Bash Printf Command: Your Guide to String Formatting
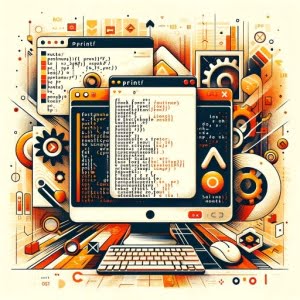
Are you finding it challenging to format your output in bash scripts? You’re not alone. Many developers find themselves puzzled when it comes to using the bash printf command, but we’re here to help.
Think of bash printf as a versatile tool – a tool that allows you to format and print data in bash scripts in a more controlled manner than echo. It’s a powerful command that can significantly enhance your scripting capabilities.
This guide will walk you through the basics to advanced usage of the bash printf command. We’ll cover everything from the basics of using printf, dealing with different format specifiers, to discussing alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering bash printf!
TL;DR: How Do I Use printf in Bash?
The
printf
command in bash is used to format and print data, with the syntasx,printf 'Hello, World!\n'
. It’s a powerful tool that can help you control the way your output is displayed in bash scripts.
Here’s a simple example:
printf 'Hello, %s\n' 'World'
# Output:
# 'Hello, World'
In this example, we use the printf command to print a formatted string. The %s
is a format specifier that tells printf to expect a string as an argument. The \n
is an escape sequence that adds a new line after the output. The ‘World’ is the argument that replaces the %s
in the output.
This is a basic way to use printf in bash, but there’s much more to learn about formatting and printing data in bash scripts. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Bash Printf: Basic Usage
- Delving Deeper: Advanced Usage of Bash Printf
- Exploring Alternatives: Echo and Awk
- Troubleshooting Common Bash Printf Issues
- Diving into Bash Scripting and Printf Fundamentals
- The Relevance of Bash Printf in Shell Scripting and Automation
- Exploring Related Concepts: Bash Variables and Control Structures
- Wrapping Up: Mastering Bash Printf for Effective Data Formatting
Understanding Bash Printf: Basic Usage
The printf command in bash is used to format and print data. It’s based on the printf function in C programming language and offers more control over formatting output compared to the echo command. The basic syntax of printf command is as follows:
printf format [arguments]
Here, the format is a string that includes text and format specifiers. The arguments are the values that will replace the format specifiers in the format string.
Let’s consider an example:
printf "Name: %s, Age: %d\n" Alice 25
# Output:
# Name: Alice, Age: 25
In this example, %s
and %d
are format specifiers where %s
expects a string and %d
expects an integer. The string ‘Alice’ and the integer 25 are arguments that replace %s
and %d
respectively in the output.
The printf command offers various advantages. It allows you to control the width of the output, align text, format numbers, and more. However, it’s crucial to use the correct format specifiers for the corresponding argument types to avoid unexpected results.
Delving Deeper: Advanced Usage of Bash Printf
As we move to the intermediate level of bash printf, we start dealing with different format specifiers and flags that provide more control over the output format. These include controlling the width of the output, aligning text, formatting numbers, and more.
Using Width and Precision Flags
Let’s start with an example where we control the width of the output with a width flag:
printf "%10s\n" Bash
# Output:
# Bash
In this example, the %10s
format specifier tells printf to reserve 10 characters for the string. Since ‘Bash’ is only 4 characters, it’s right-aligned with 6 spaces before it.
Now, let’s take precision flags, which control the number of digits after the decimal point:
printf "%.2f
" 3.14159
# Output:
# 3.14
Here, the %.2f
format specifier tells printf to print the floating-point number up to 2 places after the decimal point.
Left Aligning Text
You can also left-align text using the -
flag. Here’s how it works:
printf "%-10s\n" Bash
# Output:
# Bash
In this case, the -10s
format specifier tells printf to reserve 10 characters for the string and left-align it.
These are just a few examples of the many flags and specifiers you can use with printf in bash. The key is to understand what each flag and specifier does and use them appropriately in your scripts.
Exploring Alternatives: Echo and Awk
While bash printf is a powerful tool for formatting and printing data, it’s not the only method available in bash. Other commands, such as echo and awk, can also be used to format and print data. Let’s explore these alternatives and understand their usage and effectiveness.
Using Echo for Printing
The echo command is often the first choice for beginners due to its simplicity. Here’s a basic example:
echo "Hello, World"
# Output:
# Hello, World
In this example, we simply print a string ‘Hello, World’. However, echo lacks the advanced formatting options provided by printf.
Advanced Formatting with Awk
Awk is a powerful text processing command. It can handle complex tasks that are difficult or impossible with printf. Here’s an example of using awk to print formatted output:
echo | awk '{printf "Name: %s, Age: %d\n", "Alice", 25}'
# Output:
# Name: Alice, Age: 25
In this example, awk uses the printf function internally to format and print data. Awk can handle complex text processing tasks, but it’s more complex and has a steeper learning curve compared to printf.
While each of these methods has its advantages and disadvantages, the choice between them depends on your specific needs and the complexity of the task. For simple tasks, echo might be sufficient. For more complex formatting, printf is usually the better choice. And for complex text processing tasks, awk might be the best tool for the job.
Troubleshooting Common Bash Printf Issues
Like any command, using printf in bash can sometimes lead to unexpected results. Here, we’ll discuss some common issues you might encounter and how to troubleshoot them.
Incorrect Format Specifier
One common issue is using an incorrect format specifier. For example, if you use %d
(integer) with a string, you’ll get an unexpected result:
printf "%d\n" Hello
# Output:
# 0
In this example, printf tries to print ‘Hello’ as an integer, but since it’s a string, printf outputs 0. To fix this, use the correct format specifier for your data type.
Missing Arguments
Another common issue is missing arguments. If you provide fewer arguments than the format specifiers, printf will use a zero value for numeric format specifiers and an empty string for string specifiers:
printf "Name: %s, Age: %d\n" Alice
# Output:
# Name: Alice, Age: 0
In this example, we forgot to provide an argument for %d
. As a result, printf uses 0 as the default value.
Handling Special Characters
Special characters like backslash (\
) in the format string can also cause issues. To print a backslash, you need to escape it with another backslash:
printf "Path: %s\n" C:\\Users\\Alice
# Output:
# Path: C:\Users\Alice
In this example, we use \\
to print a single backslash. This is because the first \
escapes the second one, resulting in a single backslash in the output.
These are just a few examples of the issues you might encounter when using printf in bash. The key to troubleshooting is understanding how printf works and what each format specifier and flag does. With this knowledge, you can quickly identify and fix issues in your scripts.
Diving into Bash Scripting and Printf Fundamentals
To fully grasp the power and functionality of the bash printf command, it’s essential to understand the fundamentals of bash scripting and format specifiers.
Bash Scripting Basics
Bash (Bourne Again Shell) is a popular shell and command language interpreter for the GNU operating system. Bash scripting is writing a series of commands for the bash interpreter to execute. These scripts can automate tasks, perform file manipulations, and much more.
Here’s a simple bash script that uses the echo command to print ‘Hello, World’:
#!/bin/bash
echo 'Hello, World'
# Output:
# Hello, World
In this script, #!/bin/bash
is a shebang that tells the system to execute the script using bash. The echo 'Hello, World'
command prints ‘Hello, World’.
Understanding the Printf Command
Printf stands for ‘print formatted’, which comes from C programming language. It’s more advanced than echo, offering better control over the output format. As we’ve seen in previous sections, you can use printf to control the width of the output, align text, format numbers, and more.
Format Specifiers Demystified
Format specifiers are codes that begin with a percent sign (%
) used within the format string of the printf command. They specify the type and format of the data to be printed. Here are some common format specifiers:
%s
: String%d
: Decimal integer%f
: Floating-point number
Here’s a simple example of using format specifiers:
printf 'Name: %s, Age: %d\n' 'Alice' 25
# Output:
# Name: Alice, Age: 25
In this example, %s
and %d
are format specifiers replaced by ‘Alice’ and 25 in the output.
Understanding these fundamentals is crucial to mastering the bash printf command and effectively formatting and printing data in bash scripts.
The Relevance of Bash Printf in Shell Scripting and Automation
Bash printf isn’t just a command; it’s a vital tool for shell scripting and automation tasks. The ability to format and print data precisely comes in handy in a variety of scenarios.
Let’s consider an automation task where you need to generate a report with neatly formatted data. Bash printf can help you format the data exactly as required, making the report easy to read and understand.
Or, consider a shell script where you need to display progress information to the user. Bash printf allows you to control the output format, align text, and even overwrite the current line, making it perfect for creating progress bars.
Exploring Related Concepts: Bash Variables and Control Structures
While mastering bash printf is a significant step forward, it’s equally important to understand related concepts like bash variables and control structures.
Bash variables allow you to store and manipulate data in your scripts. Control structures like if-else statements, loops, and case statements allow you to control the flow of your scripts.
Here’s a simple example of using a bash variable and an if-else statement with printf:
#!/bin/bash
name="Alice"
if [[ -n $name ]]; then
printf "Hello, %s\n" $name
else
printf "No name provided\n"
fi
# Output:
# Hello, Alice
In this script, we first assign the string ‘Alice’ to the variable name
. Then we use an if-else statement to check if name
is non-empty. If name
is non-empty, we use printf to print a greeting. If name
is empty, we print a different message.
Further Resources for Mastering Bash Printf
If you’re interested in delving deeper into bash printf and related concepts, here are some resources to help you on your journey:
- GNU Bash Reference Manual: This is the official manual for bash, including a detailed section on the printf command.
Advanced Bash-Scripting Guide: This guide covers bash scripting in depth, with plenty of examples and exercises.
Bash printf Command: This Linuxize guide provides a comprehensive guide on the Bash printf command.
Wrapping Up: Mastering Bash Printf for Effective Data Formatting
In this comprehensive guide, we’ve explored the ins and outs of the bash printf command, a powerful tool for formatting and printing data in bash scripts.
We began with the basics, understanding how to use printf in bash, and how it works with different format specifiers. We then delved into more advanced usage, discussing how to deal with different format specifiers and flags while using printf, and how to troubleshoot common issues.
We also explored alternative approaches to data formatting and printing in bash, such as using the echo command or awk. Each of these methods has its advantages and disadvantages, and the choice between them depends on your specific needs and the complexity of the task.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Bash Printf | Advanced formatting options, control over output | Requires understanding of format specifiers |
Echo | Simple and easy to use | Lacks advanced formatting options |
Awk | Powerful text processing capabilities | More complex, steeper learning curve |
Whether you’re just starting out with bash printf or you’re looking to level up your bash scripting skills, we hope this guide has given you a deeper understanding of bash printf and its capabilities.
With its balance of power and flexibility, bash printf is a vital tool for any bash scripter. Happy scripting!