Bash Substring: String Handling in Linux Shell Script
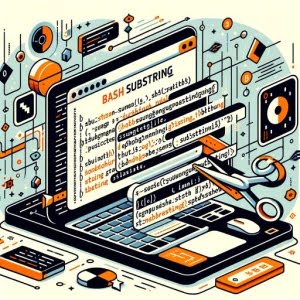
Are you finding it challenging to extract parts of a string in Bash? You’re not alone. Many developers find themselves confused when it comes to handling substrings in Bash, but we’re here to help.
Bash substring extraction allows us to precisely cut out the parts of a string we need, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with substrings in Bash, from their extraction, manipulation, and usage. We’ll cover everything from the basics of substring extraction to more advanced techniques, as well as alternative approaches.
Let’s get started and master Bash substring extraction!
TL;DR: How Do I Extract a Substring in Bash?
In Bash, you can extract a substring using the following syntax:
substring=${string:position:length}
. This command will extract a substring from the variablestring
, starting at the 7th character and taking the next 5 characters.
Here’s a simple example:
string='Hello, World!'
substring=${string:7:5}
echo $substring
# Output:
# 'World'
In this example, we’ve defined a string ‘Hello, World!’, and we’re extracting a substring starting from the 7th character (‘W’ in ‘World’) and taking the next 5 characters, which gives us ‘World’.
This is a basic way to extract a substring in Bash, but there’s much more to learn about string manipulation in Bash. Continue reading for more detailed examples and advanced techniques.
Table of Contents
Bash Substrings 101: The Basics
The basic syntax to extract a substring in Bash is substring=${string:position:length}
. Here, string
is the original string from which we want to extract a part, position
is the starting point, and length
is the number of characters we want to extract.
Let’s look at a simple example:
string='Bash substring extraction'
substring=${string:5:9}
echo $substring
# Output:
# 'substring'
In this example, our original string is ‘Bash substring extraction’. We’ve decided to extract a substring starting from the 5th character and taking the next 9 characters. This gives us ‘substring’.
Understanding the Syntax
The :
character is used to denote the start of the substring. The number immediately following the :
is the starting position of the substring. Bash strings are zero-indexed, which means counting starts at 0. So, the first character of the string is at position 0, the second character is at position 1, and so on.
The number after the :
is the length of the substring. This determines how many characters will be extracted from the string.
Advantages and Pitfalls
The advantage of this method is its simplicity and readability. It’s straightforward to understand what’s happening, and it’s easy to modify the code if necessary.
However, one potential pitfall is that Bash is zero-indexed, so the first character of the string is at position 0. If you forget this and start counting from 1, you’ll end up with an off-by-one error and extract the wrong part of the string.
Advanced Bash Substring Extraction
As you get more comfortable with Bash substrings, you can start to explore more advanced techniques. These include using variables for the start and length parameters, using negative indices, and more.
Variable Start and Length
You can use variables to specify the start and length parameters when extracting a substring. This gives you more flexibility and allows for dynamic substring extraction. Here’s an example:
string='Bash substring extraction'
start=5
length=9
substring=${string:start:length}
echo $substring
# Output:
# 'substring'
In this example, we’ve defined variables start
and length
, and used them in our substring extraction. This allows us to easily change the start and length values without having to modify the substring extraction code itself.
Negative Indices
Bash also supports negative indices for substring extraction. A negative index means start counting from the end of the string instead of the beginning. Here’s how you can use a negative index:
string='Bash substring extraction'
substring=${string: -9}
echo $substring
# Output:
# 'extraction'
In this example, we’ve used a negative index to extract the last 9 characters from the string. Note the space before the -9
. Without this space, Bash would interpret -9
as an option to the command rather than an index.
Pros and Cons
The advantage of these advanced techniques is that they provide more flexibility and control over your substring extraction. You can dynamically adjust your start and length parameters, and you can easily extract substrings from the end of your strings.
However, these techniques can also be a bit more complex and harder to read at a glance, especially for beginners. Negative indices, in particular, can be confusing if you’re not used to them. As always, it’s important to comment your code and explain what you’re doing, especially when using more advanced techniques.
Alternative Bash Substring Extraction Methods
While the built-in substring extraction in Bash is powerful and flexible, there are other tools at your disposal that can provide alternative methods for substring extraction. These include commands like cut
, awk
, and sed
.
Using cut
Command
The cut
command is a simple and effective way to extract substrings. It’s especially useful when you want to extract fields from a string based on a delimiter. Here’s an example:
string='Bash:substring:extraction'
echo $string | cut -d':' -f2
# Output:
# 'substring'
In this example, we’ve used the cut
command with the -d
option to specify a delimiter (:
), and the -f
option to specify the field number we want to extract (2). The cut
command then splits the string into fields based on the delimiter and extracts the specified field.
Using awk
Command
The awk
command is a powerful text-processing tool that can also be used for substring extraction. Here’s how you can use awk
to extract a substring:
string='Bash substring extraction'
echo $string | awk '{print substr($0, 6, 9)}'
# Output:
# 'substring'
In this example, the awk
command uses the substr
function to extract a substring from the string. The substr
function takes three arguments: the string, the start position, and the length of the substring.
Using sed
Command
The sed
(stream editor) command is another powerful tool that can be used for substring extraction. Here’s an example:
string='Bash substring extraction'
echo $string | sed 's/^\(.*\) extraction$/\1/'
# Output:
# 'Bash substring'
In this example, the sed
command uses a regular expression to match the entire string, and then uses backreferences to replace the string with the matched substring.
Pros and Cons
The advantage of these alternative methods is that they provide additional flexibility and can handle more complex substring extraction tasks. They can also handle tasks that the built-in Bash substring extraction can’t, such as extracting fields based on a delimiter.
However, these methods can also be more complex and harder to read, especially for beginners. They also require an understanding of additional tools and commands. As always, it’s important to choose the right tool for the job, and to balance complexity with readability and maintainability.
Troubleshooting Bash Substring Extraction
While Bash substring extraction is a powerful tool, it can sometimes be tricky. Let’s discuss some common issues you may encounter and their solutions.
Off-By-One Errors
As we mentioned earlier, Bash strings are zero-indexed. This can sometimes lead to off-by-one errors if you forget and start counting from 1 instead of 0. Always remember that the first character of the string is at position 0.
Handling Spaces
Spaces can sometimes cause issues in Bash substring extraction. If your string contains spaces, make sure to enclose it in quotes. Here’s an example of how spaces can affect your output:
string='Bash substring extraction'
substring=${string:5:9}
echo $substring
# Output:
# 'substring'
In this example, the substring ‘substring’ is correctly extracted even though the original string contains spaces. This is because we’ve enclosed the string in quotes, which tells Bash to treat it as a single entity.
Unintended Option Interpretation
When using negative indices, remember to include a space before the -
. Without this space, Bash will interpret -9
as an option to the command rather than an index. Here’s an example:
string='Bash substring extraction'
substring=${string: -9}
echo $substring
# Output:
# 'extraction'
In this example, we’ve used a negative index to extract the last 9 characters from the string. Note the space before the -9
. Without this space, Bash would interpret -9
as an option to the command rather than an index.
Tips for Success
Always remember to enclose your strings in quotes, especially if they contain spaces. Start counting from 0, not 1, and always include a space before a negative index. With these tips, you should be able to avoid most common issues with Bash substring extraction.
Understanding Strings and Substrings in Bash
Before diving deeper into the world of Bash substring extraction, it’s essential to understand what strings and substrings are, and how they are indexed in Bash.
Strings in Bash
In Bash, a string is a sequence of characters enclosed in quotes. It can include any character, including spaces and special characters. Here’s an example of a string in Bash:
string='Hello, World!'
echo $string
# Output:
# 'Hello, World!'
In this example, ‘Hello, World!’ is a string. It’s enclosed in quotes, which tells Bash to treat it as a single entity.
String Indexing in Bash
Bash strings are zero-indexed. This means that the first character of the string is at position 0, the second character is at position 1, and so on. Here’s an example that demonstrates this:
string='Hello, World!'
first_character=${string:0:1}
echo $first_character
# Output:
# 'H'
In this example, we’ve used the substring extraction syntax to extract the first character of the string. We’ve specified a start position of 0 and a length of 1, which gives us the first character ‘H’.
The Concept of Substrings
A substring is a portion of a string. It can be any part of the string, from a single character to the entire string itself. Substrings are crucial in scripting and programming because they allow us to manipulate and analyze text data effectively.
For instance, if you have a string that represents a date in the format ‘YYYY-MM-DD’, you can use substring extraction to extract the year, month, and day components separately. This ability to manipulate and extract data from strings is what makes substrings so powerful and important in Bash.
The Power of Bash Substring Extraction
Bash substring extraction is not just a standalone operation. It’s a fundamental tool that’s often used in larger scripts or projects. Whether you’re parsing log files, processing text data, or creating complex scripts, understanding how to effectively extract and manipulate substrings in Bash is an invaluable skill.
Exploring Related Concepts
Once you’ve mastered Bash substring extraction, there are many related concepts that you might want to explore. These include regular expressions, which allow for powerful and flexible pattern matching, and other forms of string manipulation in Bash, such as string concatenation, replacement, and formatting.
Regular expressions, in particular, can be a powerful tool in conjunction with substring extraction. They allow you to identify and extract more complex patterns, making your scripts more versatile and robust.
The Role of Substring Extraction in Larger Projects
In larger projects, substring extraction can be used to parse and process data, manipulate file paths, handle user input, and much more. It’s a fundamental tool that’s often used in shell scripting and system administration tasks.
Further Resources for Bash Mastery
If you’re interested in learning more about Bash and string manipulation, here are a few resources that you might find useful:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and detailed, making it a great resource for anyone looking to deepen their understanding of Bash.
BashGuide on Greg’s Wiki: This guide provides a thorough introduction to Bash scripting. It covers a wide range of topics, including string manipulation.
Advanced Bash-Scripting Guide: This guide goes into more depth on Bash scripting, covering advanced topics such as regular expressions and string manipulation.
By mastering Bash substring extraction and related concepts, you’ll be well-equipped to handle a wide range of scripting and system administration tasks.
Wrapping Up: Mastering Bash Substring Extraction
In this comprehensive guide, we’ve explored the ins and outs of Bash substring extraction. We’ve delved into the fundamental concepts, covered the basic and advanced usage, and even ventured into alternative methods for extracting substrings in Bash.
We began with the basics, learning how to extract a substring from a string using the built-in Bash syntax. We then ventured into more advanced territory, exploring how to use variables for the start and length parameters, and how to use negative indices for substring extraction. Along the way, we tackled common challenges you might face when using Bash substring extraction, such as off-by-one errors and handling spaces, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to Bash substring extraction, comparing the built-in method with commands like cut
, awk
, and sed
. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Bash Substring Extraction | Simple, readable, built-in | Can be tricky with spaces and negative indices |
cut Command | Great for delimited fields | Not as flexible as built-in method |
awk Command | Powerful text-processing tool | More complex, harder to read |
sed Command | Supports regular expressions | Can be complex for beginners |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of Bash substring extraction and its capabilities.
With its balance of simplicity and power, Bash substring extraction is a fundamental tool for any Bash user. Happy scripting!