[SOLVED] Bash ‘Unary Operator Expected’ Error
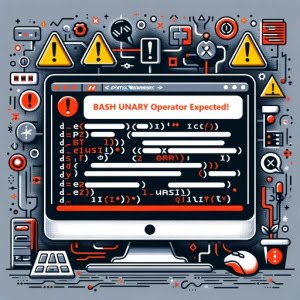
Ever found yourself stuck with a ‘bash unary operator expected’ error? You’re not alone. Many developers encounter this common Bash error, but it can be like trying to solve a mystery without any clues.
Think of this error as a detective’s case – it’s all about tracking down the missing or misbehaving variables in your Bash script. Once you know what to look for, the error becomes relatively simple to solve.
This guide will walk you through understanding and fixing the ‘bash unary operator expected’ error, from basic to advanced scenarios. We’ll cover everything from the basics of why this error occurs to more advanced techniques for preventing it in the future.
So, let’s put on our detective hats and start solving this Bash mystery!
TL;DR: How Do I Fix the ‘Bash Unary Operator Expected’ Error?
To fix the
'bash unary operator expected'
, ensure all variables in your comparisons are assigned and not empty. This error typically occurs when you’re comparing variables in a Bash script and one of the variables is unassigned or empty.
Here’s a simple example:
var=""
if [ -n "$var" ]; then
echo "Variable is not empty"
else
echo "Variable is empty"
fi
# Output:
# 'Variable is empty'
In this example, we’ve declared a variable var
and assigned it an empty string. The if
statement checks if the variable is not empty (-n "$var"
). Since var
is indeed empty, the script echoes ‘Variable is empty’.
This is a basic way to handle the ‘bash unary operator expected’ error, but there’s much more to learn about handling variables and comparisons in Bash. Continue reading for more detailed explanations and advanced scenarios.
Table of Contents
- Understanding the ‘Bash Unary Operator Expected’ Error
- Tackling Complex Scenarios: Nested If Statements and Loops
- Expert Techniques: Avoiding ‘Bash Unary Operator Expected’ Error
- Troubleshooting ‘Bash Unary Operator Expected’ and Related Errors
- Diving Deeper: Operators and Variables in Bash Scripting
- Expanding Your Bash Scripting Horizons
- Wrapping Up: Mastering the ‘Bash Unary Operator Expected’ Error
Understanding the ‘Bash Unary Operator Expected’ Error
The ‘bash unary operator expected’ error is a common issue that arises when you’re comparing variables in a Bash script, and one of the variables is unassigned or empty. This is because bash tries to evaluate an expression with an operator but only finds one operand, hence the term ‘unary operator expected’.
Let’s dive into a basic scenario to understand this more clearly.
var1=10
var2=
if [ $var1 -gt $var2 ]; then
echo "Var1 is greater than Var2"
else
echo "Var1 is not greater than Var2"
fi
# Output:
# ./script.sh: line 4: [: 10: unary operator expected
In this script, we have two variables: var1
which is assigned the value 10, and var2
which is empty. The if
statement attempts to compare these two variables using the -gt
(greater than) operator. However, because var2
is unassigned, Bash throws a ‘unary operator expected’ error.
To fix this, we need to ensure that all variables in our comparisons are assigned and not empty. Here’s how you can handle this situation:
var1=10
var2=0
if [ $var1 -gt $var2 ]; then
echo "Var1 is greater than Var2"
else
echo "Var1 is not greater than Var2"
fi
# Output:
# 'Var1 is greater than Var2'
In the corrected script, var2
is assigned a value of 0. Now, the comparison can be made without any errors. The script successfully checks if var1
is greater than var2
, and as expected, echoes ‘Var1 is greater than Var2’.
Remember, Bash operates under certain rules and expectations. When we don’t meet these (like trying to compare an unassigned variable), Bash lets us know with errors like ‘bash unary operator expected’.
Tackling Complex Scenarios: Nested If Statements and Loops
In more complex scripts that involve nested if statements or loops, the ‘bash unary operator expected’ error can become trickier to diagnose and resolve. Let’s explore an intermediate-level scenario.
for i in 1 2 3
do
var=
if [ $i -gt 2 -a -n "$var" ]; then
echo "i is greater than 2 and var is not empty"
else
echo "Either i is not greater than 2 or var is empty"
fi
done
# Output:
# ./script.sh: line 4: [: 1: unary operator expected
# Either i is not greater than 2 or var is empty
# ./script.sh: line 4: [: 2: unary operator expected
# Either i is not greater than 2 or var is empty
# ./script.sh: line 4: [: 3: unary operator expected
# Either i is not greater than 2 or var is empty
In this script, we’re looping over the values 1, 2, and 3, assigning each to i
in turn. Inside the loop, we have an empty variable var
and an if
statement that checks two conditions: if i
is greater than 2 and if var
is not empty. However, because var
is unassigned, we again encounter the ‘bash unary operator expected’ error.
To fix this, we need to ensure var
is assigned a value before the comparison. Here’s the corrected script:
for i in 1 2 3
do
var="value"
if [ $i -gt 2 -a -n "$var" ]; then
echo "i is greater than 2 and var is not empty"
else
echo "Either i is not greater than 2 or var is empty"
fi
done
# Output:
# Either i is not greater than 2 or var is empty
# Either i is not greater than 2 or var is empty
# i is greater than 2 and var is not empty
In the corrected script, var
is assigned the string ‘value’. Now, the script successfully checks the conditions in the if
statement without any errors. For the first two iterations, i
is not greater than 2, so the script echoes ‘Either i is not greater than 2 or var is empty’. On the third iteration, both conditions are true, so it echoes ‘i is greater than 2 and var is not empty’.
In more complex scripts, it’s crucial to keep track of all variables and their values, especially when dealing with comparisons in nested if statements or loops.
Expert Techniques: Avoiding ‘Bash Unary Operator Expected’ Error
While ensuring all variables are assigned before comparison is a valid approach, there are alternative methods to avoid the ‘bash unary operator expected’ error. These methods can provide additional robustness to your scripts.
Using Different Types of Comparisons
One such method involves using different types of comparisons. For instance, instead of using -n
to check if a variable is not empty, you can use -z
to check if it is empty. Here’s an example:
var=""
if [ -z "$var" ]; then
echo "Variable is empty"
else
echo "Variable is not empty"
fi
# Output:
# 'Variable is empty'
In this script, the if
statement checks if var
is empty (-z "$var"
). Since var
is indeed empty, the script echoes ‘Variable is empty’. This approach reduces the risk of encountering a ‘bash unary operator expected’ error when dealing with unassigned or empty variables.
Using the ‘set’ Command
Another alternative approach involves using the ‘set’ command to treat unset variables and parameters as an error. This makes your script fail early, where the problem is, rather than later when the variable is used.
Here’s an example:
set -u
var1=10
var2=
if [ $var1 -gt $var2 ]; then
echo "Var1 is greater than Var2"
else
echo "Var1 is not greater than Var2"
fi
# Output:
# ./script.sh: line 6: var2: unbound variable
In this script, the set -u
command is used to treat unset variables as an error. When the script attempts to compare var1
and var2
, it fails immediately because var2
is unassigned. This approach can help you detect issues early in the script execution.
These alternative approaches offer benefits but also have drawbacks. Different types of comparisons can make your script more robust but might require more complex logic. The ‘set’ command provides early error detection but could make your script fail for optional variables. Therefore, it’s important to consider your specific needs and script complexity when deciding which approach to use.
Troubleshooting ‘Bash Unary Operator Expected’ and Related Errors
While the ‘bash unary operator expected’ error is a common issue, there are other related errors that you might encounter when dealing with Bash scripts. Let’s discuss some of these errors, their solutions, and some best practices for optimization.
Dealing with Missing Brackets
A common error related to ‘bash unary operator expected’ is forgetting to surround the test condition with brackets. Here’s an example:
var1=10
if [ $var1 -gt 5
echo "Var1 is greater than 5"
else
echo "Var1 is not greater than 5"
fi
# Output:
# ./script.sh: line 3: syntax error near unexpected token `echo'
# ./script.sh: line 3: ` echo "Var1 is greater than 5"'
In this script, the closing bracket after the test condition in the if
statement is missing, which results in a syntax error. To fix this, you need to ensure the test condition is correctly enclosed in brackets:
var1=10
if [ $var1 -gt 5 ]; then
echo "Var1 is greater than 5"
else
echo "Var1 is not greater than 5"
fi
# Output:
# 'Var1 is greater than 5'
Using Double Brackets for String Comparisons
Another useful tip is to use double brackets [[ ]]
when dealing with string comparisons. Double brackets provide more robustness and allow for additional functionality compared to single brackets.
var1="bash"
if [[ $var1 == "bash" ]]; then
echo "Var1 is bash"
else
echo "Var1 is not bash"
fi
# Output:
# 'Var1 is bash'
In this script, the if
statement uses double brackets to compare var1
to the string ‘bash’. As var1
indeed equals ‘bash’, the script echoes ‘Var1 is bash’.
Remember, troubleshooting and optimization are part of the scripting process. By understanding common errors and how to fix them, you can write more efficient and error-free Bash scripts.
Diving Deeper: Operators and Variables in Bash Scripting
Understanding the fundamentals of Bash scripting is key to avoiding errors like ‘bash unary operator expected’. Two crucial concepts in Bash scripting are operators and variables.
The Role of Operators
Operators in Bash are used to perform operations on variables and values. There are various types of operators, such as arithmetic operators (-gt
, -lt
, -eq
, etc.) for numeric comparisons and string operators (==
, !=
, etc.) for string comparisons.
Here’s an example of using arithmetic operators:
var1=5
var2=10
if [ $var1 -lt $var2 ]; then
echo "Var1 is less than Var2"
else
echo "Var1 is not less than Var2"
fi
# Output:
# 'Var1 is less than Var2'
In this script, the -lt
operator is used to check if var1
is less than var2
. As var1
is indeed less than var2
, the script echoes ‘Var1 is less than Var2’.
Variables: The Building Blocks of Bash
Variables in Bash are used to store information. Assigning values to variables correctly is crucial to the functioning of your Bash script. An unassigned or empty variable can lead to errors like ‘bash unary operator expected’.
Here’s an example of variable assignment:
name="Alice"
echo "Hello, $name"
# Output:
# 'Hello, Alice'
In this script, the variable name
is assigned the value ‘Alice’. The echo
command then uses this variable to print ‘Hello, Alice’.
Understanding the role of operators and variables in Bash scripting, and the importance of proper variable assignment, can help you write more robust and error-free scripts.
Expanding Your Bash Scripting Horizons
Mastering the basics of Bash scripting, such as understanding the ‘bash unary operator expected’ error, is just the beginning. As you dive deeper into Bash scripting, you’ll realize the importance of proper operator and variable usage in larger scripts or projects.
Exploring Conditional Statements, Loops, and Functions
The power of Bash scripting goes beyond simple variable assignments and comparisons. You can construct complex scripts with conditional statements, loops, and functions. These tools allow you to control the flow of your script, perform operations repeatedly, and organize your code into reusable blocks.
Here’s an example of a Bash script that combines these elements:
function greet() {
local name=$1
echo "Hello, $name"
}
for name in Alice Bob Charlie
do
if [[ $name != "Charlie" ]]; then
greet $name
else
echo "No greeting for $name"
fi
done
# Output:
# 'Hello, Alice'
# 'Hello, Bob'
# 'No greeting for Charlie'
In this script, we define a function greet
that takes a name as an argument and prints a greeting. We then loop over the names Alice, Bob, and Charlie, greeting each one unless the name is Charlie.
Further Resources for Bash Scripting Proficiency
To continue your journey in mastering Bash scripting, here are some additional resources:
- The GNU Bash Reference Manual: This is the official manual for Bash and covers all its features in detail.
Bash Guide for Beginners: This guide provides a good introduction to Bash scripting for those new to the topic.
Advanced Bash-Scripting Guide: This guide is a more advanced resource for those who want to delve deeper into Bash scripting.
Remember, the journey to mastery is a marathon, not a sprint. Take your time, practice, and don’t be afraid to make mistakes. Happy scripting!
Wrapping Up: Mastering the ‘Bash Unary Operator Expected’ Error
In this comprehensive guide, we’ve dissected the ‘bash unary operator expected’ error, a common issue in Bash scripting. We’ve not only addressed its causes but also provided you with practical solutions and alternative approaches to avoid this error in the future.
We began with the basics, understanding what the ‘bash unary operator expected’ error is and why it occurs. We then delved into how to fix it in basic scenarios, ensuring that all variables in our comparisons are assigned and not empty. We also tackled more complex scenarios involving nested if statements and loops, demonstrating how to handle unassigned variables in these situations.
We explored alternative approaches to avoid the ‘bash unary operator expected’ error, such as using different types of comparisons and the ‘set’ command. We also discussed common errors related to the ‘bash unary operator expected’ error and their solutions, providing you with a robust toolkit for troubleshooting and optimization in Bash scripting.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Assigning Variables | Simple and straightforward | Requires careful tracking of all variables |
Different Types of Comparisons | More robust against unassigned variables | Might require more complex logic |
‘set’ Command | Provides early error detection | Could make script fail for optional variables |
Whether you’re a novice Bash user encountering the ‘bash unary operator expected’ error for the first time, or an experienced developer looking to enhance your Bash scripting skills, we hope this guide has provided you with valuable insights and practical solutions.
Remember, the key to effective Bash scripting lies in understanding and correctly using operators and variables. With the knowledge and strategies provided in this guide, you’re now well-equipped to tackle the ‘bash unary operator expected’ error head-on. Happy scripting!