Python Naming Conventions: Pythonic Style Guide
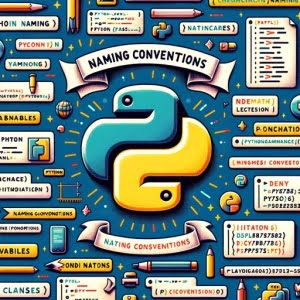
Ever felt overwhelmed by how to name your variables, functions, and classes in Python? You’re not alone. Many developers find Python’s naming conventions a bit puzzling. Think of Python’s naming conventions as a well-organized library – a system that makes your code easier to read and understand.
Naming conventions are a powerful way to bring consistency and clarity to your Python scripts, making them more readable and maintainable. They are like the signposts in a city, guiding you and others through the code.
In this guide, we’ll walk you through Python’s naming conventions, from the basics to more advanced techniques. We’ll cover everything from naming variables, functions, and classes to more complex constructs like modules, packages, and exceptions. We’ll also discuss common mistakes and how to avoid them.
Let’s dive in and start mastering Python Naming Conventions!
TL;DR: What Are Python Naming Conventions?
Python’s naming conventions, as per PEP 8, suggest using
lowercase_with_underscores
for variable and function names,PascalCase
for class names, andUPPER_CASE_WITH_UNDERSCORES
for constants. Here’s a quick example:
# Variable and function naming
my_variable = 'Hello, World!'
def my_function():
print(my_variable)
# Class naming
class MyClass:
pass
# Constant naming
MY_CONSTANT = 100
# Output:
# 'Hello, World!'
In this example, my_variable
and my_function
are named using lowercase_with_underscores
, MyClass
is named using PascalCase
, and MY_CONSTANT
is named using UPPER_CASE_WITH_UNDERSCORES
.
But Python’s naming conventions go far beyond this. Continue reading for a more detailed explanation, examples, and advanced topics.
Table of Contents
- Understanding Python Naming Conventions: The Basics
- Python Naming Conventions: Beyond the Basics
- Exploring Alternative Python Naming Conventions
- Avoiding Common Mistakes in Python Naming Conventions
- The Importance of Naming Conventions in Python
- Python Naming Conventions in Larger Coding Projects
- Wrapping Up: Mastering Python Naming Conventions
Understanding Python Naming Conventions: The Basics
Python’s naming conventions are not just arbitrary rules. They are practical guidelines to help developers write code that is easy to read and understand. Let’s take a closer look at these conventions for variables, functions, classes, and constants.
Variables and Functions
In Python, it’s recommended to name your variables and functions in lowercase_with_underscores
format. This style is also known as snake_case
. Here’s an example:
# Variable naming
my_variable = 'Hello, Python!'
# Function naming
def my_function():
print(my_variable)
my_function()
# Output:
# 'Hello, Python!'
In this example, my_variable
and my_function
are named using snake_case
. This naming convention improves the readability of your code, making it easier for you and others to understand at a glance what the variable or function does.
Classes
When it comes to naming classes in Python, we use PascalCase
(also known as CamelCase
). This means that the first letter of each word is capitalized, with no underscores between words. Here’s an example:
class MyFirstClass:
pass
In this example, MyFirstClass
is a class named using PascalCase
. This convention differentiates class names from variable and function names, making your code easier to read.
Constants
Constants in Python are usually declared in UPPER_CASE_WITH_UNDERSCORES
. This convention helps to differentiate constants from other Python constructs. Here’s an example:
# Constant naming
MY_CONSTANT = 100
print(MY_CONSTANT)
# Output:
# 100
In this example, MY_CONSTANT
is a constant named using UPPER_CASE_WITH_UNDERSCORES
. By following this convention, you make it clear to other developers that this value should not be changed.
Python Naming Conventions: Beyond the Basics
While the naming conventions for variables, functions, classes, and constants form the foundation, Python’s naming conventions extend to other constructs such as modules, packages, and exceptions. Let’s dive deeper and explore these conventions.
Modules and Packages
Python modules and packages should also be named using lowercase_with_underscores
format. This keeps the naming consistent with variable and function naming, making it easier to differentiate classes from modules and packages. Here’s an example of how you might name a module:
# my_module.py
def my_function():
return 'Hello, Python!'
In this example, my_module.py
is a module named using lowercase_with_underscores
. The function my_function
within the module also follows the same convention. This consistency in naming makes your code easier to navigate.
Exceptions
When it comes to exception classes in Python, they should be named using PascalCase
, and they should typically end with the word ‘Error’. This convention makes it clear that the class is an exception class, and what kind of error it represents. Here’s an example:
class MyCustomError(Exception):
pass
In this example, MyCustomError
is an exception class named using PascalCase
, and ending with ‘Error’. This naming convention makes it clear that MyCustomError
is an exception class.
By understanding and following Python’s naming conventions for these advanced constructs, you can write code that is more readable and maintainable, making your Python journey smoother and more enjoyable.
Exploring Alternative Python Naming Conventions
While Python’s PEP 8 guidelines provide a robust framework for naming conventions, there are alternative approaches that some developers prefer. One such alternative is camelCase
.
Understanding CamelCase
In camelCase
, the first letter of each word is capitalized except the first word. Unlike snake_case
, camelCase
does not use underscores to separate words. Here’s an example of camelCase
in action:
# camelCase example
myVariable = 'Hello, Python!'
def myFunction():
print(myVariable)
myFunction()
# Output:
# 'Hello, Python!'
In this example, myVariable
and myFunction
are named using camelCase
. This style is more common in languages like Java and JavaScript, but it’s not the standard in Python.
Comparing Snake_Case and CamelCase
While both snake_case
and camelCase
are easy to read, snake_case
is more common in Python due to the PEP 8 guidelines. However, camelCase
can be useful in certain situations, especially when integrating Python code with other languages that use camelCase
as the standard.
# snake_case
my_variable = 'Hello, Python!'
# camelCase
myVariable = 'Hello, Python!'
# Output:
# Both are valid and will work, but snake_case is preferred in Python.
In this example, my_variable
is named using snake_case
, and myVariable
is named using camelCase
. Both are valid and will work in Python, but snake_case
is the preferred convention according to PEP 8.
By understanding these alternative naming conventions, you can write Python code that is more flexible and adaptable to different situations.
Avoiding Common Mistakes in Python Naming Conventions
While Python’s naming conventions are straightforward, it’s easy to make mistakes or fall into misconceptions, especially for beginners. Let’s go over some common pitfalls and how to avoid them.
Misusing Uppercase and Lowercase Letters
One common mistake is misusing uppercase and lowercase letters when naming variables, functions, classes, and constants. Here’s an example of this mistake:
# Incorrect naming
My_Variable = 'Hello, Python!'
# Correct naming
my_variable = 'Hello, Python!'
# Output:
# Both will work, but 'my_variable' is the correct naming convention in Python.
In this example, My_Variable
is incorrectly named using a mix of uppercase and lowercase letters with an underscore. The correct naming convention, as shown with my_variable
, is lowercase_with_underscores
.
Overusing Short Names
Another common mistake is overusing short, vague names for variables and functions. While short names like x
or f
might save you typing time, they can make your code harder to understand.
# Incorrect naming
x = 'Hello, Python!'
f = lambda: print(x)
f()
# Correct naming
message = 'Hello, Python!'
print_message = lambda: print(message)
print_message()
# Output:
# Both will work, but 'message' and 'print_message' make the code more readable.
In this example, x
and f
are short names that don’t convey much information about their purpose. The names message
and print_message
are more descriptive and make the code more readable.
By being aware of these common mistakes and misconceptions, you can write Python code that is more readable, maintainable, and in line with Python’s naming conventions.
The Importance of Naming Conventions in Python
Naming conventions might seem like a small detail in the grand scheme of programming, but they play a crucial role in code readability and maintainability. Let’s delve into why naming conventions are so important in Python.
Enhancing Code Readability
The first and foremost benefit of following naming conventions is enhancing code readability. Imagine trying to understand a piece of code where all variables are named var1
, var2
, var3
, and so on. It would be like trying to read a book where all characters are named person1
, person2
, person3
, etc. It would be confusing, right?
# Without proper naming
a = 10
b = 20
def c():
return a + b
print(c())
# Output:
# 30
In this example, it’s hard to understand what a
, b
, and c
represent. Now, let’s look at the same piece of code with proper naming conventions:
# With proper naming
first_number = 10
second_number = 20
def add_numbers():
return first_number + second_number
print(add_numbers())
# Output:
# 30
Here, first_number
, second_number
, and add_numbers
make the code much more readable. You can easily understand that this code adds two numbers.
Improving Code Maintainability
Naming conventions also improve code maintainability. Properly named variables and functions make it easier to debug and update the code. It’s like having a well-organized toolbox where you can easily find the tool you need.
Good naming conventions are especially important when working on large projects or collaborating with other developers. They ensure that everyone can understand the code and contribute effectively.
In summary, Python’s naming conventions are not just rules to follow blindly. They are practical guidelines that make your code more readable and maintainable, ultimately making you a better Python programmer.
Python Naming Conventions in Larger Coding Projects
Python’s naming conventions don’t operate in a vacuum. They are an integral part of larger coding projects, contributing to the overall structure and readability of your code. Let’s discuss how these conventions fit into the bigger picture.
The Role of Naming Conventions in Project Structure
In larger coding projects, following Python’s naming conventions can have a significant impact on the project’s structure and readability. Consistent naming across modules, classes, functions, and variables can make the project easier to navigate and understand.
# my_module.py
# Class naming
class MyClass:
# Constant naming
MY_CONSTANT = 100
# Function naming
def my_function(self):
return self.MY_CONSTANT
In this example, MyClass
, MY_CONSTANT
, and my_function
follow Python’s naming conventions. This consistency makes the module easier to read and understand, especially in larger projects with multiple modules and classes.
Exploring Related Topics: Code Formatting and Documentation
While naming conventions are important, they are just one aspect of writing clean, readable Python code. Code formatting and documentation are equally important and are worth exploring further.
Code formatting involves how your code is laid out, including indentation, line breaks, and spaces. Python’s PEP 8 guidelines also provide recommendations for code formatting, ensuring your code is easy to read.
Documentation, on the other hand, involves writing clear comments and docstrings to explain what your code does. This is especially important in larger projects where you need to communicate your code’s purpose to other developers.
Further Resources for Mastering Python Naming Conventions
To delve deeper into Python’s naming conventions and related topics, check out the following resources:
- PEP 8 — Style Guide for Python Code: The official style guide for Python, including naming conventions and code formatting guidelines.
- Python’s Documentation Guide: A guide on how to write effective documentation in Python.
- Real Python: A comprehensive platform offering Python tutorials and articles, including topics on naming conventions, code formatting, and documentation.
Wrapping Up: Mastering Python Naming Conventions
In this comprehensive guide, we’ve delved into the depths of Python’s naming conventions, shedding light on the rules and guidelines that make Python code more readable and maintainable.
We began with the basics, learning how to name variables, functions, classes, and constants in Python. We then ventured into more advanced territory, exploring the naming conventions for other Python constructs like modules, packages, and exceptions.
Along the way, we tackled common mistakes and misconceptions about Python naming conventions, equipping you with the knowledge to write clean, professional Python code.
We also looked at alternative approaches to naming in Python, such as camelCase
, and compared them with the standard snake_case
and PascalCase
conventions.
Here’s a quick comparison of these conventions:
Convention | Use Case | Example |
---|---|---|
snake_case | Variables, functions, modules, and packages | my_variable , my_function() |
PascalCase | Classes and exceptions | MyClass , MyException |
UPPER_CASE_WITH_UNDERSCORES | Constants | MY_CONSTANT |
camelCase | Alternative for variables and functions | myVariable , myFunction() |
Whether you’re just starting out with Python or you’re looking to level up your coding style, we hope this guide has given you a deeper understanding of Python’s naming conventions and their importance in writing readable, maintainable code.
With its clear and consistent naming conventions, Python is a language that values readability and simplicity. Now, you’re well equipped to embrace these conventions in your own Python journey. Happy coding!