Python Sort Dictionary by Value | Handling Data Structures
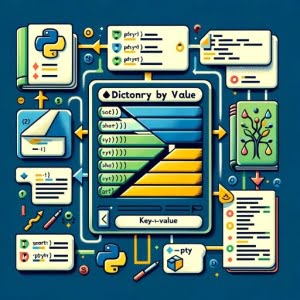
Sorting a list of dictionaries by value in Python is a common task we perform to organize complex data structures at IOFLOOD. Today’s article will explore how to sort dict by value in Python, providing practical examples to assist our bare metal hosting customers in managing their data more effectively.
This guide will show you valuable Python sort dictionary by value techniques. We’ll start with the basics and gradually move to more advanced methods. We’ll cover everything from the sorted()
function, the itemgetter()
function, and even delve into alternative approaches.
Let’s get started!
TL;DR: How Do I Sort Dictionary by Value in Python?
To sort a dictionary by value in Python, you can use the
sorted()
function in combination with theitemgetter()
function from theoperator
module, such as with the commandsorted_dict = dict(sorted(my_dict.items(), key=operator.itemgetter(1)))
.
Here’s a simple example:
import operator
my_dict = {'a': 2, 'b': 1, 'c': 3}
sorted_dict = dict(sorted(my_dict.items(), key=operator.itemgetter(1)))
print(sorted_dict)
# Output:
# {'b': 1, 'a': 2, 'c': 3}
In this example, we first import the operator
module. Then, we create a dictionary my_dict
with keys ‘a’, ‘b’, and ‘c’ and corresponding values 2, 1, and 3. We then use the sorted()
function in combination with operator.itemgetter(1)
to sort the dictionary items by value. The sorted items are then converted back into a dictionary using the dict()
function. The result is a new dictionary sorted_dict
where the items are sorted by value in ascending order.
This is a basic way to sort a dictionary by value in Python, but there’s much more to learn about sorting dictionaries in Python. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
Intro: Python Sort Dictionary by Value
In Python, the sorted()
function and the itemgetter()
function from the operator
module are your go-to tools for sorting a dictionary by value. Let’s break it down:
import operator
my_dict = {'a': 2, 'b': 1, 'c': 3}
sorted_dict = dict(sorted(my_dict.items(), key=operator.itemgetter(1)))
print(sorted_dict)
# Output:
# {'b': 1, 'a': 2, 'c': 3}
In this example, the sorted()
function is used to sort the items (key-value pairs) of the dictionary. The key
parameter of the sorted()
function is set to operator.itemgetter(1)
, which means that the sorting is done based on the dictionary values (since in a key-value pair, the value is at index 1).
Understanding the sorted()
Function
The sorted()
function returns a new sorted list from the elements of any sequence. In our case, the sequence is my_dict.items()
, which is a sequence of the dictionary’s key-value pairs.
The Role of operator.itemgetter()
The operator.itemgetter()
function returns a callable object that fetches the given item(s) from its operand. When multiple items are specified, itemgetter()
returns a tuple of looked-up values. For example, operator.itemgetter(1)
gets the value at index 1 from its operand.
Advantages and Potential Pitfalls
One of the main advantages of this method is its readability and simplicity. However, it’s worth noting that this method creates a new sorted dictionary, leaving the original dictionary unchanged. This might not be what you want if you’re dealing with a large dictionary and memory usage is a concern.
Also, this method sorts the dictionary in ascending order by default. If you want to sort the dictionary in descending order, you’ll need to use the reverse=True
parameter of the sorted()
function.
Special Cases: Sort Dict by Value
While the basic use of sorted()
and operator.itemgetter()
is straightforward, there are more complex scenarios that you might encounter when sorting dictionaries by value in Python. Let’s explore a couple of these scenarios.
Sorting a Dictionary with Multiple Values Per Key
What if your dictionary has multiple values per key and you want to sort by one of these values? Here’s how you can do it:
import operator
my_dict = {'a': [2, 4], 'b': [1, 3], 'c': [3, 2]}
sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1][0]))
print(sorted_dict)
# Output:
# {'b': [1, 3], 'a': [2, 4], 'c': [3, 2]}
In this case, we’re using a lambda function as the key for the sorted()
function. The lambda function takes an item from the dictionary and returns the first element of the value list (item[1][0]
), which is used for sorting.
Sorting a Dictionary in Reverse Order
To sort a dictionary by value in reverse order (i.e., descending order), you can use the reverse=True
parameter of the sorted()
function. Here’s how:
import operator
my_dict = {'a': 2, 'b': 1, 'c': 3}
sorted_dict = dict(sorted(my_dict.items(), key=operator.itemgetter(1), reverse=True))
print(sorted_dict)
# Output:
# {'c': 3, 'a': 2, 'b': 1}
In this example, the reverse=True
parameter tells the sorted()
function to sort in descending order. The dictionary is sorted by value, but in reverse order, with the highest value first.
Other Methods: Python Sort Dictionary
While sorted()
and operator.itemgetter()
are commonly used to sort dictionaries by value, Python provides other ways to achieve the same result. One such alternative is using lambda functions. Let’s explore this approach.
Using Lambda Functions to Sort Dictionary by Value
Lambda functions in Python are anonymous functions that can have any number of arguments but only one expression. They can be used as the key function while sorting dictionaries. Here’s how you can use a lambda function to sort a dictionary by value:
my_dict = {'a': 2, 'b': 1, 'c': 3}
sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1]))
print(sorted_dict)
# Output:
# {'b': 1, 'a': 2, 'c': 3}
In this example, we use a lambda function that takes an item from the dictionary (a key-value pair) and returns the value (item[1]
). This value is then used for sorting.
Comparing Different Methods
Both operator.itemgetter()
and lambda functions can be used to sort a dictionary by value effectively. The choice between the two often comes down to personal preference and the specific requirements of your code. Here are a few considerations:
- Readability: Some developers find that
operator.itemgetter()
makes the code more readable and explicit, while others prefer the conciseness of lambda functions. Flexibility: Lambda functions can be more flexible than
operator.itemgetter()
. For example, you can easily modify the lambda function in the above example to sort by the absolute value, which would be more complex withoperator.itemgetter()
.
In the end, the best method to sort a dictionary by value in Python depends on your specific needs and coding style.
Troubleshooting Python Sort Dict
While sorting dictionaries by value in Python is a straightforward process, you might encounter some common issues. Let’s tackle these potential problems and provide solutions.
Dealing with Non-Comparable Values
One common issue is trying to sort a dictionary that contains non-comparable values. For instance, if your dictionary contains both string and integer values, attempting to sort it by value will result in a TypeError.
my_dict = {'a': 2, 'b': '1', 'c': 3}
try:
sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1]))
except TypeError as e:
print(f'Error: {e}')
# Output:
# Error: '<' not supported between instances of 'str' and 'int'
In the above example, trying to compare an integer and a string results in a TypeError. One way to resolve this is to ensure all values in the dictionary are of the same type before sorting.
Tips for Sort Dictionary by Value
- Ensure Comparable Values: Make sure all values in the dictionary are of the same, comparable type before attempting to sort.
Use Appropriate Sorting Method: Depending on your specific needs and the complexity of your dictionary, choose the sorting method that best suits your situation. Both
operator.itemgetter()
and lambda functions have their advantages.Consider Memory Usage: Sorting a dictionary by value creates a new dictionary and leaves the original unchanged. If you’re dealing with a large dictionary and memory usage is a concern, consider alternative approaches.
Understanding Dictionary Data Type
Python’s dictionary is an incredibly useful data type that allows you to store and organize data in a unique way. Before we delve further into sorting these dictionaries, let’s take a moment to understand the fundamentals of this data type.
What is a Dictionary in Python?
In Python, a dictionary is an unordered collection of items. Each item stored in a dictionary has a key and a value, making it a key-value pair. Here’s a simple dictionary:
my_dict = {'a': 1, 'b': 2, 'c': 3}
print(my_dict)
# Output:
# {'a': 1, 'b': 2, 'c': 3}
In this dictionary, ‘a’, ‘b’, and ‘c’ are keys, and 1, 2, and 3 are their respective values.
Keys, Values, and Items
In a dictionary, keys are unique identifiers where we store our values. The value can be of any type (numbers, strings, lists, etc.) and can even change over time. The keys, however, must be of an immutable type (like numbers, strings, or tuples) and should be unique within a dictionary.
The keys()
, values()
, and items()
methods allow you to access the keys, values, and key-value pairs (items) of a dictionary, respectively.
my_dict = {'a': 1, 'b': 2, 'c': 3}
print(my_dict.keys())
print(my_dict.values())
print(my_dict.items())
# Output:
# dict_keys(['a', 'b', 'c'])
# dict_values([1, 2, 3])
# dict_items([('a', 1), ('b', 2), ('c', 3)])
Fundamentals of Python Dictionary Sorting
Sorting is a basic concept in programming that involves arranging data in a particular format. In the context of dictionaries, sorting can be done either by keys or values. As our focus is to sort dictionaries by value, we’ll use various dictionary methods like sorted()
and itemgetter()
, as well as alternative approaches like lambda functions.
Understanding the fundamentals of dictionaries and sorting is crucial to effectively sort dictionaries by value in Python. With this knowledge, we can now proceed to more advanced topics and practical examples.
Why Sort Dictionary by Value
Sorting dictionaries by value in Python is not just an academic exercise. It has practical applications in various fields, such as data analysis and machine learning. By sorting dictionaries by value, you can prioritize data, analyze frequency, and make more sense of large datasets.
Python Sorting Dictionary in Data Analysis
In data analysis, you often need to sort data to understand it better. For example, if you have a dictionary where keys represent different categories of items and values represent their frequency, sorting this dictionary by value can quickly show you the most and least frequent categories.
Python Sort Dict in Machine Learning
In machine learning, dictionaries can be used to hold feature vectors, where the keys are the features and the values are the feature values. Sorting this dictionary by value can help prioritize features.
Exploring Related Concepts
As you continue your Python journey, consider exploring related concepts like list comprehension and lambda functions. List comprehension is a concise way to create lists in Python, while lambda functions can simplify your code and make it more efficient.
Further Resources for Python Dictionary
To deepen your understanding of Python dictionaries and related concepts, here are some resources you might find helpful:
- Navigating Python Dictionaries – A Step-by-Step Tutorial – Dive into the world of Python dictionaries and unlock their potential for efficient data storage and retrieval.
Navigating Complexity with Python Nested Dictionaries – IOFlood’s Guide to Python nested dictionaries. Learn how to work with complex data structures.
Exploring Python Dictionary Methods – A Practical Tutorial – Explore Python dictionary methods for adding, updating, and deleting key-value pairs.
Official Documentation on Dictionaries – This is Python’s official guide for dictionaries, providing an in-depth explanation of their structure and methods.
Python Guide on Dictionaries – An extensive guide from Real Python, detailing the use, manipulation, and functionality of Python dictionaries.
Beginners’ Tutorial on Dictionaries – This beginner-friendly tutorial explains the concept of dictionaries in Python with simple language and practical examples.
These resources provide detailed explanations and examples that can help you become more proficient in working with dictionaries in Python.
Recap: Python Sort Dict by Value
In this comprehensive guide, we’ve navigated through the process of sorting dictionaries by value in Python. We’ve covered everything from the basic use of the sorted()
function and operator.itemgetter()
, to more advanced scenarios and alternative methods using lambda functions.
We began with the basics, learning how to sort a dictionary by value using sorted()
and operator.itemgetter()
. We then delved into more complex scenarios, such as sorting dictionaries with multiple values per key and sorting in reverse order. We also explored alternative approaches using lambda functions, providing you with more flexibility depending on your specific needs.
Along the way, we tackled common issues that you might encounter when sorting dictionaries by value, such as dealing with non-comparable values, and provided solutions to help you overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Readability | Flexibility | Memory Efficiency |
---|---|---|---|
sorted() with operator.itemgetter() | High | Moderate | Depends on the size of the dictionary |
Lambda Functions | Moderate | High | Depends on the size of the dictionary |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your Python skills, we hope this guide has given you a deeper understanding of how to sort dictionaries by value in Python. With these tools and knowledge, you’re well-equipped to handle any dictionary sorting task that comes your way. Happy coding!