Bash Conditionals | Syntax for Expressions and Statements
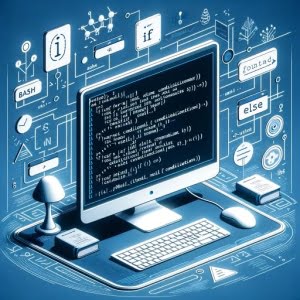
Are you finding it challenging to navigate Bash conditionals? You’re not alone. Many developers find themselves puzzled when it comes to handling Bash conditionals, but we’re here to help.
Think of Bash conditionals as a traffic light – controlling the flow of your script, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with Bash conditionals, from their basic usage to more advanced techniques. We’ll cover everything from the basics of ‘if’, ‘else’, and ‘elif’ statements to more complex uses such as nested ‘if’ statements and ‘case’ statements, as well as alternative approaches.
Let’s get started and master Bash conditionals!
TL;DR: How Do I Use Conditionals in Bash?
You can use Conditionals in Bash, such as
if
,if-else
, andelif
, to provide the ability to execute specific code blocks based on certain conditions. You can combine them with conditionals for variable comparison such aslt
, theLess Than
operator, for more complex scripting like,if [ "$a" -lt "$b" ];
. They are a fundamental part of Bash scripting, allowing you to create more complex and flexible scripts.
Here’s a simple example:
a=10
b=20
if [ "$a" -lt "$b" ]; then
echo "$a is less than $b"
fi
# Output:
# '10 is less than 20'
In this example, we’ve defined two variables, a
and b
, and used a Bash conditional to compare them. The -lt
operator checks if a
is less than b
. If the condition is true, it echoes the statement ’10 is less than 20′.
This is just a basic way to use Bash conditionals, but there’s much more to learn about controlling script flow in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Bash Conditionals: A Step-by-Step Guide
- Diving Deeper: Advanced Bash Conditionals
- Exploring Alternatives: Controlling Script Flow in Bash
- Troubleshooting Bash Conditionals: Common Errors and Solutions
- Understanding Bash Conditionals: The Core Concepts
- Expanding Horizons: Bash Conditionals in Larger Scripts
- Wrapping Up: Mastering Bash Conditionals
Basic Bash Conditionals: A Step-by-Step Guide
Bash conditionals are a fundamental part of Bash scripting. They help control the flow of your script based on specific conditions. Let’s start with the basics: ‘if’, ‘else’, and ‘elif’ statements.
The ‘if’ Statement
The ‘if’ statement in Bash is used to perform a certain action if a condition is met. Here’s an example:
a=5
if [ "$a" -gt 3 ]; then
echo "The number is greater than 3"
fi
# Output:
# 'The number is greater than 3'
In this example, we set a variable a
to 5. The ‘if’ statement checks if a
is greater than 3. If the condition is true, it echoes the statement ‘The number is greater than 3’.
The ‘else’ Statement
The ‘else’ statement is used to perform an action if the ‘if’ condition is not met. Let’s see how it works:
a=2
if [ "$a" -gt 3 ]; then
echo "The number is greater than 3"
else
echo "The number is not greater than 3"
fi
# Output:
# 'The number is not greater than 3'
In this case, since a
is not greater than 3, the script echoes ‘The number is not greater than 3’.
The ‘elif’ Statement
The ‘elif’ (else if) statement is used when you want to check multiple conditions. Here’s an example:
a=2
if [ "$a" -gt 3 ]; then
echo "The number is greater than 3"
elif [ "$a" -eq 2 ]; then
echo "The number is equal to 2"
else
echo "The number is not greater than 3 and not equal to 2"
fi
# Output:
# 'The number is equal to 2'
Here, the script first checks if a
is greater than 3. If not, it moves to the ‘elif’ condition and checks if a
is equal to 2. Since a
is indeed equal to 2, it echoes ‘The number is equal to 2’. If neither condition were met, it would echo the ‘else’ statement.
This is the basic use of Bash conditionals. They can be as simple or as complex as needed, providing flexibility in controlling script flow.
Diving Deeper: Advanced Bash Conditionals
Bash conditionals are not limited to simple ‘if’, ‘else’, and ‘elif’ statements. They can be used in more complex scenarios, including nested ‘if’ statements and ‘case’ statements. Let’s take a closer look.
Nested ‘if’ Statements
Nested ‘if’ statements are ‘if’ statements within ‘if’ statements. They allow for more complex decision-making in your scripts.
a=10
b=20
c=30
if [ "$a" -lt "$b" ]; then
echo "a is less than b"
if [ "$b" -lt "$c" ]; then
echo "b is less than c"
fi
fi
# Output:
# 'a is less than b'
# 'b is less than c'
In this example, the script first checks if a
is less than b
. If true, it echoes ‘a is less than b’ and then checks if b
is less than c
. If that condition is also true, it echoes ‘b is less than c’. This is a simple example of how nested ‘if’ statements can be used to check multiple conditions.
The ‘case’ Statement
The ‘case’ statement is used to perform different actions based on different conditions. It’s similar to using multiple ‘if’/’elif’ statements, but it provides a cleaner and more readable way to handle multiple conditions.
read -p "Enter a number (1, 2, or 3): " number
case $number in
1) echo "You entered one.";;
2) echo "You entered two.";;
3) echo "You entered three.";;
*) echo "You did not enter a number between 1 and 3.";;
esac
# Output (if you enter 2):
# 'You entered two.'
In this example, the script asks for user input. Depending on the input, it echoes a different statement. If the input is not 1, 2, or 3, it echoes ‘You did not enter a number between 1 and 3.’. This is a practical example of how the ‘case’ statement can be used to handle multiple conditions in a clean and efficient way.
These are just a few examples of the advanced uses of Bash conditionals. With these techniques, you can create more complex and flexible scripts to meet your needs.
Exploring Alternatives: Controlling Script Flow in Bash
While Bash conditionals are a powerful tool in script flow control, they are not the only ones. There are alternative approaches that can be used in different scenarios. Let’s explore ‘select’ statements and ‘until’ loops.
The ‘select’ Statement
The ‘select’ statement in Bash provides a simple menu system. It’s useful when you want the user to choose from a list of options.
echo "Please choose a drink:"
select drink in Tea Coffee Water Juice All None
do
case $drink in
"All")
echo "You get all the drinks.";;
"None")
echo "No drink for you.";;
*)
echo "You get $drink.";;
esac
break
done
# Output (if you enter 2):
# 'You get Coffee.'
In this example, the script provides a list of drinks and asks the user to choose one. Depending on the user’s choice, it echoes a different statement. This is a practical example of how the ‘select’ statement can be used to handle multiple conditions in a clean and efficient way.
The ‘until’ Loop
The ‘until’ loop in Bash is used to execute a set of commands as long as the loop’s control condition is false.
a=1
until [ "$a" -gt 5 ]
do
echo "Number: $a"
a=$((a + 1))
done
# Output:
# 'Number: 1'
# 'Number: 2'
# 'Number: 3'
# 'Number: 4'
# 'Number: 5'
In this example, the script echoes the statement ‘Number: $a’ until a
is greater than 5. This is a practical example of how the ‘until’ loop can be used to control the flow of a script based on a specific condition.
These alternative approaches give you more options for controlling script flow in Bash. Depending on your specific needs, you might find one approach more suitable than the others. The key is to understand the strengths and weaknesses of each approach and choose the one that best fits your scenario.
Troubleshooting Bash Conditionals: Common Errors and Solutions
Despite our best efforts, errors can occur when using Bash conditionals. Understanding these common mistakes and how to solve them can save you a lot of time and frustration. Let’s discuss some of these common errors and their solutions.
Missing Spaces in Conditionals
One common error when using Bash conditionals is forgetting to include spaces between the brackets and the condition.
a=10
if ["$a"-lt20]; then
echo "a is less than 20"
fi
# Output:
# bash: [10-lt20]: command not found
In this example, the script returns an error because there are no spaces between the brackets and the condition. The correct syntax should be [ "$a" -lt 20 ]
.
Using the Wrong Comparison Operator
Another common mistake is using the wrong comparison operator. Remember that Bash uses different operators for string and numerical comparisons.
a="10"
if [ "$a" -lt "20" ]; then
echo "a is less than 20"
fi
# Output:
# bash: [: 10: integer expression expected
In this example, the script returns an error because -lt
is a numerical comparison operator, but a
and 20
are strings. The correct operator for string comparison should be <
.
Forgetting the ‘then’ Keyword
Forgetting the ‘then’ keyword in an ‘if’ statement is another common error.
a=10
if [ "$a" -lt 20 ]
echo "a is less than 20"
fi
# Output:
# bash: syntax error near unexpected token `echo'
In this example, the script returns an error because the ‘then’ keyword is missing. The correct syntax should be if [ "$a" -lt 20 ]; then
.
Understanding these common errors and their solutions can help you avoid them in the future and write more effective Bash scripts. Remember, practice makes perfect. The more you work with Bash conditionals, the more comfortable you’ll become with their syntax and usage.
Understanding Bash Conditionals: The Core Concepts
Before diving deeper into the usage of Bash conditionals, it’s important to understand the fundamental concepts behind them. Let’s break down how Bash conditionals work and discuss related topics, such as Bash variables and operators.
The Anatomy of Bash Conditionals
A Bash conditional is essentially a control structure used in Bash scripting to control the flow of execution based on certain conditions. It’s composed of a few key elements:
- Test expression: This is the condition that is being checked. It could be a comparison between two variables or a specific state of a file, among other things.
Commands: These are the actions that are executed based on whether the test expression is true or false.
Control keywords: These are keywords such as ‘if’, ‘else’, ‘elif’, ‘fi’, etc. They control the flow of the conditional.
Here’s a basic example:
a=10
b=20
if [ "$a" -lt "$b" ]; then
echo "a is less than b"
else
echo "a is not less than b"
fi
# Output:
# 'a is less than b'
In this example, the test expression is "$a" -lt "$b"
, the commands are echo "a is less than b"
and echo "a is not less than b"
, and the control keywords are ‘if’, ‘else’, and ‘fi’.
Bash Variables and Operators
Bash variables and operators play a crucial role in Bash conditionals. Variables are used to store data that can be used in the script, while operators are used to perform operations on these variables.
Here’s an example demonstrating the use of variables and operators in a Bash conditional:
a=10
b=20
if [ "$a" -lt "$b" ]; then
c=$((a + b))
echo "The sum of a and b is $c"
fi
# Output:
# 'The sum of a and b is 30'
In this example, a
and b
are variables, -lt
is a comparison operator, and +
is an arithmetic operator. The script checks if a
is less than b
. If true, it calculates the sum of a
and b
, stores it in a new variable c
, and then echoes the result.
Understanding these fundamental concepts is key to mastering Bash conditionals. They provide the foundation upon which more complex scripts can be built.
Expanding Horizons: Bash Conditionals in Larger Scripts
Bash conditionals, while powerful on their own, can be even more effective when combined with other Bash features in larger scripts or projects. Let’s explore how Bash conditionals interact with other elements like loops and functions, and how they can contribute to more complex scripting scenarios.
Bash Conditionals and Loops
Bash conditionals can be used within loops to provide more complex control flow. Here’s an example of a Bash conditional within a ‘for’ loop:
for (( i=1; i<=5; i++ ))
do
if [ "$i" -eq 3 ]; then
echo "Loop has reached 3"
fi
done
# Output:
# 'Loop has reached 3'
In this example, the script loops from 1 to 5. When the loop variable i
reaches 3, the conditional triggers and the script echoes ‘Loop has reached 3’. This demonstrates how Bash conditionals can be used to control the flow within a loop.
Bash Conditionals and Functions
Bash conditionals can also be used within functions to control the function’s behavior based on certain conditions. Here’s an example:
function greet() {
if [ "$1" == "morning" ]; then
echo "Good morning!"
elif [ "$1" == "evening" ]; then
echo "Good evening!"
else
echo "Hello!"
fi
}
greet "morning"
greet "evening"
greet ""
# Output:
# 'Good morning!'
# 'Good evening!'
# 'Hello!'
In this example, the function ‘greet’ takes one argument. Depending on the argument, it echoes a different greeting. This shows how Bash conditionals can be used to control a function’s behavior.
Further Resources for Mastering Bash Conditionals
To continue your journey in mastering Bash conditionals, check out these additional resources:
- GNU Bash Manual: The official Bash manual from GNU is an exhaustive resource covering all aspects of Bash scripting.
Bash Guide for Beginners: This guide from The Linux Documentation Project is an excellent starting point for beginners to Bash scripting.
Bash Scripting Tutorial: This comprehensive tutorial covers everything from basic to advanced Bash scripting concepts.
Wrapping Up: Mastering Bash Conditionals
In this comprehensive guide, we’ve navigated through the world of Bash conditionals, the traffic lights of script control in Bash.
We began with the basics, understanding the use of ‘if’, ‘else’, and ‘elif’ statements. We then ventured into more complex territory, tackling nested ‘if’ statements and ‘case’ statements. We also explored alternative approaches like ‘select’ statements and ‘until’ loops, expanding our toolbox for controlling script flow.
Along the way, we addressed common errors when using Bash conditionals and provided solutions to help you avoid these pitfalls. We also delved into the core concepts behind Bash conditionals, discussing Bash variables and operators to give you a deeper understanding of how conditionals work.
Here’s a quick comparison of the techniques we’ve discussed:
Technique | Use Case | Complexity |
---|---|---|
‘if’, ‘else’, ‘elif’ Statements | Basic conditional logic | Low |
Nested ‘if’ Statements | More complex decision-making | Moderate |
‘case’ Statement | Handling multiple conditions cleanly | Moderate |
‘select’ Statement | Creating a menu system | High |
‘until’ Loop | Executing commands until a condition is met | High |
Whether you’re just starting out with Bash conditionals or looking for a handy reference, we hope this guide has helped you navigate the ins and outs of Bash conditionals.
Bash conditionals offer a powerful way to control the flow of your scripts. With the knowledge you’ve gained from this guide, you’re well-equipped to use Bash conditionals effectively in your scripting endeavors. Happy scripting!