Python json.dumps() | Step-by-Step Guide
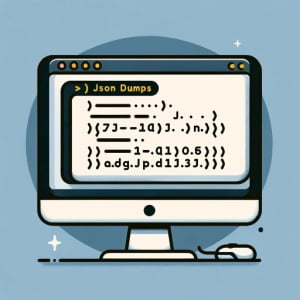
Struggling to work with JSON data in Python? You’re not alone. JSON, or JavaScript Object Notation, is a popular data format used widely in web services, data storage, and data interchange. But, when it comes to Python, dealing with JSON can be a bit tricky.
Enter Python’s json.dumps() function. Like a skilled translator, it can convert your Python objects into JSON format with ease.
Whether you’re a beginner just starting out or an experienced developer looking for advanced techniques, this comprehensive guide will walk you through the ins and outs of using json.dumps() in Python.
So, let’s dive in and master this powerful function together.
TL;DR: How Do I Use json.dumps() in Python?
The json.dumps() function is used to convert Python objects into JSON strings. Here’s a simple example:
import json
# Define a Python dictionary
data = {'name': 'John', 'age': 30}
# Use json.dumps() to convert the dictionary into a JSON string
json_data = json.dumps(data)
# Print the JSON string
print(json_data)
# Output:
# '{"name": "John", "age": 30}'
In this example, we first define a Python dictionary named ‘data’. We then use the json.dumps() function to convert this dictionary into a JSON string, which we store in the variable ‘json_data’. Finally, we print ‘json_data’, which outputs the JSON string.
For more detailed information and advanced usage scenarios, continue reading this guide.
Table of Contents
Python json.dumps(): Basic Use
The json.dumps() function in Python is a part of the json module, which provides a method to convert Python objects into their JSON string representation. Let’s break down its basic usage with a simple Python object – a dictionary.
import json
# Define a Python dictionary
data = {'name': 'John', 'age': 30}
# Use json.dumps() to convert the dictionary into a JSON string
json_data = json.dumps(data)
# Print the JSON string
print(json_data)
# Output:
# '{"name": "John", "age": 30}'
In this example, we first import the json module. We then define a Python dictionary named ‘data’. The json.dumps() function is then called with ‘data’ as the argument, converting the dictionary into a JSON string. This JSON string is stored in the ‘json_data’ variable and printed out.
The advantage of json.dumps() is its simplicity. With just a single line of code, we can convert complex Python objects into a universally accepted data format. However, it’s worth noting that json.dumps() only works with objects that are serializable. Non-serializable types like custom classes or functions will throw a TypeError.
In the next section, we’ll dive into more complex scenarios with json.dumps(), so stay tuned!
json.dumps(): Handling Complex Python Objects
While json.dumps() works seamlessly with simple Python objects like dictionaries, its true strength lies in handling more complex structures. It can handle lists, nested dictionaries, and other complex Python objects with ease. Let’s illustrate this with an example.
import json
# Define a complex Python object
data = {
'name': 'John',
'age': 30,
'pets': ['Dog', 'Cat'],
'profile': {
'job': 'Engineer',
'city': 'New York'
}
}
# Use json.dumps() to convert the object into a JSON string
json_data = json.dumps(data)
# Print the JSON string
print(json_data)
# Output:
# '{"name": "John", "age": 30, "pets": ["Dog", "Cat"], "profile": {"job": "Engineer", "city": "New York"}}'
In this example, ‘data’ is a more complex Python object, containing a list and a nested dictionary. Despite the complexity, json.dumps() handles it smoothly, converting the entire structure into a single JSON string.
The key to using json.dumps() effectively with complex objects lies in understanding Python’s data structures and how they map to JSON. Python lists become JSON arrays, dictionaries become JSON objects, and so on. However, remember that json.dumps() can only serialize data that can be represented in JSON. For instance, Python sets, which don’t have a JSON equivalent, cannot be serialized.
In the next section, we’ll explore alternative approaches to json.dumps(), so stick around!
Exploring Alternatives to json.dumps()
While json.dumps() is incredibly useful for converting Python objects to JSON strings, there are other methods you can use, especially when dealing with larger data or file operations. One such method is the json.dump() function.
Writing JSON to Files with json.dump()
The json.dump() function is similar to json.dumps(), but instead of converting the Python object to a JSON string, it writes the JSON data directly to a file. Here’s an example:
import json
# Define a Python dictionary
data = {'name': 'John', 'age': 30}
# Use json.dump() to write the dictionary into a JSON file
with open('data.json', 'w') as f:
json.dump(data, f)
# Verify the contents of the file
with open('data.json', 'r') as f:
print(f.read())
# Output:
# '{"name": "John", "age": 30}'
In this example, we first define a Python dictionary named ‘data’. We then open a file named ‘data.json’ in write mode. Using json.dump(), we write the dictionary directly into the file. Finally, we open the file in read mode and print its contents to verify.
The advantage of json.dump() is that it allows for efficient handling of large data. Instead of loading the entire JSON string into memory, it writes the data directly to a file. However, it’s less flexible than json.dumps() because it can’t be used for operations that require a JSON string.
Here’s a quick comparison of json.dumps() and json.dump():
Method | Advantage | Disadvantage |
---|---|---|
json.dumps() | Returns a JSON string which can be used in various ways | Can consume more memory with large data |
json.dump() | Efficiently handles large data by writing directly to a file | Less flexible, only writes to a file |
In the end, the choice between json.dumps() and json.dump() depends on your specific use case. For operations that require a JSON string, json.dumps() is the way to go. For dealing with larger data or writing directly to a file, json.dump() is a better choice.
Troubleshooting Python json.dumps()
While json.dumps() is a powerful function, you may encounter some common issues during the conversion process. Let’s discuss these potential pitfalls and how to navigate them.
Handling Non-Serializable Types
As mentioned earlier, json.dumps() can only serialize data that can be represented in JSON. Python sets, custom classes, or functions are non-serializable types. Trying to serialize these will throw a TypeError. Here’s an example:
import json
# Define a Python set
my_set = {1, 2, 3}
# Attempt to use json.dumps() on the set
try:
json_data = json.dumps(my_set)
except TypeError as e:
print(f'An error occurred: {e}')
# Output:
# An error occurred: Object of type set is not JSON serializable
In this example, we define a Python set ‘my_set’ and attempt to use json.dumps() on it. This results in a TypeError, as sets are not serializable.
One workaround for this issue is to convert the non-serializable type to a serializable type before using json.dumps(). For instance, you can convert a set to a list, which is serializable.
Dealing with Special Characters
Special characters in a Python string can cause issues when converting to JSON. For example, backslashes in a string will be escaped in the JSON output, which might not be the desired result. Here’s an example:
import json
# Define a Python string with a backslash
data = 'C:\Users\John'
# Use json.dumps() to convert the string into a JSON string
json_data = json.dumps(data)
# Print the JSON string
print(json_data)
# Output:
# 'C:\\Users\\John'
In this example, the backslashes in the Python string ‘data’ are escaped in the JSON string, resulting in double backslashes. To prevent this, you can use raw strings in Python by prefixing the string with an ‘r’.
Understanding these issues and their solutions can help you use json.dumps() more effectively. In the next section, we’ll dive into the fundamentals of Python’s json module and JSON data format.
Python’s json Module and JSON Data Format
To fully grasp the power of json.dumps(), it’s crucial to understand the fundamentals of Python’s json module and the JSON data format.
Python’s json Module
Python’s json module provides methods to process JSON data. It allows for encoding Python objects into JSON strings with functions like json.dumps() and json.dump(). Conversely, it can decode JSON data into Python objects using functions like json.loads() and json.load().
JSON Data Format
JSON, or JavaScript Object Notation, is a lightweight data interchange format that’s easy for humans to read and write and easy for machines to parse and generate. It’s based on a subset of JavaScript and is a text format that’s completely language-independent.
Python Data Types and JSON
Python’s data types map to JSON and vice versa, as shown below:
Python | JSON |
---|---|
dict | Object |
list, tuple | Array |
str | String |
int, long, float | Number |
True | true |
False | false |
None | null |
json.dumps() vs json.dump()
While json.dumps() and json.dump() both convert Python objects into JSON, they serve different purposes. json.dumps() returns a JSON string representing a Python object, while json.dump() writes a Python object to a JSON file.
import json
# json.dumps() example
data = {'name': 'John', 'age': 30}
json_data = json.dumps(data)
print(json_data)
# Output:
# '{"name": "John", "age": 30}'
# json.dump() example
with open('data.json', 'w') as f:
json.dump(data, f)
# Check the contents of 'data.json' file
with open('data.json', 'r') as f:
print(f.read())
# Output:
# '{"name": "John", "age": 30}'
In the json.dumps() example, we convert a Python dictionary into a JSON string and print it. In the json.dump() example, we write the Python dictionary directly to a file named ‘data.json’.
Understanding these fundamentals provides a strong foundation for mastering Python’s json.dumps() function. In the next section, we’ll discuss the relevance of Python to JSON conversion in various fields.
The Power of Python to JSON Conversion
Python’s ability to convert its objects into JSON format using json.dumps() is not just a technical trick. It has profound implications in various fields, particularly web development and data analysis.
Python and JSON in Web Development
In web development, JSON is the de facto standard for data interchange between the client and the server. It’s lightweight, easy to understand, and works seamlessly with JavaScript, the language of the web. Python’s ability to convert its objects into JSON format means Python-based back-end systems can communicate effectively with front-end JavaScript code.
Python and JSON in Data Analysis
In data analysis, JSON is often used to store and exchange data. Python’s json.dumps() function allows data analysts to convert complex Python data structures into JSON format, which can then be stored, shared, or analyzed.
Diving Deeper: APIs and Data Serialization
The power of Python’s json.dumps() function extends beyond just converting Python objects into JSON strings. It’s a key part of working with APIs, which often use JSON for data interchange. It’s also crucial for data serialization, the process of converting data into a format that can be stored or transmitted and then reconstructed later.
Further Resources for JSON and Python APIs
For those interested in exploring these concepts further, there are numerous resources available. We have gathered a few here:
- Explore Python’s JSON Module and discover how to convert Python objects to JSON format and vice versa.
Parsing JSON in Python with json.loads() – Dive into the world of JSON deserialization with Python’s “json.loads.”
Efficient YAML Parsing with Python – Learn how to process YAML files and extract meaningful data in Python.
Consult the Official Python JSON Module Documentation for in-depth understanding of the JSON module.
Python JSON Guide from W3Schools on manipulating and processing JSON data with Python.
Python JSON tutorials on on Real Python covers handling JSON data in Python.
In the next section, we’ll summarize everything we’ve learned about Python’s json.dumps() function.
Python json.dumps(): A Recap
Throughout this guide, we’ve explored the powerful json.dumps() function in Python, a key tool for converting Python objects into JSON strings. From simple dictionaries to complex nested structures, json.dumps() can handle a wide range of Python objects.
We’ve also discussed common issues you might encounter when using json.dumps(), such as handling non-serializable types and special characters, and provided solutions for these problems.
Furthermore, we’ve looked at alternative methods for converting Python objects to JSON, such as the json.dump() function for writing directly to files.
Method | Advantage | Disadvantage |
---|---|---|
json.dumps() | Returns a JSON string which can be used in various ways | Can consume more memory with large data |
json.dump() | Efficiently handles large data by writing directly to a file | Less flexible, only writes to a file |
In the end, whether you choose json.dumps() or json.dump() depends on your specific needs. Both methods offer unique advantages and can be powerful tools in your Python programming toolkit.
We hope this guide has helped you understand and master the use of json.dumps() in Python. Happy coding!