Split Strings Into Arrays in Bash: Linux Shell Scripting
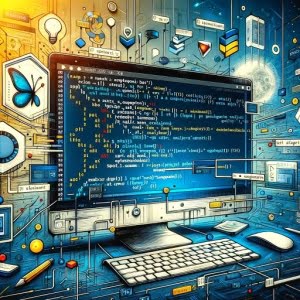
Are you finding it challenging to split a string into an array in Bash? You’re not alone. Many developers grapple with this task, but there are tools that can make this process a breeze. Once you have mastered these tools, you will have gained a fundamental skill in Bash scripting, providing a versatile and handy tool for various tasks.
This guide will walk you through the process of splitting a string into an array in Bash, from the basics to more advanced techniques. We’ll cover everything from the basic use of the read
command, handling strings with special characters, to alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering string splitting in Bash!
TL;DR: How Do I Split a String into an Array in Bash?
To split a string into an array in Bash, you can use the
read
command with the-a
option and the syntax,read -a array <<< "$string"
. This command reads a line from the standard input and splits it into fields, storing them in an array.
Here’s a quick example:
string='Hello World'
read -a array <<< "$string"
echo "${array[0]} ${array[1]}"
# Output:
# 'Hello'
# 'World'
In this example, we have a string ‘Hello World’. We use the read
command with the -a
option to split this string into an array. The `<< This is a basic way to split a string into an array in Bash, but there’s much more to learn about string manipulation in Bash. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Bash Basics: Splitting Strings with the read Command
- Intermediate Techniques: Splitting Strings with Different Delimiters
- Expert Techniques: Alternative Methods for String Splitting
- Troubleshooting Common Issues in Bash String Splitting
- Best Practices and Optimization
- Understanding String Manipulation in Bash
- Expanding Horizons: String Splitting in Larger Scripts
- Wrapping Up: Mastering Bash String Splitting
Bash Basics: Splitting Strings with the read
Command
In Bash, one of the simplest ways to split a string into an array is by using the read
command with the -a
option. This option tells the read
command to split the input into an array instead of treating it as a single string. Let’s dive into a basic example to illustrate this.
string='Bash is fun to learn'
read -a array <<< "$string"
echo "${array[2]} ${array[3]} ${array[4]}"
# Output:
# 'fun to learn'
In this example, we have a string ‘Bash is fun to learn’. The read
command with the -a
option splits this string into an array. The <<< "$string"
part is a here-string, which feeds the value of the string variable to the read
command. The echo
command then prints the third, fourth, and fifth elements of the array, which are ‘fun’, ‘to’, and ‘learn’, respectively.
This approach is simple and straightforward, making it a great starting point for beginners. However, it’s worth noting that this method splits the string based on whitespace. If your string contains other delimiters or special characters, you may need to use more advanced techniques, which we will cover later in this guide.
Additionally, this method can lead to unexpected results if your string contains leading or trailing whitespace. The read
command will interpret these as empty array elements. To avoid this, it’s a good practice to trim any leading or trailing whitespace from your string before splitting it.
Intermediate Techniques: Splitting Strings with Different Delimiters
As you become more comfortable with Bash, you’ll encounter scenarios where you need to split a string based on a delimiter other than whitespace. For instance, you might need to split a comma-separated string into an array. Let’s explore how to do this.
string='Bash,Scripting,Is,Powerful'
IFS=',' read -a array <<< "$string"
echo "${array[0]} ${array[1]} ${array[2]} ${array[3]}"
# Output:
# 'Bash Scripting Is Powerful'
In this example, we’re using the IFS
(Internal Field Separator) variable to specify a comma as the delimiter. The read
command then splits the string ‘Bash,Scripting,Is,Powerful’ into an array based on this delimiter. The echo
command prints all the elements of the array, which are ‘Bash’, ‘Scripting’, ‘Is’, and ‘Powerful’.
Handling Strings with Special Characters
Now, let’s consider a more complex scenario where your string contains special characters. In Bash, special characters like spaces, tabs, and newlines can be included in a string using escape sequences. Let’s see how to handle this.
string='Bash
Scripting
Is
Powerful'
IFS='\\n' read -a array <<< "$string"
echo "${array[0]} ${array[1]} ${array[2]} ${array[3]}"
# Output:
# 'Bash Scripting Is Powerful'
In this example, we’re using the escape sequence to include newlines in the string. We then set
IFS
to this escape sequence and split the string into an array. The echo
command prints all the elements of the array, which are ‘Bash’, ‘Scripting’, ‘Is’, and ‘Powerful’.
These intermediate techniques allow you to handle a wider range of scenarios when splitting strings in Bash. However, they require a deeper understanding of how Bash interprets special characters and delimiters. As a best practice, always test your scripts thoroughly to ensure they handle all possible input correctly.
Expert Techniques: Alternative Methods for String Splitting
While using the read
command with the -a
option is a common way to split a string into an array in Bash, there are alternative methods that offer more flexibility and control. One such method involves using the IFS
(Internal Field Separator) variable. Let’s delve into this.
Using the IFS Variable for String Splitting
The IFS
variable in Bash determines how word splitting occurs after expansion. By changing the IFS
value, you can control how Bash splits a string into an array. Here’s an example:
string='Bash:Scripting:Can:Be:Fun'
oldIFS=$IFS
IFS=':'
read -a array <<< "$string"
IFS=$oldIFS
echo "${array[0]} ${array[1]} ${array[2]} ${array[3]} ${array[4]}"
# Output:
# 'Bash Scripting Can Be Fun'
In this example, we first save the original IFS
value to a variable oldIFS
. We then change IFS
to ‘:’, which is our desired delimiter. The read
command splits the string ‘Bash:Scripting:Can:Be:Fun’ into an array based on this delimiter. After the string splitting, we restore the original IFS
value to avoid affecting other parts of the script that rely on word splitting. The echo
command prints all the elements of the array, which are ‘Bash’, ‘Scripting’, ‘Can’, ‘Be’, and ‘Fun’.
This method provides a more granular control over string splitting, making it a powerful tool for complex scripts. However, it requires careful handling of the IFS
variable to avoid unintended side effects. As a best practice, always save the original IFS
value and restore it after the string splitting operation.
Making the Decision: Which Method to Use?
Choosing the right method to split a string into an array in Bash depends on your specific needs. If you’re dealing with simple strings and want a quick solution, the read
command with the -a
option is a great choice. If you need to handle complex strings with different delimiters or special characters, using the IFS
variable provides more flexibility. Ultimately, the best method is the one that suits your use case and ensures your script functions correctly and efficiently.
Troubleshooting Common Issues in Bash String Splitting
While Bash provides powerful tools for string manipulation, it’s not uncommon to encounter errors or obstacles. Let’s go over some common issues and their solutions.
Handling Empty Fields in the Array
One common issue when splitting a string into an array in Bash is handling empty fields. If your string has consecutive delimiters, Bash will interpret this as an empty field. Here’s an example:
string='Bash::Scripting'
IFS=':' read -a array <<< "$string"
echo "${array[0]} ${array[1]} ${array[2]}"
# Output:
# 'Bash'
# ''
# 'Scripting'
In this example, we have a string ‘Bash::Scripting’ with two consecutive colons. When we split this string into an array, Bash interprets the consecutive colons as an empty field. The echo
command prints three elements: ‘Bash’, an empty string, and ‘Scripting’.
To handle this issue, you can add a check in your script to ignore empty fields. This will ensure your array only contains meaningful data.
Dealing with Special Characters
Special characters in your string can also cause issues when splitting it into an array. For instance, if your string contains a backslash (\
), Bash will interpret it as an escape character, not a literal backslash. Here’s an example:
string='Bash\Scripting'
IFS='\\' read -a array <<< "$string"
echo "${array[0]} ${array[1]}"
# Output:
# 'Bash'
# 'Scripting'
In this example, we have a string ‘Bash\Scripting’. We set IFS
to '\\'
, which represents a literal backslash. The read
command then splits the string into an array based on this delimiter. The echo
command prints two elements: ‘Bash’ and ‘Scripting’.
This issue can be tricky to spot, especially if your string contains many special characters. As a best practice, always escape special characters in your string before splitting it.
Best Practices and Optimization
When splitting a string into an array in Bash, it’s important to follow best practices to ensure your script is efficient and maintainable. Always test your script with different inputs to ensure it handles all possible scenarios. If your script is part of a larger project, consider writing unit tests to automate this process. This will save you time in the long run and help prevent bugs in your code.
Understanding String Manipulation in Bash
Before we delve further into splitting strings into arrays in Bash, it’s crucial to understand the fundamentals of string manipulation in Bash.
What is String Manipulation?
String manipulation refers to the process of handling and altering strings – a sequence of characters. In Bash, strings are used extensively in scripting. They can represent text, filenames, commands, or any other data type. Therefore, being able to manipulate strings effectively is a critical skill for Bash scripting.
How Does Bash Handle Strings?
In Bash, strings are treated as a single unit by default. However, Bash provides several built-in commands and features for manipulating strings, such as the read
command, the IFS
(Internal Field Separator) variable, and various parameter expansions.
string='Bash scripting is fun'
echo ${#string}
# Output:
# 20
In this example, we use the ${#string}
parameter expansion to get the length of the string ‘Bash scripting is fun’. The echo
command prints the length of the string, which is 20.
The Importance of String Manipulation in Bash
String manipulation is a fundamental aspect of Bash scripting. It’s used in a wide range of tasks, from parsing input to generating output. Specifically, splitting a string into an array is a common task in Bash scripting. It allows you to break down a complex string into manageable parts, which can be processed individually.
Understanding these concepts will give you a solid foundation for mastering string splitting in Bash. With this knowledge, you’ll be better equipped to understand the techniques and examples discussed in this guide.
Expanding Horizons: String Splitting in Larger Scripts
The ability to split a string into an array in Bash is not just a standalone skill, but a building block for creating more complex scripts and projects. In larger scripts, you may need to parse input from a file, manipulate it, and then output the results. String splitting is a crucial part of this process.
For instance, consider a script that reads a CSV file line by line, splits each line into fields, and processes each field individually. Here’s a brief example:
while IFS=, read -r field1 field2 field3
do
echo "Field 1: $field1"
echo "Field 2: $field2"
echo "Field 3: $field3"
done < file.csv
# Output:
# Field 1: value1
# Field 2: value2
# Field 3: value3
In this example, we use a while
loop to read the CSV file. The IFS=,
part sets the delimiter to a comma, and the read
command splits each line into fields. The echo
commands then print each field.
Related Commands and Functions
When working with string splitting in Bash, you’ll often find yourself using related commands and functions. For example, parameter expansions like ${string#*delimiter}
and ${string##*delimiter}
can be used to remove a prefix from a string. Similarly, ${string%delimiter*}
and ${string%%delimiter*}
can be used to remove a suffix.
Here’s a brief example that demonstrates these parameter expansions:
string='Bash:Scripting:Can:Be:Fun'
echo "${string#*:}"
echo "${string##*:}"
echo "${string%:*}"
echo "${string%%:*}"
# Output:
# 'Scripting:Can:Be:Fun'
# 'Fun'
# 'Bash:Scripting:Can:Be'
# 'Bash'
In this example, the ${string#*:}
expansion removes the shortest prefix matching the pattern ‘:’, and ${string##*:}
removes the longest. Similarly, ${string%:*}
removes the shortest suffix matching the pattern ‘:‘, and ${string%%:*}
removes the longest.
Further Resources for Bash Scripting Mastery
To further your understanding of string manipulation in Bash, here are some resources that offer in-depth information:
- GNU Bash Manual: This is the official manual for Bash. It’s a comprehensive resource that covers all aspects of Bash scripting, including string manipulation.
Advanced Bash-Scripting Guide: This guide provides a detailed introduction to Bash scripting. It includes numerous examples and exercises that can help you hone your skills.
Bash Guide for Beginners: This guide is a great starting point for beginners. It covers the basics of Bash scripting and gradually introduces more advanced topics.
Remember, mastering string manipulation in Bash is a journey. Take the time to understand the concepts, practice with examples, and don’t be afraid to make mistakes. Happy scripting!
Wrapping Up: Mastering Bash String Splitting
In this comprehensive guide, we’ve journeyed through the process of splitting strings into arrays in Bash, a fundamental skill in Bash scripting.
We began with the basics, learning how to use the read
command to split a string into an array. We then ventured into more advanced territory, exploring different delimiters, handling special characters, and using the IFS
(Internal Field Separator) variable for more granular control.
Along the way, we tackled common issues you might face when splitting strings in Bash, such as handling empty fields and dealing with special characters, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to string splitting, giving you a sense of the broader landscape of Bash string manipulation techniques. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity |
---|---|---|
read command | Low | Low |
IFS variable | High | Moderate |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of string splitting in Bash.
The ability to split strings into arrays is a powerful tool for various tasks in Bash scripting. With this knowledge under your belt, you’re well equipped to tackle more complex scripts and projects. Happy scripting!