x < 50) {
System.out.println('x is less than 50');
}
} else if (x > 20) {
System.out.println('x is greater than 20');
}
// Output:
// 'x is greater than 30'
// 'x is less than 40'
In this example, the program first checks if x
is greater than 30. Since x
is 35, this condition is true, and the program prints ‘x is greater than 30’. The program then moves to the nested ‘if’ statement and checks if x
is less than 40. Since this condition is also true, the program prints ‘x is less than 40’. The remaining ‘else if’ and ‘else’ statements are ignored.
Nested ‘else if’ statements allow for more precise control over the flow of your program. However, they can also make your code more complex and harder to read. Therefore, it’s important to use them sparingly and always consider whether there’s a simpler way to achieve the same result.
Exploring Alternatives: Beyond ‘else if’ in Java
While ‘else if’ statements are a powerful tool for controlling the flow of your Java programs, they are not the only tool at your disposal. In fact, Java provides other constructs that can sometimes be more effective, depending on the situation. One such alternative is the ‘switch’ statement.
The ‘switch’ statement is particularly useful when you need to check a variable against multiple values. It can often result in cleaner and more readable code compared to a series of ‘else if’ statements.
Here’s an example of how you might use a ‘switch’ statement:
int day = 3;
switch (day) {
case 1:
System.out.println('Monday');
break;
case 2:
System.out.println('Tuesday');
break;
case 3:
System.out.println('Wednesday');
break;
// ... and so on for the rest of the week
default:
System.out.println('Invalid day');
}
// Output:
// 'Wednesday'
In this example, the program checks the value of day
against each case in the ‘switch’ statement. When it finds a match (in this case, day
is 3, which matches ‘Wednesday’), it executes the associated code block and then breaks out of the ‘switch’ statement.
The ‘switch’ statement can be a more efficient alternative to ‘else if’ when you’re dealing with multiple discrete values. However, it’s less flexible than ‘else if’, as it can’t handle ranges or complex conditions. As always, the best tool depends on the specific requirements of your program.
Remember, Java is a versatile language with a range of constructs designed to help you control the flow of your programs. By understanding these different tools and when to use them, you can write more efficient, readable, and effective code.
Troubleshooting ‘else if’ in Java: Common Issues and Solutions
While ‘else if’ is a powerful tool for controlling the flow of your Java programs, it’s not without its quirks. Here, we’ll discuss some common issues you might encounter when using ‘else if’, along with solutions and workarounds.
Forgetting the Final ‘else’ Statement
One common mistake is forgetting to include a final ‘else’ statement. This can lead to unexpected behavior if none of the ‘if’ or ‘else if’ conditions are true.
Consider the following example:
int x = 5;
if (x > 10) {
System.out.println('x is greater than 10');
} else if (x > 20) {
System.out.println('x is greater than 20');
}
// Output:
// (nothing)
In this case, because x
is not greater than 10 or 20, none of the conditions are true, and the program does not output anything. To handle such cases, it’s a good practice to include a final ‘else’ statement to catch all other scenarios.
int x = 5;
if (x > 10) {
System.out.println('x is greater than 10');
} else if (x > 20) {
System.out.println('x is greater than 20');
} else {
System.out.println('x is less than or equal to 10');
}
// Output:
// 'x is less than or equal to 10'
By including this final ‘else’ statement, we ensure that the program always outputs something, even if none of the ‘if’ or ‘else if’ conditions are true.
Remember, ‘else if’ is a powerful tool, but like all tools, it’s not without its quirks. By understanding these quirks and knowing how to troubleshoot them, you can use ‘else if’ more effectively in your Java programs.
Understanding Control Flow in Java
Before we delve deeper into ‘else if’ statements, it’s essential to understand the concept of control flow in Java. Control flow refers to the order in which the program executes statements. In Java, control flow is determined by conditional statements, loops, and function calls.
The Role of ‘else if’ in Control Flow
The ‘else if’ statement plays a crucial role in controlling the flow of Java programs. It allows the program to choose between different paths based on certain conditions.
Consider this simple ‘else if’ statement:
int x = 15;
if (x > 20) {
System.out.println('x is greater than 20');
} else if (x > 10) {
System.out.println('x is greater than 10');
} else {
System.out.println('x is less than or equal to 10');
}
// Output:
// 'x is greater than 10'
In this example, the program first checks if x
is greater than 20. Since x
is 15, this condition is false. The program then moves to the ‘else if’ statement and checks if x
is greater than 10. Since this condition is true, the program prints ‘x is greater than 10’ and ignores the ‘else’ statement.
The Importance of Order in ‘else if’ Statements
The order of conditions in ‘else if’ statements is crucial. The program checks the conditions from top to bottom and executes the code block of the first true condition it encounters. Once a condition is met, the program skips the rest of the ‘else if’ and ‘else’ statements.
This means that if you place a more general condition before a more specific one, the program will never reach the more specific condition. For example, if you swap the conditions in the previous example, the program will always print ‘x is greater than 10’ for numbers greater than 10, even if they’re also greater than 20.
Understanding the fundamentals of control flow and the role of ‘else if’ statements is key to mastering Java programming. With this knowledge, you can write more efficient and effective code.
The Relevance of ‘else if’ in Larger Programs
In larger, more complex programs, ‘else if’ plays a crucial role in decision-making processes. It allows the program to choose between different paths based on a variety of conditions, making the program more dynamic and adaptable to different situations.
Consider a program that manages a digital library. The program could use ‘else if’ statements to determine what actions to take based on the user’s input. For example:
String input = getUserInput();
if (input.equals('borrow')) {
borrowBook();
} else if (input.equals('return')) {
returnBook();
} else if (input.equals('search')) {
searchBooks();
} else {
System.out.println('Invalid input');
}
In this example, the program performs different actions based on the user’s input, thanks to the ‘else if’ statement.
Exploring Related Concepts in Java
While ‘else if’ is a fundamental concept in Java, it’s just one part of the bigger picture. To truly master Java, you should also explore related concepts like loops and other control flow statements.
Loops, such as ‘for’, ‘while’, and ‘do while’, allow you to repeat a block of code multiple times. Other control flow statements, such as ‘switch’, provide more ways to control the flow of your program.
By understanding these related concepts, you can write more efficient and effective Java programs.
Further Resources for Mastering Java Control Flow
To deepen your understanding of ‘else if’ and other control flow concepts in Java, check out the following resources:
- Best Practices for Using Loops in Java – Discover advanced loop techniques for data processing in Java.
Java If-Else – Learn how to use if-else constructs to handle binary decisions in Java programs.
Java If Statement – Understand how to use if statements to make decisions based on boolean expressions
Oracle’s Java Tutorials cover all aspects of the language, including control flow statements.
Java Control Flow: if, if else, and switch article from Baeldung provides an overview of ‘if’, ‘else if’, and ‘switch’ statements.
Java Programming for Beginners on Udemy covers the basics of Java programming, including control flow.
Wrapping Up: Mastering the ‘else if’ Statement in Java
In this comprehensive guide, we’ve explored the ‘else if’ statement in Java, a fundamental tool for controlling the flow of your programs.
We began with the basics, looking at how to use ‘else if’ in simple scenarios and understanding its role in Java’s control flow. We then delved into more advanced usage, such as nested ‘else if’ statements, and discussed common issues that you might encounter when using ‘else if’, along with solutions and workarounds.
We also looked at alternative approaches to control flow in Java, such as the ‘switch’ statement. We compared these alternatives with ‘else if’, giving you a sense of the broader landscape of tools for controlling the flow of your programs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
‘else if’ | High | Moderate | Multiple conditions, ordered checks |
‘switch’ | Moderate | Low | Multiple discrete values |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of control flow, we hope this guide has shed light on the ‘else if’ statement and its alternatives.
Understanding ‘else if’ is a crucial step in mastering Java. With this knowledge, you can write more efficient and effective Java programs. Happy coding!