Learn np.where | Numpy where() Function Guide
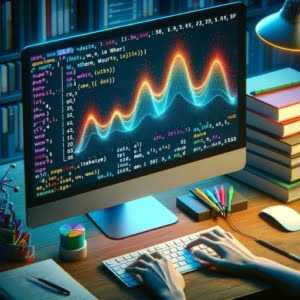
Ever wondered how to efficiently locate the indices of elements in a NumPy array that satisfy a certain condition? Just like a treasure hunter, np.where can help you find what you’re looking for.
This guide will walk you through the ins and outs of the np.where function in Python’s NumPy library. We’ll start with the basics, then dive into more complex uses, and even explore some alternatives. Along the way, we’ll provide plenty of code examples to help you grasp the concepts.
So whether you’re a beginner just starting out with NumPy, or an experienced data scientist looking to brush up on your skills, this guide has something for you. Let’s dive in!
TL;DR: How Do I Use np.where in Python’s NumPy Library?
The np.where function is used to return the indices of elements in an input array where the given condition is true. Let’s consider a simple example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(np.where(arr > 3))
# Output:
# (array([3, 4]),)
In this example, np.where is used to find the indices of elements that are greater than 3 in the array ‘arr’. The function returns a tuple of arrays, one for each dimension of ‘arr’, containing the indices of the true elements. In this case, the elements at indices 3 and 4 are greater than 3, hence the output (array([3, 4]),)
.
Dive deeper into this guide for more detailed explanations, advanced usage scenarios, and alternative approaches to using np.where in Python’s NumPy library.
Table of Contents
- Understanding np.where: A Beginner’s Guide
- Exploring np.where with Multidimensional Arrays
- Replacing Values with np.where
- Alternative Methods to np.where
- Troubleshooting Common np.where Issues
- NumPy Arrays: The Building Blocks
- np.where: A Key Player in Data Analysis and Machine Learning
- Exploring Related Concepts
- Wrapping Up: The Power of np.where
Understanding np.where: A Beginner’s Guide
The np.where function is a versatile tool in the NumPy library. It’s primarily used to find the indices of elements in an array where a specific condition holds true. Let’s break down how it works with a simple example.
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = np.where(arr > 3)
print(result)
# Output:
# (array([3, 4]),)
In this example, we first import the NumPy library. Then, we create a NumPy array named ‘arr’. We use np.where to find the indices of elements in ‘arr’ that are greater than 3. The np.where function returns a tuple of arrays, one for each dimension of ‘arr’, containing the indices of the true elements. Here, the elements at indices 3 and 4 are greater than 3, hence the output (array([3, 4]),)
.
This basic use of np.where is straightforward, but it’s also powerful. By specifying different conditions, you can use np.where to find the indices of all kinds of elements in your arrays. However, it’s important to note that np.where operates element-wise, which means it checks each element in the array individually against the condition. This can be a pitfall if you’re not expecting it, but it’s also what allows np.where to be so flexible.
Exploring np.where with Multidimensional Arrays
The np.where function is not limited to one-dimensional arrays. It can be used with multidimensional arrays as well. Let’s explore how np.where works with a two-dimensional array.
import numpy as np
arr2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
result = np.where(arr2d > 5)
print(result)
# Output:
# (array([1, 2, 2, 2]), array([2, 0, 1, 2]))
In this example, we create a two-dimensional array ‘arr2d’. We then use np.where to find the indices of elements in ‘arr2d’ that are greater than 5. The function returns a tuple of arrays, each array representing the indices along a dimension of ‘arr2d’. The first array in the tuple represents the row indices, and the second array represents the column indices. So, the elements at positions (1,2), (2,0), (2,1), and (2,2) in ‘arr2d’ are greater than 5.
Replacing Values with np.where
Another powerful feature of np.where is its ability to replace values in an array based on a condition. Here’s how you can do it:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = np.where(arr > 3, 'Greater than 3', 'Not greater than 3')
print(result)
# Output:
# array(['Not greater than 3', 'Not greater than 3', 'Not greater than 3', 'Greater than 3', 'Greater than 3'], dtype='<U16')
In this example, np.where is used with three arguments: the condition, the value to use for elements where the condition is true, and the value to use for elements where the condition is false. The result is a new array where each element is either ‘Greater than 3’ or ‘Not greater than 3’ depending on whether the corresponding element in ‘arr’ is greater than 3. This is particularly useful when you want to categorize or label data based on certain conditions.
Alternative Methods to np.where
While np.where is a powerful function, there are other ways to achieve similar results in Python’s NumPy library. Let’s explore two of these alternatives: Boolean indexing and the np.nonzero function.
Boolean Indexing
Boolean indexing is a type of indexing that allows you to select elements from an array using conditions. Let’s take a look at how we can use Boolean indexing as an alternative to np.where.
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = arr[arr > 3]
print(result)
# Output:
# array([4, 5])
In this example, we use Boolean indexing to select elements from ‘arr’ that are greater than 3. The expression arr > 3
returns a Boolean array of the same shape as ‘arr’, where each element indicates whether the corresponding element in ‘arr’ is greater than 3. This Boolean array is then used to index ‘arr’, resulting in an array of elements from ‘arr’ that are greater than 3.
While Boolean indexing is a powerful tool, it’s important to note that it returns the elements themselves rather than their indices. This can be an advantage or a disadvantage depending on your specific use case.
Using np.nonzero
Another alternative to np.where is the np.nonzero function, which returns the indices of the non-zero elements in the input array. Here’s how you can use np.nonzero to find the indices of elements that are greater than 3.
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = np.nonzero(arr > 3)
print(result)
# Output:
# (array([3, 4]),)
In this example, the expression arr > 3
returns a Boolean array where true represents elements in ‘arr’ that are greater than 3. np.nonzero then returns the indices of the true elements in this Boolean array, which are the indices of elements in ‘arr’ that are greater than 3.
The np.nonzero function is similar to np.where in many ways, but there’s one key difference: np.nonzero only takes one argument, the input array, while np.where can take up to three arguments, allowing it to replace values based on a condition. This makes np.where more flexible than np.nonzero, but np.nonzero can be a simpler and more efficient choice when you only need to find the indices of non-zero or true elements.
Troubleshooting Common np.where Issues
While np.where is a highly versatile function, you might encounter some issues when using it. Let’s explore some of these common problems and their solutions.
Dealing with NaN Values
One common issue when working with np.where is dealing with NaN (Not a Number) values. Let’s look at a code example:
import numpy as np
arr = np.array([1, 2, np.nan, 4, 5])
result = np.where(arr > 3)
print(result)
# Output:
# (array([2, 3, 4]),)
In this example, the np.where function considers the NaN value as being greater than 3. This might not be the desired behavior in many cases. One way to handle this is by using the np.isnan function to exclude NaN values:
import numpy as np
arr = np.array([1, 2, np.nan, 4, 5])
result = np.where((~np.isnan(arr)) & (arr > 3))
print(result)
# Output:
# (array([3, 4]),)
In this revised example, we use ~np.isnan(arr)
to create a Boolean array that is true where ‘arr’ is not NaN. This is combined with arr > 3
using the &
operator to create a condition that is true where ‘arr’ is not NaN and is greater than 3.
Handling Complex Conditions
Another potential issue with np.where is handling complex conditions. For example, you might want to find the indices of elements that satisfy more than one condition. Let’s consider an example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = np.where((arr > 2) & (arr < 5))
print(result)
# Output:
# (array([2, 3]),)
In this example, we use np.where with a complex condition to find the indices of elements in ‘arr’ that are greater than 2 and less than 5. The &
operator is used to combine the two conditions into one. This allows us to use np.where with complex conditions, making it an even more powerful tool for array manipulation.
NumPy Arrays: The Building Blocks
To fully understand the power of np.where, it’s essential to grasp the basics of NumPy arrays. NumPy arrays are multi-dimensional array objects that provide a fast and efficient way to store and manipulate data in Python.
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr)
# Output:
# array([1, 2, 3, 4, 5])
In this example, we create a one-dimensional NumPy array. Each item in the array is an element, and the position of an element in the array is its index. NumPy arrays can be multi-dimensional, meaning they can have more than one index. For example, a two-dimensional array has two indices: the first representing the row and the second representing the column.
Boolean Arrays and Indexing
A key concept to understanding np.where is Boolean arrays and indexing. A Boolean array is a NumPy array with the data type ‘bool’, meaning it contains true and false values. Boolean indexing allows you to select elements from an array using a Boolean array of the same shape.
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
bool_arr = arr > 3
print(bool_arr)
# Output:
# array([False, False, False, True, True])
In this example, we create a Boolean array ‘bool_arr’ by using a condition on ‘arr’. The condition arr > 3
is true where the elements in ‘arr’ are greater than 3 and false otherwise. This results in a Boolean array of the same shape as ‘arr’ where each element indicates whether the corresponding element in ‘arr’ is greater than 3.
Understanding these fundamentals of NumPy arrays, Boolean arrays, and indexing is crucial to mastering np.where and other array manipulation functions in Python’s NumPy library.
np.where: A Key Player in Data Analysis and Machine Learning
The np.where function is not just a handy tool for array manipulation. It plays a crucial role in fields like data analysis and machine learning, where handling and processing large amounts of data efficiently is key.
For instance, in data analysis, you might need to categorize or label data based on certain conditions. The np.where function, with its ability to replace values in an array based on a condition, is perfect for this task.
In machine learning, you might need to preprocess your data before feeding it into a model. This could involve tasks like normalizing your data or handling missing values, both of which can be accomplished using np.where.
Exploring Related Concepts
The np.where function is just one of many powerful tools in Python’s NumPy library. To further improve your array manipulation skills, consider exploring the following online resources:
- Numpy Made Easy for Python Developers contains tips and tricks for complex mathematical functions and operations.
Exploring np.arange() in NumPy – A Step-by-Step tutorial on how to create arrays with regularly spaced values via “numpy np.arange.”
Expanding Arrays with numpy.append() – An in-depth guide on array manipulation and how “numpy append” simplifies array expansion.
Official Numpy Documentation – The official documentation of Numpy offering a detailed overview of the module and its functionalities.
Numpy on Python Wiki – The official Python Wiki page for Numpy, providing an overview of its features and functionalities.
Numpy Tutorial Video on YouTube – A YouTube video tutorial providing visual instruction for understanding and using Numpy.
Other resources like Python tutorials, coding bootcamps, and online forums can also provide valuable insights and help deepen your understanding of np.where and other NumPy functions.
Wrapping Up: The Power of np.where
Throughout this guide, we’ve explored the np.where function in Python’s NumPy library in depth. We’ve seen how it can be used to locate the indices of elements in an array that satisfy a certain condition, making it a powerful tool for array manipulation.
We’ve also discussed common issues you might encounter when using np.where, such as dealing with NaN values and handling complex conditions. Solutions and workarounds for these issues were presented, along with code examples to illustrate how these solutions work in practice.
In addition, we’ve explored alternative approaches to using np.where, including Boolean indexing and the np.nonzero function. Each of these methods has its own advantages and disadvantages, and the best one to use depends on your specific use case.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
np.where | Flexible, can replace values based on a condition | Can be confusing with NaN values |
Boolean indexing | Simple, powerful | Returns elements, not indices |
np.nonzero | Simple, efficient | Less flexible than np.where |
Finally, we’ve discussed how np.where is used in fields like data analysis and machine learning, and suggested related concepts for further study. Mastering np.where and these related concepts will greatly enhance your ability to manipulate and analyze data in Python.
Whether you’re a beginner just starting out with NumPy, or an experienced data scientist looking to brush up on your skills, we hope this guide has been helpful in your journey to mastering np.where in Python’s NumPy library.