Using Math.min in Java: A Detailed Tutorial
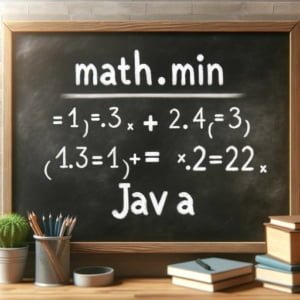
Are you finding it challenging to determine the smallest value between two numbers in Java? You’re not alone. Many developers find themselves in a similar situation, but there’s a method that can make this task straightforward.
Like a careful judge, the Math.min() function in Java can help you make the decision. This function is a handy tool that can seamlessly return the smallest of two numbers.
In this guide, we’ll walk you through the process of using the Math.min() function in Java, from the basics to more advanced techniques. We’ll cover everything from using Math.min() with different data types to alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering Math.min() in Java!
TL;DR: How Do I Find the Minimum of Two Numbers in Java?
To find the minimum of two numbers in Java, you can use the
Math.min()
function, with the syntax,int min = Math.min(firstInt, secondInt);
. This function takes two arguments and returns the smallest of the two.
Here’s a simple example:
int a = 5;
int b = 10;
int min = Math.min(a, b);
System.out.println(min);
# Output:
# 5
In this example, we’ve declared two integer variables a
and b
with values 5 and 10 respectively. We then use the Math.min() function to find the smallest value between a
and b
. The result, which is 5, is stored in the variable min
and then printed out.
This is a basic way to use Math.min() in Java, but there’s much more to learn about this function and its applications. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Math.min() in Java
- Handling Different Data Types with Math.min()
- Best Practices for Using Math.min()
- Exploring Alternatives to Math.min()
- Comparing the Methods
- Troubleshooting Common Issues with Math.min()
- Diving Deep into Java’s Math Class
- Math.min() in Real-World Applications
- Exploring Related Concepts
- Wrapping Up: Math.min() in Java
Understanding Math.min() in Java
The Math.min() function in Java is a simple yet powerful tool that can save you a lot of time and effort when dealing with numerical comparisons. The function takes two arguments and returns the smaller of the two. It’s part of the Math class, which is a built-in class in Java that provides a rich set of mathematical functions.
Let’s look at a basic example of how to use Math.min():
int x = 25;
int y = 18;
int smallest = Math.min(x, y);
System.out.println("The smallest number is: " + smallest);
# Output:
# The smallest number is: 18
In this example, we’ve created two integer variables, x
and y
, and assigned them the values 25 and 18, respectively. We then use the Math.min() function to find the smallest of the two numbers. The result is stored in the smallest
variable, which we then print out.
The advantage of using Math.min() is its simplicity and readability. It’s a straightforward, clear way to find the minimum of two numbers, and it’s easily recognizable to other developers who might read your code.
However, it’s important to note that Math.min() only works with two arguments. If you need to find the smallest number in a larger set of numbers, you’ll need to use different techniques, which we’ll cover in the ‘Advanced Use’ section of this guide.
Handling Different Data Types with Math.min()
In Java, the Math.min() function isn’t limited to integers. It can also work with other data types like ‘double’, ‘float’, and ‘long’. This flexibility allows us to use Math.min() in a variety of scenarios.
Let’s look at an example where we use Math.min() with ‘double’ data types:
double x = 25.5;
double y = 18.6;
double smallest = Math.min(x, y);
System.out.println("The smallest number is: " + smallest);
# Output:
# The smallest number is: 18.6
In this example, we’ve created two ‘double’ variables, x
and y
, and assigned them the values 25.5 and 18.6 respectively. We then use the Math.min() function to find the smallest of the two numbers. The result is stored in the smallest
variable, which we then print out.
The same process applies to ‘float’ and ‘long’ data types. It’s important to use the correct data type for your needs, as each one has its own range of values and precision.
Best Practices for Using Math.min()
When using Math.min(), it’s crucial to ensure that both arguments are of the same data type. If they’re not, Java will automatically convert the smaller data type to the larger one, which can lead to unexpected results. For example, if one argument is an ‘int’ and the other is a ‘double’, the ‘int’ will be converted to a ‘double’ before the comparison is made.
Always check the data types of your variables before using them with Math.min() to ensure accurate results.
Exploring Alternatives to Math.min()
While Math.min() is a handy function for finding the smallest of two numbers, Java offers other methods that can achieve the same result. Let’s explore some of these alternatives and their advantages and disadvantages.
Using Conditional Statements
One of the most straightforward alternatives to Math.min() is using a simple ‘if-else’ conditional statement. Here’s an example:
int x = 25;
int y = 18;
int smallest;
if (x < y) {
smallest = x;
} else {
smallest = y;
}
System.out.println("The smallest number is: " + smallest);
# Output:
# The smallest number is: 18
In this code, we compare x
and y
using an ‘if’ statement. If x
is less than y
, x
is assigned to smallest
; otherwise, y
is assigned to smallest
.
Leveraging the Ternary Operator
Java provides a more compact way to write ‘if-else’ statements known as the ternary operator. Here’s how you can use it to find the minimum of two numbers:
int x = 25;
int y = 18;
int smallest = (x < y) ? x : y;
System.out.println("The smallest number is: " + smallest);
# Output:
# The smallest number is: 18
The ternary operator checks whether x
is less than y
. If true, it assigns x
to smallest
; otherwise, it assigns y
to smallest
.
Comparing the Methods
Method | Advantages | Disadvantages |
---|---|---|
Math.min() | Simple and clear | Limited to two numbers |
Conditional Statements | Can handle more than two numbers | More verbose |
Ternary Operator | Compact and can handle more than two numbers | May be harder to read for beginners |
In conclusion, while Math.min() is a straightforward method for finding the minimum of two numbers, conditional statements and the ternary operator provide more flexibility and can handle more complex scenarios. Choose the method that best fits your needs and coding style.
Troubleshooting Common Issues with Math.min()
While Math.min() is a powerful function, it’s not immune to issues, especially when dealing with special cases. Let’s discuss some common issues and their solutions.
Dealing with NaN Values
NaN stands for ‘Not a Number’. If either of the arguments passed to Math.min() is NaN, the function will return NaN.
double x = Double.NaN;
double y = 18;
double smallest = Math.min(x, y);
System.out.println("The smallest number is: " + smallest);
# Output:
# The smallest number is: NaN
In this example, we passed a NaN value and an integer to Math.min(). As a result, Math.min() returned NaN.
Handling Negative Numbers
Math.min() can handle negative numbers. It correctly identifies the smallest number, even if it’s negative.
int x = -5;
int y = 10;
int smallest = Math.min(x, y);
System.out.println("The smallest number is: " + smallest);
# Output:
# The smallest number is: -5
In this case, Math.min() correctly identified -5 as the smallest number.
Best Practices and Tips
- Check for NaN values: Before passing values to Math.min(), ensure they’re valid numbers. If there’s a chance of encountering NaN values, add checks to handle them.
Remember that negative numbers are smaller than positive numbers: When dealing with negative numbers, remember that they’re considered smaller than all positive numbers.
Ensure consistent data types: When comparing numbers of different data types, Java will automatically convert the smaller data type to the larger one, which can lead to unexpected results. Always ensure your data types are consistent when using Math.min().
Diving Deep into Java’s Math Class
To understand how Math.min() works, it’s essential to have a solid grasp of the Math class in Java. The Math class is part of java.lang package and provides a collection of static methods that perform mathematical operations.
Unpacking the Math Class
The Math class includes methods for basic mathematical operations like absolute value, square root, and trigonometric functions. It also provides constants such as PI and E.
double pi = Math.PI;
System.out.println("Value of PI is: " + pi);
# Output:
# Value of PI is: 3.141592653589793
In this example, we’re accessing the constant PI from the Math class and printing its value.
Understanding Data Types in Java
Java is a statically-typed language, which means the type of every variable must be declared explicitly. Java supports several data types, including ‘int’, ‘double’, ‘float’, and ‘long’. Each data type has a different range of values it can represent and a different level of precision.
For example, an ‘int’ can hold a whole number between -2^31 and 2^31-1, while a ‘double’ can hold a floating-point number with up to 15 decimal places.
int myInt = 10;
double myDouble = 10.99;
System.out.println("myInt: " + myInt);
System.out.println("myDouble: " + myDouble);
# Output:
# myInt: 10
# myDouble: 10.99
In this code, we’ve declared an ‘int’ variable and a ‘double’ variable. We then print out their values.
Understanding data types is crucial when using Math.min(), as the function behaves differently depending on the types of its arguments. As we discussed earlier, if the arguments are of different types, Java will automatically convert the smaller type to the larger one before making the comparison.
Math.min() in Real-World Applications
The Math.min() function isn’t just a theoretical concept confined to textbooks. It has practical applications in various domains like game development and data analysis.
For instance, in game development, Math.min() can be used to limit a player’s score to a maximum value or to determine the lowest score. Similarly, in data analysis, it can be used to find the minimum value in a dataset, which is a common requirement in statistical analysis.
Exploring Related Concepts
After mastering Math.min(), you might want to explore related concepts in Java. For instance, Math.max() is a function that works similarly to Math.min() but returns the maximum of two numbers. It’s equally simple to use and can be invaluable in different scenarios.
Conditional statements, which we touched on earlier, are another essential part of Java. They allow your program to make decisions based on certain conditions, and they’re a key part of any programming language.
Further Resources for Mastering Java
To deepen your understanding of Java and its mathematical functions, consider exploring the following resources:
- Use Cases Explored: Math Class in Java – Discover how Java’s Math class simplifies complex mathematical tasks.
Java BigDecimal Overview – Explore BigDecimal in Java for precise numerical calculations with arbitrary precision.
Math.abs() in Java – Explore Math.abs() in Java, a method for finding the absolute value of a number.
Oracle’s Java Documentation covers everything from basics to advanced topics.
GeeksforGeeks’ Java Programming tutorials offers a wealth of info Java’s mathematical functions.
Codecademy’s Learn Java Course offers hands-on practice and teaches you how to code in Java from scratch.
These resources should give you a solid foundation in Java and its mathematical functions. Happy coding!
Wrapping Up: Math.min() in Java
In this comprehensive guide, we’ve delved deep into the world of Java’s Math.min() function, a simple yet powerful tool for finding the smallest of two numbers.
We began with the basics, understanding how to use Math.min() with integers. We then explored how to handle different data types like ‘double’, ‘float’, and ‘long’ while using Math.min(). We also covered alternative approaches, such as using conditional statements and the ternary operator, providing you with a broader understanding of how to find the minimum value in Java.
Along the way, we tackled common issues you might face when using Math.min(), such as dealing with NaN values and negative numbers, and provided solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Math.min() | Simple and clear | Limited to two numbers |
Conditional Statements | Can handle more than two numbers | More verbose |
Ternary Operator | Compact and can handle more than two numbers | May be harder to read for beginners |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up on your skills, we hope this guide has given you a deeper understanding of the Math.min() function and its applications.
With the knowledge you’ve gained from this guide, you’re now well-equipped to handle a variety of scenarios that require finding the minimum value in Java. Happy coding!