Python JSON Pretty Print | Guide (With Examples)
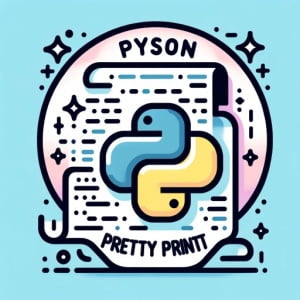
Ever found yourself wrestling with the task of reading JSON data in Python? You’re not alone. Many developers find the task daunting, but Python offers a solution that can turn this challenge into a walk in the park.
Just like a skilled formatter, Python has the ability to pretty print JSON data, transforming it into a format that’s easier to read and understand. This feature is a lifesaver when dealing with complex data structures.
In this guide, we’ll walk you through the process of pretty printing JSON in Python. We’ll start with the basics and gradually delve into more advanced techniques. We’ll also cover alternative approaches and discuss common issues you might encounter and how to solve them.
So, let’s dive in and start mastering Python JSON pretty print!
TL;DR: How Do I Pretty Print JSON in Python?
To pretty print JSON in Python, you can use the
json.dumps()
function with theindent
parameter, such asjson.dumps(data, indent=4)
. This function takes your JSON data and formats it in a more readable way.
Here’s a simple example:
import json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
pretty_json = json.dumps(data, indent=4)
print(pretty_json)
# Output:
# {
# 'name': 'John',
# 'age': 30,
# 'city': 'New York'
# }
In this example, we import the json module and create a dictionary. We then use the json.dumps()
function with the indent
parameter set to 4. This formats the JSON data with 4 spaces of indentation, making it easier to read. The result is then printed to the console.
This is just a basic example of pretty printing JSON in Python. There’s much more to learn about handling JSON data in Python, including dealing with more complex data structures and alternative methods for pretty printing. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Mastering Basic JSON Pretty Print in Python
- Dealing with Complex JSON Structures in Python
- Exploring Alternative Methods for Pretty Printing JSON
- Overcoming Challenges in JSON Pretty Printing
- Understanding Python’s JSON Module
- Exploring the Power of Pretty Printing JSON
- Wrapping Up: Mastering Python JSON Pretty Printing
Mastering Basic JSON Pretty Print in Python
To understand how to pretty print JSON in Python, we’ll first need to get familiar with the json.dumps()
function. This function is part of Python’s built-in json module, and it’s used to convert a Python object into a JSON string.
The json.dumps()
function takes two parameters: the data you want to convert, and the indent
parameter. The indent
parameter is optional, but it’s what allows us to pretty print the JSON data.
Let’s look at a simple example:
import json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
pretty_json = json.dumps(data, indent=4)
print(pretty_json)
# Output:
# {
# 'name': 'John',
# 'age': 30,
# 'city': 'New York'
# }
In the above code, we first import the json module. We then create a dictionary named data
, which we want to convert into a JSON string. We pass data
to the json.dumps()
function, along with the indent
parameter set to 4. This tells Python to format the JSON string with 4 spaces of indentation, making it much easier to read. Finally, we print the result to the console.
The json.dumps()
function is a powerful tool for handling JSON data in Python, but it’s not without its pitfalls. One thing to be aware of is that it only works with data that can be serialized into JSON. If you try to pass data that can’t be serialized, like a custom Python object, you’ll get a TypeError. We’ll discuss more about handling these types of issues later in the guide.
Dealing with Complex JSON Structures in Python
As you delve deeper into Python’s JSON handling capabilities, you’ll often encounter more complex data structures. These could include nested dictionaries and lists, which can further complicate the pretty printing process. But don’t worry, Python’s json.dumps()
function is more than capable of handling these scenarios.
Let’s look at an example of a nested dictionary:
import json
data = {
'employee': {
'name': 'John',
'age': 30,
'city': 'New York',
'skills': ['Python', 'Java', 'C++']
}
}
pretty_json = json.dumps(data, indent=4)
print(pretty_json)
# Output:
# {
# 'employee': {
# 'name': 'John',
# 'age': 30,
# 'city': 'New York',
# 'skills': [
# 'Python',
# 'Java',
# 'C++'
# ]
# }
# }
In this example, we have a dictionary within a dictionary, and a list inside the inner dictionary. When we use json.dumps()
with the indent
parameter, it neatly formats all the elements, including the nested dictionary and list. The output is a well-structured and readable JSON string.
By understanding how to handle complex data structures, you’ll be able to pretty print JSON data in Python more effectively. Remember, the key is to always ensure your data can be serialized into JSON. If you encounter data types that can’t be serialized, you’ll need to convert them into a format that can be, or use a custom JSON encoder. We’ll discuss more about this in the troubleshooting section.
Exploring Alternative Methods for Pretty Printing JSON
While json.dumps()
is a powerful function for pretty printing JSON in Python, it’s not the only tool at your disposal. There are other built-in modules and third-party libraries that can also get the job done. Let’s look at a couple of these alternatives.
Using the pprint
Module
Python’s built-in pprint
module is another great tool for pretty printing. It stands for ‘pretty printer’, and it can pretty print both JSON data and Python data structures.
Here’s how you can use it to pretty print JSON data:
import json
from pprint import pprint
data = {'name': 'John', 'age': 30, 'city': 'New York'}
pprint(data)
# Output:
# {'age': 30, 'city': 'New York', 'name': 'John'}
In this example, we import the pprint
function from the pprint
module and use it to pretty print our dictionary. The output is similar to json.dumps()
, but the pprint
function also sorts the keys in the dictionary, which can be useful in certain situations.
Using the simplejson
Library
simplejson
is a third-party library that can also pretty print JSON data. It’s similar to the built-in json module, but it offers more options for customization.
Here’s an example of how to use simplejson
to pretty print JSON data:
import simplejson as json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
pretty_json = json.dumps(data, indent=4, sort_keys=True)
print(pretty_json)
# Output:
# {
# 'age': 30,
# 'city': 'New York',
# 'name': 'John'
# }
In this example, we use simplejson
to pretty print our dictionary. We use the sort_keys
parameter to sort the keys in the dictionary, which is a feature not available in the built-in json module.
While these alternatives can be useful, they may not always be the best choice. The built-in json module is more than capable for most pretty printing tasks, and it doesn’t require any additional installations. However, if you need more customization options or specific features, these alternatives can be a good choice.
Overcoming Challenges in JSON Pretty Printing
As you work with Python’s JSON module, you may encounter certain challenges. One common issue is dealing with non-serializable data types. The json.dumps()
function can only serialize basic data types like lists, dictionaries, strings, numbers, booleans, and None
. If you try to serialize a data type that’s not serializable, you’ll get a TypeError.
Handling Non-Serializable Data Types
Let’s look at an example of what happens when you try to serialize a non-serializable data type:
import json
data = {'name': 'John', 'age': 30, 'city': 'New York', 'birthday': datetime.datetime(1990, 1, 1)}
pretty_json = json.dumps(data, indent=4)
# Output:
# TypeError: Object of type datetime is not JSON serializable
In this example, we’re trying to serialize a dictionary that contains a datetime object. When we run this code, we get a TypeError because datetime objects can’t be serialized into JSON.
So, how can we deal with this issue? One solution is to convert the non-serializable data into a serializable format before passing it to json.dumps()
. In the case of datetime objects, we can convert them into strings:
import json
import datetime
data = {
'name': 'John',
'age': 30,
'city': 'New York',
'birthday': datetime.datetime(1990, 1, 1).isoformat()
}
pretty_json = json.dumps(data, indent=4)
print(pretty_json)
# Output:
# {
# 'name': 'John',
# 'age': 30,
# 'city': 'New York',
# 'birthday': '1990-01-01T00:00:00'
# }
In this updated code, we use the isoformat()
method to convert the datetime object into an ISO formatted string. Now, when we pass the dictionary to json.dumps()
, it successfully pretty prints the JSON data.
Knowing how to troubleshoot and work around common issues like this is a crucial part of mastering Python’s JSON module. As you continue to explore and experiment, you’ll become more adept at handling these challenges.
Understanding Python’s JSON Module
Before we delve further into pretty printing JSON in Python, it’s crucial to understand the basics of Python’s JSON module and JSON data structures.
Python’s JSON Module
Python’s JSON module is a built-in module that allows you to work with JSON data. You can import it into your script with a simple import json
command. This module provides functions for serializing Python objects into JSON format (json.dumps()
), and deserializing JSON data into Python objects (json.loads()
).
Here’s an example of how you can use the json.dumps()
function to serialize a Python dictionary into a JSON string:
import json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
json_string = json.dumps(data)
print(json_string)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, we first import the json module. We then create a dictionary and pass it to json.dumps()
, which returns a JSON formatted string. We then print this string to the console.
JSON Data Structure
JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s easy for humans to read and write, and easy for machines to parse and generate. It’s based on a subset of the JavaScript Programming Language, but it’s language-independent, with parsers available for virtually every programming language.
JSON data is represented in key/value pairs, similar to Python dictionaries. Here’s an example of what JSON data looks like:
{
'name': 'John',
'age': 30,
'city': 'New York'
}
In this example, ‘name’, ‘age’, and ‘city’ are keys, and ‘John’, 30, and ‘New York’ are their respective values.
Understanding Python’s JSON module and the basics of JSON data structures is the foundation of pretty printing JSON in Python. With this knowledge, you’ll be better equipped to handle more complex scenarios and troubleshoot any issues you may encounter.
Exploring the Power of Pretty Printing JSON
Pretty printing JSON in Python is not just about making data look good. It’s an essential skill that has wide-ranging applications in various areas of programming and data analysis.
Pretty Printing in Data Analysis
In the world of data analysis, pretty printing JSON can be incredibly useful. JSON data is often used in data analysis due to its lightweight nature and ease of use. By pretty printing JSON data, you can make complex data structures easier to understand, which can be critical when analyzing large datasets.
Web Scraping and API Data
Web scraping is another area where pretty printing JSON is invaluable. Many modern websites use APIs that return JSON data. By pretty printing this data, you can better understand the structure of the data you’re dealing with, making it easier to extract the information you need.
Additionally, when working with APIs, you’ll often need to handle JSON data. APIs typically return data in JSON format, and pretty printing can help you understand the data you’re receiving.
File I/O and JSON
Python’s JSON module also allows you to read and write JSON data to files. This means you can pretty print JSON data to a file, making it easier to share and analyze. It’s also useful when you’re storing data for later use.
import json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
# Writing pretty printed JSON data to a file
with open('data.json', 'w') as f:
json.dump(data, f, indent=4)
In this example, we’re pretty printing JSON data and writing it to a file. We use the json.dump()
function, which is similar to json.dumps()
, but writes the data to a file instead of returning a string.
Further Resources for Mastering Python JSON
To deepen your understanding of pretty printing JSON in Python, consider exploring these additional resources:
- IOFlood’s Python JSON Guide explores Python’s support for working with JSON files and APIs.
Python XML Processing – holds techniques and examples on XML parsing and navigation in Python.
Excel Handling in Python – An overview on Python’s support for working with Excel files and data.
Python’s Official JSON Documentation – This is the official documentation for Python’s JSON module and its functions.
Real Python’s Guide to Working with JSON Data in Python provides anintroduction on JSON data in Python, including pretty printing.
Python for Beginners’ JSON Tutorial offers an introduction to JSON in Python, with plenty of examples and explanations.
By understanding the power of pretty printing JSON in Python, you’ll be well on your way to mastering Python’s JSON module and handling JSON data like a pro.
Wrapping Up: Mastering Python JSON Pretty Printing
In this comprehensive guide, we’ve unraveled the process of pretty printing JSON in Python. We’ve explored how Python’s built-in JSON module, specifically the json.dumps()
function, can transform JSON data into a more readable format.
We began with the basics, learning how to use json.dumps()
to pretty print simple JSON data. We then delved into more complex scenarios, handling nested dictionaries and lists. Along the way, we tackled common issues you might encounter, such as dealing with non-serializable data types, and provided solutions to help you overcome these challenges.
We also explored alternative methods for pretty printing JSON, including Python’s pprint
module and the third-party simplejson
library. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
json.dumps() | Powerful, handles complex data structures | Only works with serializable data |
pprint | Built-in, sorts keys | Less options for customization |
simplejson | More customization options, sorts keys | Requires additional installation |
Whether you’re just starting out with Python JSON pretty printing or you’re looking to level up your skills, we hope this guide has provided you with a deeper understanding of the topic and its applications.
Python JSON pretty printing is a powerful tool for data analysis, web scraping, and working with APIs. Now, you’re well equipped to handle JSON data in Python like a pro. Happy coding!