Using Python Assert to Write Bug Free Code
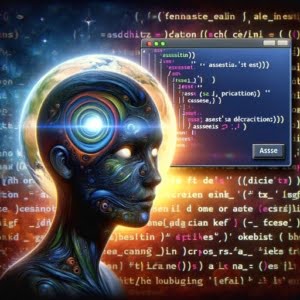
Ever wondered how to use Python’s assert statement? This seemingly modest keyword is actually a powerhouse in Python programming, offering the ability to fortify your code, enhance readability, and streamline debugging.
The assert statement serves as a crucial debugging aid in Python. It tests a specific condition within your code, and if the condition is not met, the program raises an AssertionError. This simple mechanism provides an effective way to identify and manage potential errors in your code.
This article aims to arm you with the knowledge to proficiently harness the assert statement in your Python code. We’ll decode its capabilities, investigate its application, and delve into its role in Python programming. Whether you’re a seasoned Python developer or just embarking on your coding journey, let’s dive into the intriguing world of Python’s assert statement!
TL;DR: What is Python’s Assert Statement?
Python’s assert statement is a debugging tool that tests a specific condition in the code. If the condition is met, the program continues as usual. However, if the condition is not met, the program raises an AssertionError. This mechanism helps to identify and manage potential errors in the code. For more advanced methods, background, tips and tricks, continue reading the article.
x = 10
assert x == 10
# This will pass as x is indeed 10
x = 5
assert x == 10, 'x is not 10!'
# This will raise an AssertionError as x is not 10
Table of Contents
Basics of Python’s Assert Statement
The assert statement in Python is fundamentally a debugging tool that tests a specific condition. If the condition holds true, the program continues to run without any interruption. However, if the condition turns out to be false, the assert statement halts the program and throws an AssertionError exception.
This mechanism is essential for detecting and managing potential errors in your code.
The syntax of the assert statement is quite simple. It involves writing assert
followed by the condition you want to test, and optionally, a string to display if the condition is false.
Consider this basic example of the assert statement:
x = 10
assert x == 10
In this scenario, the assert statement doesn’t interfere because the condition is true (x is indeed 10).
However, if we modify the value of x to 5, the assert statement will raise an AssertionError:
x = 5
assert x == 10, 'x is not 10!'
The assert statement proves particularly useful in debugging and testing. It allows you to establish conditions that you anticipate in your code, and to detect scenarios where these conditions are not met. This greatly aids in catching and rectifying bugs, thereby enhancing the robustness and error-free nature of your Python code.
Advanced Usage: Python Assert
The assert statement extends beyond simple condition-checking, offering a suite of advanced uses that can aid in crafting cleaner, more efficient, and robust Python code. Let’s delve into these advanced use cases.
Assert in Complex Code Structures
Imagine you’re scripting a function that operates on a list of integers. You anticipate the list to always contain a minimum of one element. An assert statement can be employed to validate this:
def calculate_average(numbers):
assert len(numbers) > 0, 'List is empty!'
return sum(numbers) / len(numbers)
In this scenario, if an empty list is passed to the function, the assert statement will raise an AssertionError, halting any further execution and potential errors.
Exception Handling with Assert
The assert statement serves as a potent tool for exception handling. It enables early detection and management of potential errors during code execution, thereby simplifying debugging and maintenance.
It’s crucial to remember that assert should not be employed for data validation or to manage runtime errors. It is a debugging aid, not a mechanism for handling runtime errors.
Below is a Python code snippet which demonstrates how assert can be used for exception handling.
This example checks if a number is not negative:
def enter_positive_number(num):
# This will raise AssertionError if num is less than 0
assert num >= 0, "Only positive numbers are allowed"
return num * num
try:
num = int(input("Enter a number: "))
print(enter_positive_number(num))
except AssertionError as e:
print(e)
except ValueError:
print("Please enter a valid integer.")
In the above example, if a negative number is entered, assert raises an AssertionError with the message “Only positive numbers are allowed”. If a non-integer value is entered, a ValueError is raised, which is then handled in the second exception block.
The assert statement therefore allows us to handle exceptions in situations where we want to ensure certain conditions are met.
Pitfalls and Troubleshooting
Despite its utility, the assert statement has its pitfalls. A common blunder is using it to manage conditions that could occur during the normal operation of the program. Assert is designed for debugging and catching programmer errors, not for handling runtime errors or data validation.
Misusing Assert for Data Validation
A common issue with the assert statement is misunderstanding its purpose. It’s not intended for data validation or error handling during normal operation of your program. Instead, it serves as a debugging aid, designed to catch and alert you of programming errors.
For instance, using the assert statement to validate user input is a misuse of the tool. Proper error handling mechanisms like try/except blocks should be used in these situations.
Incorrect Usage | Correct Usage |
---|---|
assert user_age > 0, 'Age must be positive!' | if user_age <= 0: raise ValueError('Age must be positive!') |
# Don't do this
assert user_age > 0, 'Age must be positive!'
# Do this instead
if user_age <= 0:
raise ValueError('Age must be positive!')
Assert When Running in Optimized Mode
Another important aspect to remember is that Python’s optimization mode, invoked with the -O command line switch, will cause assert statements to be disregarded. Thus, avoid using assert if the subsequent code depends on the assert statement for proper function.
Here is a demonstration of Python’s optimization mode and its effect on assertions:
Firstly, create a Python script test.py
:
# contents of test.py
def foo(x):
assert x > 0, "Only positive numbers allowed"
return x * 10
print(foo(-5))
If you run it, you can see the AssertionError
:
$ python3 test.py
Traceback (most recent call last):
File "test.py", line 5, in <module>
print(foo(-5))
File "test.py", line 2, in foo
assert x > 0, "Only positive numbers allowed"
AssertionError: Only positive numbers allowed
Secondly, run the same file but with Python’s optimization mode:
$ python3 -O test.py
-50
Here we can see that when Python’s optimization mode is on (-O), the assert statement will be disregarded and the function foo(-5)
executes normally without raising an AssertionError
.
Assert statements should not be used for handling errors that can occur in normal operation of the program. It is designed as a debugging aid, not as a way of handling runtime errors.
Instead of using assert statements, error handling code should be used to ensure that the program continues to function correctly in spite of errors.
Assert Usage Best Practices
In this section, we’ll outline best practices for using assert statements, explore their role in test-driven development and provide insights on how to leverage them in a “defensive programming” usage model.
Assert in Test-Driven Development
In the realm of test-driven development (TDD), the assert statement plays a pivotal role. TDD is a software development approach where tests are written before the actual code.
In such a scenario, the assert statement can be used to define the expected outcomes of your functions, making it an indispensable tool in your Python testing toolkit.
In test-driven development, a typical workflow may look like this:
- Write a test case using assert to check the expected outcome of a function.
- Initially, the function may not be implemented yet, so the test will fail.
- Write the function to satisfy the test case.
- Run the test again. If it passes, you know the function is correctly implemented.
- If the test does not pass, modify the function until the test passes.
Here is a concrete example using Python’s built-in unittest
framework:
Let’s say you are creating a function to add two numbers. Before writing the function, you first write a test:
import unittest
class TestAddFunction(unittest.TestCase):
def test_add(self):
self.assertEqual(add(3,4), 7)
if __name__ == '__main__':
unittest.main()
Running this code will fail because add
function is not defined yet.
Now, let’s implement the add
function:
def add(x, y):
return x + y
import unittest
class TestAddFunction(unittest.TestCase):
def test_add(self):
self.assertEqual(add(3,4), 7)
if __name__ == '__main__':
unittest.main()
The test will now pass, confirming that your function is working as expected.
This is a simplified example. In a full-fledged project, you’d separate your tests and implementation into different modules, among other things. But fundamentally, TDD revolves around this flow: write tests, write code to pass tests, refactor, and repeat.
Assert Statement and Defensive Programming
In the context of defensive programming, the assert statement plays a significant role. Defensive programming is a practice where the programmer anticipates and prepares for potential errors and issues in the code.
Here’s an example that demonstrates how to use assert for defensive programming:
Let’s say you are writing a function that calculates the area of a triangle. One defensive programming practice is to ensure that the base and height values inputted are positive:
def calculate_triangle_area(base, height):
# Defensive programming using assert
assert base > 0, "Base of a triangle must be positive"
assert height > 0, "Height of a triangle must be positive"
return 0.5 * base * height
try:
print(calculate_triangle_area(10, -5))
except AssertionError as e:
print(f"Caught an error: {e}")
In this code, if negative values are passed to the calculate_triangle_area
function, an AssertionError
would be raised with a relevant error message. This acts as a safety check, ensuring that unexpected or invalid values don’t propagate further down and cause hard-to-trace bugs.
More Python Debugging and Testing
While the assert statement is a powerful tool in Python, it’s just one piece of the larger picture of debugging and testing in Python.
Debugging and testing are crucial aspects of Python development, and understanding the various tools and methods available is key to writing robust, efficient, and error-free code.
Python provides a range of tools and features for debugging and testing, including built-in functions, libraries, and modules.
For example, Python’s built-in pdb
module is a powerful tool for interactive debugging, allowing you to pause your program, look at the values of variables, and watch program execution step-by-step.
In addition to the built-in tools, there are also many third-party libraries available for debugging and testing in Python. For example, PyTest
is a popular testing framework that provides a wealth of features for advanced testing, including support for fixture reuse, setup and teardown code for tests, and powerful assert introspection.
Assert vs. Other Debugging and Testing Tools
While the assert statement is a simple and straightforward tool for catching errors, other debugging and testing tools offer more advanced features.
Tool | Description |
---|---|
Assert Statement | A simple tool for catching errors in Python code |
Python Debugger (pdb) | A built-in interactive source debugger for Python |
PyTest | A third-party testing framework for Python with advanced features |
pdb++ | A third-party library that provides an enhanced interface for pdb |
PuDB | A third-party library that provides a full-screen, console-based visual debugger for Python |
In addition to Python’s built-in tools, the broader Python community has developed an array of third-party libraries that can boost your debugging and testing capabilities. Libraries like PyTest and Nose offer advanced testing features, while debugging libraries like pdb++ and PuDB provide enhanced debugging interfaces and additional features.
For example, testing frameworks like PyTest provide more comprehensive testing capabilities, including the ability to write complex tests with setup and teardown code, and to generate detailed reports on test results.
PyTest includes a feature known as ‘assert rewriting’ that provides more detailed information on failing assert statements, thereby making your debugging process even more efficient.
Further Resources for Debugging and Testing
To expand your prowess with debugging and testing in Python, we have curated some useful resources for you:
- Pytest Tutorial: Writing Python Test Cases – Dive deep into Pytest’s advanced features, including test organization and customization.
Effective Logging Guide with Python – Discover the Python logging module and how it enables effective logging in your applications.
Python unittest Framework Tutorial – Dive into the details of Python’s unittest module and its role in test-driven development.
How Python Debugging Works in VS Code – A helpful guide from the VS Code-Jupyter project explaining how Python debugging works in Visual Studio Code.
Troubleshooting Python Scripts – This PDF by Esri shares tips and techniques for troubleshooting Python scripts.
Python Errors and Exceptions – The official Python tutorial on handling and understanding different types of errors and exceptions.
By incorporating these resources into your Python development routine and furthering your knowledge on debugging and testing, you will enhance your proficiency and efficiency as a Python developer. Dive into these resources and evolve your debugging and testing skills in Python.
Wrapping Up: Python Assert Guide
We’ve delved into the assert statement in Python, shedding light on how this seemingly simple keyword can significantly enhance your Python code, making it more robust, readable, and debug-friendly.
We’ve dissected the basics of the assert statement, understanding its syntax, usage, and its pivotal role in Python programming. We’ve seen how it serves as a tool to test conditions in your code, aiding in catching and handling potential errors.
We’ve dived into the advanced uses of the assert statement, gaining insights into its role in complex code structures, exception handling, and test-driven development. We’ve also discussed the potential pitfalls and best practices when using the assert statement, such as the impact of Python’s optimization mode on the assert statement.
We also zoomed out to discuss the larger picture of debugging and testing in Python. We’ve learned about other tools and methods used for debugging and testing, and compared the assert statement with these tools.
So, the next time you’re working on a Python project, remember the assert statement. It’s more than just a keyword – it’s a powerful tool that can help you write better Python code. Happy coding!