Python Dictionary Methods: A Complete Usage Guide
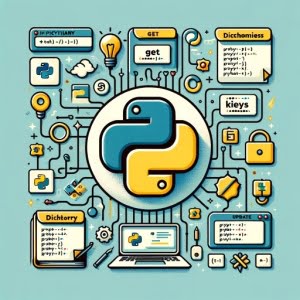
Are you finding it challenging to work with Python dictionary methods? You’re not alone. Many developers find themselves puzzled when it comes to understanding and using these methods effectively. But, think of Python’s dictionary methods as a Swiss army knife – versatile and handy for various tasks.
Whether you’re manipulating data, creating dynamic mappings, or even debugging, understanding how to use Python dictionary methods can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using Python dictionary methods, from the basics to more advanced techniques. We’ll cover everything from the get()
, keys()
, values()
, items()
, update()
, to pop()
methods, as well as alternative approaches.
Let’s get started!
TL;DR: What Are the Main Methods for Python Dictionaries?
Python dictionaries have several methods such as
get()
,keys()
,values()
,items()
,update()
, andpop()
. These methods allow you to access, modify, and manipulate data in your dictionaries in various ways.
Here’s a simple example of using some of these methods:
my_dict = {'name': 'John', 'age': 30}
print(my_dict.keys())
print(my_dict.values())
print(my_dict.get('name'))
# Output:
# dict_keys(['name', 'age'])
# dict_values(['John', 30])
# 'John'
In this example, we create a dictionary my_dict
with keys ‘name’ and ‘age’. We then use the keys()
method to get a list of all keys, the values()
method to get a list of all values, and the get()
method to access the value of a specific key.
This is just a basic introduction to Python dictionary methods. There’s much more to learn about these methods, including more advanced techniques and alternative approaches. Continue reading for a more comprehensive guide.
Table of Contents
- Discovering Basic Python Dictionary Methods
- Exploring Advanced Python Dictionary Methods
- Alternative Techniques for Python Dictionary Manipulation
- Troubleshooting Python Dictionary Methods
- Unpacking the Python Dictionary
- The Impact of Python Dictionary Methods on Different Applications
- Wrapping Up: Mastering Python Dictionary Methods
Discovering Basic Python Dictionary Methods
Python dictionaries come with a set of built-in methods that make it easier to work with them. Let’s start with the basic ones: get()
, keys()
, values()
, and items()
.
The get()
Method
The get()
method allows you to retrieve the value of a specific key from the dictionary. It’s a safer way to access a key-value pair because it will not result in an error if the key does not exist.
Here’s how you can use it:
my_dict = {'name': 'John', 'age': 30}
print(my_dict.get('name'))
print(my_dict.get('address', 'Not Found'))
# Output:
# 'John'
# 'Not Found'
In this example, the get()
method returns ‘John’ when we try to access the key ‘name’. When we try to access ‘address’, which does not exist in the dictionary, it returns ‘Not Found’ instead of throwing an error.
The keys()
and values()
Methods
The keys()
and values()
methods return a list of all the keys and values in the dictionary, respectively.
Let’s see them in action:
my_dict = {'name': 'John', 'age': 30}
print(my_dict.keys())
print(my_dict.values())
# Output:
# dict_keys(['name', 'age'])
# dict_values(['John', 30])
In this code, keys()
returns a list of all keys in my_dict
, and values()
returns a list of all values.
The items()
Method
The items()
method is used to return a list of all key-value pairs in the dictionary.
Here’s an example:
my_dict = {'name': 'John', 'age': 30}
print(my_dict.items())
# Output:
# dict_items([('name', 'John'), ('age', 30)])
In this example, items()
returns a list of tuples, where each tuple contains a key-value pair from the dictionary.
These basic methods are the building blocks for working with Python dictionaries. Mastering them will help you manipulate and access data in your dictionaries more efficiently.
Exploring Advanced Python Dictionary Methods
Once you’re comfortable with the basic Python dictionary methods, it’s time to move on to some of the more advanced techniques. We’ll focus on update()
, pop()
, setdefault()
, and popitem()
methods.
The update()
Method
The update()
method allows you to add new key-value pairs to a dictionary or modify existing ones.
Here’s an example:
my_dict = {'name': 'John', 'age': 30}
my_dict.update({'age': 31, 'address': '123 Street'})
print(my_dict)
# Output:
# {'name': 'John', 'age': 31, 'address': '123 Street'}
In this example, we use update()
to change the value of ‘age’ and add a new key-value pair ‘address’.
The pop()
Method
The pop()
method is used to remove a key-value pair from a dictionary. It requires the key of the pair you want to remove as an argument and returns the value of the removed pair.
Here’s how it works:
my_dict = {'name': 'John', 'age': 30, 'address': '123 Street'}
removed_value = my_dict.pop('address')
print(removed_value)
print(my_dict)
# Output:
# '123 Street'
# {'name': 'John', 'age': 30}
In this example, pop()
removes the ‘address’ key-value pair from the dictionary and returns the value ‘123 Street’.
The setdefault()
Method
The setdefault()
method is a safer way to add new key-value pairs to a dictionary. It only adds the pair if the key does not already exist in the dictionary.
Here’s an example:
my_dict = {'name': 'John', 'age': 30}
my_dict.setdefault('address', '123 Street')
print(my_dict)
# Output:
# {'name': 'John', 'age': 30, 'address': '123 Street'}
In this example, setdefault()
adds the ‘address’ key-value pair to the dictionary because ‘address’ does not exist in my_dict
.
The popitem()
Method
The popitem()
method removes and returns the last key-value pair added to the dictionary.
Here’s how you can use it:
my_dict = {'name': 'John', 'age': 30, 'address': '123 Street'}
removed_pair = my_dict.popitem()
print(removed_pair)
print(my_dict)
# Output:
# ('address', '123 Street')
# {'name': 'John', 'age': 30}
In this example, popitem()
removes the last added key-value pair (‘address’, ‘123 Street’) and returns it.
These advanced methods provide more control over how you can manipulate Python dictionaries. Understanding how to use them effectively can significantly improve your coding efficiency.
Alternative Techniques for Python Dictionary Manipulation
Python offers a variety of alternative techniques for manipulating dictionaries. These include comprehensions, the dict()
constructor, and using third-party libraries. Understanding these methods can provide you with more tools to tackle complex problems.
Dictionary Comprehensions
Dictionary comprehensions provide a concise way to create dictionaries. They are similar to list comprehensions, but with key-value pairs instead of single values.
Here’s an example:
squares = {x: x**2 for x in range(6)}
print(squares)
# Output:
# {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
In this example, we use a dictionary comprehension to create a dictionary where the keys are numbers from 0 to 5 and the values are their corresponding squares.
The dict()
Constructor
The dict()
constructor allows you to create dictionaries in a different way. It can be useful when you need to create a dictionary from sequences of elements.
Here’s how you can use it:
my_dict = dict(name='John', age=30)
print(my_dict)
# Output:
# {'name': 'John', 'age': 30}
In this example, we use the dict()
constructor to create a dictionary with ‘name’ and ‘age’ as keys.
Third-Party Libraries
Python’s vast ecosystem includes several third-party libraries that provide additional methods to work with dictionaries. Libraries like pandas
and numpy
can be particularly useful when working with large datasets.
Here’s an example of using pandas
to create a DataFrame from a dictionary:
import pandas as pd
my_dict = {'name': ['John', 'Jane'], 'age': [30, 25]}
df = pd.DataFrame(my_dict)
print(df)
# Output:
# name age
# 0 John 30
# 1 Jane 25
In this example, we use the pandas
library to create a DataFrame from a dictionary. This allows us to manipulate and analyze the data in a tabular format.
These alternative approaches provide additional flexibility when working with dictionaries. Depending on your specific use case, one method may be more suitable than others. It’s worthwhile to experiment with these techniques to find the one that works best for you.
Troubleshooting Python Dictionary Methods
As with any coding process, you may encounter some issues when working with Python dictionary methods. Let’s discuss some of the common problems and their solutions.
Understanding KeyError
A KeyError is a common issue that occurs when you try to access a key that does not exist in the dictionary. Here’s an example:
my_dict = {'name': 'John', 'age': 30}
print(my_dict['address'])
# Output:
# KeyError: 'address'
In this example, we try to access the ‘address’ key, which does not exist in the dictionary, resulting in a KeyError. To avoid this, you can use the get()
method, which returns None or a default value if the key does not exist.
Dealing with TypeError
A TypeError can occur if you try to use an unhashable object as a dictionary key. In Python, only immutable objects (like strings, numbers, or tuples) can be used as dictionary keys.
Here’s an example of a TypeError:
my_dict = {['name']: 'John'}
# Output:
# TypeError: unhashable type: 'list'
In this example, we try to use a list as a dictionary key, which results in a TypeError. To avoid this, make sure to use only immutable objects as dictionary keys.
Other Considerations
While working with Python dictionary methods, it’s also essential to consider the size of the dictionary and the efficiency of different methods. For example, if you’re working with a large dictionary, using the keys()
or values()
methods can consume a lot of memory as they return a new list of keys or values. In such cases, using the iterkeys()
or itervalues()
methods can be more efficient as they return an iterator.
Understanding these common issues and their solutions can help you work with Python dictionary methods more effectively. Remember, the key to mastering Python dictionaries is practice and experimentation.
Unpacking the Python Dictionary
Before we delve deeper into Python dictionary methods, let’s take a step back and understand what Python dictionaries are and why they are useful.
Understanding Python Dictionaries
A Python dictionary is a built-in data type that stores data in key-value pairs. It’s an example of a data structure known as a hash table. Unlike other data types like lists or tuples, which store a single value at each index, dictionaries allow us to store a value against a unique key. This makes dictionaries incredibly useful for tasks where you need to associate values with unique identifiers.
Here’s an example of a Python dictionary:
my_dict = {'name': 'John', 'age': 30}
print(my_dict)
# Output:
# {'name': 'John', 'age': 30}
In this example, ‘name’ and ‘age’ are keys, and ‘John’ and 30 are their corresponding values.
The Role of Hash Tables
Under the hood, Python dictionaries use a concept known as hashing to store and retrieve data. When you insert a key-value pair into a dictionary, Python uses a hash function to convert the key into a hash code, which is then used as the index to store the corresponding value. This allows Python dictionaries to retrieve values for a given key in constant time, regardless of the size of the dictionary.
Keys and Values
The keys in a Python dictionary are unique and immutable, meaning each key can appear only once and cannot be changed after it’s created. On the other hand, values can be of any type and can be modified. You can use the keys()
method to get a list of all keys and the values()
method to get a list of all values in the dictionary.
my_dict = {'name': 'John', 'age': 30}
print(my_dict.keys())
print(my_dict.values())
# Output:
# dict_keys(['name', 'age'])
# dict_values(['John', 30])
In this example, keys()
returns a list of all keys in the dictionary, and values()
returns a list of all values.
Understanding these fundamentals of Python dictionaries is crucial to grasp the various dictionary methods and their uses effectively.
The Impact of Python Dictionary Methods on Different Applications
Python dictionary methods are not just limited to solving specific coding problems. They also play a crucial role in various applications such as data analysis, web development, and more.
Python Dictionary Methods in Data Analysis
In data analysis, Python dictionaries are often used to store and manipulate data. The get()
, keys()
, values()
, and items()
methods are particularly useful for accessing and analyzing data. For example, you can use the get()
method to retrieve specific data points for analysis, or the keys()
and values()
methods to perform operations on all data points.
Python Dictionary Methods in Web Development
In web development, Python dictionaries are commonly used to handle data sent and received in web requests. For instance, when you send a POST request to a web server, the data is typically sent as a dictionary. The update()
, pop()
, and setdefault()
methods can be used to manipulate this data as needed.
Exploring Related Concepts
While Python dictionary methods are powerful, they are just one part of the larger Python ecosystem. To become a proficient Python developer, it’s important to explore related concepts like sets, lists, and tuples. These data structures have their own set of methods and use-cases, and understanding them can help you write more efficient and effective code.
Further Resources for Mastering Python Dictionary Methods
To continue your journey towards mastering Python dictionary methods, here are some resources that provide in-depth tutorials and exercises:
- Python Dictionary – Your Complete Guide – Explore the power of Python dictionaries with this comprehensive guide, covering examples, syntax, and advanced uses.
IOFlood’s Python DefaultDict Guide – Handling Missing Keys with Ease – Learn how to use Python’s defaultdict for handling missing keys.
Python Dictionary Sorting Techniques – Sorting by Values – Dive into Python’s techniques for sorting dictionaries by values.
Official Documentation on Dictionaries – This is the official Python documentation on dictionaries.
Deep Dive Into Python Dictionaries – This comprehensive guide from Real Python dives deeply into Python dictionaries. It covers all aspects of dictionaries including their definition, methods, and usage scenarios.
Python Dictionary Methods – This article from Geeks for Geeks provides a detailed exploration of Python dictionary methods.
These resources provide a wealth of information on Python dictionaries and their methods, and can help you deepen your understanding of this essential Python feature.
Wrapping Up: Mastering Python Dictionary Methods
In this comprehensive guide, we’ve journeyed through the world of Python dictionary methods, unlocking the power of this essential tool in the Python ecosystem.
We began with the basics, exploring fundamental methods like get()
, keys()
, values()
, and items()
. We then ventured into more advanced territory, delving into methods like update()
, pop()
, setdefault()
, and popitem()
. We also looked at alternative techniques for manipulating dictionaries, such as dictionary comprehensions, the dict()
constructor, and third-party libraries.
Along the way, we tackled common challenges you might encounter when using Python dictionary methods, such as KeyError and TypeError, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Advantage |
---|---|---|
get() | Accessing values | Avoids KeyError for non-existent keys |
update() | Modifying dictionaries | Can add new key-value pairs or modify existing ones |
pop() | Removing key-value pairs | Removes a pair and returns the value |
Dictionary Comprehensions | Creating dictionaries | Provides a concise way to create dictionaries |
dict() Constructor | Creating dictionaries | Useful for creating dictionaries from sequences |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your Python dictionary skills, we hope this guide has given you a deeper understanding of Python dictionary methods and their capabilities.
Armed with this knowledge, you’re now better equipped to manipulate and access data in Python dictionaries. Happy coding!