Python Unittest Library: Unit Testing Guide
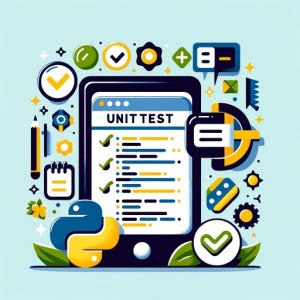
Are you finding it difficult to handle unit testing in Python? You’re not alone. Many developers find themselves in a bind when it comes to implementing unit tests in their Python code. But, consider Python’s unittest module as your safety net – it’s designed to catch bugs before they become a real problem.
In this guide, we’ll walk you through the process of using unittest in Python, from the basics to more advanced techniques. We’ll cover everything from creating simple test cases, using assertions, to handling complex testing scenarios with setup and teardown methods, testing exceptions, and using mock objects.
So, whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, there’s something in this guide for you.
Let’s dive in and start mastering Python unittest!
TL;DR: How Do I Use Unittest in Python?
To use unittest in Python, you create a test case by subclassing
unittest.TestCase
and then define test methods. Each test method should start with the word ‘test’.
Here’s a simple example:
import unittest
class TestMyFunction(unittest.TestCase):
def test_addition(self):
self.assertEqual(1 + 1, 2)
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# OK
In this example, we’ve created a test case TestMyFunction
that inherits from unittest.TestCase
. Inside this test case, we’ve defined a test method test_addition
that checks if 1 + 1 equals 2 using the assertEqual
method. Running this script will execute the test and, if successful, print out a message indicating that the test passed.
This is a basic way to use unittest in Python, but there’s much more to learn about creating and managing tests in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
The Basics of Python Unittest Module
If you’re new to Python or unittest, don’t worry. We’ll start with the basics and gradually move towards more complex concepts. Here’s what you need to know to get started with unittest.
Creating Test Cases
A test case is a set of conditions or variables under which a tester determines whether a system under test satisfies requirements or works correctly. In Python unittest, we create a test case by subclassing unittest.TestCase
.
Let’s look at a simple example:
import unittest
class TestMyFunction(unittest.TestCase):
pass
if __name__ == '__main__':
unittest.main()
In this example, we’ve imported the unittest module and then created a test case TestMyFunction
that inherits from unittest.TestCase
. The pass
statement is a placeholder, indicating that this block of code will be filled in later.
Defining Test Methods
Test methods are the actual tests that we run. In Python unittest, each test method should start with the word ‘test’. This naming convention tells unittest which methods represent tests.
Here’s how you can define a test method:
import unittest
class TestMyFunction(unittest.TestCase):
def test_addition(self):
self.assertEqual(1 + 1, 2)
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# OK
In this example, we’ve defined a test method test_addition
that checks if 1 + 1 equals 2 using the assertEqual
method. Running this script will execute the test and, if successful, print out a message indicating that the test passed.
Using Assertions
Assertions are the heart of any test framework. In Python unittest, assertions are helper methods that let you compare the output of your function to the expected result. The assertEqual(a, b)
method, for example, checks if a
equals b
.
import unittest
class TestMyFunction(unittest.TestCase):
def test_addition(self):
self.assertEqual(1 + 1, 2)
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# OK
In this example, the assertEqual
method checks if 1 + 1 equals 2. If the assertion is True, the test passes. If the assertion is False, the test fails.
Advantages and Potential Pitfalls
The unittest module is a powerful tool for writing and running tests. It provides a rich set of assertions, automatic discovery of test cases, and the ability to group and isolate tests. However, it’s worth noting that unittest follows the xUnit style, which can be more verbose compared to other testing frameworks like pytest. Moreover, unittest’s heavy use of object-oriented features might not be to everyone’s liking.
That said, unittest is a built-in Python module, which means it’s always available and doesn’t require any additional installation. This makes it a great choice for beginners or for projects that want to minimize their dependencies.
Advanced Features of Python Unittest
Once you’ve got the basics down, it’s time to explore some of the more advanced features of Python’s unittest module. These features can help you write more robust and comprehensive tests.
Setup and Teardown Methods
In Python unittest, setUp
and tearDown
methods provide a way to set up some context for each test. The setUp
method runs before each test, while the tearDown
method runs after each test.
Here’s an example:
import unittest
class TestMyFunction(unittest.TestCase):
def setUp(self):
self.list = [1, 2, 3]
def test_count(self):
self.assertEqual(len(self.list), 3)
def tearDown(self):
self.list = None
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# OK
In this example, the setUp
method initializes a list that’s used in the test_count
method. After the test runs, the tearDown
method cleans up by setting the list to None
.
Testing Exceptions
Python unittest provides a way to test if a certain exception is raised by using the assertRaises
method.
Here’s how you can use it:
import unittest
class TestMyFunction(unittest.TestCase):
def test_division(self):
with self.assertRaises(ZeroDivisionError):
1 / 0
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# OK
In this example, the test_division
method checks if dividing 1 by 0 raises a ZeroDivisionError
. If the exception is raised, the test passes.
Using Mock Objects
Mock objects are simulated objects that mimic the behavior of real objects in controlled ways. In Python unittest, you can use the unittest.mock
module to create and use mock objects.
Here’s an example:
import unittest
from unittest.mock import MagicMock
class TestMyFunction(unittest.TestCase):
def test_mock_method(self):
mock = MagicMock()
mock.method.return_value = 'Hello, World!'
self.assertEqual(mock.method(), 'Hello, World!')
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# OK
In this example, we’ve created a mock object and set its method
to return ‘Hello, World!’. When we call mock.method()
, it returns ‘Hello, World!’, and the test passes.
These advanced features of Python unittest can help you write more comprehensive tests and handle a wider range of testing scenarios. It’s worth taking the time to learn and understand them.
Alternative Python Testing Frameworks
While Python’s unittest module is a powerful tool for writing and running tests, it’s not the only testing framework available in Python. Let’s explore some alternatives, namely pytest and doctest, and consider their benefits, drawbacks, and when to use them.
Pytest: A Robust, Feature-Rich Framework
Pytest is a popular testing framework known for its simplicity, ease of use, and powerful features. It supports unittest-style tests out of the box, but also introduces a simpler, more pythonic way of writing tests.
Here’s a simple pytest test:
import pytest
def test_addition():
assert 1 + 1 == 2
To run pytest tests, simply execute the pytest
command in your terminal:
pytest test_file.py
# Output:
# ============================= test session starts ==============================
# platform win32 -- Python 3.7.4, pytest-5.2.1, py-1.8.0, pluggy-0.13.0
# rootdir: ...
# collected 1 item
#
# test_file.py . [100%]
#
# ============================== 1 passed in 0.03s ===============================
As you can see, pytest tests are less verbose and more readable than unittest tests. However, pytest is not a built-in module and requires installation, which might not be ideal for all projects.
Doctest: Testing Through Documentation
Doctest is another built-in Python module that allows you to write tests as part of the documentation of a function. This makes doctest a great tool for testing and documenting at the same time.
Here’s a simple doctest:
def add(a, b):
"""
Adds two numbers together.
>>> add(1, 1)
2
"""
return a + b
In this example, the test is part of the function’s docstring. To run doctest tests, you can use the doctest
module:
import doctest
doctest.testmod()
# Output:
# TestResults(failed=0, attempted=1)
The output indicates that one test was attempted and none failed.
Doctest is great for simple tests and for ensuring that your documentation stays up-to-date with your code. However, it’s not as powerful or flexible as unittest or pytest, and might not be suitable for more complex testing scenarios.
Making the Right Choice
When choosing a testing framework, consider your project’s needs and constraints. Unittest is a good default choice, especially for beginners or for projects that need to minimize dependencies. Pytest is a great option if you want a more pythonic, less verbose testing framework, and don’t mind installing an additional module. Doctest is ideal for simple tests and for projects where documentation is a priority.
Remember, the best testing framework is the one that helps you write and run tests effectively. Choose the one that best fits your project and your personal preference.
Troubleshooting Python Unittest Issues
As with any tool, you might encounter some issues while using Python’s unittest module. Let’s discuss some common problems and their solutions.
Dealing with Failing Tests
When a test fails, unittest provides a detailed report, including the traceback and the failed assertion. Here’s an example of a failing test:
import unittest
class TestMyFunction(unittest.TestCase):
def test_addition(self):
self.assertEqual(1 + 1, 3)
if __name__ == '__main__':
unittest.main()
# Output:
# F
# ======================================================================
# FAIL: test_addition (__main__.TestMyFunction)
# ----------------------------------------------------------------------
# Traceback (most recent call last):
# File "test_file.py", line 5, in test_addition
# self.assertEqual(1 + 1, 3)
# AssertionError: 2 != 3
#
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# FAILED (failures=1)
In this example, the test fails because 1 + 1 does not equal 3. The output shows the failed test, the traceback, and the failed assertion. To fix this, you would need to correct the assertion or the code being tested.
Testing Exceptions
As we discussed earlier, you can test if a certain exception is raised using the assertRaises
method. If the exception is not raised, the test fails. Here’s an example:
import unittest
class TestMyFunction(unittest.TestCase):
def test_division(self):
with self.assertRaises(ZeroDivisionError):
1 / 1
if __name__ == '__main__':
unittest.main()
# Output:
# F
# ======================================================================
# FAIL: test_division (__main__.TestMyFunction)
# ----------------------------------------------------------------------
# Traceback (most recent call last):
# File "test_file.py", line 5, in test_division
# 1 / 1
# AssertionError: ZeroDivisionError not raised
#
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# FAILED (failures=1)
In this example, the test fails because dividing 1 by 1 does not raise a ZeroDivisionError
. To fix this, you would need to correct the assertion or the code being tested.
Isolating Test Cases
Sometimes, tests can interfere with each other, especially if they’re not properly isolated. One way to ensure isolation is by using the setUp
and tearDown
methods to set up and clean up any shared resources or state.
In conclusion, while Python’s unittest module is a powerful tool, it’s not without its challenges. However, with a good understanding of the module and some troubleshooting skills, you can overcome these challenges and write robust, comprehensive tests for your Python code.
Fundamentals of Python Unit Testing
Before we delve deeper into Python unittest, it’s essential to understand the underlying concepts of unit testing and why it’s crucial in software development.
What is Unit Testing?
Unit testing is a software testing method where individual components of a software are tested. The purpose is to validate that each unit of the software performs as expected. A unit is the smallest testable part of any software, often a function or method in object-oriented programming.
Here’s a simple Python function and a corresponding unittest test:
# Function to be tested
def add(a, b):
return a + b
# Corresponding unittest test
import unittest
class TestAddition(unittest.TestCase):
def test_addition(self):
self.assertEqual(add(1, 2), 3)
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.001s
#
# OK
In this example, we’ve defined a function add
that adds two numbers. We then created a unittest test test_addition
that checks if add(1, 2)
equals 3. If the function performs as expected, the test passes.
The Importance of Test-Driven Development (TDD)
Test-Driven Development (TDD) is a software development approach where tests are written before the actual code. The process is often described as ‘Red, Green, Refactor’: Write a failing test (Red), make it pass by implementing the code (Green), and then improve the code while keeping the tests green (Refactor).
TDD offers several benefits, including simpler code, better design, and fewer bugs. It also makes it easier to maintain and extend the code in the future.
The Role of Testing in Software Development
Testing plays a vital role in software development. It ensures that the code works as expected and helps catch bugs before they cause problems. Testing also improves the quality of the code and makes it more maintainable.
In conclusion, unit testing, and testing in general, are fundamental aspects of software development. Understanding these concepts can help you write better tests and, ultimately, better code.
Practical Applications of Unittest
Python’s unittest module is not just for small projects or simple scripts. It’s a robust and flexible testing framework that can handle larger projects and integrate with continuous integration/continuous deployment (CI/CD) systems. Furthermore, it’s an essential tool for measuring and improving test coverage.
Unittest in Larger Projects
In larger projects, managing tests can be challenging. However, unittest provides features like test discovery and test suite, which can make it easier to organize and run tests. Test discovery allows unittest to automatically find and run tests, while test suite lets you group tests and run them together.
Here’s an example of a test suite:
import unittest
class TestAddition(unittest.TestCase):
def test_addition(self):
self.assertEqual(1 + 1, 2)
class TestSubtraction(unittest.TestCase):
def test_subtraction(self):
self.assertEqual(2 - 1, 1)
if __name__ == '__main__':
suite = unittest.TestSuite()
suite.addTest(unittest.makeSuite(TestAddition))
suite.addTest(unittest.makeSuite(TestSubtraction))
runner = unittest.TextTestRunner()
runner.run(suite)
# Output:
# ..
# ----------------------------------------------------------------------
# Ran 2 tests in 0.002s
#
# OK
In this example, we’ve created two test cases TestAddition
and TestSubtraction
, and added them to a test suite. Running this script will execute all the tests in the suite.
Unittest and CI/CD
Continuous integration/continuous deployment (CI/CD) is a method to frequently deliver apps to customers by introducing automation into the stages of app development. One crucial part of CI/CD is running tests automatically whenever changes are made to the codebase. Python’s unittest module can easily integrate with CI/CD systems like Jenkins, Travis CI, or GitHub Actions, ensuring that your code is always tested before it’s deployed.
Understanding Test Coverage
Test coverage is a measure of the amount of testing performed by a set of tests. It includes different levels like function coverage, statement coverage, branch coverage, etc. High test coverage can help you catch bugs and ensure that your code is working as expected. Python’s unittest module, combined with tools like coverage.py, can help you measure your test coverage and identify areas that need more testing.
Exploring Related Concepts
While unit testing is a fundamental part of software testing, it’s not the whole story. There are other types of testing like integration testing, functional testing, system testing, etc. Each type of testing has its own purpose and can help you catch different kinds of bugs. It’s worth exploring these concepts to get a more complete understanding of software testing.
Further Resources for Python Unittest Mastery
For those interested in diving deeper into Python unittest and related topics, here are some resources that might help:
- IOFlood’s Article on the benefits of Pytest explores the integration of Pytest with other Python testing libraries and frameworks.
Python Assert Guide | Ensuring Correctness in Your Code – Dive into the details of using assertions for code verification and debugging.
Type Checking in Python with mypy – A practical tutorial that explains mypy, a static type checker for Python, and how it helps catch type-related errors.
Python’s official unittest documentation – A comprehensive guide on unittest module, covering everything from basic use to advanced features.
Real Python’s guide on Python testing includes examples, tips, and use cases on Python testing, including unittest, pytest, doctest, and more.
Python Testing with pytest – An in-depth book on pytest, a popular alternative to unittest.
Remember, mastering Python unittest or any testing framework requires practice. Don’t be afraid to experiment, make mistakes, and learn from them. Happy testing!
Recap: Mastering Python Unittest
In this comprehensive guide, we’ve journeyed through the world of unit testing in Python using the built-in unittest module. From creating basic test cases to handling complex testing scenarios, we’ve covered a wide spectrum of techniques and concepts that will help you write effective and robust tests for your Python code.
We began with the basics, learning how to create test cases, define test methods, and use assertions in unittest. As we progressed, we ventured into advanced territory, discussing the setup and teardown methods, testing exceptions, and using mock objects. We also explored some common issues you might encounter while using unittest and provided solutions to help you overcome these challenges.
Along the way, we looked at alternative approaches to unit testing in Python, comparing unittest with other testing frameworks like pytest and doctest. Here’s a quick comparison of these libraries:
Library | Built-in | Ease of Use | Flexibility |
---|---|---|---|
unittest | Yes | Moderate | High |
pytest | No | High | High |
doctest | Yes | High | Low |
Whether you’re a beginner just starting out with Python unittest or an experienced developer looking to level up your unit testing skills, we hope this guide has given you a deeper understanding of Python unittest and its capabilities.
With its robustness and flexibility, Python unittest is a powerful tool for unit testing in Python. Happy coding!