Axios NPM Guide | Installation and Advanced Use
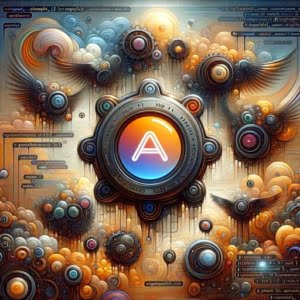
At IOFLOOD, we’ve encountered the challenge of effectively integrating Axios with npm more often than we’d like. That’s why we’ve put together a practical guide on using Axios with npm. By following our straightforward instructions, you’ll seamlessly incorporate Axios into your projects, empowering you to handle HTTP requests with confidence.
This guide will walk you through installing Axios with npm and harnessing its full potential. We’ll start from the ground up, covering everything from simple HTTP requests to advanced Axios features. By the end, you’ll see just how Axios can make your web applications more dynamic and responsive, making your development process smoother and more efficient.
Let’s simplify the integration of Axios and npm together!
TL;DR: How Do I Use Axios with npm?
To use Axios in your project, start by installing it via npm with the command
npm install axios
. Below is a quick example of making a GET request:
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => console.log(response.data))
.catch(error => console.error(error));
# Output:
# {"userId": 1, "id": 1, "title": "Example Data", "completed": false}
In this example, we’re using Axios to make a simple GET request to https://api.example.com/data
. Upon success, it logs the response data to the console, and in case of an error, it catches and logs the error. This demonstrates the basic use of Axios for making HTTP requests, handling responses, and managing errors.
Ready to dive deeper into Axios’s capabilities? Continue reading for more detailed instructions, advanced features, and troubleshooting tips.
Table of Contents
Installing Axios with npm
Embarking on your journey with Axios begins with its installation. Axios is a promise-based HTTP client for the browser and node.js, making it a versatile choice for developers. To install Axios, you’ll need npm (Node Package Manager), which comes with Node.js. If you haven’t already, download and install Node.js from its official website, which will automatically install npm.
Once Node.js and npm are set up, open your terminal or command prompt and run the following command:
npm install axios
This command tells npm to download the Axios package and add it to your project, making it available for use.
Making a GET Request
After installing Axios, you’re ready to make HTTP requests. A common task is fetching data from a server using a GET request. Here’s how you can do it with Axios:
const axios = require('axios');
axios.get('https://jsonplaceholder.typicode.com/todos/1')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
# Output:
# {"userId": 1, "id": 1, "title": "delectus aut autem", "completed": false}
In this example, we’re requesting data from a JSON placeholder service, a free fake API for testing and prototyping. The .get
method of Axios is used to send a GET request to the specified URL. Upon success, the then
method captures the response, logging the data to the console. In case of an error, the catch
method catches and logs the error, demonstrating Axios’s built-in error handling capabilities.
Making a POST Request
Sending data to a server is another common use case. This is typically done using a POST request. Here’s an example of how to send data with Axios:
const axios = require('axios');
axios.post('https://jsonplaceholder.typicode.com/posts', {
title: 'Axios with npm',
body: 'Learning Axios',
userId: 1
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
# Output:
# {"id": 101, "title": "Axios with npm", "body": "Learning Axios", "userId": 1}
This POST request example sends a new post to the JSON placeholder service. The .post
method is used here, with the URL of the service and an object containing the data to be sent. The response, upon success, includes the data sent to the server, demonstrating how Axios can be used to easily manage POST requests and handle server responses.
Axios Advanced Features
As you grow more comfortable with Axios for your HTTP requests, you’ll find that its advanced features can significantly enhance your web applications. Let’s delve into some of these features, such as interceptors, creating instances, and configuring global defaults, to see how they can streamline your workflow and make your code more efficient.
Utilizing Interceptors
Interceptors are a powerful feature in Axios that allow you to run your code or alter the request and response before the then or catch blocks are executed. This can be particularly useful for logging, authentication, or any repetitive task for requests and responses.
axios.interceptors.request.use(config => {
// Perform actions before request is sent
console.log('Request was sent');
return config;
}, error => {
// Do something with request error
return Promise.reject(error);
});
# Output:
# 'Request was sent'
In this example, an interceptor is added to log a message every time a request is sent. This is a simple demonstration of how interceptors can be used to perform actions before requests are processed.
Creating Instances
Creating instances of Axios can simplify the management of API calls by allowing you to pre-configure settings for a specific API.
const apiClient = axios.create({
baseURL: 'https://api.example.com',
timeout: 1000,
headers: {'X-Custom-Header': 'foobar'}
});
# Output:
# AxiosInstance {defaults: {...}, interceptors: {...}, ...}
This code snippet demonstrates creating an Axios instance with a base URL, timeout, and custom headers. Using instances can make your code cleaner and more reusable, especially when dealing with multiple endpoints or configurations.
Configuring Global Defaults
Axios allows you to configure global defaults for your requests, which is particularly useful when you have common configurations across your application.
axios.defaults.baseURL = 'https://api.example.com';
axios.defaults.headers.common['Authorization'] = AUTH_TOKEN;
# Output:
# Axios global defaults set
By setting global defaults, you ensure consistency in your requests, reducing the need to repeatedly set the same configurations. This example sets a base URL and a common header for authorization, illustrating how global defaults can streamline your Axios usage.
In summary, Axios’s advanced features like interceptors, instances, and global defaults provide powerful tools for optimizing your HTTP requests. By leveraging these features, you can make your code more efficient, maintainable, and scalable.
Axios vs. Fetch API vs. SuperAgent
When it comes to making HTTP requests in JavaScript, Axios isn’t the only game in town. The Fetch API and SuperAgent are two other popular choices, each with its own set of advantages and use cases. Understanding the differences can help you choose the right tool for your project.
Fetch API: The Native Option
The Fetch API is a native browser API for making HTTP requests. It’s promise-based, like Axios, but it’s built into modern browsers, eliminating the need to include an external library.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Fetch error:', error));
# Output:
# {"data": "Sample fetch API response"}
This example demonstrates making a GET request using the Fetch API. The simplicity of Fetch is apparent, but unlike Axios, it doesn’t automatically transform JSON data in the response, requiring an additional step to parse the response.
SuperAgent: The Fluent API
SuperAgent is another library that emphasizes a fluent and chainable API. It’s often praised for its readability and straightforward syntax.
const request = require('superagent');
request.get('https://api.example.com/data')
.then(response => {
console.log(response.body);
})
.catch(error => {
console.error('SuperAgent error:', error);
});
# Output:
# {"data": "Sample SuperAgent response"}
In this SuperAgent example, a GET request is made, showcasing its easy-to-understand syntax. SuperAgent, like Axios, handles JSON data automatically, making it a strong alternative for API interactions.
Performance Considerations and Use Cases
When choosing between Axios, Fetch API, and SuperAgent, consider factors like performance, browser support, and feature set. Axios and SuperAgent provide more out-of-the-box features, such as automatic JSON transformation and request cancellation, which can be advantageous for complex applications. The Fetch API, being a native browser feature, offers a lightweight alternative without the need for external libraries, making it ideal for projects with minimal dependencies.
In conclusion, the choice between Axios, Fetch API, and SuperAgent depends on your project’s specific needs and environment. Each option has its strengths, and understanding these can guide you to the most suitable tool for making HTTP requests in your JavaScript projects.
Axios Troubleshooting Guide
Even with a tool as powerful and versatile as Axios, developers might encounter issues that can hinder their project’s progress. Understanding how to troubleshoot these common problems is crucial. This section will cover handling errors, dealing with timeouts, and managing request cancellations, providing solutions and best practices to keep your projects running smoothly.
Handling Errors Gracefully
Error handling is a critical aspect of working with HTTP requests. Axios provides a straightforward way to catch and handle errors, whether they are due to network issues, server problems, or incorrect configurations.
axios.get('https://api.example.com/nonexistent')
.catch(error => {
if (error.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.error(error.response.data);
console.error(error.response.status);
console.error(error.response.headers);
} else if (error.request) {
// The request was made but no response was received
console.error(error.request);
} else {
// Something happened in setting up the request that triggered an Error
console.error('Error', error.message);
}
});
# Output:
# 'Error 404: Not Found'
In this example, an attempt to access a non-existent resource results in an error. Axios’s error handling allows you to differentiate between various types of errors, enabling more precise troubleshooting and user feedback.
Managing Timeouts
Timeouts are another common issue, particularly when dealing with slow networks or unresponsive servers. Setting a timeout for your requests can prevent your application from hanging indefinitely.
axios.get('https://api.example.com/data', { timeout: 5000 })
.then(response => console.log(response.data))
.catch(error => console.error('Timeout exceeded', error));
# Output:
# 'Timeout exceeded Error: timeout of 5000ms exceeded'
Here, a GET request is made with a timeout of 5000 milliseconds. If the request takes longer than this, Axios throws a timeout error, allowing your application to handle the situation appropriately, instead of waiting indefinitely for a response.
Request Cancellation
There may be scenarios where you need to cancel a request after it has been sent. Axios provides a mechanism for request cancellation, which can be especially useful in applications with dynamic user interfaces.
const CancelToken = axios.CancelToken;
const source = CancelToken.source();
axios.get('https://api.example.com/data', { cancelToken: source.token })
.catch(function (thrown) {
if (axios.isCancel(thrown)) {
console.log('Request canceled', thrown.message);
} else {
// handle error
}
});
// Cancel the request
source.cancel('Operation canceled by the user.');
# Output:
# 'Request canceled Operation canceled by the user.'
This snippet demonstrates initiating a request with a cancellation token and then canceling it. This feature is invaluable for improving user experience by stopping unnecessary network activity when a user navigates away from a page or changes their mind.
In summary, mastering Axios involves not just leveraging its features for making requests but also understanding how to troubleshoot common issues effectively. By handling errors gracefully, managing timeouts, and utilizing request cancellation, you can ensure your applications are robust and user-friendly.
Understanding HTTP Requests
Before diving deep into Axios and its capabilities, it’s crucial to grasp the basics of HTTP requests and how they form the backbone of client-server communication. At its core, an HTTP request is a structured way for clients (like web browsers or applications) to communicate with servers. It’s what happens when you type a URL into your browser or when an app fetches data from a remote source.
The Role of Promises in JavaScript
JavaScript’s asynchronous nature is both a powerful feature and a complexity source, especially when dealing with HTTP requests. This is where promises come into play. A promise in JavaScript represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
new Promise((resolve, reject) => {
const dataLoaded = true; // Simulate data loading
if (dataLoaded) {
resolve('Data loaded successfully');
} else {
reject('Data loading failed');
}
}).then(message => {
console.log(message);
}).catch(error => {
console.error(error);
});
# Output:
# 'Data loaded successfully'
In this example, a promise is created that simulates the loading of data. If dataLoaded
is true, the promise is resolved with a success message. If not, it’s rejected with an error message. This illustrates how promises allow for handling both successful and unsuccessful operations in asynchronous tasks.
Axios: Leveraging HTTP and Promises
Axios stands out by combining the power of HTTP requests with the flexibility of promises. It simplifies making requests and processing responses, whether success or failure. Here’s a basic example of using Axios to make an HTTP GET request:
axios.get('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
# Output:
# {"userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit
suscipit recusandae consequuntur expedita et cum
reprehenderit molestiae ut ut quas totam
nostrum rerum est autem sunt rem eveniet architecto"}
This code block demonstrates making a simple GET request to fetch a specific post from a placeholder API. The .then()
method processes the response upon success, logging the returned data. In case of an error, the .catch()
method catches and logs it. This example underscores Axios’s ability to seamlessly integrate with JavaScript’s promises, providing a straightforward and efficient way to handle asynchronous HTTP requests.
In essence, understanding the fundamentals of HTTP requests and promises is key to appreciating how Axios enhances client-server communication. By leveraging these concepts, Axios offers a robust solution for making and managing HTTP requests in JavaScript applications.
Web Applications Utilizing Axios
Axios is not just a tool for making simple HTTP requests; it’s a powerful library that can be seamlessly integrated into larger projects, including those using modern JavaScript frameworks like React and Vue. Its promise-based nature makes it an ideal choice for managing API interactions and state in these applications.
Axios in React Applications
React, a popular JavaScript library for building user interfaces, often requires data to be fetched from a server. Axios simplifies this process. Here’s an example of using Axios within a React component to fetch data:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
function App() {
const [data, setData] = useState(null);
useEffect(() => {
axios.get('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
setData(response.data);
})
.catch(error => console.error('Fetching error:', error));
}, []);
return (
<div>
<h1>Post Title: {data?.title}</h1>
<p>{data?.body}</p>
</div>
);
}
export default App;
# Output:
# Post Title: sunt aut facere repellat provident occaecati excepturi optio reprehenderit
# quia et suscipit
# suscipit recusandae consequuntur expedita et cum
# reprehenderit molestiae ut ut quas totam
# nostrum rerum est autem sunt rem eveniet architecto
This example demonstrates how Axios can be used within React to fetch data and update the component’s state, leading to a dynamic user interface that displays data from a server.
Axios in Vue Applications
Vue.js, another popular framework, also benefits from Axios for API interactions. Vue’s reactive data system pairs well with Axios, enabling developers to easily fetch, display, and manage data.
import Vue from 'vue';
import axios from 'axios';
new Vue({
el: '#app',
data: {
post: null
},
mounted() {
axios.get('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
this.post = response.data;
})
.catch(error => console.error('Error:', error));
}
});
# Output:
# Post Title: sunt aut facere repellat provident occaecati excepturi optio reprehenderit
# quia et suscipit
# suscipit recusandae consequuntur expedita et cum
# reprehenderit molestiae ut ut quas totam
# nostrum rerum est autem sunt rem eveniet architecto
In this Vue example, Axios is used to fetch data once the component is mounted, updating the Vue instance’s data property and thus the UI.
Further Resources for Mastering Axios
To deepen your understanding of Axios and its integration with modern web development frameworks, here are three valuable resources:
- Axios Documentation – Check out the official Axios docs for a comprehensive guide to its features.
React with Axios Tutorial – Info on integrating Axios with React for efficient fetching of data and managing state.
Vue.js Axios Integration – Learn to effectively use Axios in Vue applications for efficient data fetching and state management.
Recap: Mastering Axios with npm
In this comprehensive guide, we’ve explored the ins and outs of using Axios with npm, a journey that began with the basics of installation and culminated in leveraging advanced features for more dynamic and responsive web applications.
We began by detailing how to install Axios using npm, a process that opens the door to making HTTP requests in your JavaScript projects. We navigated through the creation of simple GET and POST requests, illustrating how Axios simplifies interacting with APIs and handling server responses.
Moving on, we dove into the advanced capabilities of Axios, such as utilizing interceptors for pre-processing requests and responses, creating instances for managing API calls with specific configurations, and setting global defaults to streamline your project’s Axios usage.
We also compared Axios with alternative HTTP request libraries like the Fetch API and SuperAgent, highlighting performance considerations and scenarios where one might be preferred over the others. This comparison underscores the importance of choosing the right tool based on your project’s specific needs and environment.
Feature | Axios | Fetch API | SuperAgent |
---|---|---|---|
Ease of Use | High | Moderate | High |
Browser Support | All (with polyfill for older browsers) | Modern browsers | All |
Automatic JSON Transformation | Yes | No | Yes |
Interceptors | Yes | No | Yes |
As we wrap up, it’s clear that Axios offers a robust solution for making HTTP requests in JavaScript, providing a balance of ease of use, flexibility, and functionality. Whether you’re just starting out or looking to enhance your web applications with advanced HTTP request capabilities, Axios with npm is a powerful tool in your development arsenal.
With the skills and knowledge you’ve gained from this guide, you’re now well-equipped to harness the full potential of Axios in your projects. Happy coding!