How to Enable CORS in Node.js | NPM User’s Guide
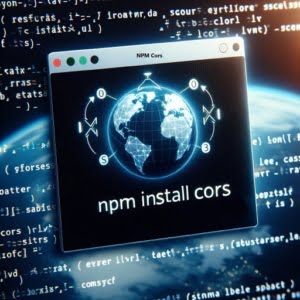
Enabling CORS in a Node.js application is crucial for handling cross-origin requests smoothly. After tackling this challenge in our projects at IOFlood, we decided to compile this comprehensive guide on how to use npm to enable CORS. By following the step-by-step instructions, you’ll be able to ensure secure communication with external resources in your Node.js applications.
This guide will walk you through enabling CORS in your Node.js applications using npm packages, covering everything from basic setup to advanced configuration. Whether you’re a beginner looking to understand the basics or an experienced developer aiming for advanced configurations, this guide has something for everyone.
Let’s embark on this journey together and unlock the full potential of CORS in your Node.js projects.
TL;DR: How Do I Enable CORS in My Node.js Application Using npm?
To enable CORS in your Node.js application, you will need to install it with npm,
npm install cors
. You can then configure it, and enable it with the commandapp.use(cors())
.
Here’s a quick example to get you started:
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
// Your routes go here
app.listen(3000, () => console.log('Server running on port 3000'));
# Output:
# Server running on port 3000
In this snippet, we set up a basic Express server and enable CORS by using the cors
middleware with its default settings. By doing so, your Node.js application can accept cross-origin requests from any source. If you need to restrict or customize the origins, keep reading for more detailed instructions and advanced configurations.
This is just the beginning of mastering CORS in Node.js. Dive deeper into our guide for more insights and advanced techniques.
Table of Contents
Installing CORS with npm
Before diving into the nitty-gritty of CORS, let’s start with the basics. CORS stands for Cross-Origin Resource Sharing. It’s a mechanism that allows or restricts requested resources on a web server depending on where the HTTP request was initiated. This security feature is crucial because it prevents malicious sites from reading sensitive data from another site without permission.
Why CORS Matters
Imagine you’re building a web application that fetches user data from an API. Without CORS, browsers would block your app from accessing the API if it’s hosted on a different domain, labeling it as a potential security threat. CORS settings on the server tell the browser it’s safe to allow the request, making your app functional and secure.
Enabling CORS in Node.js
To get started, you’ll first need to install the cors
package via npm, Node.js’s package manager. Open your terminal and run the following command:
npm install cors
This command installs the cors
package into your Node.js project, adding it to your package.json
dependencies.
Integrating CORS into Your Application
After installing the cors
package, it’s time to integrate it into your Express application. Here’s a simple example of how to do it:
const express = require('express');
const cors = require('cors');
const app = express();
// Enable CORS for all requests
app.use(cors());
app.get('/data', (req, res) => {
res.json({ message: 'This route is CORS-enabled for all origins!' });
});
app.listen(3000, () => console.log('Example app listening on port 3000!'));
# Output:
# Example app listening on port 3000!
In this code snippet, we’ve created a basic Express server and enabled CORS for all requests using app.use(cors());
. The /data
route sends a JSON response, which can now be accessed from any origin. This setup is ideal for development and testing environments where you want to allow requests from any source.
By enabling CORS in your Node.js application, you’re taking a significant step towards building secure and accessible web applications. As you become more familiar with CORS and its configurations, you’ll be able to fine-tune which requests are allowed, ensuring your app’s data stays protected while still being accessible where it’s needed.
Advanced CORS Configurations
After mastering the basics of enabling CORS in your Node.js applications, it’s time to dive deeper into more sophisticated configurations. These advanced settings allow you to fine-tune how your application handles cross-origin requests, enhancing security and functionality.
Specific Route CORS
One common requirement is enabling CORS for specific routes rather than applying it globally to all routes. This targeted approach ensures that only certain parts of your application are accessible from other origins. Here’s how you can achieve this:
const express = require('express');
const cors = require('cors');
const app = express();
// CORS-enabled for a single route
app.get('/api/data', cors(), (req, res) => {
res.json({ message: 'This route is CORS-enabled for all origins!' });
});
app.listen(3000, () => console.log('Server listening on port 3000'));
# Output:
# Server listening on port 3000
In the above example, CORS is enabled only for the /api/data
route. This is done by passing the cors()
middleware directly to the route. It allows for more granular control over which parts of your application can interact with different origins.
Custom CORS Settings
For scenarios that require more control over the CORS policy, such as specifying allowed origins, methods, or headers, the cors
package offers a flexible configuration object. Here’s how you can customize your CORS settings:
const express = require('express');
const cors = require('cors');
const corsOptions = {
origin: 'https://example.com',
methods: 'GET,POST',
allowedHeaders: 'Content-Type,Authorization'
};
const app = express();
app.use(cors(corsOptions));
app.get('/', (req, res) => {
res.send('CORS settings customized!');
});
app.listen(3000, () => console.log('Custom CORS policy in action'));
# Output:
# Custom CORS policy in action
This configuration specifies that only requests from ‘https://example.com’, using the GET or POST methods, and including the specified headers, will be allowed. By customizing the CORS policy in this way, you enhance your application’s security by ensuring that only trusted requests are accepted.
Understanding and implementing these advanced CORS configurations in your Node.js applications using npm can significantly impact your application’s security and functionality. It allows for a more controlled and secure environment, ensuring that your application behaves exactly as intended.
Exploring CORS Alternatives
While the cors
npm package is a popular choice for handling CORS in Node.js applications, it’s not the only option. For developers seeking more control or different features, alternative npm packages and manual configurations can offer tailored solutions. Let’s delve into some of these alternatives, comparing them to the cors
package to help you make an informed decision.
Using Helmet for Security Headers
Helmet is a middleware for Express applications that sets various HTTP headers to secure your app. While not exclusively for CORS, it includes the helmet.crossOriginResourcePolicy()
function, which can be used to control cross-origin requests.
const express = require('express');
const helmet = require('helmet');
const app = express();
app.use(helmet());
app.use(helmet.crossOriginResourcePolicy({ policy: "cross-origin" }));
app.get('/', (req, res) => {
res.send('Using Helmet for enhanced security.');
});
app.listen(3000, () => console.log('Helmet in action'));
# Output:
# Helmet in action
In this example, Helmet enhances your application’s security, including a policy to manage cross-origin requests. This approach is beneficial for projects that require a broader security framework, not just CORS management.
Manual CORS Configuration
For those preferring to avoid third-party packages, manual CORS configuration is an option. This approach involves setting the CORS headers directly in your responses. It offers maximum control but requires a detailed understanding of CORS policies.
const express = require('express');
const app = express();
app.use((req, res, next) => {
res.header('Access-Control-Allow-Origin', '*');
res.header('Access-Control-Allow-Methods', 'GET,POST,PUT,DELETE,OPTIONS');
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
next();
});
app.get('/', (req, res) => {
res.send('Manual CORS configuration in place.');
});
app.listen(3000, () => console.log('Custom CORS setup running'));
# Output:
# Custom CORS setup running
This snippet demonstrates how to manually set CORS headers on all responses. This method gives you the freedom to specify exactly which origins, methods, and headers are permitted, making it a powerful option for complex applications.
Choosing the Right Approach
Each method of handling CORS in Node.js has its advantages and drawbacks. The cors
package offers simplicity and ease of use, making it an excellent choice for most projects. Helmet provides a broader security solution, including but not limited to CORS. Manual configuration, while complex, offers the most control over your application’s cross-origin requests. Your choice should be guided by your project’s specific needs and your comfort level with managing security configurations.
Troubleshooting CORS Issues
When implementing CORS in your Node.js applications using npm, you might encounter several common errors. These issues can halt your progress, but with the right knowledge, they can be quickly resolved. Let’s explore some of these common errors, their causes, and how to fix them.
No ‘Access-Control-Allow-Origin’ Header
One of the most frequent errors related to CORS is the absence of the ‘Access-Control-Allow-Origin’ header in the response. This error occurs when your server doesn’t include the necessary CORS headers in its response to a cross-origin request, leading browsers to block the request for security reasons.
To resolve this issue, ensure your server explicitly sets the ‘Access-Control-Allow-Origin’ header. Here’s an example of setting this header for all incoming requests:
const express = require('express');
const app = express();
app.use((req, res, next) => {
res.header('Access-Control-Allow-Origin', '*');
next();
});
app.listen(3000, () => console.log('CORS headers set for all origins'));
# Output:
# CORS headers set for all origins
In this code block, we’re configuring our Express app to include the ‘Access-Control-Allow-Origin’ header with a value of ‘‘ in every response. This setup allows all domains to make requests to your server. While this is suitable for development or open APIs, you’ll want to replace ‘‘ with specific domain names for production environments to enhance security.
Testing and Debugging CORS Configurations
Properly testing and debugging your CORS configurations is crucial for ensuring your application behaves as expected. Here are some best practices:
- Use Browser Developer Tools: Most modern browsers include developer tools that allow you to inspect network requests and responses. Look for CORS-related errors in the console and examine the request and response headers.
Leverage Testing Tools: Tools like Postman and curl can simulate cross-origin requests, helping you test your server’s CORS configurations without needing to set up a client-side application.
Logging Middleware: Implement logging middleware in your Node.js application to log all incoming requests and their headers. This can help you identify issues with incoming requests that might not be correctly setting the expected headers.
By understanding these common CORS errors and how to troubleshoot them, you can ensure a smoother development process and create more secure, accessible web applications. Remember, the goal of CORS is not to prevent requests but to secure them, enabling your applications to safely share resources across different origins.
Understanding CORS Fundamentals
To master CORS (Cross-Origin Resource Sharing) in Node.js applications, it’s crucial to grasp the underlying concepts that make it necessary and beneficial. CORS is a security feature implemented by web browsers to prevent malicious websites from accessing resources and data from another domain without permission. This mechanism is built on the same-origin policy, a critical aspect of web application security.
The Same-Origin Policy
The same-origin policy is a foundational security measure that restricts how documents or scripts loaded from one origin can interact with resources from another origin. An origin is defined by the scheme (protocol), host (domain), and port of a URL. For example, https://example.com
and https://example.com:443
are considered the same origin, but http://example.com
(different scheme) and https://sub.example.com
(different host) are not.
This policy prevents a malicious site from reading sensitive information from another site. However, it also poses challenges for legitimate cross-origin requests, such as API calls from a web application hosted on a different domain than its backend server.
Preflight Requests and CORS Headers
CORS works by adding specific HTTP headers to requests and responses. These headers tell the browser that a web application running on one origin has permission to access selected resources from a server on a different origin.
A preflight request is an automatic process that occurs with certain types of requests, sending an HTTP OPTIONS request to the server before the actual request. This preflight checks if the actual request is safe to send, based on the CORS policies of the server.
Here’s an example demonstrating a preflight request and the CORS headers involved:
// Server-side express code to handle preflight requests
const express = require('express');
const cors = require('cors');
const app = express();
app.options('/api/resource', cors()) // Enable preflight request for /api/resource path
app.get('/api/resource', cors(), (req, res) => {
res.json({ message: 'CORS-enabled response' });
});
app.listen(3000, () => console.log('Handling preflight requests'));
# Output:
# Handling preflight requests
In this code block, the app.options()
method is used to explicitly handle preflight requests for the /api/resource
route, ensuring that the actual request that follows is accepted by the server. The cors()
middleware is applied to both the preflight and actual requests, demonstrating how to configure CORS for specific HTTP methods and routes.
Understanding these fundamental concepts of CORS, the same-origin policy, preflight requests, and the importance of HTTP headers in enabling cross-origin resource sharing is essential for developing secure and functional web applications. By implementing CORS correctly, you can ensure that your Node.js applications can communicate safely with resources on different origins, enhancing the user experience without compromising security.
Practical Usage Cases of CORS
As web technologies evolve, CORS (Cross-Origin Resource Sharing) continues to play a pivotal role in the architecture of modern web applications, including single-page applications (SPAs), APIs, and microservices. Understanding and implementing CORS correctly is not just about allowing your Node.js applications to communicate with other origins; it’s about securing and optimizing your applications for development and production environments.
CORS in Single-Page Applications
Single-page applications offer a dynamic user experience by loading content asynchronously without refreshing the page. However, SPAs often need to request resources from different origins, making CORS a critical consideration.
// Example of an SPA making a cross-origin request
fetch('https://api.example.com/data', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
},
credentials: 'include',
})
.then(response => response.json())
.then(data => console.log(data));
# Output:
# {"message": "Data fetched successfully"}
In this example, a SPA fetches data from an API hosted on a different origin. The browser automatically handles the CORS preflight request, ensuring the API server’s CORS policy permits the request. This seamless integration highlights the importance of CORS in SPAs for providing a smooth user experience.
CORS in APIs and Microservices
For APIs and microservices, CORS enables the safe exposure of endpoints to web clients from different origins. This is particularly vital in microservices architectures where services might be spread across different domains.
// Enabling CORS for an API endpoint in a microservice
app.use('/api/service', cors(), (req, res) => {
res.json({ message: 'CORS-enabled microservice endpoint' });
});
# Output:
# CORS-enabled microservice endpoint
This code snippet demonstrates how to enable CORS for a specific API endpoint in a microservice architecture. By doing so, the microservice can accept requests from web applications hosted on different origins, facilitating a distributed system’s interconnectedness.
Further Resources for Mastering CORS
To deepen your understanding of CORS and its applications in modern web development, consider exploring these resources:
- Understanding CORS – An educational resource on Cross-Origin Resource Sharing (CORS).
CORS Middleware – This page details the CORS middleware for the Express.js web application framework.
HTML5 CORS Guide – An insightful guide that comprehensively explains CORS within the scope of HTML5.
These resources provide valuable insights and practical examples to help you navigate the complexities of CORS in your projects, ensuring your web applications are secure, functional, and user-friendly.
Recap: CORS and npm in Node.js
In this comprehensive guide, we’ve navigated through the complexities of enabling and configuring CORS in your Node.js applications using npm. From understanding the basics of CORS, its significance in modern web development, to implementing it in your projects, we’ve covered a wide range of topics to equip you with the knowledge needed to handle cross-origin requests effectively.
We began by introducing CORS and its necessity for allowing secure communication between different origins. We explored how to easily enable CORS in your Node.js applications using the cors
npm package, providing you with a simple code snippet to get started. This foundational knowledge sets the stage for more advanced configurations.
Moving forward, we delved into advanced use cases, demonstrating how to apply CORS to specific routes and how to customize CORS policies for enhanced security and functionality. These intermediate-level insights enable you to fine-tune your application’s CORS settings according to your specific needs.
We also discussed alternative approaches for handling CORS, comparing the cors
package with other solutions like Helmet and manual configurations. This expert-level exploration offers you a broader perspective on CORS management, allowing you to choose the most suitable approach for your project.
Approach | Flexibility | Ease of Use |
---|---|---|
cors npm package | High | Very High |
Helmet | Moderate | High |
Manual Configuration | Very High | Moderate |
As we wrap up, it’s clear that understanding and implementing CORS is crucial for the security and functionality of your Node.js applications. Whether you’re a beginner just starting out, an intermediate developer looking to expand your knowledge, or an expert exploring alternative methods, this guide has provided you with the tools and insights needed to master CORS.
With the ability to enable, configure, and troubleshoot CORS, your Node.js applications can safely and efficiently communicate with resources across different origins. Happy coding!