Python Excel Handling: Your Ultimate Guide
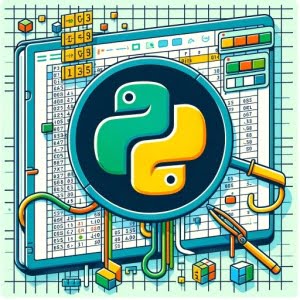
Are you finding it challenging to handle Excel files in Python? You’re not alone. Many developers find themselves in a similar predicament, but Python, like a skilled accountant, can easily manage Excel files with the help of a few libraries.
Python’s ability to interact with Excel files extends beyond mere reading and writing. It can manipulate data, apply formulas, and even handle complex operations like working with multiple worksheets. This versatility makes Python a powerful tool for data analysis and manipulation.
In this guide, we’ll walk you through the process of reading, writing, and manipulating Excel files using Python. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Excel with Python!
TL;DR: How Do I Work with Excel Files in Python?
To work with Excel files in Python, you can use the
pandas
library functions such asread_excel()
andto_excel()
. It allows you to read and write Excel files with ease. Here’s a simple example:
import pandas as pd
df = pd.read_excel('file.xlsx')
df.to_excel('output.xlsx')
# Output:
# An Excel file named 'output.xlsx' is created with the same data as 'file.xlsx'.
In this example, we import the pandas
library and use the read_excel
function to read an Excel file named ‘file.xlsx’. The data from the file is stored in a DataFrame df
. Then, we use the to_excel
function to write the data from the DataFrame to a new Excel file named ‘output.xlsx’.
This is a basic way to work with Excel files in Python, but there’s much more to learn about handling Excel files in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Python and Excel
Python’s pandas
library is a powerful tool for handling Excel files. It’s capable of reading and writing Excel files, among other things. Let’s start with the basics.
Reading Excel Files with Python
To read an Excel file in Python, we use the pd.read_excel()
function. Here’s a simple example:
import pandas as pd
df = pd.read_excel('file.xlsx')
print(df)
# Output:
# Displays the content of the Excel file 'file.xlsx' in the console.
In this example, we start by importing the pandas
library. We then use the pd.read_excel()
function to read the Excel file named ‘file.xlsx’. The content of the file is stored in a pandas
DataFrame, which we print to the console.
Writing Excel Files with Python
Writing to an Excel file is just as straightforward. We use the df.to_excel()
function, where df
is the DataFrame containing the data we want to write. Here’s how it works:
df.to_excel('output.xlsx')
# Output:
# Creates a new Excel file named 'output.xlsx' with the data from the DataFrame.
In this code block, we use the df.to_excel()
function to write the data from the DataFrame df
to a new Excel file named ‘output.xlsx’. If the file already exists, it will be overwritten.
Advantages and Potential Pitfalls
The pandas
library makes it simple to read and write Excel files, but there are a few things to keep in mind. The biggest advantage is its simplicity and efficiency. However, when dealing with large files, pandas
can be slow and consume a lot of memory. Also, it might not handle complex Excel features like formulas or macros.
Advanced Excel Operations with Python
As you become more comfortable with handling Excel files in Python, you might find yourself needing to perform more complex operations. This could include managing multiple worksheets, applying formulas, or formatting cells. Let’s explore these in more detail.
Handling Multiple Worksheets
Python’s pandas
library can handle multiple worksheets in an Excel file. Here’s how you can read from and write to multiple worksheets.
with pd.ExcelWriter('output.xlsx') as writer:
df1.to_excel(writer, sheet_name='Sheet1')
df2.to_excel(writer, sheet_name='Sheet2')
# Output:
# Creates an Excel file 'output.xlsx' with two worksheets 'Sheet1' and 'Sheet2'.
In this example, we use the ExcelWriter
object from the pandas
library to create an Excel file named ‘output.xlsx’. We then write two DataFrames (df1
and df2
) to separate worksheets named ‘Sheet1’ and ‘Sheet2’ respectively.
Applying Formulas
While pandas
doesn’t support Excel formulas directly, we can use the openpyxl
library to achieve this. Here’s an example:
from openpyxl import load_workbook
book = load_workbook('output.xlsx')
writer = pd.ExcelWriter('output.xlsx', engine='openpyxl')
writer.book = book
df.to_excel(writer, index=False, sheet_name='Sheet1')
writer.sheets['Sheet1']['A1'] = '=SUM(A2:A4)'
writer.save()
# Output:
# Adds a formula to cell A1 in 'Sheet1' of 'output.xlsx' that sums the values in cells A2 to A4.
In this case, we load the ‘output.xlsx’ file using openpyxl
‘s load_workbook
function. We then create an ExcelWriter
object using the ‘openpyxl’ engine and write the DataFrame df
to ‘Sheet1’. Finally, we add a SUM formula to cell A1 that adds the values in cells A2 to A4.
Formatting Cells
Again, while pandas
doesn’t directly support cell formatting, we can use openpyxl
for this. Here’s an example of how to change the font color of a cell:
from openpyxl.styles import Font, Color
sheet = book.active
cell = sheet['A1']
cell.font = Font(color='FFFF0000')
book.save('output.xlsx')
# Output:
# Changes the font color of cell A1 in 'output.xlsx' to red.
This code block changes the font color of cell A1 to red. We first access the active worksheet and then the cell we want to format (‘A1’). We then change the font color of the cell using the Font
class from openpyxl.styles
.
These are just a few examples of the advanced operations you can perform when working with Excel files in Python. With these tools at your disposal, you’ll be able to handle even the most complex Excel tasks with ease.
Exploring Alternative Libraries for Excel Handling
While pandas
is a powerful library for handling Excel files in Python, it’s not the only option. Other libraries, such as openpyxl
and xlrd
, can provide more flexibility or functionality in certain scenarios. Let’s explore these alternatives.
The Openpyxl Library
openpyxl
is another excellent library for working with Excel files. It’s especially useful when you need to read and write .xlsx files, and it supports more advanced Excel features like charts, images, and even conditional formatting.
Here’s a simple example of reading an Excel file with openpyxl
:
from openpyxl import load_workbook
wb = load_workbook(filename='file.xlsx')
sheet = wb.active
for row in sheet.iter_rows(values_only=True):
print(row)
# Output:
# Prints the content of each row in the active worksheet of 'file.xlsx'.
In this example, we import the load_workbook
function from openpyxl
, use it to load ‘file.xlsx’, and then print the contents of each row in the active worksheet.
The Xlrd Library
xlrd
is a library for reading data and formatting information from Excel files. While it doesn’t support writing to Excel files, it’s very efficient at reading them, and it supports both .xls and .xlsx files.
Here’s an example of how to read an Excel file with xlrd
:
import xlrd
book = xlrd.open_workbook('file.xlsx')
sheet = book.sheet_by_index(0)
for i in range(sheet.nrows):
print(sheet.row_values(i))
# Output:
# Prints the content of each row in the first worksheet of 'file.xlsx'.
In this code block, we use xlrd
‘s open_workbook
function to open ‘file.xlsx’ and then print the contents of each row in the first worksheet.
Choosing the Right Tool
Each of these libraries has its strengths and weaknesses. pandas
is powerful and versatile, but it can be slow with large files and doesn’t support some advanced Excel features. openpyxl
supports more advanced features and .xlsx files, but it’s slower than xlrd
and doesn’t support .xls files. xlrd
is fast and supports both .xls and .xlsx files, but it can only read, not write, Excel files.
In the end, the right tool depends on your specific needs. If you’re just getting started or need to both read and write Excel files, pandas
is a great choice. If you need to work with advanced Excel features or .xlsx files, consider openpyxl
. If you need to read large Excel files quickly, xlrd
might be the best option.
Troubleshooting Common Python-Excel Issues
Working with Excel files in Python can sometimes be tricky. Let’s discuss some common issues you might encounter and how to solve them.
Handling Large Files
When dealing with large Excel files, pandas
can be slow and consume a lot of memory. One solution is to read the file in chunks.
chunksize = 50000
chunks = []
for chunk in pd.read_excel('large_file.xlsx', chunksize=chunksize):
chunks.append(chunk)
# Output:
# Reads 'large_file.xlsx' in chunks of 50,000 rows and stores each chunk in a list.
This code reads ‘large_file.xlsx’ in chunks of 50,000 rows at a time, reducing memory usage.
Handling Different Data Types
Excel files can contain different data types (numbers, strings, dates, etc.), which pandas
might not interpret correctly. One solution is to specify the data type when reading the file.
import numpy as np
df = pd.read_excel('file.xlsx', dtype={'column1': np.float64, 'column2': 'string'})
# Output:
# Reads 'file.xlsx', interpreting the data in 'column1' as float64 and 'column2' as string.
In this example, we specify that ‘column1’ should be interpreted as float64 and ‘column2’ as string.
Other Considerations
When working with Excel files in Python, you might encounter other issues, such as dealing with missing data or understanding Excel’s zero-based indexing. It’s important to read the documentation of the libraries you’re using and understand the underlying concepts.
Remember, troubleshooting is a normal part of the development process. Don’t be discouraged by these challenges. With practice and patience, you’ll be able to handle Excel files in Python with ease.
Understanding Excel Files and Python
To effectively work with Excel files in Python, it’s important to understand their structure and how Python libraries interact with them.
Excel File Structure
An Excel file is a binary file that stores data in cells, which are organized into rows and columns. These cells can contain numbers, text, or formulas. The cells are grouped into worksheets, and one Excel file can contain multiple worksheets.
import pandas as pd
# Load an Excel file
file = pd.ExcelFile('file.xlsx')
# Get the names of all worksheets in the file
print(file.sheet_names)
# Output:
# Prints the names of all worksheets in 'file.xlsx'.
In this example, we use pandas
to load an Excel file and print the names of all worksheets in the file. This illustrates how an Excel file is structured and how Python can interact with it.
Interacting with Excel Files in Python
Python libraries like pandas
, openpyxl
, and xlrd
interact with Excel files by reading their binary data and converting it into a format that Python can understand. For example, pandas
reads an Excel file and stores its data in a DataFrame, a two-dimensional data structure with rows and columns that’s very similar to an Excel worksheet.
# Read an Excel file into a DataFrame
df = pd.read_excel('file.xlsx')
# Display the DataFrame
print(df)
# Output:
# Displays the content of 'file.xlsx' as a DataFrame.
In this code block, we read an Excel file into a DataFrame and print the DataFrame. This shows how Python can convert an Excel file into a format it can work with.
Underlying Concepts of the Pandas Library
At the core of pandas
is the DataFrame, a data structure that’s designed to handle tabular data, like the data in an Excel file. When pandas
reads an Excel file, it stores the data in a DataFrame. This allows you to manipulate the data using pandas
‘ powerful data manipulation functions.
For example, you can use pandas
to calculate the sum of a column, sort the data by a specific column, or filter the data based on certain criteria. Understanding these underlying concepts is key to mastering the handling of Excel files in Python.
Expanding Your Python-Excel Skills
Working with Excel files in Python is a valuable skill, but it’s just the beginning. The real power of Python comes from its vast ecosystem of libraries and its versatility in various fields, including data analysis, machine learning, and more.
Python and Data Analysis
Python’s ability to handle Excel files makes it a powerful tool for data analysis. With Python, you can automate the process of reading and cleaning data, perform complex calculations, and even create visualizations. For example, you could read an Excel file containing sales data, calculate the average sales per month, and then create a bar chart to visualize your results.
import pandas as pd
import matplotlib.pyplot as plt
df = pd.read_excel('sales_data.xlsx')
average_sales = df.groupby('Month')['Sales'].mean()
average_sales.plot(kind='bar')
plt.show()
# Output:
# Reads 'sales_data.xlsx', calculates the average sales per month, and displays a bar chart.
Python and Machine Learning
Python’s ability to handle Excel files also makes it a great tool for machine learning. You can use Python to read and clean your data, perform feature engineering, and then train and evaluate machine learning models. For example, you could read an Excel file containing customer data, engineer features based on the data, and then train a logistic regression model to predict customer churn.
Exploring Related Concepts
Beyond handling Excel files, Python offers a wide range of possibilities. You might want to explore related concepts like data visualization with libraries like Matplotlib and Seaborn, or data cleaning with libraries like NumPy and SciPy. Each of these areas adds another tool to your Python toolkit, allowing you to tackle more complex problems and create more powerful solutions.
Further Resources for Python-Excel Mastery
To deepen your understanding of handling Excel files in Python, consider exploring these external resources:
- Python JSON Handling Tips – Discover how to handle large JSON datasets efficiently in Python.
JSON Data Formatting in Python: Pretty Print with json.dumps() – Dive into the world of pretty-printing JSON for improved readability.
Excel Automation with openpyxl in Python – Discover how to leverage openpyxl for data extraction and reporting in Python.
Working with Excel Files in Python – This website is a comprehensive resource for working with Excel files in Python.
Automate the Boring Stuff with Python – This chapter from Al Sweigart’s book provides a practical guide to automating Excel tasks with Python.
Python for Data Analysis – This book by Wes McKinney, the creator of
pandas
, provides an in-depth look at using Python for data analysis.
Wrapping Up: Mastering Excel Files with Python
In this comprehensive guide, we’ve delved into the world of Python and Excel, exploring how to efficiently manipulate Excel files using Python. We’ve seen Python’s capabilities in handling Excel files, from basic reading and writing to more advanced operations, such as handling multiple worksheets and applying formulas.
We began with the basics, learning how to read and write Excel files using the pandas
library. We then ventured into more advanced territory, exploring complex Excel operations like dealing with multiple worksheets, applying formulas, and formatting cells.
Along the way, we tackled common issues you might encounter when working with Excel files in Python, such as handling large files and different data types, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handling Excel files in Python, comparing pandas
with other libraries like openpyxl
and xlrd
. Here’s a quick comparison of these libraries:
Library | Reading and Writing | Advanced Features | Speed | Memory Efficiency |
---|---|---|---|---|
pandas | Yes | Limited | Moderate | Low |
openpyxl | Yes | Yes | Slow | Moderate |
xlrd | Read Only | Limited | Fast | High |
Whether you’re just starting out with Python and Excel or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of Python’s capabilities in handling Excel files.
With its balance of simplicity, power, and versatility, Python is a formidable tool for manipulating Excel files. As you continue your journey with Python and Excel, remember to explore, experiment, and most importantly, have fun. Happy coding!