Install Specific Package Versions with NPM | Step-by-Step
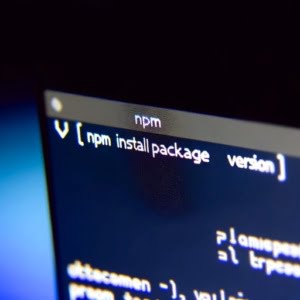
At IOFlood, we have encountered situations where we need to install a specific version of a package to ensure compatibility with our projects. To help others with this challenge, we have created this guide on using npm and the command npm install @
. By following the steps outlined in tis guide, you’ll be able to manage package versions in your projects and minimize compatibility issues.
This guide will walk you through the process of using npm to install specific package versions, ensuring you have the right tools for your project. By mastering how to install specific package versions with npm command, you’ll gain greater control over your development environment, leading to more stable and predictable outcomes.
Ready to take control of your package versions? Let’s dive into the world of npm installation with precision.
TL;DR: How Do I Install a Specific Version of a Package Using npm?
To install a specific version of a package with npm, use the command
npm install @
.
Here’s a quick example:
npm install [email protected]
# Output:
# '[email protected] installed'
This command installs version 4.17.15 of lodash, ensuring you have the exact functionality and compatibility needed for your project. By specifying the version number after the package name, you’re telling npm to fetch and install that particular version from the npm registry. This is crucial for maintaining consistency across development environments and avoiding unexpected behavior from newer package versions.
Keep reading for more detailed instructions, advanced options, and troubleshooting tips.
Table of Contents
NPM Install Version: Beginner’s Guide
Step-by-Step to Specific Versions
Embarking on your journey with npm can feel like navigating a vast ocean of packages. One of the first skills to master is installing a specific version of a package. This precision not only ensures compatibility but also project consistency. Let’s dive into how you can achieve this with a simple command.
Imagine you’re working on a project that requires version 1.0.0 of a package named cool-package
. To install this specific version, you would use the following command:
npm install [email protected]
# Output:
# '+ [email protected] added'
In this example, npm install [email protected]
tells npm to look for version 1.0.0 of cool-package
in the npm registry and install it in your project. This command is straightforward but powerful, allowing you to specify exactly which version of a package you need.
Understanding how npm resolves version requests is crucial. When you specify a version number, npm searches its vast registry for that exact version. If found, it’s downloaded and added to your project, ensuring that the specific version you requested is what’s used. This process is vital for maintaining consistency across development environments and avoiding the pitfalls of automatically updating to newer versions that might introduce breaking changes or incompatibilities.
By mastering this simple command, you’re taking a significant step towards more predictable and stable development outcomes.
Advanced npm Version Control
Beta Versions and Version Ranges
As you grow more comfortable with npm and begin to tackle more complex projects, you’ll find yourself needing to use advanced features of npm install. These include installing beta versions of packages, specifying version ranges, and understanding how npm handles semantic versioning (semver).
Installing Beta Versions
Beta versions of packages are often used for testing new features before they are officially released. To install a beta version of a package, you might use a command like the following:
npm install package-name@beta
# Output:
# 'package-name@beta-version installed'
This command installs the latest beta version of package-name
. The @beta
tag directs npm to fetch this specific pre-release version. It’s crucial for testing new features in a controlled environment before they make their way into the stable release.
Using Version Ranges
Version ranges allow you to specify a range of versions that you are willing to accept for a package. This can be particularly useful when you want to ensure compatibility without locking down a specific version. An example of specifying a version range is:
npm install package-name@">=1.0.0 <2.0.0"
# Output:
# 'Compatible version of package-name installed'
This command installs a version of package-name
that is compatible within the specified range. npm’s handling of version ranges is based on semantic versioning principles, allowing for flexibility while maintaining compatibility.
Understanding Semantic Versioning
Semantic versioning, or semver, is a system for versioning software in a way that conveys meaning about the underlying changes. It’s structured as major.minor.patch (e.g., 2.0.1), where major changes introduce backward-incompatible updates, minor changes add functionality in a backward-compatible manner, and patch changes make backward-compatible bug fixes.
Understanding semver is crucial for managing package dependencies effectively. It helps you make informed decisions about updating packages and resolving version conflicts. npm’s version resolution algorithm uses semver to determine which version of a package to install, ensuring compatibility and reducing the risk of introducing breaking changes.
By mastering these advanced npm install features, you’ll enhance your ability to manage package versions precisely, leading to more stable and reliable projects.
Alternative npm Version Strategies
npm ci: Consistency in Installation
When managing package versions, especially in team environments or continuous integration (CI) setups, ensuring that everyone uses exactly the same package version is paramount. An alternative to the standard npm install
is the npm ci
command, which provides a more consistent installation process by using the package-lock.json
file.
npm ci
# Output:
# 'Installed packages based on package-lock.json'
The npm ci
command deletes your node_modules
folder and installs the exact versions listed in your package-lock.json
, mirroring the exact environment. This is crucial for avoiding discrepancies between development environments and production, ensuring that your project runs smoothly everywhere.
npx: Running Packages with Ease
Another advanced technique is leveraging npx
, a tool that comes with npm 5.2.0 and higher, allowing you to run packages without installing them globally. This is particularly useful for running packages on a one-off basis or when testing different versions of a package.
npx package-name@version
# Output:
# 'Executed package-name@version'
In this example, npx package-name@version
runs a specific version of package-name
directly, without the need to permanently install it. This approach is excellent for quick tests or running scripts with different versions, providing flexibility and reducing global package clutter.
Comparing Methods
Both npm ci
and npx
offer distinct advantages over direct version installation with npm install
. npm ci
ensures that your project dependencies are consistent across all environments, a critical factor for project stability. On the other hand, npx
provides the flexibility to run any version of a package instantly, perfect for testing and temporary usage.
Understanding these alternative approaches enhances your npm toolkit, allowing you to choose the best method for your specific scenario. Whether you prioritize consistency with npm ci
or flexibility with npx
, mastering these tools will elevate your package management strategy.
Troubleshooting npm Version Issues
Incorrect Version Installation
One of the most common hurdles when working with npm is inadvertently installing the wrong version of a package. This can disrupt your project’s functionality and lead to compatibility issues. To verify the installed package version, you can use the npm list
command.
npm list package-name
# Output:
# package-name@installed-version
This command displays the version of package-name
that’s currently installed in your project. If the installed version doesn’t match your project requirements, you can reinstall the correct version using the npm install package-name@specific-version
command, ensuring you specify the version you need.
Handling Dependency Conflicts
Dependency conflicts are another challenge that can arise when managing package versions. These occur when different parts of your project require different versions of the same package. To address this, npm offers the npm dedupe
command, which attempts to resolve these conflicts by optimizing the dependency tree.
npm dedupe
# Output:
# 'Optimized dependency tree'
After running npm dedupe
, npm tries to find a common version that satisfies all dependent packages, reducing the number of versions installed and potentially resolving conflicts.
Resolving Version Mismatches
Version mismatches can occur when your project’s package.json
specifies a different version than what’s installed. This can lead to unexpected behavior or errors. To ensure you’re using the intended version, it’s crucial to align the version in your package.json
with the installed version. If discrepancies are found, updating your package.json
and running npm install
again can rectify this issue.
Understanding these common issues and how to address them is essential for maintaining a healthy npm environment. By adopting these troubleshooting techniques and considerations, you’ll be better equipped to manage your project’s dependencies effectively, ensuring a smoother development process.
Core Concepts of NPM
Package Management Essentials
NPM, standing for Node Package Manager, is the cornerstone of modern JavaScript development. It serves as a public repository for Node.js packages, offering a plethora of libraries and tools to streamline project development. Understanding npm’s role in package management is crucial for leveraging its full potential.
The Role of package.json
Every npm project begins with a package.json
file, a manifest that contains metadata about the project. This includes the project’s name, version, scripts, and, importantly, dependencies. Here’s a basic example:
{
"name": "your-project",
"version": "1.0.0",
"dependencies": {
"express": "^4.17.1"
}
}
This package.json
file declares a dependency on the Express framework, specifying that versions compatible with 4.17.1 are acceptable. The caret (^) symbol before the version number indicates that minor updates and patches are allowed, adhering to semantic versioning rules.
Understanding package-lock.json
While package.json
provides a broad overview of your project’s dependencies, package-lock.json
goes a step further. It locks down the exact versions of each package (and their dependencies) that your project uses. This ensures that all installations or deployments of your project use the same versions, preventing discrepancies between environments.
{
"name": "your-project",
"version": "1.0.0",
"lockfileVersion": 1,
"dependencies": {
"express": {
"version": "4.17.1",
"resolved": "https://registry.npmjs.org/express/-/express-4.17.1.tgz",
"integrity": "sha512-...",
"requires": {
"accepts": "~1.3.7",
"array-flatten": "1.1.1"
}
}
}
}
This file is automatically generated by npm when you install packages and is crucial for achieving consistent builds. It precisely pins down the version and source of each package, ensuring that every team member and deployment pipeline works with the exact same setup.
Navigating the NPM Registry
The npm registry is a vast database of public and private packages available for installation. It’s where npm looks up package versions when you run an npm install
command. Understanding how npm interacts with this registry is key to mastering npm version control. When you specify a version or version range in your npm install
command, npm queries the registry to find the best match that satisfies your requirements.
Grasping these fundamentals provides a solid foundation for working with npm and managing package versions effectively. It’s the bedrock upon which safe, reliable, and predictable software development practices are built, ensuring that projects remain stable as they evolve.
Applications of npm Install Versions
The Ripple Effect on Development Workflows
Understanding and utilizing npm install
version commands not only affects the immediate task of adding a package to your project but also has profound implications on your overall development workflow. It ensures consistency across development, staging, and production environments, which is crucial for the smooth operation of Continuous Integration (CI) environments and deployment strategies.
For instance, consider the impact of a minor version update that unintentionally breaks backward compatibility. Without specific version control, this update could be automatically applied, potentially causing unexpected issues during deployment. By specifying package versions, you mitigate these risks, ensuring that updates are deliberate and tested.
Automating npm Updates
Automation plays a vital role in modern development practices, especially in managing dependencies. npm offers tools like npm update
and npm outdated
to help manage package updates more efficiently.
npm outdated
# Output:
# Package Current Wanted Latest Location
# lodash 4.17.15 4.17.20 4.17.20 your-project
This command checks for packages that are outdated in your project. It provides a clear overview of your current versions versus the latest available versions, helping you make informed decisions about updating.
After reviewing, you might decide to update lodash
to its latest version. This is where npm update
comes into play:
npm update lodash
# Output:
# '+ [email protected] updated'
The npm update
command updates the specified package to the latest version, adhering to the version rules set in your package.json
. This ensures that your project stays up-to-date with the latest features and bug fixes without breaking existing functionality.
Further Resources for npm Version Mastery
To deepen your understanding of npm and its version management capabilities, here are three resources that offer valuable insights:
- The official npm Documentation provides comprehensive guides on every aspect of npm, including version control.
NodeSource Blog offers insightful articles on Node.js and npm, including tips for optimizing your Node.js applications.
npm Trends is a useful tool for comparing the popularity and download trends of different npm packages.
Leveraging these resources will enhance your npm skills, making you more adept at managing package versions and ensuring the stability and reliability of your projects.
Recap: Mastering npm Install Version
In this comprehensive guide, we’ve navigated the complexities of using npm to install specific package versions. This journey has equipped you with the knowledge to precisely control the versions of packages in your projects, ensuring compatibility and stability.
We began with the basics, learning how to install a specific version of a package using the simple command npm install package-name@version
. This foundational skill is crucial for maintaining consistency across your development environment. We then explored more advanced techniques, such as installing beta versions, using version ranges, and understanding semantic versioning (semver) to manage dependencies more effectively.
Our exploration didn’t stop there. We delved into alternative approaches for version management, discussing the benefits of npm ci
for consistent installations and npx
for running packages without global installation. These tools offer flexibility and control, enhancing your project’s integrity.
Approach | Use Case | Benefits |
---|---|---|
npm install | Installing specific package versions | Precise control over package versions |
npm ci | Consistent installations | Ensures environment consistency |
npx | Running packages without installation | Offers flexibility and reduces global clutter |
Whether you’re just starting out with npm or looking to refine your version management strategy, this guide has provided you with a deeper understanding of npm’s capabilities. With these tools and techniques at your disposal, you’re well-equipped to tackle any project challenges that come your way.
The ability to install specific versions of packages using npm is a powerful tool in your development arsenal. By mastering these commands and strategies, you ensure that your projects are built on a stable, reliable foundation. Happy coding!