Bash Script Array Looping | How to Iterate Through Values
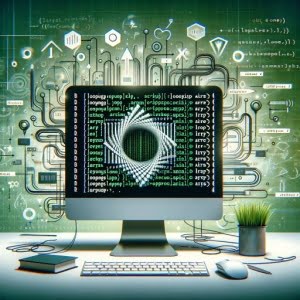
Are you finding it difficult to loop through arrays in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling arrays in Bash, but we’re here to help.
Think of Bash as a powerful conductor, capable of orchestrating each element in an array in turn. It’s a versatile and handy tool for various tasks, especially when dealing with large amounts of data.
In this guide, we’ll walk you through the process of looping through arrays in Bash, from the basics to more advanced techniques. We’ll cover everything from the simple ‘for’ loop to more complex loops with conditional statements, as well as alternative approaches.
Let’s get started and master looping through arrays in Bash!
TL;DR: How Do I Loop Through an Array in Bash?
You can use a
'for'
loop to iterate through an array in Bash, with the syntaxfor i in "${array[@]}"
. This is a fundamental technique in Bash scripting that allows you to process each element in an array individually.
Here’s a simple example:
array=('item1' 'item2' 'item3')
for i in "${array[@]}"
do
echo "$i"
done
# Output:
# item1
# item2
# item3
In this example, we’ve created an array with three elements: ‘item1’, ‘item2’, and ‘item3’. We then use a ‘for’ loop to iterate through each element in the array. The "${array[@]}"
syntax is used to access all elements in the array. For each iteration, the current element’s value is stored in the i
variable, which we then print out using the echo
command. This results in each item in the array being printed on a new line.
This is a basic way to loop through an array in Bash, but there’s much more to learn about Bash scripting and array manipulation. Continue reading for more detailed information and advanced usage examples.
Table of Contents
- Mastering the Basics: Looping Through Arrays in Bash
- Advanced Techniques: Bash Array Looping
- Exploring Alternatives: Other Ways to Loop Through Arrays in Bash
- Troubleshooting Bash Array Loops: Common Pitfalls and Solutions
- Understanding the Basics: Arrays and Loops in Bash
- Applying Bash Array Loops in Real-World Scenarios
- Wrapping Up: Mastering Bash Array Loops
Mastering the Basics: Looping Through Arrays in Bash
Looping through an array in Bash is a fundamental skill that every developer should master. The most common way to do this is by using a ‘for’ loop. Let’s dive into how this works.
Understanding ‘For’ Loops in Bash
A ‘for’ loop is a control flow statement that allows code to be executed repeatedly. When it comes to arrays, a ‘for’ loop can iterate through each element, allowing you to perform operations on individual items.
Here’s an example of using a ‘for’ loop to loop through an array in Bash:
fruits=('apple' 'banana' 'cherry')
for fruit in "${fruits[@]}"
do
echo "I like $fruit."
done
# Output:
# I like apple.
# I like banana.
# I like cherry.
In this example, we’ve created an array named fruits
with three elements: ‘apple’, ‘banana’, and ‘cherry’. The ‘for’ loop then iterates over each element in the array. For each iteration, the current element’s value is stored in the fruit
variable, which we then use in the echo
command to print out a sentence.
Advantages and Potential Pitfalls
The main advantage of using a ‘for’ loop to iterate through an array is its simplicity and readability. The code is straightforward, making it easy for anyone (even those new to Bash scripting) to understand what’s happening.
However, there are potential pitfalls. If you’re not careful, you might run into issues with whitespace in your array elements, as Bash will split on whitespace by default. To avoid this, always remember to quote your array expansion, like so: "${array[@]}"
.
In the next section, we’ll take a look at more complex uses of loops with arrays in Bash.
Advanced Techniques: Bash Array Looping
As you become more comfortable with Bash scripting, you’ll find that there are more complex scenarios where you need to loop through arrays. This might include using nested loops or incorporating conditional statements within your loops. Let’s explore these advanced techniques.
Nested Loops in Bash
A nested loop is a loop within a loop. When working with arrays, you might find a situation where you need to loop through multiple arrays simultaneously. For example, you might have a script that needs to compare the elements of two arrays. Here’s an example:
fruits=('apple' 'banana' 'cherry')
colors=('red' 'yellow' 'red')
for i in "${!fruits[@]}"
do
echo "The ${fruits[$i]} is ${colors[$i]}"
done
# Output:
# The apple is red
# The banana is yellow
# The cherry is red
In this example, we have two arrays: fruits
and colors
. We use a ‘for’ loop to iterate through the indices of the fruits
array (obtained using the !
symbol before the array variable). For each iteration, we print out a sentence that combines elements from both arrays at the same index.
Loops with Conditional Statements
You might also find situations where you need to perform different actions depending on the value of the array element. This is where conditional statements come in. Here’s an example:
numbers=(1 2 3 4 5)
for num in "${numbers[@]}"
do
if ((num % 2 == 0))
then
echo "$num is even"
else
echo "$num is odd"
fi
done
# Output:
# 1 is odd
# 2 is even
# 3 is odd
# 4 is even
# 5 is odd
In this example, we have an array numbers
with five elements. We use a ‘for’ loop to iterate through each element in the array. Inside the loop, we have a conditional ‘if’ statement that checks if the current number is even or odd (using the modulus operator %
). Depending on the result, we print out a different message.
These are just a few examples of the advanced techniques you can use when looping through arrays in Bash. As you gain more experience, you’ll find that the possibilities are endless.
Exploring Alternatives: Other Ways to Loop Through Arrays in Bash
While ‘for’ loops are the most common way to loop through arrays in Bash, they are not the only option. There are other control flow statements you can use, such as ‘while’ and ‘until’ loops. Let’s delve into these alternative approaches.
Looping with ‘While’ Loops
A ‘while’ loop will continue to execute as long as the condition in the loop remains true. When working with arrays, you can use a counter and increment it in each iteration until you’ve gone through all the elements. Here’s an example:
fruits=('apple' 'banana' 'cherry')
index=0
while [ $index -lt ${#fruits[@]} ]
do
echo "I like ${fruits[$index]}"
((index++))
done
# Output:
# I like apple
# I like banana
# I like cherry
In this example, we initialize a counter index
at 0. The ‘while’ loop runs as long as index
is less than the length of the fruits
array (obtained using ${#fruits[@]}
). Inside the loop, we print out the current fruit, and then increment the index
by 1.
Looping with ‘Until’ Loops
An ‘until’ loop is similar to a ‘while’ loop, but the loop will continue to execute until the condition in the loop becomes true. Here’s an example of how you can use an ‘until’ loop with an array:
numbers=(1 2 3 4 5)
index=0
until [ $index -eq ${#numbers[@]} ]
do
echo "Processing number: ${numbers[$index]}"
((index++))
done
# Output:
# Processing number: 1
# Processing number: 2
# Processing number: 3
# Processing number: 4
# Processing number: 5
In this example, the ‘until’ loop runs until index
equals the length of the numbers
array. Inside the loop, we print out a message with the current number, and then increment the index
by 1.
Comparing the Different Loop Methods
Each of these loop methods has its advantages and disadvantages. ‘For’ loops are simple and straightforward, making them a good choice for basic array looping. ‘While’ and ‘until’ loops offer more control over the loop condition, which can be useful in more complex scenarios. However, they require you to manually manage a counter variable, which can lead to off-by-one errors if you’re not careful.
In the end, the best method to use depends on your specific needs and the complexity of your script.
Troubleshooting Bash Array Loops: Common Pitfalls and Solutions
As you work with Bash arrays and loops, you might encounter some common issues. These can range from off-by-one errors to challenges with empty arrays. Let’s discuss these problems and provide solutions and workarounds.
Off-By-One Errors
One of the most common mistakes when working with loops and arrays is the off-by-one error. This typically happens when you’re using a counter to iterate through an array, and you go one index too far, leading to an ‘index out of range’ error.
Here’s an example of what can go wrong:
numbers=(1 2 3 4 5)
index=0
while [ $index -le ${#numbers[@]} ]
do
echo "Processing number: ${numbers[$index]}"
((index++))
done
# Output:
# Processing number: 1
# Processing number: 2
# Processing number: 3
# Processing number: 4
# Processing number: 5
# Processing number:
In this example, you can see that the loop tries to process one more element than the array contains, resulting in an empty output on the last iteration. To avoid this, ensure that your loop condition is index -lt ${#numbers[@]}
(less than the length of the array), not index -le ${#numbers[@]}
(less than or equal to the length of the array).
Handling Empty Arrays
Another common issue is how to handle empty arrays. If you attempt to loop through an empty array without checking if it’s empty first, your loop might not behave as expected.
Here’s an example of how you can check if an array is empty before looping through it:
empty_array=()
if [ ${#empty_array[@]} -eq 0 ]; then
echo "Array is empty"
else
for i in "${empty_array[@]}"
do
echo "Processing item: $i"
done
fi
# Output:
# Array is empty
In this example, we first check if the length of the array is 0, which indicates that the array is empty. If it is, we print a message and skip the loop. If it’s not, we proceed with the loop as usual.
Understanding these common issues and how to address them will help you write more robust Bash scripts and avoid frustrating bugs.
Understanding the Basics: Arrays and Loops in Bash
Before we delve deeper into looping through arrays in Bash, it’s crucial to understand the fundamental concepts of arrays and loops in Bash.
What are Arrays in Bash?
An array is a data structure that stores a collection of elements. These elements, also known as values, can be accessed by their corresponding indices. In Bash, arrays are zero-indexed, which means the first element is at index 0.
Here’s an example of creating an array in Bash:
fruits=('apple' 'banana' 'cherry')
echo "The first fruit is ${fruits[0]}"
# Output:
# The first fruit is apple
In this example, we define an array fruits
with three elements. We then print out the first element of the array using its index (0
).
What are Loops in Bash?
A loop is a programming construct that allows you to execute a block of code repeatedly. In Bash, there are several types of loops, including ‘for’, ‘while’, and ‘until’ loops. Loops are a powerful tool that can automate repetitive tasks.
Here’s a simple example of a ‘for’ loop in Bash:
for i in 1 2 3
do
echo "Processing number $i"
done
# Output:
# Processing number 1
# Processing number 2
# Processing number 3
In this example, the ‘for’ loop iterates over the numbers 1, 2, and 3. For each iteration, it runs the echo
command, which prints out a message with the current number.
Understanding arrays and loops is the first step towards mastering the art of looping through arrays in Bash. With these basics in mind, you’ll be able to better comprehend the examples and techniques discussed in this guide.
Applying Bash Array Loops in Real-World Scenarios
Bash array loops are not just academic concepts. They have practical applications in larger scripts or projects, and understanding them can significantly enhance your Bash scripting skills.
Bash Array Loops in Larger Scripts
In larger scripts, you might need to process large amounts of data stored in arrays. For instance, you might have a script that reads lines from a file into an array and then processes each line individually. In such cases, knowing how to loop through arrays in Bash is invaluable.
mapfile -t lines < file.txt
for line in "${lines[@]}"
do
echo "Processing line: $line"
done
# Output:
# Processing line: [Line 1 from file]
# Processing line: [Line 2 from file]
# ...
In this example, the mapfile
command reads lines from a file into an array. We then use a ‘for’ loop to process each line.
Exploring Related Concepts: Associative Arrays in Bash
Once you’re comfortable with regular arrays and loops in Bash, you might want to explore related concepts like associative arrays. These are arrays that use keys instead of indices to access values, similar to dictionaries in Python or objects in JavaScript.
declare -A fruits
fruits=( [apple]='red' [banana]='yellow' [cherry]='red' )
for fruit in "${!fruits[@]}"
do
echo "The $fruit is ${fruits[$fruit]}"
done
# Output:
# The apple is red
# The banana is yellow
# The cherry is red
In this example, we declare an associative array with the -A
option. We then use a ‘for’ loop to iterate over the keys of the array (obtained using the !
symbol before the array variable).
Further Resources for Bash Array Loop Mastery
To deepen your understanding of Bash array loops and related concepts, consider the following resources:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and a great reference for all things Bash.
- Bash Academy: An online guide that provides a deep dive into Bash scripting, including arrays and loops.
- Bash Scripting Tutorial by Ryan’s Tutorials: A beginner-friendly tutorial that covers the basics of Bash scripting, with a section dedicated to loops.
Remember, mastering Bash array loops requires practice. Keep experimenting, making mistakes, and learning from them. Happy scripting!
Wrapping Up: Mastering Bash Array Loops
In this comprehensive guide, we’ve journeyed through the process of looping through arrays in Bash, from basic to advanced techniques. We’ve covered the use of ‘for’ loops, delved into more complex scenarios with nested loops and conditionals, and even explored alternative approaches with ‘while’ and ‘until’ loops.
We began with the basics, understanding how to use a ‘for’ loop to iterate through an array in Bash. We then ventured into more complex territory, discussing nested loops, loops with conditional statements, and how to use ‘while’ and ‘until’ loops to iterate through arrays. We also touched on potential pitfalls and how to avoid them, such as off-by-one errors and handling empty arrays.
Along the way, we tackled common challenges you might face when working with Bash array loops, providing you with solutions and workarounds for each issue. We also compared the different loop methods, highlighting their pros and cons to help you choose the best method for your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘For’ Loop | Simple and straightforward | May struggle with whitespace in array elements |
‘While’ Loop | More control over the loop condition | Requires manual management of a counter variable |
‘Until’ Loop | Executes until the condition becomes true | Same as ‘while’ loop |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to loop through arrays in Bash. With this knowledge, you’re well-equipped to handle array manipulation in your Bash scripts. Happy scripting!