Spring Boot Framework | Java App Development Guide
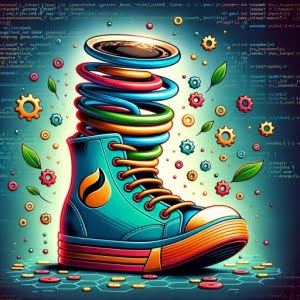
Are you looking to streamline your Java application development? You’re not alone. Many developers are constantly seeking ways to simplify their work, and Spring Boot is a tool that can do just that.
Think of Spring Boot as a turbocharger for your Java development process. It’s a powerful framework that can supercharge your development, making it faster and more efficient.
This guide will walk you through the essentials of Spring Boot, from setting up a simple application to exploring advanced features. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Spring Boot!
TL;DR: What is Spring Boot and How Do I Get Started?
Spring Boot
is a powerful framework designed to simplify the setup and development of Spring applications. To get started, you can use Spring Initializr to generate a basic project structure, accessed at You can access it athttps://start.spring.io/
. Here’s a simple example:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In this example, we’ve created a basic Spring Boot application. The @SpringBootApplication
annotation is a convenience annotation that adds all of the following:
@Configuration
: Tags the class as a source of bean definitions for the application context.@EnableAutoConfiguration
: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.@ComponentScan
: Tells Spring to look for other components, configurations, and services in thecom/example
package, allowing it to find the controllers.
The main()
method uses Spring Boot’s SpringApplication.run()
method to launch an application.
This is just a basic way to use Spring Boot, but there’s much more to learn about creating and managing Spring Boot applications. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Use: Setting Up a Simple Spring Boot Application
- Configuring Application Properties
- Exploring Alternatives to Spring Boot: Micronaut and Quarkus
- Comparing Spring Boot, Micronaut, and Quarkus
- Troubleshooting Common Spring Boot Issues
- Understanding the Spring Framework
- Spring Boot in Larger Projects and Microservices Architecture
- Exploring Related Topics: Spring Security and Spring Cloud
- Wrapping Up: Java Spring Boot
Basic Use: Setting Up a Simple Spring Boot Application
Let’s start with the basics: setting up a simple Spring Boot application. Here are the steps to follow:
- Create a New Project: Use Spring Initializr to generate a basic project structure. You can access it at https://start.spring.io/.
Choose Your Project Options: On the Spring Initializr page, you’ll select Maven Project (which is the default) and the language (we’re using Java). For the Spring Boot version, it’s typically best to choose the most recent stable release.
Name Your Project: Next, you’ll fill in the ‘Group’ and ‘Artifact’ fields to name your project. The ‘Group’ is usually your organization or company name, and the ‘Artifact’ is the name of your project. For example, you might use ‘com.example’ for the Group, and ‘myproject’ for the Artifact.
Choose Your Dependencies: You can search for and add any dependencies your project needs. For a simple project, you might just add ‘Spring Web’ to create a web application.
Once you’ve filled in all your options, you can click ‘Generate’ to download a .zip file with your project.
- Unzip and Open Your Project: After you’ve downloaded the .zip file, you can unzip it and open it in your favorite IDE (like IntelliJ IDEA or Eclipse).
Now, let’s look at the main application class and how to run the application.
The Main Application Class
The main application class is the heart of any Spring Boot application. It’s typically named after your project, with ‘Application’ appended to the end. For example, if you named your project ‘myproject’, your main application class would be ‘MyprojectApplication’.
Here’s what a typical main application class looks like:
@SpringBootApplication
public class MyprojectApplication {
public static void main(String[] args) {
SpringApplication.run(MyprojectApplication.class, args);
}
}
This class is annotated with @SpringBootApplication
, which is a convenience annotation that adds all of the following:
@Configuration
: Tags the class as a source of bean definitions for the application context.@EnableAutoConfiguration
: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.@ComponentScan
: Tells Spring to look for other components, configurations, and services in thecom/example
package, allowing it to find the controllers.
The main()
method uses Spring Boot’s SpringApplication.run()
method to launch an application.
Running the Application
You can run a Spring Boot application from the command line with a built-in Maven wrapper (mvnw if you’re on Unix/macOS, or mvnw.cmd if you’re on Windows) that’s included in the project you generated with Spring Initializr.
Here’s how you can do it:
./mvnw spring-boot:run
# Output:
# ...
# 2022-01-01 00:00:00.000 INFO 12345 --- [ main] com.example.myproject.MyprojectApplication : Starting MyprojectApplication v0.1.0 on mycomputer with PID 12345 (/path/to/myproject/target/classes started by user in /path/to/myproject)
# ...
This command compiles and runs the application. The spring-boot:run
command triggers the Spring Boot functionality in the Maven build system.
In the output, you can see the log messages from Spring Boot when it starts up. The ‘INFO’ messages give you information about what’s happening as the application starts. You can see the application starting, the configuration it’s using, and when the application is ready to use.
Congratulations, you’ve just set up and run your first Spring Boot application! But this is just the beginning. Continue reading to dive deeper into Spring Boot and learn about its more advanced features.
Configuring Application Properties
Spring Boot allows you to externalize your configuration so you can work with the same application code in different environments. You can use properties files, YAML files, environment variables, and command-line arguments to externalize configuration.
Spring Boot uses a very particular PropertySource order that is designed to allow sensible overriding of values. Properties are considered in the following order:
- Devtools global settings properties on your home directory (~/.spring-boot-devtools.properties when devtools is active).
- @TestPropertySource annotations on your tests.
- @SpringBootTest#properties annotation attribute on your tests.
- Command line arguments.
- Properties from SPRING_APPLICATION_JSON (inline JSON embedded in an environment variable or system property)
- ServletConfig init parameters.
- ServletContext init parameters.
- JNDI attributes from java:comp/env.
- Java System properties (System.getProperties()).
- OS environment variables.
- A RandomValuePropertySource that has properties only in random.*.
- Profile-specific application properties outside of your packaged jar (application-{profile}.properties and YAML variants)
- Profile-specific application properties packaged inside your jar (application-{profile}.properties and YAML variants)
- Application properties outside of your packaged jar (application.properties and YAML variants).
- Application properties packaged inside your jar (application.properties and YAML variants).
- @PropertySource annotations on your @Configuration classes.
- Default properties (specified using SpringApplication.setDefaultProperties).
You can’t override properties in the application.properties file by adding another application.properties in your own configuration files.
Configuring a REST API
Spring Boot makes it easy to create stand-alone, production-grade RESTful APIs that you can “just run”. Let’s create a simple REST API:
@RestController
public class GreetingController {
private static final String template = "Hello, %s!";
private final AtomicLong counter = new AtomicLong();
@GetMapping("/greeting")
public Greeting greeting(@RequestParam(value = "name", defaultValue = "World") String name) {
return new Greeting(counter.incrementAndGet(), String.format(template, name));
}
}
In this example, we have a GreetingController
that handles GET requests for /greeting by returning a new instance of the Greeting class.
Integrating with Databases
Spring Boot provides a powerful batch processing and supports for the JDBC, JPA, JTA, and JMS technologies for communicating with relational databases.
Let’s create a simple application that uses Spring Data JPA to save objects to and fetch them from a database, all without writing a concrete repository implementation.
@Entity
public class Customer {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private Long id;
private String firstName;
private String lastName;
protected Customer() {}
public Customer(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
@Override
public String toString() {
return String.format(
"Customer[id=%d, firstName='%s', lastName='%s']",
id, firstName, lastName);
}
// getters & setters omitted for brevity
}
Here we have a Customer
class that is marked up with @Entity
to indicate that it is a JPA entity. The @Id
and @GeneratedValue
annotations are JPA annotations to note the primary key and that it is automatically generated by the database. The Customer
constructor is protected because it doesn’t need to be public: it is only ever used by the repository, and it is there to prevent direct instantiation.
The toString()
method prints out information about the Customer
for debugging purposes.
We have only scratched the surface of what you can do with Spring Boot. As you continue to explore, you’ll find that it offers much more advanced features and capabilities that can help you develop high-quality, robust applications.
Exploring Alternatives to Spring Boot: Micronaut and Quarkus
While Spring Boot is a powerful and popular choice for Java developers, it’s not the only option. Let’s delve into two alternative Java frameworks: Micronaut and Quarkus.
Micronaut: A Modern, JVM-Based Framework
Micronaut is a modern, JVM-based, full-stack framework for building modular, easily testable microservice applications. It’s designed to provide you with essential features you need for your microservices at compile time, without the performance cost.
mn create-app example.micronaut.micronautguide
This command creates a new Micronaut application.
# Output:
# | Application created at /path/to/example/micronaut/micronautguide
Micronaut’s benefits include fast startup time, reduced memory footprint, and a fluent and easy-to-use API. However, it might have a steeper learning curve if you’re already familiar with Spring Boot.
Quarkus: Supersonic Subatomic Java
Quarkus is a Kubernetes-native Java stack tailored for GraalVM and OpenJDK HotSpot, crafted from the best of breed Java libraries and standards. It’s designed to work out of the box with popular Java standards, frameworks, and libraries like Eclipse MicroProfile, Apache Kafka, RESTEasy (JAX-RS), Hibernate ORM (JPA), CDI, Angular/React and many more.
mvn io.quarkus:quarkus-maven-plugin:2.5.1.Final:create \
-DprojectGroupId=org.acme \
-DprojectArtifactId=getting-started \
-DclassName="org.acme.getting.started.GreetingResource" \
-Dpath="/hello"
This command creates a new Quarkus application.
# Output:
# ...
# [INFO] Installing the application locally using the native executable
# [INFO] Execute the following command to run your application:
# ./target/getting-started-1.0.0-SNAPSHOT-runner
# ...
Quarkus aims to make Java a leading platform in Kubernetes and serverless environments while offering developers a unified reactive and imperative programming model. However, it may not be as feature-rich as Spring Boot or as fast as Micronaut.
Comparing Spring Boot, Micronaut, and Quarkus
Feature | Spring Boot | Micronaut | Quarkus |
---|---|---|---|
Startup Time | Medium | Fast | Fast |
Memory Footprint | High | Low | Low |
Learning Curve | Low | Medium | Medium |
Community Support | High | Medium | High |
Compatibility with Existing Technologies | High | Medium | High |
In conclusion, while Spring Boot remains a popular choice for many developers, alternatives like Micronaut and Quarkus offer unique advantages and could be a better fit depending on your specific needs and environment.
Troubleshooting Common Spring Boot Issues
Even with a robust framework like Spring Boot, you may encounter challenges. Let’s discuss some common issues and how to resolve them.
Configuration Problems
One of the most common issues when working with Spring Boot is configuration problems. You might encounter an error message that says ‘Failed to configure a DataSource: no appropriate constructor found.’ This usually means that Spring Boot is trying to set up a database connection, but it can’t find the necessary configuration.
Here’s how you can resolve this issue:
- Check Your Application Properties: Make sure you have the correct database connection properties in your application.properties or application.yml file.
spring.datasource.url=jdbc:mysql://localhost/test
spring.datasource.username=dbuser
spring.datasource.password=dbpass
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
In this example, we’re setting up a connection to a MySQL database. Make sure to replace ‘localhost/test’, ‘dbuser’, and ‘dbpass’ with your actual database URL, username, and password.
- Include the Correct Dependencies: Ensure that you have the correct database driver in your project dependencies. For a MySQL database, you would need the MySQL Connector/J dependency.
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
This is a Maven dependency for MySQL Connector/J. If you’re using Gradle or another build tool, the syntax will be different.
Runtime Errors
Another common issue is runtime errors. These can occur for a variety of reasons, such as missing beans or components, incorrect annotations, or application code errors.
Here’s a common runtime error and how to resolve it:
Error Creating Bean: This error often occurs when Spring Boot can’t create a bean for a particular component. The error message might look something like this:
Caused by: org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'myComponent': Injection of autowired dependencies failed; nested exception is java.lang.IllegalArgumentException: Could not resolve placeholder 'my.property' in value "${my.property}"
This error is saying that Spring Boot can’t create the ‘myComponent’ bean because it can’t resolve the ‘my.property’ placeholder.
To resolve this error, you need to ensure that ‘my.property’ is correctly defined in your application.properties or application.yml file. If it’s supposed to be an environment variable, make sure it’s correctly set in your environment.
Remember, troubleshooting requires patience and a systematic approach. By carefully reading error messages and understanding the basics of Spring Boot, you can resolve most issues that come your way.
Understanding the Spring Framework
Before we delve deeper into Spring Boot, it’s crucial to understand the underlying Spring Framework that it’s built upon. The Spring Framework is a comprehensive tool for supporting applications in Java, and it’s at the heart of many enterprise-level applications.
Dependency Injection: Simplifying Object Management
One of the core concepts in Spring is Dependency Injection (DI), a design pattern that removes the dependency from the programming code. This makes it easier to manage your code and keeps it clean and modular.
Here’s an example of how Dependency Injection works in Spring:
@Component
public class ExampleClass {
private final AnotherClass anotherClass;
public ExampleClass(AnotherClass anotherClass) {
this.anotherClass = anotherClass;
}
public void doSomething() {
anotherClass.doSomethingElse();
}
}
In this example, ExampleClass
depends on AnotherClass
. Instead of instantiating AnotherClass
within ExampleClass
, we’re injecting it through the constructor. This is a basic example of Dependency Injection.
Aspect-Oriented Programming: Separating Concerns
Another key concept in Spring is Aspect-Oriented Programming (AOP), a programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns. It does so by adding additional behavior to existing code without modifying the code itself.
Here’s an example of how AOP works in Spring:
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.myapp.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method:" + joinPoint.getSignature());
}
@After("execution(* com.example.myapp.*.*(..))")
public void logAfter(JoinPoint joinPoint) {
System.out.println("After method:" + joinPoint.getSignature());
}
}
In this example, LoggingAspect
is an aspect that logs information before and after the execution of methods in the com.example.myapp
package. The @Before
and @After
annotations are called advice, and they define when the aspect should be applied.
Understanding these core concepts of the Spring Framework will give you a solid foundation for working with Spring Boot. While Spring Boot simplifies many aspects of using Spring, the underlying principles remain the same.
Spring Boot in Larger Projects and Microservices Architecture
Spring Boot isn’t only for simple applications—it’s also a powerful tool for building complex systems. In larger projects, Spring Boot can help you manage complexity and keep your codebase organized and maintainable.
One of the ways Spring Boot excels in larger projects is through its support for microservices architecture. Microservices architecture is a design pattern where an application is made up of small, loosely coupled services. Each service represents a specific business capability, and can be developed and deployed independently of the others.
Spring Boot provides several features that make it a good fit for microservices architecture. For example, it has built-in support for embedded servers, which means each service can run in its own process. It also has excellent support for RESTful APIs, which are often used for communication between services.
Here’s an example of a simple microservice in Spring Boot:
@SpringBootApplication
@RestController
public class ProductService {
@GetMapping("/products")
public List<Product> getProducts() {
return List.of(new Product("Apple"), new Product("Orange"));
}
public static void main(String[] args) {
SpringApplication.run(ProductService.class, args);
}
}
In this example, we’ve created a simple product service that returns a list of products. Each product is represented by a Product
object.
Exploring Related Topics: Spring Security and Spring Cloud
As you continue your journey with Spring Boot, you might find it helpful to explore related topics. Two topics that often come up in the context of Spring Boot are Spring Security and Spring Cloud.
Spring Security is a powerful and highly customizable authentication and access-control framework. It’s the de-facto standard for securing Spring-based applications.
Spring Cloud provides tools for developers to quickly build some of the common patterns in distributed systems (e.g. configuration management, service discovery, circuit breakers, intelligent routing, micro-proxy, control bus, one-time tokens, global locks, leadership election, distributed sessions, cluster state). Coordination of distributed systems leads to boiler plate patterns, and using Spring Cloud developers can quickly stand up services and applications that implement those patterns.
Further Resources for Mastering Spring Boot
To deepen your understanding of Spring Boot and its ecosystem, check out these resources:
- Understanding Java Import – Learn to manage dependencies efficiently.
Exploring Spring Boot Starter – Learn to bootstrap projects quickly with pre-configured dependencies.
Spring Boot Project Page includes a comprehensive reference guide, as well as links to many other resources.
Baeldung’s Spring Boot Guides covers a wide range of Spring Boot topics.
Udemy Spring Boot Course covers Spring Boot in depth, with a focus on practical, hands-on learning.
Wrapping Up: Java Spring Boot
In this comprehensive guide, we’ve delved into the world of Java Spring Boot, a powerful framework designed to simplify the process of setting up and developing Spring applications. Spring Boot’s capabilities range from creating simple applications to more complex systems, making it an invaluable tool for any Java developer.
We began with the basics, exploring how to set up a simple Spring Boot application and understanding the essential components involved in this process. Gradually, we ventured into more advanced territory, discussing how to configure application properties, create REST APIs, and integrate with databases.
To give you a broader perspective, we also introduced alternative Java frameworks like Micronaut and Quarkus, discussing their unique advantages and how they compare with Spring Boot. Along the way, we addressed common issues you might encounter when working with Spring Boot, providing solutions and workarounds for each issue.
Here’s a quick comparison of Spring Boot and its alternatives:
Framework | Learning Curve | Community Support | Performance |
---|---|---|---|
Spring Boot | Low | High | High |
Micronaut | Medium | Medium | High |
Quarkus | Medium | High | High |
Whether you’re a beginner just starting out with Spring Boot or an experienced developer seeking to deepen your knowledge, we hope this guide has provided valuable insights and made your journey with Spring Boot more fruitful.
Spring Boot’s capability to simplify Spring application development, combined with its flexibility and robustness, makes it a powerful tool in your Java development toolkit. Here’s to creating more efficient and high-quality Java applications with Spring Boot. Happy coding!