The ‘Not Equal’ (!=) Operator | Bash Script Explained
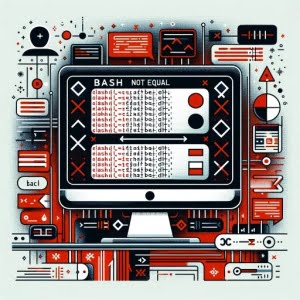
Are you finding it challenging to understand the ‘not equal’ operator in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling this operator in Bash, but we’re here to help.
Think of Bash’s ‘not equal’ operator as a traffic signal – controlling the flow of your scripts, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using the ‘not equal’ operator in Bash, from its basic usage, advanced techniques, to alternative approaches. We’ll cover everything from the basics of this operator to more complex scenarios, as well as common issues and their solutions.
Let’s get started and master the ‘not equal’ operator in Bash!
TL;DR: How Do I Use the ‘Not Equal’ Operator in Bash?
In Bash, the
'not equal'
operator is represented by'!='
. It’s primarily used in conditional statements to compare two values, such asif [ "$a" != "$b" ]
. If the values are not equal, the condition becomes true.
Here’s a simple example:
a="Hello"
b="World"
if [ "$a" != "$b" ]
then
echo "a and b are not equal"
fi
# Output:
# 'a and b are not equal'
In this example, we have two variables, a
and b
, holding the values ‘Hello’ and ‘World’ respectively. The ‘not equal’ operator !=
is used in the if
statement to compare these two variables. Since ‘Hello’ and ‘World’ are not equal, the condition is true, and the echo statement is executed, printing ‘a and b are not equal’.
This is just a basic use of the ‘not equal’ operator in Bash, but there’s much more to learn about it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the ‘Not Equal’ Operator in Bash
- Advanced Usage of ‘Not Equal’ in Bash
- Exploring Alternative Operators in Bash
- Troubleshooting the ‘Not Equal’ Operator in Bash
- Bash Scripting and Conditional Statements: A Deep Dive
- Expanding the ‘Not Equal’ Operator to Larger Projects
- Further Resources for Bash Scripting Mastery
- Wrapping Up: Mastering the ‘Not Equal’ Operator in Bash
Understanding the ‘Not Equal’ Operator in Bash
The ‘not equal’ operator in Bash, represented by ‘!=’, is a fundamental tool in Bash scripting. It’s used primarily in conditional statements, which are the backbone of any script, allowing you to make decisions and control the flow of your script.
Use of ‘Not Equal’ in a Simple Script
Let’s dive into a simple example to illustrate this:
name="Anton"
if [ "$name" != "John" ]
then
echo "You are not John!"
fi
# Output:
# 'You are not John!'
In this script, we have a variable name
holding the value ‘Anton’. We then use the ‘not equal’ operator !=
in an if
statement to compare the name
variable with the string ‘John’. As ‘Anton’ is not equal to ‘John’, the condition is true, and the script prints ‘You are not John!’.
Advantages and Pitfalls
The ‘not equal’ operator is a powerful tool, allowing you to add complexity and flexibility to your scripts. However, it’s essential to remember that it only works for string comparison. When comparing numerical values, the ‘not equal’ operator might not behave as expected, which is a common pitfall for beginners.
In the next sections, we’ll explore more advanced uses of the ‘not equal’ operator and how to avoid common mistakes. Stay tuned!
Advanced Usage of ‘Not Equal’ in Bash
By now, you should have a basic understanding of the ‘not equal’ operator in Bash. Let’s step it up a notch and explore how this operator can be utilized in more complex scenarios, such as within while loops or case statements.
‘Not Equal’ in While Loops
The ‘not equal’ operator can be used in while loops to control the flow of the script based on the comparison of two variables. Let’s examine this with a code example:
counter=1
while [ "$counter" != "10" ]
do
echo "Counter: $counter"
counter=$((counter+1))
done
# Output:
# Counter: 1
# Counter: 2
# ...
# Counter: 9
In this script, we initialize a counter
variable with the value ‘1’. We then use a while
loop that continues to run as long as counter
is not equal to ’10’. Inside the loop, we print the current value of counter
and increment it by 1. The loop stops once counter
equals ’10’.
‘Not Equal’ in Case Statements
The ‘not equal’ operator can also be used in case
statements to perform different actions based on the value of a variable. Here’s an example:
fruit="Apple"
case "$fruit" in
"Banana") echo "You have a Banana." ;;
*) echo "You do not have a Banana." ;;
esac
# Output:
# 'You do not have a Banana.'
In this script, we have a fruit
variable holding the value ‘Apple’. We then use a case
statement to check the value of fruit
. If fruit
is ‘Banana’, it prints ‘You have a Banana.’ If fruit
is not equal to ‘Banana’ (represented by ‘*’), it prints ‘You do not have a Banana.’
These examples demonstrate how the ‘not equal’ operator can be used in more complex scenarios in Bash. In the next section, we’ll explore alternative approaches and related operators.
Exploring Alternative Operators in Bash
While the ‘not equal’ operator is a powerful tool, Bash provides other operators that can be used in different contexts. One such operator is ‘-ne’, which is primarily used for numeric comparison.
Numeric Comparison with ‘-ne’
Let’s take a look at an example of how ‘-ne’ can be used:
num1=10
num2=20
if [ "$num1" -ne "$num2" ]
then
echo "num1 and num2 are not equal"
fi
# Output:
# 'num1 and num2 are not equal'
In this script, we have two variables, num1
and num2
, holding the values ’10’ and ’20’ respectively. We then use the ‘-ne’ operator in an if
statement to compare these two variables. Since ’10’ is not equal to ’20’, the condition is true, and the script prints ‘num1 and num2 are not equal’.
The ‘-ne’ operator is particularly useful when you’re working with numerical values. It can help you avoid unexpected results that can occur when using the ‘not equal’ operator for numeric comparison.
Deciding Between ‘!=’ and ‘-ne’
When deciding between the ‘not equal’ operator and the ‘-ne’ operator, it’s essential to consider the type of values you’re comparing. If you’re comparing string values, the ‘not equal’ operator is the way to go. However, if you’re dealing with numerical values, the ‘-ne’ operator is a safer choice.
In the next section, we’ll discuss common issues when using the ‘not equal’ operator in Bash and how to troubleshoot them.
Troubleshooting the ‘Not Equal’ Operator in Bash
While the ‘not equal’ operator is a handy tool in Bash, it’s not uncommon to run into some issues or obstacles. Let’s discuss some common problems and their solutions.
Issue: Comparing Numbers with ‘!=’
A common mistake is using the ‘not equal’ operator to compare numbers, which can lead to unexpected results. Here’s an example:
num1=007
num2=7
if [ "$num1" != "$num2" ]
then
echo "num1 and num2 are not equal"
fi
# Output:
# 'num1 and num2 are not equal'
In this script, even though ‘007’ and ‘7’ are numerically equal, the ‘not equal’ operator treats them as strings and therefore considers them as not equal.
Solution: Using ‘-ne’ for Numbers
To avoid this issue, use the ‘-ne’ operator for numeric comparison:
num1=007
num2=7
if [ "$num1" -ne "$num2" ]
then
echo "num1 and num2 are not equal"
else
echo "num1 and num2 are equal"
fi
# Output:
# 'num1 and num2 are equal'
In this script, the ‘-ne’ operator correctly identifies ‘007’ and ‘7’ as numerically equal.
Best Practices and Optimization
When using the ‘not equal’ operator in Bash, it’s important to follow best practices for optimal results:
- Always use double quotes around variables in conditionals to prevent word splitting and pathname expansion.
- Use the ‘not equal’ operator for string comparison and ‘-ne’ for numeric comparison.
- Always test your scripts with different inputs to ensure they work as expected.
In the next section, we’ll delve deeper into the fundamentals of Bash scripting and conditional statements.
Bash Scripting and Conditional Statements: A Deep Dive
Bash scripting is a powerful tool for automating tasks in Unix-based systems. At the heart of Bash scripting are conditional statements, which allow scripts to make decisions based on certain conditions.
The Role of Operators in Bash
Operators in Bash, such as the ‘not equal’ operator, play a crucial role in these conditional statements. They enable comparison of values, which forms the basis of decision-making in scripts.
Let’s look at an example of a conditional statement in Bash that uses the ‘not equal’ operator:
name="Alice"
if [ "$name" != "Bob" ]
then
echo "Hello, Alice"
else
echo "Hello, Bob"
fi
# Output:
# 'Hello, Alice'
In this script, we’re comparing the variable name
with the string ‘Bob’ using the ‘not equal’ operator. Since ‘Alice’ is not equal to ‘Bob’, the script prints ‘Hello, Alice’.
This example demonstrates how operators control the flow of a script. If the condition is true (i.e., name
is not equal to ‘Bob’), the script executes one block of code. If the condition is false, it executes another block of code.
The Importance of Conditional Statements
Conditional statements are vital in Bash scripting. They allow scripts to respond differently to different scenarios, making them more flexible and powerful. By understanding how to use operators like the ‘not equal’ operator in conditional statements, you can harness the full power of Bash scripting.
In the next section, we’ll discuss how the ‘not equal’ operator can be applied in larger scripts or projects and suggest related topics for further exploration.
Expanding the ‘Not Equal’ Operator to Larger Projects
The ‘not equal’ operator in Bash is not just for simple scripts or standalone conditional statements. It can be effectively used in larger scripts or projects, providing the ability to handle complex scenarios and make your scripts more dynamic and flexible.
Incorporating ‘Not Equal’ in Complex Scripts
Imagine a script that interacts with a database or a file system. The ‘not equal’ operator can be used to compare values retrieved from the database or files, allowing the script to make decisions based on these values. This could involve determining whether to update a database record, delete a file, or send a notification, among other things.
Related Topics: Loops and Case Statements
The ‘not equal’ operator often goes hand-in-hand with other Bash constructs such as loops and case statements. For instance, in a while
loop, the ‘not equal’ operator can be used to control the loop’s execution. Similarly, in a case
statement, it can help perform different actions based on a variable’s value.
Further Resources for Bash Scripting Mastery
To further deepen your understanding of Bash scripting and the ‘not equal’ operator, you might find the following resources helpful:
- Advanced Bash-Scripting Guide: This guide offers an in-depth look at Bash scripting, covering everything from basic to advanced topics.
Bash Beginners Guide: If you’re new to Bash scripting, this guide provides a great starting point, covering all the basics.
GNU Bash Manual: The official manual for Bash from GNU, the organization that maintains Bash. It’s a comprehensive resource that covers all aspects of Bash scripting.
By mastering the ‘not equal’ operator and understanding how it fits into the broader context of Bash scripting, you can create more powerful and flexible scripts. Happy scripting!
Wrapping Up: Mastering the ‘Not Equal’ Operator in Bash
In this comprehensive guide, we’ve explored the ‘not equal’ operator in Bash, a crucial tool for controlling the flow of your scripts.
We began with the basics, understanding the use of the ‘not equal’ operator in simple conditional statements. We then delved into more advanced usage, such as incorporating the operator within while loops and case statements. Along the way, we tackled common issues and their solutions, such as the pitfalls of using ‘!=’ for numeric comparison and the correct use of ‘-ne’ for such cases.
We also looked at alternative approaches, discussing the ‘-ne’ operator for numeric comparison. This provided a broader perspective on the various tools available in Bash for comparison and decision-making.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘!=’ Operator | Useful for string comparison | Can lead to unexpected results with numbers |
‘-ne’ Operator | Ideal for numeric comparison | Not suitable for string comparison |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of the ‘not equal’ operator and its uses in Bash.
The ‘not equal’ operator is a powerful tool in Bash scripting, enabling you to add complexity and flexibility to your scripts. Now, you’re well equipped to harness its power. Happy scripting!