Python venv: Your Guide to Virtual Environments
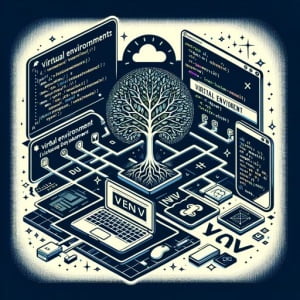
Are you finding it challenging to manage Python dependencies for your projects? You’re not alone. Many developers face this issue, especially when working on multiple projects with different dependencies.
Thankfully, Python’s venv module provides a solution to this problem. Much like a personal workspace, venv allows you to create isolated environments for your Python projects, ensuring that dependencies do not interfere with each other.
In this guide, we will walk you through the process of creating and using virtual environments in Python with venv.
Whether you’re a beginner just starting out or an experienced developer looking to streamline your workflow, this guide will provide you with the knowledge and tools you need to effectively use Python’s venv module.
TL;DR: How Do I Create and Use a Python venv Virtual Environment?
You can create and activate a virtual environment using the venv module in Python with the following commands:
python3 -m venv /path/to/new/virtual/environment
# And Activate it:
source /path/to/new/virtual/environment/bin/activate
This will isolate your Python project and its dependencies, ensuring that it doesn’t interfere with other projects. It’s like having a dedicated workspace for each of your Python projects.
Keep reading for a more detailed guide on how to effectively create and use virtual environments in Python with venv.
Table of Contents
- Basics of Python Venv Management
- Handling Multiple Venvs and Versions
- Specific Python Versions and Venvs
- Exploring Alternatives to Python venv
- Troubleshooting Issues in Python venv
- What is a Python Venv?
- Packages, Dependencies, and Venvs
- Python venv in Larger Projects
- Professional Usage with Python venv
- What’s Next? pip Package Managemer
- Further Readings and Info
- Recap: Python Virtual Environments
Basics of Python Venv Management
To create a virtual environment in Python using the venv module, you can use the following command:
python3 -m venv /path/to/new/virtual/environment
This command tells Python to run the venv module as a script, which will then create a new virtual environment in the directory you specify. You can replace /path/to/new/virtual/environment
with your desired directory path.
Activating the Virtual Environment
After creating your virtual environment, you need to activate it. You can do this using the following command:
source /path/to/new/virtual/environment/bin/activate
Upon activation, your terminal’s prompt will change to indicate that you are now operating within a Python virtual environment. It will look something like this:
(myenv) user@host:~$
The (myenv)
indicates that you are currently in the ‘myenv’ virtual environment. You can replace ‘myenv’ with the name of your virtual environment.
Installing Packages in the Virtual Environment
Once you’ve activated your virtual environment, you can start installing packages into it. For instance, if you want to install the requests package, you can do so using the following command:
pip install requests
This will install the requests package into your virtual environment, isolated from your system’s Python environment. Any Python scripts run within this virtual environment will have access to the requests package, but scripts run outside of this environment will not.
Remember, managing Python dependencies using virtual environments can help ensure that your projects remain clean and organized. It’s a crucial step in Python development, especially as your projects grow in complexity.
Handling Multiple Venvs and Versions
Managing Multiple Python venv Virtual Environments
As your projects grow, you may find yourself needing to manage multiple virtual environments. Each of your Python projects should ideally have its own virtual environment to keep dependencies isolated. You can create and activate these environments using the commands we discussed earlier.
Using Python venv with Different Python Versions
Python venv is versatile enough to work with different versions of Python. To create a virtual environment with a specific version of Python, you can use the python
command followed by the version number. For example:
python3.6 -m venv /path/to/new/virtual/environment
This will create a new virtual environment that uses Python 3.6. Remember to replace /path/to/new/virtual/environment
with your desired directory path.
Integrating Python venv with IDEs
Most Integrated Development Environments (IDEs) support Python venv. This means you can create, activate, and manage your virtual environments directly from your IDE. For example, in PyCharm, you can create a new virtual environment by going to File > New Project Settings > Project Interpreter and then clicking on the gear icon and selecting Create VirtualEnv.
Specific Python Versions and Venvs
Let’s say you have a project that requires Python 3.6. Here’s how you can create a new virtual environment with Python 3.6:
python3.6 -m venv my_project_env
Then, to activate the virtual environment:
source my_project_env/bin/activate
Your terminal’s prompt should change to indicate that you are now in the ‘my_project_env’ virtual environment:
(my_project_env) user@host:~$
Remember, using Python’s venv module to create and manage virtual environments can greatly simplify your workflow, especially as you start to work on larger, more complex projects.
Exploring Alternatives to Python venv
While Python’s venv module is a powerful tool for creating and managing virtual environments, it’s not the only option available. There are other tools such as virtualenv and conda that you can use to achieve the same goal.
Virtualenv: A Versatile Alternative
Virtualenv is a popular alternative to venv. It’s an older tool that provides similar functionality but with a few additional features. For instance, virtualenv allows you to create virtual environments for different Python versions, even if they’re not installed system-wide.
To create a virtual environment with virtualenv, you can use the following command:
virtualenv /path/to/new/virtual/environment
This will create a new virtual environment in your specified directory.
Conda: An All-in-One Solution
Conda is another alternative that you might consider. Unlike venv and virtualenv, conda is more than just a virtual environment tool. It’s also a package manager that can install packages for any language, not just Python.
To create a new conda environment, you can use the following command:
conda create --name myenv
This will create a new conda environment named ‘myenv’.
Choosing the Right Tool
When it comes to choosing between venv, virtualenv, and conda, it largely depends on your specific needs. If you’re working purely with Python and need a simple, straightforward tool, venv is a great choice. If you need a bit more flexibility and don’t mind the additional complexity, virtualenv might be the way to go. And if you’re working with multiple languages and need a comprehensive solution, conda could be worth considering.
Remember, the best tool is the one that fits your workflow and makes your development process smoother and more efficient.
Troubleshooting Issues in Python venv
While working with Python venv virtual environments, you might encounter some common issues. Let’s discuss these problems and their solutions.
Issue: Unable to Activate Virtual Environment
If you’re unable to activate your virtual environment, make sure that you’re in the correct directory and that the virtual environment exists. You can verify this by checking if the activate
script is present in the bin
directory of your virtual environment.
ls /path/to/new/virtual/environment/bin
# Output:
# activate activate.csh activate.fish easy_install easy_install-3.8 pip pip3 pip3.8 python python3
If the activate
script is present, you should be able to activate the virtual environment using the source
command.
Issue: Packages Installed in the Virtual Environment are not Available
If you find that the packages installed in the virtual environment are not available, make sure that the virtual environment is activated. Only then will Python be able to access the packages installed in the virtual environment.
Best Practices and Optimization Tips
- Always activate the virtual environment before installing packages or running Python scripts that depend on these packages.
Try to keep each project in its own virtual environment to avoid conflicts between dependencies.
Regularly update your packages to ensure you have the latest features and security updates.
When done working in a virtual environment, you can deactivate it using the
deactivate
command. This will revert back to using the system-wide Python interpreter and packages.
Remember, Python’s venv module is a powerful tool for managing dependencies and keeping your projects organized. With these troubleshooting tips and best practices, you can avoid common pitfalls and make the most of virtual environments.
What is a Python Venv?
A virtual environment in Python is an isolated workspace for your Python projects. It’s a self-contained directory tree that includes a Python installation and a number of additional packages.
This isolation prevents packages and dependencies from interfering with each other across different projects. It allows you to manage your Python projects more effectively, especially when they have different dependencies.
# Creating a virtual environment
python3 -m venv my_project_env
# Activating the virtual environment
source my_project_env/bin/activate
# Output:
# (my_project_env) user@host:~$
In the example above, we created a new virtual environment named ‘my_project_env’. We then activated the environment, which changed our terminal prompt to indicate that we’re now operating within ‘my_project_env’.
Packages, Dependencies, and Venvs
A Python package is a collection of modules, which are essentially Python scripts that perform specific tasks. These packages are used to extend the functionality of Python and can be installed in your virtual environment using a package manager like pip.
A dependency, on the other hand, is a package that another package needs to function properly. For example, if you’re developing a web application in Python, you might use the Flask package. Flask, in turn, depends on other packages like Werkzeug and Jinja2 to function properly. These are Flask’s dependencies.
By using a virtual environment, you can manage these packages and dependencies on a per-project basis, ensuring that they don’t conflict with each other across different projects.
Python venv in Larger Projects
Python’s venv module isn’t just for small projects. It’s also a crucial tool for larger projects and professional development. As your projects grow in complexity, the number of dependencies can also increase. By using venv, you can manage these dependencies effectively and ensure that your project remains organized and maintainable.
Professional Usage with Python venv
In a professional setting, using virtual environments becomes even more important. It allows different developers working on the same project to have the same setup and avoid conflicts in dependencies. It also makes it easier to deploy your application, as you can ensure that your production environment matches your development environment.
What’s Next? pip Package Managemer
While this guide focuses on creating and using virtual environments with venv, it’s also important to understand how to manage Python packages with pip. Pip is a package manager for Python and is used to install and manage Python packages. It’s a powerful tool that complements venv and can greatly enhance your Python development workflow.
For a more in-depth guide on using pip, you can refer to the official pip documentation.
Further Readings and Info
A deep understanding of Python development starts with installation, so Click Here for a Complete Guide on Installing Python in Ubuntu and discover how it powers your development projects.
To further your understanding of related Python environment topics, you might want to explore other resources such as:
- Installing Jupyter Notebook: A Step-by-Step Setup Guide – Discover how to install Jupyter Notebook, a powerful tool for interactive data analysis and coding.
Taking Control of Python Versions: An Introduction to pyenv – Master the art of Python version management with pyenv to enhance your development workflow.
Python’s Official Guide to Importing: Official Python documentation detailing the import statement.
Installing Setuptools for Python on Linux: Learn how to install the necessary setuptools for Python development on Linux systems.
GitPython Official Documentation: Read the official documentation for GitPython, a Python library used to interact with Git repositories.
Remember, mastering Python’s venv module and understanding virtual environments is a crucial step in becoming a proficient Python developer. It’s a tool that will serve you well in your Python development journey.
Recap: Python Virtual Environments
In this guide, we’ve explored the process of creating and using virtual environments in Python with venv.
We’ve learned how to create a virtual environment using python3 -m venv /path/to/new/virtual/environment
, and how to activate it using source /path/to/new/virtual/environment/bin/activate
. We’ve also discussed how to install packages into the virtual environment using pip install package-name
.
We’ve addressed common issues such as being unable to activate the virtual environment or access installed packages, and provided solutions for these problems. We’ve also shared some best practices and optimization tips to help you make the most of Python’s venv module.
In addition to venv, we’ve explored alternative tools for creating virtual environments in Python, such as virtualenv and conda. These tools provide additional features and can be used based on your specific needs.
Tool | Use Case |
---|---|
venv | Simple, straightforward tool for creating and managing Python virtual environments |
virtualenv | Provides additional features and flexibility, can create virtual environments for different Python versions |
conda | Comprehensive solution for creating virtual environments and managing packages for any language |
Mastering Python’s venv module and understanding virtual environments is a crucial step in becoming a proficient Python developer. It’s a tool that will serve you well in your Python development journey, whether you’re working on small projects or complex professional applications.