How to Find Length of String: Bash Script Reference Guide
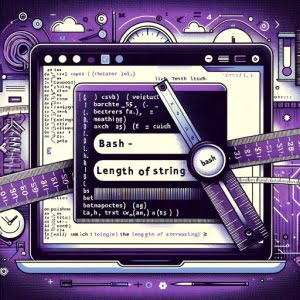
Are you finding it challenging to determine the length of a string in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling string length in Bash, but we’re here to help.
Think of Bash’s string length feature as a tailor’s tape measure – it allows us to accurately measure the length of a string, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of finding the length of a string in Bash, from the basics to more advanced techniques. We’ll cover everything from the simple ${#string}
syntax to handling strings with special characters and even alternative approaches.
Let’s get started and master the art of finding string length in Bash!
TL;DR: How Do I Find the Length of a String in Bash?
In Bash, you can use the
${#string}
syntax to find the length of a string. This simple yet powerful feature allows you to quickly and accurately measure the length of any given string.
Here’s a quick example:
string='Hello, World!'
echo ${#string}
# Output:
# 13
In this example, we’ve defined a string variable string
with the value ‘Hello, World!’. We then use the ${#string}
syntax to find the length of the string. The output is ’13’, which is the length of the string including spaces and punctuation.
This is just the tip of the iceberg when it comes to handling strings in Bash. Continue reading for a more detailed explanation, advanced usage scenarios, and alternative approaches to find the length of a string in Bash.
Table of Contents
- The Basics: Finding String Length in Bash
- Advanced Techniques: Handling Variables and Special Characters
- Expert Techniques: Alternative Methods to Find String Length in Bash
- Troubleshooting String Length in Bash
- Understanding Bash Scripting and Strings
- Bash Scripting: Beyond String Length
- Wrapping Up: Mastering String Length in Bash
The Basics: Finding String Length in Bash
The primary way to find the length of a string in Bash is by using the ${#string}
syntax. This simple yet powerful command is the cornerstone of string length manipulation in Bash.
Let’s dive into an example:
string='Bash scripting is fun!'
echo ${#string}
# Output:
# 21
In this example, we’ve defined a string variable string
with the value ‘Bash scripting is fun!’. We then use the ${#string}
syntax to find the length of the string. The output is ’21’, which is the length of the string including spaces and punctuation.
Understanding the ${#string}
Syntax
The ${#string}
syntax in Bash is a special parameter expansion. The #
tells Bash to find the length of the variable following it, in this case, string
. This is a powerful feature of Bash scripting that allows you to manipulate and analyze strings with ease.
Advantages of the ${#string}
Syntax
Using the ${#string}
syntax for finding string length in Bash has several advantages. It’s simple, straightforward, and doesn’t require any external tools or commands. This makes it a highly efficient and reliable method for handling string length in Bash.
Potential Pitfalls
While the ${#string}
syntax is incredibly useful, it’s important to remember that it counts all characters in the string, including spaces and punctuation. This can lead to unexpected results if you’re not careful. Always ensure you’re considering all characters when working with string lengths in Bash.
Advanced Techniques: Handling Variables and Special Characters
As you become more comfortable with Bash scripting, you’ll encounter scenarios that require a more nuanced approach to finding string length. Two such situations involve working with strings stored in variables and handling strings with special characters.
Finding Length of a String Stored in a Variable
In Bash, you can store a string in a variable and then find its length. This is particularly useful when working with dynamic data. Here’s an example:
name='Anton'
message="Hello, $name!"
echo ${#message}
# Output:
# 12
In this example, we’ve stored the string ‘Anton’ in the variable name
. We then use this variable to construct a greeting message and store it in the message
variable. Using the ${#message}
syntax, we find that the length of our greeting message is 12.
Dealing with Special Characters
When your string includes special characters, Bash still counts them as part of the string length. Let’s see how this works:
special_string='Hello, $name!'
echo ${#special_string}
# Output:
# 14
In this example, even though $name
is a variable, it’s enclosed in single quotes. This makes Bash treat it as a literal string, not a variable. Thus, the length of the string is 14, including the $
, n
, a
, m
, e
characters.
Understanding these advanced techniques will equip you with the skills to handle more complex scenarios in Bash scripting, enhancing your ability to manipulate and analyze strings.
Expert Techniques: Alternative Methods to Find String Length in Bash
While the ${#string}
syntax is the most straightforward way to find the length of a string in Bash, there are alternative methods you can use. These alternative approaches can come in handy in certain scenarios or when you’re working with different tools.
Using External Tools: The wc
Command
The wc
(word count) command is a versatile tool in Unix-like operating systems that can count words, lines, characters, and bytes. With the -m
option, wc
can count the number of characters in a string.
Here’s an example:
string='Hello, Bash!'
echo -n $string | wc -m
# Output:
# 12
In this example, we pipe the string into wc -m
, which then counts the number of characters in the string. The -n
option with echo
is used to prevent a new line character from being added to the end of the string, which wc
would count as an additional character.
While this method is slightly more complex, it can be useful when you’re already working with wc
or other Unix tools.
Creating a Function to Find String Length
If you frequently need to find the length of strings in your Bash scripts, you might find it useful to create a function to do this. Here’s how you can do it:
function string_length {
string=$1
echo ${#string}
}
string_length 'Hello, Bash!'
# Output:
# 12
In this example, we’ve created a function called string_length
that takes a string as an argument and prints its length. This can be a handy tool in your Bash scripting toolbox, especially when working on larger scripts or projects.
These alternative approaches provide additional flexibility when finding the length of a string in Bash. Depending on your specific needs and the tools you’re working with, you might find one method more suitable than the others.
Troubleshooting String Length in Bash
While working with Bash and finding the length of a string, you may encounter some common issues. Let’s discuss these potential roadblocks and how to navigate around them.
Special Characters and Whitespaces
A common issue arises when your string contains special characters or whitespaces. Bash counts these as part of the string length, which can lead to unexpected results.
Consider this example:
special_string='Hello, $name!'
echo ${#special_string}
# Output:
# 14
In this example, even though $name
is a variable, it’s enclosed in single quotes. This makes Bash treat it as a literal string, not a variable. Thus, the length of the string is 14, including the $
, n
, a
, m
, e
characters.
Uninitialized Variables
Another common issue is trying to find the length of an uninitialized variable. Bash will return 0 in such cases.
unset string
echo ${#string}
# Output:
# 0
In the above example, we unset the variable string
and then try to find its length. Bash returns 0, indicating that the variable is uninitialized or empty.
Strings with Newlines
If your string contains newline characters, Bash will count them as part of the string length. This can lead to unexpected results if you’re not aware of it.
string_with_newline="Hello,\n World!"
echo -n $string_with_newline | wc -m
# Output:
# 13
In this example, the string contains a newline character (represented by \n
). The wc -m
command counts this as a character, resulting in a length of 13.
Understanding these common issues and their solutions will help you work more effectively with string lengths in Bash. Remember, Bash counts all characters in a string, including whitespaces, special characters, and newline characters. Always consider these aspects when finding the length of a string in Bash.
Understanding Bash Scripting and Strings
To effectively work with string length in Bash, it’s essential to understand the basics of Bash scripting and how strings function within this environment.
Bash Scripting: A Brief Overview
Bash, or the Bourne Again SHell, is a Unix shell and command language. It’s widely used for its powerful scripting capabilities. In Bash scripting, you can create variables, use conditional statements, loops, and perform operations on strings – which brings us to our next point.
Strings in Bash: The Basics
A string in Bash is a sequence of characters. It’s one of the fundamental data types in Bash scripting, used to store and manipulate text. You can create a string by enclosing a sequence of characters in single or double quotes.
Here’s an example:
string='Hello, Bash!'
echo $string
# Output:
# Hello, Bash!
In this example, we’ve created a string variable string
with the value ‘Hello, Bash!’. We then use the echo
command to print the string.
String Length in Bash: The Importance
Finding the length of a string in Bash is a common operation, especially when dealing with text processing. It can be used to validate input, parse text, or control the flow of a script based on the length of a string.
Understanding these fundamental concepts will provide a solid foundation for mastering the process of finding the length of a string in Bash. With this knowledge, we can better appreciate the power and versatility of the ${#string}
syntax and other methods for finding string length.
Bash Scripting: Beyond String Length
Finding the length of a string is just one aspect of Bash scripting. The ability to effectively manipulate and analyze strings is a crucial skill for any Bash scripter and forms the backbone of many larger scripts or projects.
The Role of String Length in Larger Scripts
In larger Bash scripts, finding the length of a string can play a crucial role. It can be used to validate user input, parse log files, control the flow of a script, and much more. Mastering this skill will provide you with a versatile tool in your Bash scripting toolbox.
Exploring Related Concepts: String Manipulation in Bash
Beyond finding the length of a string, Bash offers a wide range of features for string manipulation. This includes concatenating strings, extracting substrings, replacing parts of a string, and much more. Here’s a quick example of string concatenation in Bash:
string1='Hello,'
string2=' Bash!'
combined_string="$string1$string2"
echo $combined_string
# Output:
# Hello, Bash!
In this example, we’ve created two string variables string1
and string2
. We then concatenate these strings by simply placing them next to each other, creating a combined string ‘Hello, Bash!’.
Understanding these related concepts will enhance your Bash scripting skills and open up new possibilities for text processing and manipulation.
Further Resources for Bash Scripting Mastery
To deepen your understanding of Bash scripting and string manipulation, consider exploring these resources:
- GNU Bash Manual: The official manual for Bash, providing comprehensive coverage of all its features.
- Bash Guide for Beginners: A beginner-friendly guide to Bash scripting, covering all the basics and more.
- Advanced Bash-Scripting Guide: An in-depth guide to Bash scripting for more advanced users, covering topics like string manipulation, regular expressions, and more.
Wrapping Up: Mastering String Length in Bash
In this comprehensive guide, we’ve delved into the process of finding the length of a string in Bash. We’ve explored the basic ${#string}
syntax, tackled common issues, and looked at alternative approaches for handling this task.
We began with the basics, learning how to use the ${#string}
syntax to find the length of a string. We then explored more advanced scenarios, such as dealing with strings stored in variables and handling strings with special characters. Along the way, we tackled common issues you might encounter when finding the length of a string in Bash, providing solutions and tips for each problem.
We also ventured into alternative approaches, learning how to use the wc
command and creating a function to find string length. These methods provide additional flexibility and can be particularly useful in certain scenarios or when working with different tools.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
${#string} Syntax | Simple, efficient | Counts all characters, including spaces and special characters |
wc Command | Useful when working with Unix tools | Slightly more complex |
Function | Handy for larger scripts or projects | Requires additional coding |
Whether you’re just starting out with Bash or you’re looking to enhance your scripting skills, we hope this guide has given you a deeper understanding of how to find the length of a string in Bash.
Understanding string length in Bash is a crucial skill for any Bash scripter. It’s a fundamental part of text processing and can play a key role in many scripts. With this knowledge, you’re well equipped to tackle any string length challenge in Bash. Happy scripting!