‘For Loop’ in Bash: Shell Script Conditional Statements
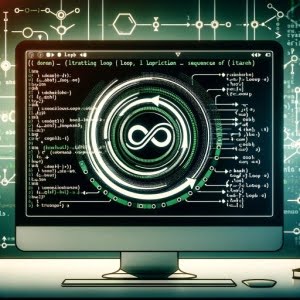
Are you finding it challenging to master for loops in Bash? You’re not alone. Many developers find themselves tangled in the syntax and logic of for loops. But, think of Bash for loops as a well-oiled machine, automating repetitive tasks in your scripts, making your life easier.
In this guide, we’ll walk you through the process of using for loops in Bash, from the basics to more advanced techniques. We’ll cover everything from writing a simple for loop to dealing with more complex scenarios like nested loops and loops with arrays. We’ll also discuss common issues you might encounter when using for loops in Bash and how to troubleshoot them.
Let’s dive in and start mastering for loops in Bash!
TL;DR: How Do I Use a For Loop in Bash?
To create a
for loop
in Bash, you specify the variable, the list of values, and the action to be performed, for examplefor variable in list...
.
A basic for loop in Bash can be written as follows:
for i in {1..5}
do
echo $i
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we’ve created a simple for loop that iterates over the numbers 1 to 5 and echoes each number. The variable ‘i’ takes on each value in the list {1..5}
, and for each value, the echo
command is executed, printing the number.
This is a basic way to use a for loop in Bash, but there’s much more to learn about controlling the flow of your scripts. Continue reading for more detailed examples and advanced looping techniques.
Table of Contents
Understanding the Basics of Bash For Loop
In Bash scripting, a for loop is a control flow statement that allows code or commands to be repeatedly executed based on a condition. Let’s break down the syntax of a basic for loop in Bash.
The basic syntax of a for loop in Bash is as follows:
for variable in list
do
command1
command2
...
done
Here, the variable
is a placeholder that represents each element in the list
sequentially. The command1
, command2
, etc., are the commands that will be executed for each element in the list.
Let’s look at a practical example to understand better. Suppose we have a list of names and we want to print a personalized greeting for each name. Here’s how we can do it using a for loop in Bash:
names='Alice Bob Charlie'
for name in $names
do
echo "Hello, $name!"
done
# Output:
# Hello, Alice!
# Hello, Bob!
# Hello, Charlie!
In this example, names
is a variable that holds a list of names. We then create a for loop where name
is the variable that will take on each value in the names
list. For each name, the echo
command is executed, printing a personalized greeting.
The key takeaway here is that a for loop in Bash allows you to automate repetitive tasks, making your scripts more efficient and easier to manage.
Delving into Advanced Bash For Loop
As you become more comfortable with Bash for loops, you can start to explore more complex uses, such as nested loops and loops with arrays.
Nested Loops in Bash
A nested loop is a loop within a loop. It allows you to iterate over multiple lists. Here’s an example to illustrate this concept:
for i in {1..3}
do
for j in {a..c}
do
echo "$i$j"
done
done
# Output:
# 1a
# 1b
# 1c
# 2a
# 2b
# 2c
# 3a
# 3b
# 3c
In this example, we have a for loop that iterates over the numbers 1 to 3. Inside this loop, we have another for loop that iterates over the letters ‘a’ to ‘c’. For each iteration of the outer loop, the inner loop runs completely, resulting in all possible combinations of numbers and letters.
For Loops with Arrays
Bash for loops are not just limited to iterating over lists. They can also iterate over arrays. Here’s how you can do it:
array=(apple banana cherry)
for fruit in "${array[@]}"
do
echo $fruit
done
# Output:
# apple
# banana
# cherry
In this example, we have an array of fruits. The for loop iterates over each element in the array and prints it. The notation “${array[@]}” is used to represent all elements of the array.
These advanced uses of Bash for loops allow you to handle more complex tasks and structures in your scripts. Keep practicing and experimenting with different scenarios to become more proficient.
Exploring Alternatives to Bash For Loop
While for loops are incredibly useful in Bash, they aren’t the only way to control the flow of your scripts. Let’s explore a couple of other types of loops you can use: the while
and until
loops.
The While Loop
The while
loop in Bash executes a block of commands as long as a condition is true. Here’s a basic example:
counter=1
while [ $counter -le 5 ]
do
echo $counter
((counter++))
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the while
loop continues to echo the counter
variable as long as it is less than or equal to 5. After each iteration, the counter
variable is incremented by 1 using the ((counter++))
syntax.
The Until Loop
The until
loop is similar to the while
loop, but it does the opposite. It executes a block of commands as long as a condition is false. Here’s how you can use an until
loop:
counter=1
until [ $counter -gt 5 ]
do
echo $counter
((counter++))
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the until
loop continues to echo the counter
variable until it becomes greater than 5. Just like in the while
loop example, the counter
variable is incremented by 1 after each iteration.
While for loops are great for iterating over lists or arrays, while
and until
loops are more suitable when you need to execute a block of commands based on a condition. By understanding these different types of loops, you can choose the most efficient way to control the flow of your Bash scripts.
Troubleshooting Common Issues in Bash For Loop
While using for loops in Bash, you might encounter some common issues. Let’s discuss a few of these problems and their solutions.
Syntax Errors
One of the most common issues when writing for loops in Bash is syntax errors. These can occur if you forget to include certain key elements of the for loop structure. For example:
for i in {1..5}
echo $i
done
# Output:
# Syntax error: "do" or "{" expected
In this code, we’ve forgotten to include the do
keyword. The correct syntax should be:
for i in {1..5}
do
echo $i
done
# Output:
# 1
# 2
# 3
# 4
# 5
Infinite Loops
Another common issue is creating an infinite loop. This happens when the loop condition always evaluates to true. Here’s an example:
for (( ; ; ))
do
echo "This is an infinite loop. Press CTRL+C to stop."
done
In this code, there’s no condition specified for the for loop, so it will run indefinitely. To stop it, you need to press CTRL+C in your terminal.
Using Variables in For Loops
When using variables in for loops, be careful with variable scope. A variable defined inside a for loop is only accessible within that loop. Here’s an example:
for i in {1..5}
do
loop_variable=$i
done
echo $loop_variable
# Output:
# 5
In this code, loop_variable
is only accessible within the for loop. When we try to echo loop_variable
outside the loop, it prints the last value it was assigned in the loop.
Understanding these common issues and their solutions can help you write more robust and error-free Bash scripts.
Understanding Loops and Control Structures
Before we delve deeper into the usage of for loops in Bash, it’s crucial to understand the fundamental concepts of loops and control structures in programming.
What are Loops?
In programming, a loop is a sequence of instructions that is continually repeated until a certain condition is reached. Essentially, a loop allows you to execute a block of code multiple times. For example, if you want to print numbers 1 to 5, you can do it manually, or you can use a loop to automate the task.
Here’s a simple Bash for loop that does this:
for i in {1..5}
do
echo $i
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the for loop iterates over the numbers 1 to 5 and echoes each number. This is much more efficient than writing five separate echo commands.
Control Structures in Programming
Control structures determine the flow of your program’s execution. They allow your program to make decisions based on certain conditions. The basic types of control structures in programming include sequence, selection, and repetition (loops).
- Sequence: This is the default control structure where instructions are executed one after the other in the order they appear.
- Selection: This control structure allows your program to choose between different paths based on certain conditions (e.g., if-else statements).
- Repetition (Loops): This control structure allows your program to execute a block of code multiple times (e.g., for, while, and do-while loops).
Understanding these basic concepts will provide a strong foundation as you learn more about Bash for loops and other control structures.
Applying Bash For Loop in Larger Projects
For loops in Bash are not just for small tasks or scripts. They play a crucial role in larger projects where you need to automate repetitive tasks, manipulate data, or control the flow of your scripts.
Consider a scenario where you’re handling a large dataset, and you need to perform the same operation on each data point. A for loop can be extremely useful in such cases. Here’s an example:
data_points=(23 56 89 45 67 34 78 90)
for data in "${data_points[@]}"
do
processed_data=$((data * 2))
echo $processed_data
done
# Output:
# 46
# 112
# 178
# 90
# 134
# 68
# 156
# 180
In this example, we have an array of data points. We use a for loop to iterate over each data point, process it (in this case, multiply it by 2), and then print it. This is a simple example, but it illustrates how for loops can be used in data manipulation tasks in larger projects.
Exploring Related Topics
As you continue to master Bash for loops, you might want to explore related topics that can further enhance your Bash scripting skills. These topics include conditional statements (like if-else statements), functions, and other control structures like while and until loops.
Further Resources for Mastering Bash For Loop
To further your understanding of Bash for loops and related topics, here are a few resources you might find helpful:
- Bash Scripting For Loop: This GeeksforGeeks article provides an overview of the for loop in Bash scripting.
Bash For Loop Examples: This Cyberciti article provides a collection of examples and explanations for using the for loop in Bash scripting.
Advanced Bash-Scripting Guide: The Advanced Bash-Scripting Guide, hosted by TLDP (The Linux Documentation Project), is a comprehensive resource for Bash scripting.
Remember, mastering any programming concept takes practice. So, keep experimenting with different for loop scenarios and challenges. Happy scripting!
Wrapping Up: Mastering Bash For Loop
In this comprehensive guide, we’ve delved into the world of Bash for loops, a powerful tool for automating repetitive tasks in Bash scripts.
We began with the basics, understanding how to use a for loop in Bash, with simple code examples and their explanations. We discussed the syntax, variables, and how to control the flow of the loop. We then ventured into more advanced territory, discussing more complex uses of for loops in Bash, such as nested loops and loops with arrays. We provided intermediate-level code examples and an analysis of each.
Along the way, we also discussed other types of loops in Bash, such as while and until loops, and when to use them over for loops. We tackled common issues one may encounter when using for loops in Bash, such as syntax errors and infinite loops, providing solutions and workarounds for each issue.
Here’s a quick comparison of the loop types we’ve discussed:
Loop Type | Use Case | Complexity |
---|---|---|
For Loop | Iterating over lists or arrays | Low to High |
While Loop | Executing a block of commands as long as a condition is true | Moderate |
Until Loop | Executing a block of commands as long as a condition is false | Moderate |
Whether you’re just starting out with Bash for loops or looking to level up your Bash scripting skills, we hope this guide has given you a deeper understanding of Bash for loops and their capabilities. With practice and application, you’ll be able to write more efficient and powerful Bash scripts. Happy scripting!