Running Bash Scripts Made Easy: Programmer’s Guide
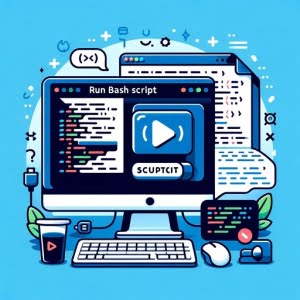
Ever found yourself struggling to run bash scripts? You’re not alone. Many developers find bash scripting a bit challenging, but think of bash scripts as a series of instructions that your computer follows step by step, like a well-rehearsed play.
Bash scripts are an integral part of Unix-like operating systems, serving as a powerful tool for automating tasks and managing system configurations.
In this guide, we’ll walk you through the process of running bash scripts, from the basics to more advanced techniques. We’ll cover everything from making a script executable, using the bash
command, to handling more complex scenarios such as running scripts with arguments or options, and even running scripts in the background.
So, let’s dive in and start mastering bash scripts!
TL;DR: How Do I Run a Bash Script?
To run a bash script in a Unix-like operating system, you use the
bash
command followed by the script name with the syntax,bash example_script.sh
. This command tells the system to interpret the script using the Bash shell, executing the commands within.
Here’s a simple example:
bash my_script.sh
In this example, my_script.sh
is the name of your bash script. When you run this command, the system will execute the instructions in the my_script.sh
script using the Bash shell.
This is just the basic way to run a bash script, but there’s much more to learn about bash scripting, including how to handle more complex scenarios such as running scripts with arguments or options, and even running scripts in the background. Continue reading for more detailed instructions and advanced usage scenarios.
Table of Contents
- The Basics of Running Bash Scripts
- Different Methods and Parameters of Script Running
- Exploring Alternative Shells
- Common Errors and How to Fix Them
- Best Practices and Optimization Tips
- Understanding Bash Scripts
- Bash Scripts in Larger Projects and Real-World Scenarios
- Expand Your Bash Scripting Knowledge
- Wrapping Up: Running Bash Scripts
The Basics of Running Bash Scripts
Before you can run a bash script, you need to make sure it’s executable. This is done using the chmod
command (short for ‘change mode’). This command changes the permissions of a file. Here’s how you can make your bash script executable:
chmod +x my_script.sh
In this example, my_script.sh
is the name of your bash script. The +x
option tells chmod
to make the file executable. Now, your script is ready to run!
To run the script, you use the bash
command followed by the name of the script. Here’s an example:
bash my_script.sh
Let’s break this down. The bash
command tells the system to interpret the script using the Bash shell. my_script.sh
is the name of your script.
Let’s consider a simple script that prints ‘Hello, World!’ to the console. Here’s what your my_script.sh
might look like:
echo 'Hello, World!'
And here’s how you would run it:
bash my_script.sh
# Output:
# Hello, World!
In this example, the echo
command prints ‘Hello, World!’ to the console. When you run the script using the bash
command, you should see ‘Hello, World!’ printed to your console.
Different Methods and Parameters of Script Running
Once you’ve grasped the basics of running Bash scripts, it’s time to delve into advanced techniques that can enhance your scripting capabilities. In this section, we will explore how to effectively utilize arguments, options, and running scripts in the background. By understanding these concepts, you can take your Bash scripting skills to the next level and ensure your scripts are flexible and efficient in various scenarios.
Running Scripts with Arguments and Options
As you progress, you’ll find that bash scripts can accept arguments and options, just like any other command-line program. These arguments and options can be accessed within your script using special variables.
Consider a script that greets the user by name. The name is passed as an argument when the script is run. Here’s what your greet.sh
script might look like:
echo 'Hello, ' $1 '!'
In this script, $1
is a special variable that represents the first argument passed to the script. Now, let’s run the script:
bash greet.sh Alice
# Output:
# Hello, Alice!
In this example, ‘Alice’ is passed as an argument to the greet.sh
script. The script then uses this argument to print a personalized greeting.
Running Scripts in the Background
Sometimes, you may want to run a script in the background while you continue to use the terminal for other tasks. You can do this by appending an ampersand (&
) to the end of your command.
Let’s say you have a script called background.sh
that takes a while to run. Here’s how you can run it in the background:
bash background.sh &
With this command, the script will run in the background, freeing up your terminal for other tasks. You can check the progress of background tasks using the jobs
command. If you want to bring a background task back to the foreground, you can use the fg
command.
Exploring Alternative Shells
While bash
is the most common shell used in Unix-like operating systems, there are other shells available, such as sh
, ksh
, and zsh
. You can run scripts using these shells in a similar way as you would with bash
. For example, to run a script using the zsh
shell, you would use the zsh
command followed by the name of the script.
zsh my_script.sh
This command tells the system to interpret my_script.sh
using the zsh
shell.
Running Scripts with Other Programming Languages
In addition to bash, you can write scripts in other programming languages such as Python or Perl. Here’s an example of a Python script that prints ‘Hello, World!’ to the console:
print('Hello, World!')
To run this script, you would use the python
command followed by the name of the script:
python my_script.py
# Output:
# Hello, World!
In this example, my_script.py
is a Python script that prints ‘Hello, World!’ to the console. The python
command tells the system to interpret the script using Python.
Choosing the Right Tool for the Job
When deciding which shell or programming language to use for your scripts, consider the nature of the task at hand. Bash is ideal for simple tasks and system administration. However, for more complex tasks, you might find a full-fledged programming language like Python or Perl more suitable.
Common Errors and How to Fix Them
While running bash scripts, you may encounter some common errors. Let’s look at some of these and how to fix them.
Permissions Error
One common error is the ‘Permission denied’ error. This occurs when you try to run a script that isn’t executable. Here’s what the error looks like:
bash: ./my_script.sh: Permission denied
To fix this error, you need to make the script executable using the chmod
command:
chmod +x my_script.sh
This command changes the permissions of my_script.sh
to make it executable.
Command Not Found Error
Another common error is the ‘Command not found’ error. This error occurs when the system can’t find the command you’re trying to run. Here’s what the error looks like:
my_script.sh: command not found
This error often occurs when the script isn’t in your system’s PATH. To fix this error, you can specify the full path to the script when you run it. Alternatively, you can add the directory containing the script to your system’s PATH.
Best Practices and Optimization Tips
When writing bash scripts, it’s important to follow best practices to ensure your scripts are efficient, maintainable, and easy to understand.
- Use Comments: Comments can help others understand what your script does. You can add a comment in bash by starting a line with a
#
symbol. Error Handling: Incorporate error handling in your scripts to make them more robust. You can use the
set -e
command at the start of your script to make it exit if any command fails.Use Functions: Functions can help you organize your script and avoid repeating code. They can make your script easier to read and maintain.
Variable Naming: Use meaningful names for your variables to make your script easier to understand. Avoid using single-letter variable names or names that are too generic.
Indentation: Consistent indentation can make your script easier to read. It can also help you spot errors more easily.
Understanding Bash Scripts
Bash scripts are essentially a series of commands that are executed in sequence. They are incredibly versatile, allowing you to automate tasks, perform complex operations, and manage system configurations.
A bash script is written in the Bash scripting language, which is a powerful command language that is standard on Unix-like operating systems such as Linux and macOS.
Consider this simple bash script:
#!/bin/bash
echo 'Starting script...'
echo 'Current directory:'
pwd
echo 'Done.'
When you run this script, it prints a start message, displays the current directory using the pwd
command, and then prints a completion message.
bash my_script.sh
# Output:
# Starting script...
# Current directory:
# /home/user
# Done.
Bash and Shell Scripting
Bash stands for ‘Bourne Again SHell’, a pun on the Bourne shell (sh
) that it replaces. Bash is a type of ‘shell’, or command language interpreter, that provides a command line interface for the Unix operating system.
Shell scripting is the process of automating tasks by writing a series of commands in a file (the script). When the script is run, the shell reads and executes these commands in sequence.
Bash scripts start with a ‘shebang’ (#!
) followed by the path to the bash interpreter (/bin/bash
or /usr/bin/env bash
). This line tells the system that the file is a bash script and should be executed as such.
Bash scripting is a powerful tool for system administrators and developers alike. By understanding how to run bash scripts, you can automate tasks, streamline your workflows, and even create your own command-line tools.
Bash Scripts in Larger Projects and Real-World Scenarios
Bash scripts have a wide range of applications, especially in larger projects and real-world scenarios. Their power and versatility make them an invaluable tool for developers and system administrators alike.
Automation with Bash Scripts
One of the most common uses of bash scripts is automation. By writing a script that performs a specific task, you can automate that task and save yourself the time and effort of performing it manually. For example, you could write a bash script to automate the process of backing up your files, updating your system, or deploying your code.
System Administration with Bash Scripts
Bash scripts are also widely used in system administration. They can be used to manage system configurations, monitor system performance, and automate routine tasks. For example, a system administrator might use a bash script to check the disk usage of a system and send an alert if it exceeds a certain threshold.
Software Development with Bash Scripts
In software development, bash scripts can be used to automate common tasks such as building, testing, and deploying code. For example, you might have a bash script that runs your unit tests and reports any failures.
Expand Your Bash Scripting Knowledge
Once you’ve mastered the basics of running bash scripts, you might want to learn some advanced techniques. These could include things like error handling, user input, and file operations. By learning these advanced techniques, you can write more robust and versatile scripts.
Other Scripting Languages
While bash is a powerful scripting language, it’s not the only one out there. Other scripting languages like Python, Perl, and Ruby offer their own unique features and advantages. Depending on the task at hand, one of these other languages might be more suitable.
Further Resources for Mastering Bash Scripts
If you’re interested in learning more about bash scripting, here are some resources that you might find helpful:
- GNU Bash Manual: This is the official manual for the Bash shell and contains a wealth of information about all aspects of bash scripting.
Devconnected – How to Run a Bash Script: This tutorial provides a step-by-step guide on running a Bash script in Linux with various methods, including executing the script directly, running it with bash command, and using shebang to specify the interpreter.
Bash Scripting Guide: This guide provides a detailed introduction to bash scripting, including numerous examples and exercises.
Wrapping Up: Running Bash Scripts
In this comprehensive guide, we’ve navigated through the intricacies of running bash scripts, a fundamental skill for any Unix-like system user or administrator.
We began with the basics, learning how to run a bash script and make it executable. We then delved into more advanced techniques, such as running scripts with arguments, options, and even running them in the background. We also explored alternative approaches, including using different shells and programming languages to run scripts.
Along our journey, we addressed common errors you might encounter when running bash scripts and provided solutions to overcome these challenges. We also discussed best practices to ensure your scripts are efficient, maintainable, and easy to understand.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Bash | High | Low | System administration, automation |
Python | High | Moderate | Complex scripting tasks |
Other Shells (sh, ksh, zsh) | Moderate | Low | Specific system environments |
Whether you’re just starting out with bash scripting or you’re looking to enhance your existing skills, we hope this guide has provided you with a deeper understanding of how to run bash scripts and their applications.
Bash scripting is a powerful tool, enabling you to automate tasks and streamline workflows. Now, you’re well-equipped to leverage these benefits. Happy scripting!