Spring vs Spring Boot: Which to Choose?
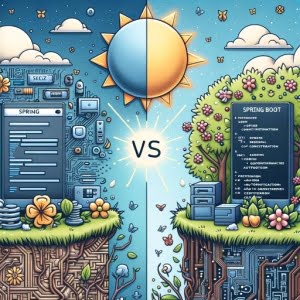
Are you finding it difficult to choose between Spring and Spring Boot? It’s a common dilemma. Like choosing between a manual and automatic car, each has its own benefits and use cases.
Think of Spring as a comprehensive toolkit for building Java applications, while Spring Boot is a module of Spring that simplifies the setup process. Spring gives you more control, but requires more setup, whereas Spring Boot is all about ease of use and speed of development.
In this guide, we’ll delve into the differences between these two popular Java frameworks and help you decide which one is right for your project.
So, let’s dive in and start exploring Spring and Spring Boot!
TL;DR: Should you choose Spring or Spring Boot?
Spring is a comprehensive framework for building Java applications
, whileSpring Boot is a module of Spring that simplifies the setup process
. The differences are defined in Class/Object Creation. Spring requires more setup, for example:Car car = new Car();
is needed as well as necessary setters,car.setEngine(new Engine());
andcar.setWheels(new Wheels());
. While Spring Boot is more streamlined,Car car = new Car(new AutomaticDrivingFeatures());
. If you want more control and don’t mind more setup, go for Spring. If you prefer ease of use and faster development, choose Spring Boot.
Here’s a simple analogy:
// Spring is like manual car driving
Car car = new Car();
car.setEngine(new Engine());
car.setWheels(new Wheels());
car.drive();
// Spring Boot is like automatic car driving
Car car = new Car(new AutomaticDrivingFeatures());
car.drive();
// Output:
// Both cars will drive, but the Spring Boot car requires less setup.
In this example, we can see that both Spring and Spring Boot will get the job done. However, Spring Boot requires less setup, making it a more convenient option for many developers.
This is just a basic comparison between Spring and Spring Boot. There’s much more to learn about these two powerful Java frameworks. Continue reading for a more detailed comparison and advanced usage scenarios.
Table of Contents
- Setting Up a Simple Project in Spring and Spring Boot
- Delving Deeper: Advanced Features in Spring and Spring Boot
- Exploring Alternatives: Other Java Frameworks
- Troubleshooting Common Issues in Spring and Spring Boot
- Understanding Java Frameworks
- The Evolution of Spring and Spring Boot
- Spring and Spring Boot in the Larger Java Ecosystem
- Further Resources for Spring and Spring Boot
- Wrapping Up: Spring vs Spring Boot
Setting Up a Simple Project in Spring and Spring Boot
Spring Project Setup
With Spring, setting up a project involves a few more steps. Here’s an example of setting up a simple Spring project:
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
MyComponent myComponent = context.getBean(MyComponent.class);
myComponent.doStuff();
context.close();
}
}
// Output:
// 'Doing stuff...'
In this example, we’re creating an application context and getting a bean from it. We then call a method on the bean. It’s a simple operation, but setting it up requires an understanding of the Spring framework.
Spring Boot Project Setup
Now, let’s look at setting up a similar project in Spring Boot:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Main {
public static void main(String[] args) {
SpringApplication.run(Main.class, args);
}
}
// Output:
// 'Application starts running...'
In the Spring Boot example, all we need to do is add the @SpringBootApplication
annotation and call SpringApplication.run()
. The rest is handled by Spring Boot.
Pros and Cons
While Spring offers more control, it also means more manual configuration. On the other hand, Spring Boot’s auto-configuration feature simplifies the setup process, making it a better choice for beginners or for those who prefer a faster development cycle.
Delving Deeper: Advanced Features in Spring and Spring Boot
Dependency Injection in Spring and Spring Boot
Dependency Injection (DI) is a core feature of both Spring and Spring Boot. It allows you to inject dependencies into your components, making them easier to test and maintain.
Here’s an example of DI in Spring:
@Component
public class MyComponent {
private final MyDependency myDependency;
@Autowired
public MyComponent(MyDependency myDependency) {
this.myDependency = myDependency;
}
public void doStuff() {
myDependency.doStuff();
}
}
// Output:
// 'Doing stuff with dependency...'
In this example, MyDependency
is injected into MyComponent
via the constructor, which is annotated with @Autowired
.
Spring Boot handles DI in a similar way, but with a key difference: it automatically injects beans where they’re needed, so you don’t need to use the @Autowired
annotation.
Aspect-Oriented Programming in Spring and Spring Boot
Aspect-Oriented Programming (AOP) is another powerful feature provided by both Spring and Spring Boot. It allows you to define cross-cutting concerns in one place, improving code modularity.
Here’s an example of AOP in Spring:
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.MyComponent.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature());
}
}
// Output:
// 'Before method: com.example.MyComponent.doStuff'
In this example, LoggingAspect
logs a message before every method in MyComponent
.
Spring Boot supports AOP in the same way as Spring, but it also provides auto-configuration for AspectJ, making it easier to use.
Auto-Configuration in Spring and Spring Boot
Auto-configuration is a feature that sets up your project based on the dependencies in your classpath. While Spring does provide some auto-configuration, it’s a standout feature of Spring Boot.
In Spring Boot, you can control auto-configuration using properties in the application.properties
or application.yml
file. For example, you can change the server port like this:
server:
port: 8081
# Output:
# The application will start on port 8081
This level of simplicity and convenience is what makes Spring Boot a popular choice for many developers.
Exploring Alternatives: Other Java Frameworks
While Spring and Spring Boot are popular choices for Java application development, they’re not the only options. Let’s take a look at some alternative Java frameworks: Java EE, Micronaut, and Quarkus.
Java EE: The Standard
Java EE, or Jakarta EE as it’s now known, is the standard for enterprise Java. It offers a wide range of features for building large-scale applications.
Here’s an example of a simple Java EE application:
@Stateless
public class MyBean {
public void doStuff() {
System.out.println("Doing stuff...");
}
}
// Output:
// 'Doing stuff...'
In this example, we’re defining a stateless session bean that performs a simple operation. Java EE offers a lot of control, but it can also be complex and heavyweight.
Micronaut: The Speedy Contender
Micronaut is a modern, JVM-based, full-stack framework for building modular, easily testable microservice applications.
@Controller("/hello")
public class HelloController {
@Get("/world")
public String helloWorld() {
return "Hello, World";
}
}
// Output:
// 'Hello, World'
In this Micronaut example, we’re defining a simple controller that returns a greeting. Micronaut is designed for speed and minimal memory footprint, making it a good choice for microservices.
Quarkus: The Supersonic Subatomic Java
Quarkus is a Kubernetes-native Java framework tailored for GraalVM and HotSpot, crafted from best-of-breed Java libraries and standards.
@Path("/hello")
public class GreetingResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "hello";
}
}
// Output:
// 'hello'
In this Quarkus example, we’re defining a simple JAX-RS resource that returns a greeting. Quarkus aims to make Java a leading platform in Kubernetes and serverless environments while offering developers a unified reactive and imperative programming model.
When deciding between these frameworks, consider your project’s requirements, your team’s knowledge, and the specific features and benefits of each framework. Remember, the best tool is the one that best fits your needs.
Troubleshooting Common Issues in Spring and Spring Boot
Configuration Problems
One common issue developers face when working with Spring and Spring Boot is configuration problems. These can be due to incorrect property settings, missing dependencies, or incompatible versions.
For example, if you’re missing a required property in your application.properties
file, you might see an error like this:
Caused by: java.lang.IllegalArgumentException: Could not resolve placeholder 'my.property' in value "${my.property}"
In this case, you need to ensure that my.property
is correctly defined in your properties file.
Dependency Issues
Dependency issues are another common problem. These can occur when you have conflicting versions of the same dependency, or when a required dependency is missing.
Here’s an example of a dependency issue:
Caused by: org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type 'com.example.MyComponent' available
This error means that Spring couldn’t find a bean of type MyComponent
. To fix this, you need to ensure that MyComponent
is correctly defined and annotated with @Component
or another stereotype annotation.
Performance Considerations
Finally, performance can be a consideration when using Spring and Spring Boot. While both frameworks are designed to be efficient, improper use can lead to performance issues.
For example, using @Autowired
on a field or method can lead to tight coupling and make your code harder to test. Instead, consider using constructor injection, which is more flexible and testable.
@Component
public class MyComponent {
private final MyDependency myDependency;
public MyComponent(MyDependency myDependency) {
this.myDependency = myDependency;
}
}
In this example, we’re injecting MyDependency
via the constructor, which makes MyComponent
easier to test and less coupled to MyDependency
.
When troubleshooting issues in Spring and Spring Boot, remember to read the error messages carefully, understand the concepts behind the frameworks, and always consider the impact of your decisions on performance and maintainability.
Understanding Java Frameworks
Java frameworks play a crucial role in application development. They provide a pre-built structure and set of guidelines that developers can follow to build applications more efficiently and effectively.
A Java framework can include APIs, tools, and libraries that help with tasks like database access, web page templating, and session management. By using a framework, developers can focus more on their application’s unique functionality and less on boilerplate code.
The Evolution of Spring and Spring Boot
The Birth of Spring
Spring was first released in 2002 as a response to the complexity of the early Java Enterprise Edition (J2EE) specifications. Its goal was to simplify enterprise Java development and improve testability and scalability.
// A simple Spring application
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
MyComponent myComponent = context.getBean(MyComponent.class);
myComponent.doStuff();
context.close();
// Output:
// 'Doing stuff...'
In this example, we’re creating a simple Spring application. The AnnotationConfigApplicationContext
is a Spring container that manages the lifecycle of the beans. The MyComponent
bean is retrieved from the context and its doStuff
method is called.
The Emergence of Spring Boot
Spring Boot, first released in 2014, was developed to simplify the setup and configuration of Spring applications. It uses a ‘convention over configuration’ approach, providing sensible defaults that can be overridden as needed.
// A simple Spring Boot application
@SpringBootApplication
public class Main {
public static void main(String[] args) {
SpringApplication.run(Main.class, args);
}
}
// Output:
// 'Application starts running...'
In this example, we’re creating a simple Spring Boot application. The @SpringBootApplication
annotation enables auto-configuration and component scanning. The SpringApplication.run()
method launches the application.
Spring Boot’s simplicity and productivity have made it incredibly popular among developers, and it’s now considered a standard for building Spring applications.
Spring and Spring Boot in the Larger Java Ecosystem
The Bigger Picture: Microservices and Cloud-Native Java
Spring and Spring Boot are more than just standalone frameworks. They’re part of a larger ecosystem that includes other projects under the Spring umbrella, such as Spring Cloud and Spring Security, and they play a crucial role in modern application architectures like microservices and cloud-native Java.
// A simple Spring Boot microservice
@RestController
public class HelloController {
@RequestMapping("/hello")
public String hello() {
return "Hello, World";
}
}
// Output:
// 'Hello, World'
In this example, we’re creating a simple microservice with Spring Boot. The @RestController
and @RequestMapping
annotations define a RESTful endpoint that returns a greeting.
Embracing Reactive Programming
Another important trend in the Java world is reactive programming, which is a programming paradigm that deals with asynchronous data streams and the propagation of change. Spring 5 introduced a new module called Spring WebFlux to bring reactive programming to Spring applications.
// A simple Spring WebFlux controller
@RestController
public class HelloController {
@GetMapping("/hello")
public Mono<String> hello() {
return Mono.just("Hello, World");
}
}
// Output:
// 'Hello, World'
In this example, we’re creating a reactive endpoint with Spring WebFlux. The Mono
type represents a single value (or no value) that will be provided asynchronously.
Further Resources for Spring and Spring Boot
If you want to delve deeper into Spring Boot, Click Here for a quick start guide!
Furthermore, we have gathered some additional Spring resources that you might find helpful:
- Spring Bean: Basics – Explore Spring beans for managing components.
Exploring Spring Boot Actuator Features – Master Actuator configuration for effective app management.
Spring Guides – Official guides that cover topics from getting started with Spring Boot to building microservices.
Baeldung is a blog that publishes high-quality articles on Java and Spring, among other topics.
Spring Boot in Action provides a comprehensive introduction to Spring Boot.
Wrapping Up: Spring vs Spring Boot
In this detailed guide, we’ve explored the intricacies of Spring and Spring Boot, two popular Java frameworks. We’ve examined their respective strengths and weaknesses, and provided a roadmap for choosing the right one for your project.
We began with the basics, understanding the core differences between Spring and Spring Boot. We then delved into the practical aspects, with step-by-step guides on setting up a simple project in both frameworks. We ventured further into more advanced features, discussing dependency injection, aspect-oriented programming, and auto-configuration. We also highlighted some common issues developers might face when using these frameworks, and provided solutions for each.
We broadened our scope to look at the larger Java ecosystem, discussing alternative Java frameworks like Java EE, Micronaut, and Quarkus. Here’s a quick comparison of these frameworks:
Framework | Control | Ease of Use | Speed of Development |
---|---|---|---|
Spring | High | Moderate | Moderate |
Spring Boot | Moderate | High | High |
Java EE | High | Low | Low |
Micronaut | High | High | High |
Quarkus | High | High | High |
Whether you’re just starting out with Spring and Spring Boot or you’re an experienced developer looking to make an informed choice for your next project, we hope this guide has provided you with the insights you need.
Understanding the nuances of Spring and Spring Boot can empower you to make the most of these powerful Java frameworks. Happy coding!